Introduction: ElecTool - a DIY All-in-one Handy Hardware Tool That Measures Inclination, Height and Has Inbuilt Stroboscope
Inspiration
Being engineering students, we realized how in industries and several other aspects of work, a person and especially an engineer needs to use different tools for the sole purpose of measuring. Hence we thought of building a hack that is an all-in-one solution for measuring requirements, which can also come in handy to beginner electricians. Many times we hear during the drilling of holes in walls, humans get shocked by rupturing live wires. So we thought of incorporating a live wire detector that can sense live wires. Along with that, we took inspiration from theodolites that were used to measure very tall structures, to build the height measuring device.
About ElecTool
It is an electronic tool that can measure if a surface is leveled or not, measure pretty high heights using a laser pointer, the concept of theodolites, RPM of rotating objects using the stroboscopic effect. And a live wire detector can be useful for measuring live wires behind walls of concealing wiring. The purpose of Laser theodolite was to give the electricians a rough Idea of how much wire to buy for tall buildings. This can also be used by painter to estimate area of faces of buildings.
Supplies
- Arduino (pick your fav one)
- Accelerometer
- Laser
- OLED
- Push Button
- Potentiometer
- 3.7v Battery
Step 1: How We Built It
ElecTool contains an accelerometer to measure the inclination angles. The level meter was pretty straight forward it senses the inclination on the X-axis. Upon tilting the board, the acceleration due to the gravity vector changes at an angle phi. Along with that using some trigonometry, we find the exact angle. The next was the stroboscope, where it uses a potentiometer to change the flickering rate i.e how fast the LED oscillates and upon matching that with a moving or rotating object, we can see the object is still and steady, by calculating the period of oscillation and inversing it, we calculate the frequency of the object. Here my camera's shutter speed is being approximated. Next is the Live wire detector, where it can detect the presence of live wires. Using transistors as Darlington pairs, it was picking up very small signals. Upon bringing that near an AC supply, there's a metallic plate behind the breadboard which acts as a surface capacitor and triggers the Arduino. Finally, the Height measurement tool is made by a laser pointer and the inclination readings. Using basic geometry and the tan(phi)=height/base, we were able to measure the height. The phi is the inclination angle measured by the accelerometer and the base is kept unity. Thus the height equals the tan(phi). These were all controlled by the Arduino, and along with that, an OLED display is used for displaying the menu and a button and potentiometer for selecting.
Step 2: Connections
Modules -------> Arduino
LIS3DHTR SDA SDA
LIS3DHTR SCL SCL
0.96" OLED SDA SDA
0.96" OLED SCL SCL
Laser Module +pin 4
White LED +pin 13
Step 3: Code
#include "LIS3DHTR.h" #include <Arduino.h> #include <U8x8lib.h> #include <Wire.h> LIS3DHTR<TwoWire> LIS; #define WIRE Wire U8X8_SSD1306_128X64_NONAME_HW_I2C u8x8(U8X8_PIN_NONE); int x = 0, y = 0, ch = 0, lch = -1; boolean f = false, goMenu = true; long ps = 0, out; void setup() { pinMode(4, OUTPUT); pinMode(13, OUTPUT); pinMode(A0, INPUT); pinMode(A1, INPUT); Serial.begin(115200); LIS.begin(WIRE, 0x19); delay(100); LIS.setFullScaleRange(LIS3DHTR_RANGE_4G); LIS.setOutputDataRate(LIS3DHTR_DATARATE_50HZ); LIS.setHighSolution(true); u8x8.begin(); u8x8.setPowerSave(0); u8x8.setFont(u8x8_font_px437wyse700b_2x2_r); u8x8.drawString(0, 2, "Welcome"); delay(1000); } int ct = 0; void loop() { while (!goMenu) { if (ch == 0) { digitalWrite(13, 0); u8x8.drawString(0, 2, "Level "); level(); } else if (ch == 1) { u8x8.drawString(0, 2, "Height "); u8x8.drawString(0, 6, "in cm "); theodolite(); } else if (ch == 2) { digitalWrite(13, 0); u8x8.drawString(0, 2, "Freq. "); u8x8.drawString(0, 6, "in Hz "); strob(); } else if (ch == 3) { digitalWrite(13, 0); u8x8.drawString(0, 2, "HVac "); u8x8.drawString(0, 6, " "); liveWire(); } } selectMenu(); } char buf[10]; float xraw = 0, yraw = 0, zraw = 0, an0 = 0.0; float ax = 0.0; void level() { float sum = 0; for (int i = 0; i < 100; i++) { xraw = xraw * 0.98 + 0.02 * LIS.getAccelerationX(); yraw = yraw * 0.98 + 0.02 * LIS.getAccelerationY(); zraw = zraw * 0.98 + 0.02 * LIS.getAccelerationZ(); ax = ax * 0.98 + 0.02 * atan(xraw / sqrt((yraw * yraw) + (zraw * zraw))) ; sum += ax; } ax = sum / 100.0; ax -= 0.069; int incl = ax * 57.2957795; itoa(incl, buf, 10); Serial.println(ax); if (incl < 10 && ax >= 0) { buf[1] = ' '; buf[2] = ' '; buf[3] = ' '; buf[4] = ' '; } u8x8.drawString(0, 5, buf); out = millis(); while (digitalRead(2)) { if (millis() - out >= 3000) { goMenu = true; u8x8.drawString(0, 0, "Going "); u8x8.drawString(0, 2, "back "); u8x8.drawString(0, 4, ".... "); u8x8.drawString(0, 6, " "); delay(2500); lch = -1; return; } } } void theodolite() { digitalWrite(13, HIGH); float sum = 0; for (int i = 0; i < 100; i++) { xraw = xraw * 0.98 + 0.02 * LIS.getAccelerationX(); yraw = yraw * 0.98 + 0.02 * LIS.getAccelerationY(); zraw = zraw * 0.98 + 0.02 * LIS.getAccelerationZ(); ax = ax * 0.98 + 0.02 * (xraw / sqrt((yraw * yraw) + (zraw * zraw))) ; sum += ax; } ax = sum / 100.0; ax -= 0.069; float height = ax * 100; itoa(height, buf, 10); if (height < 10 && ax >= 0) { buf[1] = ' '; buf[2] = ' '; buf[3] = ' '; buf[4] = ' '; } else if (height >= 10 && height <= 99) { buf[2] = ' '; buf[3] = ' '; buf[4] = ' '; } else if (height >= 100 && height <= 999) { buf[3] = ' '; buf[4] = ' '; } u8x8.drawString(0, 4, buf); Serial.println(ax); out = millis(); while (digitalRead(2)) { if (millis() - out >= 3000) { u8x8.drawString(0, 0, "Going "); u8x8.drawString(0, 2, "back "); u8x8.drawString(0, 4, ".... "); u8x8.drawString(0, 6, " "); goMenu = true; delay(2500); lch = -1; return; } } } void strob() { while(1) { digitalWrite(4, HIGH); delay(an0); an0 = analogRead(A0) / 4; digitalWrite(4, LOW); delay(an0); out = millis(); while (digitalRead(2)) { float temp=1/an0*2000; itoa(temp, buf, 10); if (temp < 10 && temp >= 0) { buf[1] = ' '; buf[2] = ' '; buf[3] = ' '; buf[4] = ' '; } else if (temp >= 10 && temp <= 99) { buf[2] = ' '; buf[3] = ' '; buf[4] = ' '; } else if (temp >= 100 && temp <= 999) { buf[3] = ' '; buf[4] = ' '; } u8x8.drawString(0, 4,buf); if (millis() - out >= 3000) { u8x8.drawString(0, 0, "Going "); u8x8.drawString(0, 2, "back "); u8x8.drawString(0, 4, ".... "); u8x8.drawString(0, 6, " "); goMenu = true; delay(2500); lch = -1; return; } } } } void liveWire() { if(analogRead(A1)>2) { u8x8.drawString(0, 4, "Detected"); digitalWrite(4,HIGH); } else { u8x8.drawString(0, 4, "--------"); digitalWrite(4,LOW); } out = millis(); while (digitalRead(2)) { if (millis() - out >= 3000) { u8x8.drawString(0, 0, "Going "); u8x8.drawString(0, 2, "back "); u8x8.drawString(0, 4, ".... "); u8x8.drawString(0, 6, " "); goMenu = true; delay(2500); lch = -1; return; } } } void selectMenu() { ch = map(analogRead(A0), 0, 1020, 0, 3); Serial.println(ch); x = 0; y = 0; if (lch != ch) { if (ch == 0) { u8x8.drawString(0, 0, "Incl. "); u8x8.drawString(0, 2, "Level "); } else if (ch == 1) { u8x8.drawString(0, 0, "Measure "); u8x8.drawString(0, 2, "Height "); } else if (ch == 2) { u8x8.drawString(0, 0, "Strob. "); u8x8.drawString(0, 2, " "); u8x8.drawString(0, 4, " "); u8x8.drawString(0, 6, " "); } else if (ch == 3) { u8x8.drawString(0, 0, "Live "); u8x8.drawString(0, 2, "Wire "); u8x8.drawString(0, 4, " "); u8x8.drawString(0, 6, " "); } lch = ch; } if (digitalRead(2) == 1) { u8x8.drawString(0, 0, " "); u8x8.drawString(0, 2, " "); goMenu = false; delay(100); } out = millis(); }
Step 4: Challenges
Completing the hardware prototype and making the code work for the different parts was the biggest challenge of them all. Mainly getting rid of noises that were coming from the accelerometer data. Which was resulting in very jittery measurements of the angles thus bad readings. The live wire detector was false triggering sometimes. Making the demo video finally was very constrained, as it contains a demo of 4 tools.
Step 5: Accomplishments
Watching the height measurements giving accurate results was pretty amazing, as it was a very crude device. Removing the false triggering of the live wire detector.
Step 6: What's Next for ElecTool
Making a perfect enclosure and a PCB for making a perfect handy tool for Engineers and as well as beginner Electricians.
Step 7: It's Not Only Me
Kudos to Deblina Chattopadhyay for creating this project with me :D
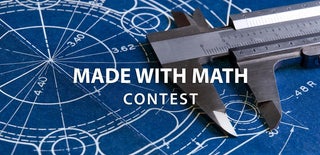
Participated in the
Made with Math Contest