Introduction: DMX LED Strips
Around the perimeter of our Circus ring we have 17 fences, decorated using RGB LED strips. I wanted to control them with the rest of our lighting using DMX.
DMX is an industry standard for communicating with lighting equipment. Wiki DMX
Searching for a device that would handle this, I found most devices only catered for 1-3 strips and are quite expensive.
So I decided I could build my own. I have previously built Arduino based DMX relays and figured that it couldn't be too hard to adapt to LED strips.
This Instructable is written to help you design, build and program your own units with any changes you need.
Please visit github.com/mtongnz for the latest code and some of my other projects.
Step 1: Research
I based this project on a few different projects and libraries that I found very useful. These guys did an awesome job and meant the programming was a lot easier.
Matthias Hertel has made excellent DMX libraries and a DMX shield for Arduino. This is the library I use to receive the DMX and I used his shield schematic as the basis for the DMX receiver in my design. I opted to not provide RDM functionality as it isn't required by me.
DMX Shield
DMX Serial Library
Elco Jacobs created a library for generating PWM using shift registers. This meant that I could output 15 channels using only a few pins. you can add more outputs very easily and cheaply to my design.
ShiftPWM Library (currently his site is down)
ShiftPWM on GitHub
These were the main sources of information. I also used Google and Youtube to find small bits and tips along the way. This included how to use Eagle and how to deal with SMD parts.
Step 2: Tools and Supplies Needed
Essential Parts:
- Parts according to your final design. Find my BOM attached.
- PCB. We design and order this in the next few steps.
- Power supply (I used 12V 150W)
- Case to house everything
- LED strips and wire/plugs to connect them
Essential Tools:
- Soldering iron
- Wire cutters
- Computer for programming and PCB design
- Lighting console with DMX output
Optional (but recommended):
- Tweezers
- Breadboard and jumpers
- Arduino (I used a Nano)
- ICSP programmer
Step 3: Schematic Design
I used Eagle to design the schematic and PCB for this project. This is the first time I have used it so Youtube was very helpful. I wont go into the details as it is not the purpose of this Instructable.
My Design Process:
I wanted the board to be as compact as possible which meant I ditched the Arduino and used an ATMega328p (the processor from an Arduino Nano) with the bare minimum components required as my starting point.
Next I added the DMX receiver components. This included the DC-DC converter and an optical isolator. Removing these and connecting the MAX485 directly to the arduino will work fine for testing but I would recommend them for the final design to protect your device and others on the DMX line from excessive voltages.
The final components are the 74HC595C shift registers and FETs. I used 2x 74HC595Cs which would allow a total of 16 outputs (I need 5x RGB = 15 total).
I also put a 100nF cap on each IC, a 10uF cap on the 5V input, and a 5V power regulator with the recommended caps.
More Outputs:
More shift registers can be added if needed. Note that this will use more processing cycles and may cause issues. You can reduce the refresh frequency to counter this but don't drop it much below 50Hz or it may start to flicker. On Elco Jacobs' website, he has a calculator to determine resource usage.
Address Selection:
I don't need to change the address once these are built so there is no address selection in my design. There are heaps of Arduino inputs available so you could easily add a 10 way dip switch for this if desired.
Fuses:
I would recommend adding an smd fuse to each output. See the final step for why.
Crystal Package:
Change the crystal for a different package type - probably through hole. The package I chose was extremely hard to hand solder.
Attachments
Step 4: Programming
Please visit github.com/mtongnz for the latest code.
I used the 2 libraries mentioned in Step 1. ShiftPWM needed to be slightly modified to remove it's serial commands - they interfered with the DMX transmission.
I have included downloads for both libraries but highly recommend visiting the authors' sites. Download the libraries and import them into the Arduino IDE. To do this, unzip them into the libraries folder and restart the IDE. Google it if you have issues.
My code is very simple to follow as the libraries handle all the heavy lifting.
First set some variables. They are very self explanatory and set the options for ShiftPWM, the number of outputs we are running, and the DMX address.
As I wanted 4 continuous units, I simply had the first DMX start address and then a unit number. I found this easier than manually entering an address for each unit.
In the setup routine we start the DMX receiver, start ShiftPWM and set the outputs to off, set our status LED to on to show we have power, clear the strobe counter, and start a timer interrupt for use with our status LED and strobe.
In our timer interrupt routine we simply increase our timer and strobeCount variables. We dont want to do too much here or it will cause other parts of our code to function erratically.
In our main loop, we first set our status LED. It will blink while receiving DMX and will stay solidly lit 1 second after losing DMX.
Next we set the outputs to the required values. If we haven't received DMX for 10 seconds then all outputs are turned off. If our strobe channel is between 20 and 220 (255 max DMX value) then we apply the RGB values in bursts to the outputs - 20 is slow strobe, 220 is fast strobe. If our strobe channel is out of this range then we simply apply the RGB values to the outputs.
I plan to add a random strobe but have not had time yet.
Attachments
Step 5: Breadboard Prototype
I did this step in combination with the Schematic Design and Programming step.
Note that the photo is not the unit I used - it may be incorrect as I didn't test it. I forgot to take a photo of it so I quickly put it back together. My large bread board is in use for another project which is why its so cramped.
Connect all your components up on a breadboard according to your schematic. You'll need through-hole components here so you may need to do some substitutions if you've used SMD in your design as I have.
My Substitutions:
- Arduino Nano instead of a bare 328p for ease of use on the bread board.
- Standard red LEDs instead of full RGB strips and FETs. I also only used a few LEDs to keep jumper wire spaghetti at bay. I changed which output the jumpers attached to in order to test all the outputs.
- No DMX isolation circuitry to make things a bit simpler since I have already used the same circuitry before and know it works.
Program and Test:
Flash your sketch to your Arduino and test everything out. If it all works as expected, you can start on your PCB.
You can also use this prototype to add or change any programming while you wait for your PCB to arrive.
Step 6: PCB Layout
Once again, I will not explain how to use Eagle as there are plenty of brilliant tutorials and videos already available.
This was one of the more difficult steps. Trying to get everything to fit while using the minimum number of vias is a challenge. It took me 4 attempts before I settled on my final design.
For the final design, I started by placing the shift registers as they had the most traces coming off them. Once they were in place with all the outputs along the top of the board, everything else just fell into place.
I kept the dmx circuitry away from the power lines to minimize interference. I don't think it would be an issue but I didn't want to find out.
Note that my design needs to be tweaked to allow the correct plugs to fit. As I found this after the boards were made, I just used normal headers which works fine. It would be better if I had the Molex plugs for power to the board. It will be annoying having to de-solder the power lines if I need to remove the board. I decided these issues are not major enough to warrant getting the boards remade.
I also made a slight mistake in the wiring of the MAX485, hence the wire across it in the photo. As I said earlier.... check everything over and over :) This is fixed in the design files I've uploaded.
Attachments
Step 7: Export Gerbers and Order PCBs
I found this tutorial helpful with this.
I ordered my boards from Seeed Studios using their Fusion PCB service. I found it to be cheap, the boards are a decent quality and it only took a few weeks to arrive. They have a minimum order of 5 boards. There are plenty of other manufacturers you can use.
Run your manufacturer's DRC (Design Rules Check) in Eagle. This ensures they can actually make what you have designed.
Now you need to generate the required Gerber files and a drill file. It's easiest to download their Gerber generation file if they have one (Seeed Studios does in their help). In Eagle, select File > CAM Processor while in PCB view. Open the Gerber generation job file and create your Gerber and drill files. If you don't have a job file, you'll need to manually configure which files to make (check with your manufacturer).
Ensure you have all the files your manufacturer needs, zip them and send them to your manufacturer. Seeed Studios has an online form you fill out with all the options you would like on your board and an upload function.
Step 8: Soldering
Start with your smallest components and work your way up.
I started with the resistors and caps. Then the crystal and AVR. Then the through-hole components. And finally all the headers. Make sure you place your components in the correct orientation.
Your tweezers will be a massive help here. I also found using helping hands or blue tack helpful to hold the board while you solder.
Step 9: Flashing the Board
Note that the board will need 5V for these steps. In my design, I use a 12-5V DC-DC converter for efficiency and cost reasons.
I made the case and wired it first as the unit pictured was the second unit I made.
If you opt to use a full blown Arduino in your final design, simply program it from the IDE as you normally would. You can skip the rest of this step.
Software and ICSP Programmer:
To keep cost and size down, I decided to use ICSP (in circuit serial programming) to flash my board rather than the normal USB to serial used on Arduino boards. This requires a separate programmer (mine uses USBasp).
Follow this tutorial to set up your Arduino IDE, AVRFuses and your USBasp. This is for Macs but there is similar software available for Linux and Windows with plenty of tutorials to help.
You could also use another Arduino to do this if you wish. I am not sure if you can set the fuses using this method as I haven't tried it.
Set the Fuses:
I found setting fuses one at a time to be the best way. Be careful with this step as you can render your processor useless if you flash the wrong bits.
Low Fuse: 0xFF
High Fuse: 0xDA
Extended Fuse: 0×05
Flash the HEX File:
In the Arduino IDE, save your sketch and click the Verify tick button as you did in the tutorial above. Now select your hex file in AVRFuses and click program.
You can also select USBasp as the programmer in the Arduino IDE then select Flash with Programmer from the file menu. I found this after finishing this project. It's much easier than using a separate program to flash the HEX while you fine tune your code.
Step 10: Power Supply
The power supply you select will vary depending on your design. I chose to use LED strip with 30ppm in order to reduce power requirements and heat. Most LED strip is 60ppm. Check the requirements for your strips.
I have 5 LED strips, each about 4.5m with 30 LEDs per metre, so about 130 LEDs per strip. Each 5050 LED uses 0.24A at 12V on full white.
5 strips * 130 LEDs * 0.24A / 12V = 13A
I'd recommend leaving 10% or more to allow for resistance in cables and the strip itself. I chose a 15A 12V supply.
5V Supply:
I opted to use a 12-5V DC-DC converter to power my circuit. I found some on eBay for about $2 each.
Step 11: Test It Out
Your board is now complete and ready for it's first test run.
Connect your 5V and 12V supplies and a small section of LED strip to the board and run the output_test file. I used a full roll - it got quite hot and melted the reel it was on so dont do that. It should flash red, green, blue, white. If there are any outputs not working, check your soldering and schematics then try again.
If all outputs function correctly, flash the final_code sketch and connect your DMX. Turn it on and send some DMX values. Hopefully you should see your LEDs doing what you want from them.
Troubleshooting:
Try flashing a sketch which eliminates the DMX like output_test. If all the outputs work as expected, it's a dmx issue - see below for some ideas to narrow the issue further.
- All ICs have 5V power.
- Dry solder joints. I found that I got sporadic flashing on one unit after running it for over 2 hours. It turned out to be a dry joint on one of the shift registers.
- Silly errors in code, schematic or construction including component orientation.
- Check PCB traces are all fine using a continuity tester.
If your unit is still not working, check each component's data sheet. Ensure you have them wired correctly, supplied with the correct voltage and all other IO pins are connected correctly. Going back to the breadboard may be helpful. You may also have a defective component - but this is unlikely unless you have supplied an incorrect voltage to it.
Troubleshooting DMX:
- Ensure the DMX address is correct. If you've added a method of entering an address (eg. DIP switches), try by-passing this by hard coding a value in your sketch.
- DMX polarity - try changing pins 2&3.
- Check MAX485 has power and is connected correctly.
- Try bypassing OK1. If this is the issue, ensure OK1 is connected correctly.
- Check the DMX source works with a known working fixture.
Step 12: Box It Up
Now that your electronics are complete and working, it's time to put it in a case.
Warning:
In this step, I remove the PSU case and modify it. If you do this, be super careful as there are voltages in there that will kill you. The caps can also store huge amounts of power when everything is unplugged. Treat it as live at all times and don't touch anything metal in there. I highly recommend you seek assistance from a suitably qualified or experienced person if you wish to open the PSU. It may also be illegal to make certain changes in some countries.
I chose to use a jiffy box from Jaycar. I found one that just fits the power supply. This meant I had to remove the mesh cage and cut some excess off the PSU to fit the other electronics in. I removed the entire PCB from the PSU before I made any cuts. You don't want metal filings in the PSU when you turn it on so ensure they are all cleaned away before you put it all back together. I removed as little as possible as it is used as a heatsink.
Once I had made everything fit, I cut holes for all the plugs, hot glued everything in place, and wired it all up.
Make it so it opens easily. This way you can reprogram it and repair it without hassle should the need arise.
Step 13: Final Installation
Now you can set everything up, program your console, then sit back and watch the show.
After initially testing everything worked, I left the units on full white for 3 hours, constantly checking the case temperature. They never got too hot... about 50C with 25-30C ambient. That is fine as all components can handle over 85C. This was great as I didn't want to add a heatsink or fan.
This intensive 3 hours of white caused one issue to show. One of the units had a random flicker/strobe on outputs 4 & 5 when I changed the colour to blue or red. As both outputs are on the same shift register, that is where I started troubleshooting. I figured it would be a dry solder joint on the register. Instead of doing a full inspection, I simply re-soldered both registers and ran the test again. This fixed the issue as expected.
I have been using 4 of these units to control 17 LED strips for the past few weeks without any further issues.
Step 14: What I'd Do Differently
Make the pcb larger so the sockets fit better. I would also print it at full scale prior to sending away for manufacture to ensure everything fit.
Add fuses to each output. I stupidly cut one strip while it was plugged in and going. This fried a number of the transistors and a couple of traces. This showed that if anything shorts, it will most likely destroy that output.
Use all SMD components (except crystal). I used through-hole only because I had them in stock already but smd would look so much better.
Use a through-hole crystal for easier soldering.
Consider adding RDM support, dip switches or screen/buttons for address and function changing. I left these out for simplicity, cost and I don't need to change address often (maybe once a year).
Scrap the multi colour status LED. I ended up only using a standard LED so it's a waste of space. I'd also add a header for it so it can mount on the case.
Add random strobe functionality. This is in software so I may still implement this. I would use a random period between bursts but the same duration.
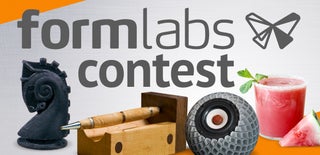
Finalist in the
Formlabs Contest
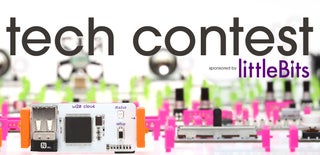
Participated in the
Tech Contest
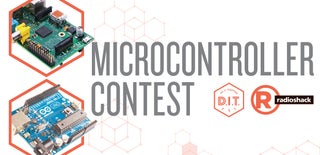
Participated in the
Microcontroller Contest