Introduction: How to Create a Mouse-Driven Menu As EXE Using Windows Batch Code
This tutorial will explain how to create a mouse driven menu to carry out subs in a compiled EXE. (i.e. the user chooses menu options with a left-mouse click.) This tutorial assumes you understand and are familiar with basic windows batch file programing. This tutorial uses a specific compiler available as freeware. .bat files NOT compiled as EXE with this program WILL NOT function properly, because the program includes a set of advanced commands in syntax that is not standard with regular batch files.
Step 1: Download and Install the Advanced BAT to EXE Compiler
The First thing you will need to do is to download and Install a Windows Program called Advanced BAT To EXE Converter. This program compiles Windows Batch (.bat) files into Windows Executable Programs (.exe), that act as stand-alone executables.
The two major differences between the compiled executable and its parent batch file are, First, the executable file cannot be opened with a text editor to view or modify the code, unlike the batch file. Second, we are able to compile advanced commands into the exe that allow for drawing images, etc. on the screen and allow you to provide a mouse driven interface, as well as compile standard batch commands for windows XP and later.
HOWEVER, this program will only work for XP and later, due to the fact that the program relies on features within the command interpreter that were not available within the WIN-98 Platform.
You can get this program for FREE at http://www.battoexeconverter.com/
Step 2: Write the Code for the Graphics (Part 1)
First, A word about the plane notation for the graphics. The compiler's interface, and the commands, use the structure of designating Y, X ( though sometimes they will be ordered X, Y for certain commands.) "X" means the Horizontal axis, from left to right, which value may range from 1 (Far left) to 80 (far right). The "Y" Axis is the vertical axis, and has a value from 1 to 25. (The limits on the values suit the majority of functional purposes, and unless your trying to use special graphics, it is generally not necessary to go beyond these values.)
Therefore the point in the Top-Left corner of the executable's window will be Y=1 and X=1, and the bottom-right will be Y= 25 and X= 80. We generally separate the two numbers with a space, except when declaring the values in blocks, in which the values are separated by comas and the blocks are separated by the space.
Also, in this program, the rem command both functions as the standard remark command (Which shows up GREEN in the compiler) as in regular batch coding, but ALSO functions as the beginning tag of an Advanced command, which turn BLUE when in a proper form, and RED when they have incorrect syntax. Most of the commands we will be using will be advanced commands. Like a regular batch file, each line contains a new command, and the commands process from top to bottom, except where we employ sub-program blocks.
SO NOW We will get to it. We will start with our echo turned off and our screen cleared,. as we would for a regular batch file. W have also declared "load" as a sub-block. The commands are:
@echo off
:load
cls
Step 3: Write the Code for the Graphics (Part 2 )
Now we move on to writing the code to draw a nice box. To draw a box, we use the command PrintBoxAt. The proper syntax of the command is " rem PrintBoxAt (y value) (x value) (y value) (x value) (border style). The first set of y-x values are the coordinates for the top-left corner of the box. The Second set are for the bottom-right. the style number may be 1 or 2, which changes the styling of the box from being single- or double- line. ALL of the values must be separated by spaces. For our example the command is as follows:
rem PrintBoxAt 5 9 18 64 2
next, we insert a wait command, to allow the interpreter to catch up. the syntax for a wait command is rem wait (ms), where a value of 1000 is equal to one whole second. This is similar to pause, but it just waits the predetermined amount of time and then proceeds to process, as opposed to requiring the user to press any key, as with the standard pause command. Remember to insert each command on a new line.
rem wait 200
Now we get to the part of inserting our menu and options text. The command to insert text is rem PrintColorAt ( Your Text String) (Y) (X) (Forground Color) (Background Color) . The coordinates mark the position of the first character of the text string, and the rest of the string proceeds to the right. Each Character will be 1X by 1Y in size, INCLUDING spaces in the string. The Color codes we will be using are: 15= WHITE and 0 = BLACK. We then use the command rem LocateAt to move the cursor to the bottom left of our screen. (syntax is rem LocateAt (Y) (X). Our example proceeds as follows:
rem PrintColorAt MAIN MENU 7 32 15 0
rem PrintColorAt 1) Test Menu 10 32 15 0
rem PrintColorAt 2) Reload Main Menu 14 32 15 0
rem PrintColorAt 3) Exit Program Menu 18 32 15 0
rem LocateAt 25 1
rem wait 200
Step 4: Program the Code for the Mouse-Driven GUI.
Now its time to write the code for the mouse-driven GUI block. It is important to understand in this step, unlike everything else we have done so far, coridinates will be entered X first, then Y, which is backwards from how we've been doing it. We will be starting our clickable zones on the first character of the option, and ending it at X= 53 of the line.
The command we use to do this is rem Mousecmd. The syntax for each block (which represents a single option), is (X,Y) of the beginning of the clickable zone, then (X,Y) of the end of the clickable zone. The four numbers for each block are separated by Commas, and each block is separated by a single space. The interpreter assigns each block a number, where the first block will be 1, the second block will be 2, and so on.
When the program is executed, clicking the mouse on any point within the range of coordinates will store the block number which was clicked as the variable %result%. We then use an 'IF" statement to carry out a sub-program of our choosing. The ending go-to statement Is filled when none of the 'IF" statements are true. Our example proceeds as follows:
rem MouseCmd 32,10,53,10 32,14,53,14 32,18,53,18
if %result%==1 goto test
if %result%==2 goto load
if %result%==3 goto end
goto eror
We then write the code for each of the four subs. We need to use the colon (:) to declare the sub, but NOT to call it within a "go-to" expression. We proceed as follows:
:test
cls
rem PrintBoxAt 5 9 18 64 2
rem wait 200
rem PrintColorAt THE MENU TEST WORKED! 13 27 15 0
rem LocateAt 25 1
rem wait 200
pause
goto load
And we then write the code for the remaing subs in similar fashion as with a regular Batch File, integrating the Advanced Commands as appropriate into the code.
Step 5: Compile the Code to an EXE File.
Now, its time to compile the program as an Executable file, as opposed to the batch file we have been working with so far. We do this with the menu bar of the compiler. File > Build EXE. An options screen will then appear, with several tabs. Most of the options are self-explanatory. You will only need an administrative-manifest if your program requires elevated user privileges. The Password option will keep others from snooping out your code by encrypting the raw code.
After your done, click the "Build EXE" button at the bottom. A "SAVE AS" dialog will open up to save the EXE file. This EXE file is your finished product.
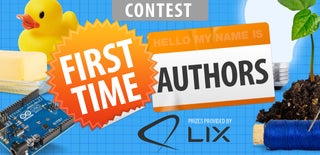
Participated in the
First Time Author Contest 2016