Introduction: Develop for the ESP8266 on the Raspberry Pi
This article will show how to use a Raspberry Pi 2 to develop native applications, firmware and Lua scripts for the ESP8266 family of microcontrollers. The ESP8266 is a small WiFi enabled micro-controller that is becoming extremely popular for DIY projects due to it's high-speed, low cost and the ease of developing for the platform.
While generally set-up on desktop systems, the esp-open-sdk builds and runs quite well on the Raspberry Pi 2. This allows us to use it as an inexpensive (less than $40) development environment to write and flash native firmware and apps to ESP modules as well as write and edit Lua code.
It should be noted that this is one of several alternatives for ESP8266 development. Other alternatives include-
- flashing nodemcu-firmware on the ESP and using ESPlorer from Windows (or Linux or MacOS)
- using the Arduino IDE add-on to write and load applications on the ESP8266 from Windows
- set up the sdk on Windows using the instructions and files created by CHERTZ
- setting up the esp-open-sdk on a Linux desktop/laptop
This instructable will go through how to set up the development environment, the SDK and the Eclipse IDE. What that means is that we will be installing a cross-compiler to build native code for the xtensa-lx106-elf platform. It sounds complicated. Luckily the esp-open-sdk Makefile and crosstools takes care of most of the details for us.
We will also use our new Raspberry Pi cross-compiler to build and flash the latest nodemcu-firmware to the ESP and show how to connect to the NodeMCU firmware with ESPlorer.
Lets' Get Started! (This tutorial does assume some familiarity with linux, Raspbian OS and with the ESP8266 family of microcontrollers. It's also a work in progress. I'll be adding more information and photos as I organize it...)
Step 1: Step 1: Set Up Raspbian OS
The first thing you'll need to do (unless you're installing into an existing environment) is download ind install the latest Raspbian OS onto onto your SD card. If this is a new install you'll need to expand the filesystem and set your locale/timezone/keyboard using raspi-config. More information on how to do that can be found here.
Once that is complete open a terminal and enter the following commands-
sudo apt-get update
sudo apt-get dist-upgrade
sudo apt-get install build-essential srecord make unrar-free autoconf automake libtool-bin gcc g++ gperf flex bison texinfo gawk ncurses-dev libexpat-dev python python-serial sed git unzip
Once this is complete, you have successfully set up the OS and required prerequisites.
Step 2: Step 2: Get and Build the Esp-open-sdk
The next step is to download and install the sdk source. To do that enter the following terminal commands-
cd /opt
sudo git clone --recursive https://github.com/pfalcon/esp-open-sdk.git
chown -R pi:pi /opt/esp-open-sdk
cd esp-open-sdk
Here is where we can edit the Makefile and choose our vendor SDK version. (At the time of this writing the current version is 1.5.2) . If in doubt leave it be. Once you are done, go back to the terminal and type -
make
The build takes around 2 hours so this is a good time to go get a coffee.
Step 3: Step 3: Set Up the PATH
Now the esp-open-sdk is installed. In order to use it, the files need to be in your PATH. To do that you can either edit the file /home/pi/.profile in an editor (photo) or just open a terminal and type the following commands-
cd ~
echo "/opt/esp-open-sdk/xtensa-lx106-elf/bin:$PATH" >> .profile
echo "/opt/esp-open-sdk/esptool:$PATH" >> .profile
Now you can log off (or reboot) and the cross compiler will be in your PATH.
Step 4: Step 4: Test the Compiler
Now that we've got the toolchain installed, he next step is to test it. (Note that this is optional but highly recommended. First we download the code examples. Open a terminal and enter the command-
git clone https://github.com/esp8266/source-code-examples.git
Now, in your favorite text editor, open the Makefile for the 'blinky' project ( at ./sourcecode-examples/blinky/Makefile) and-
- Change the XTENSA_TOOLS_ROOT variable to /opt/esp-open-sdk/xtensa-lx106-elf/bin
- Change the SDK_BASE variable to /opt/esp-open-sdk/sdk
Now save the Makefile and go back to the terminal and do-
cd source-code-examples/blinky
make
If all goes well the make should complete without error. If you run into issues-
- check your PATH (echo $PATH) to make sure he cross compiler is in it
- check the 'blinky' Makefile to verify the paths to the tools and SDK are correct
Now you can flash the example to an ESP module. This varies from module to module but generally you will-
- connect the ESP module to a serial adapter being careful to only supply 3.3VDC to the ESP8266 +V pin (some serial adapters have a separate 3.3V pin, others a jumper, see photo for examples of both)
- boot the ESP with it's GPIO0 pin grounded
- now plug the UART adapter into your Raspi USB port
- from the 'blinky' project terminal enter the command sudo make flash
If successful you should see the progress of the firmware push on the command prompt. You can test the program by connecting a red LED and 1K resistor in series between GPIO2 and ground like this. The LED should blink when you boot the ESP8266.
Step 5: Additional Tools: Eclipse IDE and ESPlorer
Now that you've got a toolchain to develop your own ESP8266 firmware, the next thing you will want is an IDE. I recommend Eclipse. Installing it is easy. Just open a command prompt and enter-
sudo apt-get install eclipse-cdt
It'll take a while to download and install. But at the end you'll have one of the most useful and robust IDEs available anywhere!
For Lua development on the ESP8266 the other tool you'll want right away is ESPlorer. The only requirement for it is Java (which is installed by default anyway...) To install ESPLorer -
- Download the ESPlorer.zip package from it's homepage here (Big Blue Download Button) and place the file in your home directory on the pi
- In a terminal and execute the following commands
- cd ~
- unzip ESPlorer.zip
- sudo mv ESPlorer /opt/
- sudo chown -R pi:pi /opt/ESPlorer
That's it! To run ESPlorer simply open a terminal and run -
java -jar /opt/ESPlorer/ESPlorer.jar
To connect to an ESP8266 with nodemcu on it, just plug it in, choose your serial speed (chosen at compile time) and click the connect button. You should see the lua prompt and possibly file system or heap data depending on your firmware. (see photo)
You can use ESPlorer to upload lua scripts and run commands on the ESP8266 interactively. There's a really good article on using ESPlorer, lua and the ESP8266 here.
Step 6: Bonus: Roll Your Own NodeMCU Firmware!
Note - This is a more advanced example and assumes some familiarity with the ESP8266, NodeMCU, LUA and ESPlorer. In short, if you don't know what this is, you probably don't need it. ;-)
One of the neat things about the ESP8266 is the existence of the nodemcu-firmware project. This is an open-source firmware for the ESP8266 which allows one to write interpretive code in the lua language and upload it to the ESP8266 using the ESPlorer app we set up in the previous step. You might already be familiar with it. Most people download and flash pre-compiled versions of this onto their ESP modules.
But now that we have a working development environment we can actually 'roll our own' version of this firmware! ( Reasons to do this include to to get the latest updates and bug-fixes and/or to increase stability and reduce memory usage by only including the modules we're actually going to use. )
First we get the source code. Open a terminal and enter the following commands-
cd /opt
sudo git clone https://github.com/nodemcu/nodemcu-firmware.git
chown -R pi:pi /opt/nodemcu-firmware
cd nodemcu-firmware
Before you build the firmware you'll want to configure your set-up.
- edit the file /opt/nodemcu-firmware/app/include/user_config.h to set the default serial baud by setting BIT_RATE_DEFAULT (mine is set to BIT_RATE_115200) (You can also set the flash size of your module here if it is not autodetected during flash.)
- edit the file /opt/nodemcu-firmware/app/include/user_modules.h to choose the modules you will include. enable only what you need. Choosing too many can make your system unstable.
Once you have edited your configuration, you can build by going back to the terminal form earlier and from the /opt/nodemcu-firmware directory entering the command-
make
Flashing is exactly like the 'blinky' example, just hook up the UART adapter and boot the ESP8266 with GPIO0 grounded, then do-
make flash
A succesful flash looks like the photo above. Once you have flashed your module you will want to test it. The easiest way to do this is to connect to it via ESPlorer and throw some test commands to the Lua interpreter. There are some good Lua samples to get started on here.
If you've followed all of these steps your Raspberry Pi is now configured as a budget development environment to create applications for the ESP8266. Happy Coding and thanks for reading!
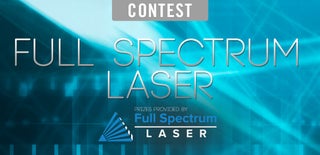
Participated in the
Full Spectrum Laser Contest 2016
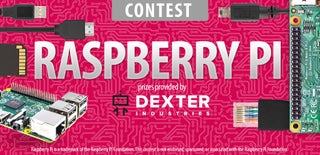
Participated in the
Raspberry Pi Contest 2016