Introduction: How to Control LEDs Using a Remote Control
In an earlier instructable I demonstrated how to get the HEX codes for the remote control buttons. Here is the link:
https://www.instructables.com/id/How-to-Capture-Rem...
Now that we have the codes, we can use them to control what ever we wish, in this instructable I will show you how to turn on and off LEDs with a remote control using the codes that we captured.
Step 1: Here Is What You'll Need for This Project
For this project you will need the following items:
- An IR receiver, I used the 1838B data sheet included in this step.
- 5 LEDs, one of each color: red, blue, yellow, orange and green
- 5 220 Ohms resistors
- 1 remote control
- Lots of jumper wires
- A solderless bread board
- An Arduino, I used an Arduino Nano
Attachments
Step 2: Connect the Components
Use the schematic diagram above as a guide to connect the components.
First connect the GND from the Arduino to the Blue rail on the Breadboard and the 5V to the red rail on the breadboard.
Second connect the IR receiver:
Connect the signal pin from the IR receiver to PIN 2 on the Arduino.
Connect the Ground pin from the IR receiver to the Ground on the breadboard .
Connect the VCC pin from the IR receiver to the +ve red rail on the breadboard.
Third connect the LEDs:
Start with the red LED, connect the long end of the red LED to PIN 8 on the Arduino and the short end to a resistor and then to the GND on the breadboard.
Then the blue LED, connect the long end of the blue LED to PIN 9 on the Arduino and the short end to a resistor and then to the GND on the breadboard.
Then the orange LED, connect the long end of the orange LED to PIN 10 on the Arduino and the short end to a resistor and then to the GND on the breadboard.
Then the yellow LED, connect the long end of the yellow LED to PIN 11 on the Arduino and the short end to a resistor and then to the GND on the breadboard.
lastly the green LED, connect the long end of the green LED to PIN 12 on the Arduino and the short end to a resistor and then to the GND on the breadboard.
Step 3: The Sketch
This sketch will make good use of the "if" statement. It will use the buttons 0 to 5 on the remote control. When you press any number between 1 and 5, it will verify if the LED is on then it will turn it off or if the LED is off, then it will turn it on. If you press 0, it will turn off all the LEDs.
Number 1 will control the red LED.
Number 2 will control the blue LED.
Number 3 will control the orange LED.
Number 4 will control the yellow LED.
Number 5 will control the green LED.
Number 0 will turn off all the LEDs.
The Sketch:
Start by defining the variables:
for IR receiver
int RECV_PIN = 2;
IRrecv irrecv(RECV_PIN);
decode_results results;
for the LEDs
int RLED = 8;
int BLED = 9;
int OLED = 10;
int YLED = 11;
int GLED = 12;
In the void setup, Start the receiver and set the pin mode for all LEDs to Output.
void setup() {
irrecv.enableIRIn(); //start the reciever
pinMode(RLED, OUTPUT); //set the pin 8 to output
pinMode(BLED, OUTPUT); //set the pin 9 to output
pinMode(OLED, OUTPUT); //set the pin 10 to output
pinMode(YLED, OUTPUT); //set the pin 11 to output
pinMode(GLED, OUTPUT); //set the pin 12 to output }
-----------------------------------------------------------------------------------------------------------
From the earlier instructable, we discovered the codes for 0 to 5 and they are :
1 is FF30CF
2 is FF18E7
3 is FF7A85
4 is FF10EF
5 is FF38C7
and 0 is FF6897
-----------------------------------------------------------------------------------------------------------
In the void loop we start by verifying if there is any signal from the remote.
If the IR receives one of the codes above (I used the 0x because I used the hex code) then it will either turn OFF or ON the LED.
First the code checks to see if the LED is off, if it is then it turns it on, if it is on then it turns it off
Unless the code FF6897 (0) is received, then it turns off all the LEDs
void loop() <br> { if (irrecv.decode(&results)) //this line checks if we received a signal from the IR receiver { if (results.value == 0xFF30CF) //if the result is 1, turn on or off the red LED { if (digitalRead(RLED) == LOW) //if the red LED is off { digitalWrite(RLED, HIGH); //turn it on irrecv.resume(); // wait for another signal from the remote } else {digitalWrite(RLED, LOW);} //if the red LED is on, then turn it off } if (results.value == 0xFF18E7) //if the result is 2, turn onor off the blue LED { if (digitalRead(BLED) == LOW) //if the blue LED is off { digitalWrite(BLED, HIGH); //turn it on irrecv.resume(); } else {digitalWrite(BLED, LOW);} //if the blue LED is on, then turn it off } if (results.value == 0xFF7A85) //if the result is 3, turn on of off the orange LED { if (digitalRead(OLED) == LOW) //if the orange LED is off { digitalWrite(OLED, HIGH); //turn it on irrecv.resume(); } else {digitalWrite(OLED, LOW);} //if the orange LED is on, then turn it off } if (results.value == 0xFF10EF) //if the result is 4, turn on or off the yellow LED { if (digitalRead(YLED) == LOW) //if the yellow LED is off { digitalWrite(YLED, HIGH); //turn it on irrecv.resume(); } else {digitalWrite(YLED, LOW);} //if the yellow LED is on, then turn it off } if (results.value == 0xFF38C7) //if the result is 5, turn on or off the green LED { if (digitalRead(GLED) == LOW) //if the green LED is off { digitalWrite(GLED, HIGH); //turn it on irrecv.resume(); } else {digitalWrite(GLED, LOW);} //if the green LED is on, then turn it off } if (results.value == 0xFF6897) //if the result is 0, turn off all the LEDs { digitalWrite(RLED, LOW); //turn off the red LED digitalWrite(BLED, LOW); //turn off the blue LED digitalWrite(OLED, LOW); //turn off the orange LED digitalWrite(YLED, LOW); //turn off the yellow LED digitalWrite(GLED, LOW); //turn off the green LED } irrecv.resume(); //receive the next value } }
Attachments
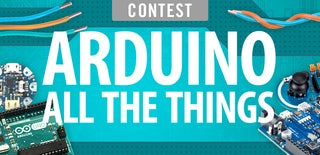
Participated in the
Arduino All The Things! Contest