Introduction: Using the Esplora As a Game Controller
In this instructable, I will be teaching you how to use your Arduino Esplora as a joystick/pushbutton controller for all games. I have programmed this to run Kerbal Space Program, but you can make it run whatever you want it to. It is a rather simple project. It uses the onboard accelerometer, the onboard joystick, and the onboard buttons. You can program this to manipulate clicks and mouse control, but that was not needed for the games I programmed it for.
Step 1: The Code
The code is long, but its essential pieces are simple. Here it is:
#include < Esplora.h >
boolean buttonStates[8];
const byte buttons[] = {
JOYSTICK_DOWN,
JOYSTICK_LEFT,
JOYSTICK_UP,
JOYSTICK_RIGHT,
SWITCH_RIGHT,
SWITCH_LEFT,
SWITCH_UP,
SWITCH_DOWN,
};
const char keystrokes[] = {
'S',
'A',
'W',
'D',
'Q ',
'X',
'T',
'Z'
};
//You can change the keys to whatever you want.
void setup() {
Keyboard.begin();
}
void loop() {
for(byte thisButton = 0; thisButton < 8; thisButton++) {
boolean lastState = buttonStates[thisButton];
boolean newState = Esplora.readButton(buttons[thisButton]);
if (lastState != newState) {
if (newState == PRESSED) {
Keyboard.press(keystrokes[thisButton]);
}
else if (newState ==RELEASED) {
Keyboard.release(keystrokes[thisButton]);
}
}
buttonStates[thisButton] = newState;
}
delay(50);
int xAxis = Esplora.readAccelerometer(X_AXIS);
if (xAxis < 0) { Keyboard.press('E'); delay(50);
};
if (xAxis > 50) {
Keyboard.press('Q');
delay(50);
};
delay(50);
}
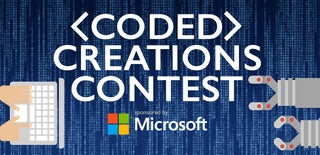
Participated in the
Coded Creations