Introduction: First Step to Your Smarthome With Arduino
The first step in creating a smarthome, is to simply switch on and off lights.
In order to to start this project the following items are needed:
• relay module compatible to Arduino ( means operating at 5V)
• any Arduino, I choose the Mega 2560 because of its many Inputs and Outputs (I/O)
• buttons
• wires to connect the Arduino to the relay module (ribbon cable)
• wires to connect the arduino to the buttons
The wire connecting the buttons to the Arduino can be up to 100m long (tested with Y-(ST)-Y 2x2x0,8mm)
**Falls erwünscht kann ich das instructable um eine deutsche Übersetzung erweitern.**
In order to to start this project the following items are needed:
• relay module compatible to Arduino ( means operating at 5V)
• any Arduino, I choose the Mega 2560 because of its many Inputs and Outputs (I/O)
• buttons
• wires to connect the Arduino to the relay module (ribbon cable)
• wires to connect the arduino to the buttons
The wire connecting the buttons to the Arduino can be up to 100m long (tested with Y-(ST)-Y 2x2x0,8mm)
**Falls erwünscht kann ich das instructable um eine deutsche Übersetzung erweitern.**
Step 1: Make Connections
First we start to connect the arduino to the relay module.
The easiest way is using a ribbon cable.
Just connect the ground of the module to ground of the Arduino and the power input of the relay to the Vin of the Arduino, to make sure that not to much current will pass the arduino.
Next we connect the relay input to any output pin of the arduino (in my example (Pin 22- 29)
Now we connect the buttons to the Arduino.
One pin of the buttons to ground and the other one to a input Pin (in my example to Pin 40 and 41)
The easiest way is using a ribbon cable.
Just connect the ground of the module to ground of the Arduino and the power input of the relay to the Vin of the Arduino, to make sure that not to much current will pass the arduino.
Next we connect the relay input to any output pin of the arduino (in my example (Pin 22- 29)
Now we connect the buttons to the Arduino.
One pin of the buttons to ground and the other one to a input Pin (in my example to Pin 40 and 41)
Step 2: Coding First Try
Hardware is set up, but an Arduino without programming won't be that much fun, so lets start.
First I started with the following code:
int relay = 22;
int button = 40;
int buttonState = 0;
int buttonPushCounter = 0;
int lastButtonState = 0; void setup()
{ pinMode(relay, OUTPUT);
pinMode(button, INPUT_PULLUP);
}
void loop() {
buttonState = digitalRead(button); if (buttonState != lastButtonState) {
if (buttonState == HIGH) {
buttonPushCounter++;
}
delay(200);
}
lastButtonState = buttonState; if (buttonPushCounter % 2 == 0) {
digitalWrite(RELAY1, HIGH);
} else {
digitalWrite(RELAY1, LOW);
}
}
It works well but it is just for one button and the counting and mathematical operation at the end did not really satisfy me.
First I started with the following code:
int relay = 22;
int button = 40;
int buttonState = 0;
int buttonPushCounter = 0;
int lastButtonState = 0; void setup()
{ pinMode(relay, OUTPUT);
pinMode(button, INPUT_PULLUP);
}
void loop() {
buttonState = digitalRead(button); if (buttonState != lastButtonState) {
if (buttonState == HIGH) {
buttonPushCounter++;
}
delay(200);
}
lastButtonState = buttonState; if (buttonPushCounter % 2 == 0) {
digitalWrite(RELAY1, HIGH);
} else {
digitalWrite(RELAY1, LOW);
}
}
It works well but it is just for one button and the counting and mathematical operation at the end did not really satisfy me.
Step 3: Simplify Code
Next step was to eliminate the counting and implement more buttons.
This one is okay for just a few input and outputs. But as more you add the more confusing the code get.
int buttonState1 = 0;
int buttonState2 = 0;
int lastButtonState1 = 0;
int lastButtonState2 = 0;
void setup()
{
// Initialise the Arduino data pins for input
pinMode(22, OUTPUT);
pinMode(23, OUTPUT);
// Initialise the Arduino data pins for input, the pullup command saves real resistors
pinMode(40, INPUT_PULLUP);
pinMode(41, INPUT_PULLUP);
}
void loop() {
// read the pushbutton input pin
buttonState1 = digitalRead(40);
buttonState2 = digitalRead(41);
// compare the buttonState to its previous state and check if button is pressed
if (buttonState1 == LOW && buttonState1 != lastButtonState1) {
//toggle RELAY
digitalWrite(22, !digitalRead(22));
}
if (buttonState2 == LOW && buttonState2 != lastButtonState2) {
//toggle RELAY
digitalWrite(23, !digitalRead(23));
}
lastButtonState1 = buttonState1;
lastButtonState2 = buttonState2;
delay(50);
}
This one is okay for just a few input and outputs. But as more you add the more confusing the code get.
int buttonState1 = 0;
int buttonState2 = 0;
int lastButtonState1 = 0;
int lastButtonState2 = 0;
void setup()
{
// Initialise the Arduino data pins for input
pinMode(22, OUTPUT);
pinMode(23, OUTPUT);
// Initialise the Arduino data pins for input, the pullup command saves real resistors
pinMode(40, INPUT_PULLUP);
pinMode(41, INPUT_PULLUP);
}
void loop() {
// read the pushbutton input pin
buttonState1 = digitalRead(40);
buttonState2 = digitalRead(41);
// compare the buttonState to its previous state and check if button is pressed
if (buttonState1 == LOW && buttonState1 != lastButtonState1) {
//toggle RELAY
digitalWrite(22, !digitalRead(22));
}
if (buttonState2 == LOW && buttonState2 != lastButtonState2) {
//toggle RELAY
digitalWrite(23, !digitalRead(23));
}
lastButtonState1 = buttonState1;
lastButtonState2 = buttonState2;
delay(50);
}
Step 4: Much More Attractive Coding
As seen in the former steps of coding adding additional I/O is not very comfortable and makes the code long and susceptible to errors.
I added 8 in and outputs and recognised that there must be a much more elegant way of writing.
The answer to the question was: arrays
So at the final step we are using arrays to solve the former problems.
int i;
int x = 9; // Number of inputs
int y = 9; // Number of outputs
char buttonState; //actual state of button
char lastButtonState[9]; //last state of button
int pinOUTarray[] = {22, 23, 24, 25, 26, 27, 28, 29, 30}; //PIN OUT Array for pin 22-30
int pinINarray[] = {40, 41, 42, 43, 44, 45, 46, 47, 48, 53}; //PIN IN Array for pin 40-48 and 53
void setup()
{
// Initialise the Arduino data pins for output
for (i=0; i
pinMode(pinOUTarray[i], OUTPUT);
}
// Initialise the Arduino data pins for input with Pullup resistor
for (i=0; i
pinMode(pinINarray[i], INPUT_PULLUP);
}
// Initialise the Arduino data pins for last button state
for (i=0; i
lastButtonState[i] = digitalRead(pinINarray[i]);
}
}
void loop() {
for (i=0; i
//read button state
buttonState = digitalRead(pinINarray[i]);
if (buttonState == LOW && buttonState != lastButtonState[i]) {
//toggle RELAY
digitalWrite(pinOUTarray[i], !digitalRead(pinOUTarray[i]));
}
lastButtonState[i] = buttonState; // save last button state
}
delay(30); //simple debounce switch
}
I added 8 in and outputs and recognised that there must be a much more elegant way of writing.
The answer to the question was: arrays
So at the final step we are using arrays to solve the former problems.
int i;
int x = 9; // Number of inputs
int y = 9; // Number of outputs
char buttonState; //actual state of button
char lastButtonState[9]; //last state of button
int pinOUTarray[] = {22, 23, 24, 25, 26, 27, 28, 29, 30}; //PIN OUT Array for pin 22-30
int pinINarray[] = {40, 41, 42, 43, 44, 45, 46, 47, 48, 53}; //PIN IN Array for pin 40-48 and 53
void setup()
{
// Initialise the Arduino data pins for output
for (i=0; i
pinMode(pinOUTarray[i], OUTPUT);
}
// Initialise the Arduino data pins for input with Pullup resistor
for (i=0; i
pinMode(pinINarray[i], INPUT_PULLUP);
}
// Initialise the Arduino data pins for last button state
for (i=0; i
lastButtonState[i] = digitalRead(pinINarray[i]);
}
}
void loop() {
for (i=0; i
//read button state
buttonState = digitalRead(pinINarray[i]);
if (buttonState == LOW && buttonState != lastButtonState[i]) {
//toggle RELAY
digitalWrite(pinOUTarray[i], !digitalRead(pinOUTarray[i]));
}
lastButtonState[i] = buttonState; // save last button state
}
delay(30); //simple debounce switch
}
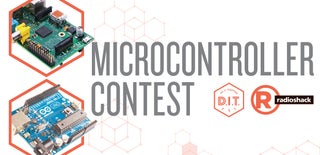
Participated in the
Microcontroller Contest
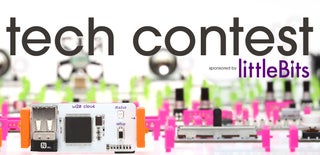
Participated in the
Tech Contest