Introduction: Distance and ADC Sensing Device
This a device which consists the Raspberry Pi and the Arduino and a number of external components to used to measure the distance of the object from the Infra-Red sensor, the ADC and have its external components respond according to the distance of the object from the Infra-Red sensor.
Step 1: Contruction
Other than having a Raspberry Pi (3) and an Arduino UNO, you will also need:
- 1 RGB LED
- 1 Infra Red Sensor
- 1 Laser emitter
- 1 Buzzer
- 1 Breadboard
- An assortment of wires
Once you have all of the materials, construct the circuit according to the diagram shown above.
Step 2: Coding the Arduino UNO
Before beginning this section, make sure you have the following:
- A computer/laptop (You will be using this for coding of course!)
- Arduino App installed in your computer/laptop
- A Micro USB cable (For you computer to communicate from the Arduino UNO)
- Once you have all these ready, we can begin focusing on programming the Arduino!
- Connect the Micro USB cable from the Arduino UNO to the Computer
- Open the Arduino App in your computer
- Around the top left corner of the app, click on "tools" > "port" > the port number that you plugged the USB into. (This is to tell the app which USB port your Arduino UNO is connected to!)
Now copy and paste the following code into the sketch:
#include
#define SLAVE_ADDRESS 0x40 int AVERAGING_LOOP_SIZE = 50; int READ_PIN = 0; // Analogue pin int laser = 8; int redPin = 9; int greenPin = 10; int bluePin = 11; int buzzer = 12;
int DISTANCE_BUFFER = 1; //cm int MIN_DISTANCE = 7; // cm int MAX_DISTANCE = 60; // cm int IGNORE_BUFFER_THRESHHOLD = 35; // Readings int thisDistance, lastDistance, ignoreBuffer, val; String thisPrint, lastPrint; char dist[8]; char value[8];
void setup() { pinMode(READ_PIN, INPUT); Serial.begin(9600); ignoreBuffer = 0; Wire.begin(SLAVE_ADDRESS); pinMode(laser, OUTPUT); pinMode(buzzer,OUTPUT); pinMode(redPin, OUTPUT); pinMode(greenPin, OUTPUT); pinMode(bluePin, OUTPUT); }
void loop() { val = 0; // Averaging Loop for (int i = 0; i < AVERAGING_LOOP_SIZE ; i++) { { val += analogRead(READ_PIN); } } val = val / AVERAGING_LOOP_SIZE;
thisDistance = (6762 / (val - 9)) - 4;
if ((thisDistance < MIN_DISTANCE) || (thisDistance > MAX_DISTANCE)) { thisDistance = 0 ; val = 0; thisPrint = "No Object"; } else if ((thisDistance < lastDistance + DISTANCE_BUFFER) || (thisDistance > lastDistance - DISTANCE_BUFFER)) { ignoreBuffer ++; if (ignoreBuffer > IGNORE_BUFFER_THRESHHOLD) { thisPrint = "Object is now at: " + String(thisDistance) + "cm."+val; lastDistance = thisDistance; ignoreBuffer = 0; } }
if (thisPrint != lastPrint) { Serial.println(thisPrint); lastPrint = thisPrint; }
if (thisDistance <= 10 && thisDistance != 0){
tone(buzzer,1000); delay(100); noTone(buzzer); delay(1000);
setColor(255, 0, 0);
}
else if (thisDistance <= 15 && thisDistance != 0){ digitalWrite(laser,HIGH); delay(500); digitalWrite(laser,LOW);
setColor(220, 20, 0); }
else if (thisDistance <= 20 && thisDistance != 0){
setColor(255, 248, 0); }
else if (thisDistance < 30 && thisDistance != 0){
setColor(60, 200, 0);
}
else if (thisDistance >= 30 && thisDistance != 0){
setColor(0, 255, 0); }
else{ setColor(0, 0, 0); }
// Convert to character array // Length is 4 + decimal point = 5 dtostrf(thisDistance, 3, 0, dist); dtostrf(val,3,0,value);
// Send telemetry data to Pi Wire.onRequest(sendDataToPi); }
void setColor(int red, int green, int blue) { analogWrite(redPin,red); analogWrite(greenPin,green); analogWrite(bluePin,blue); }
void sendDataToPi() { Wire.write(dist); Wire.write(value); // Length is 5 bytes
}
*END OF CODE, DO NOT COPY
Now press the upload button (Displayed as an arrow around the top left corner) to upload to the Arduino UNO for execution!
Observe the output by placing an object in front of the IR sensor at different distances (at least from 7cm to 60cm!)
Also study the code see what does it do.
Step 3: Coding Windows Studio 2015
Now that our Arduino is completed, we shall proceed to programming the Raspberry Pi using Microsoft's Windows Studio 2015. The purpose of doing this to configure the Raspberry Pi is to collect data from the Arduino and then display measurements through its UI.
Before starting you will need:
- A pair for Ethernet cables
- 1 Switch
- Have Windows 10 iot core install in your Raspberry Pi.
If you are new to using Windows Studio 2015 for projects like these follow these steps:
- Click "File" > "New" > "New Project"
- In the project creator make sure change the framework to ver. 4.5.1
- On the left side, select Visual C# > Windows > Universal
- Select "Blank App"
- Name the project and create it
Download the attached Project and transfer the codes to your project. (One for XAML and one for XAML.CS)
Set the Raspberry Pi as your deployment target and deploy.
Observe the Raspberry Pi's UI and the code in Windows Studio 2015.
That concludes the instructions
Thank you!
Attachments
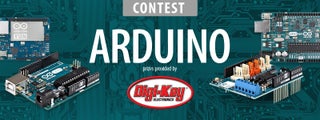
Participated in the
Arduino Contest 2016
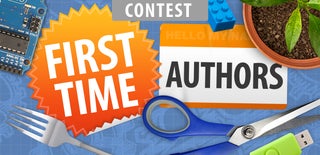
Participated in the
First Time Authors Contest 2016