Introduction: How to Measure Temperature Very Accurately With an Arduino and a TSYS01 Temperature Sensor Board.
While studying in university we were challenged as part of a course work into designing a box with very accurate temperature control. If the project were to succeed, multiple boxes were to be built and used in a research project studying the effect of surface material on the perceived temperature of flooring and other building materials.
The project was deemed as very challenging by the university staff because the temperature inside the boxes should stay within ± 0.1°C of the desired value.
Therefore we would require the most accurate temperature sensor we could find. On one hand, the sensor should be factory calibrated to the desired temperature, because calibrating to within 0.1°C of the national temperature standard is next to impossible on a simple setup of boiling and freezing water. On the other hand, an analog temperature sensor with a proclaimed accuracy of ± 0.1°C might still produce inaccurate results after the value is converted to a digital value by an ADC (https://en.wikipedia.org/wiki/Analog-to-digital_converter).
The only solution we could find was to use the TSYS01 digital output single chip temperature sensor by Measurement Specialties and communicate with it using a Arduino microcontroller.
In this instructable I will explain how to use the TSYS01 Temperature Sensor Board with an Arduino microcontroller for very accurate temperature measurements. If you have never used an Arduino microcontroller you can get started by using this instructable: https://www.instructables.com/id/Intro-to-Arduino/ I will not go into detail on how to use the Arduino platform on this instructable, but will only focus on the usage of the TSYS01 temperature sensor board with an Arduino.
Step 1: Introduction of the TSYS01 and the Temperature Sensor Board.
With the correct peripheral circuit TSYS01 can give provide ±0.1°C accuracy between -5°C and +50 °C. It's 24 bit ADC conversion result is factory calibrated separately for each chip with a 4th degree polynomial. It has low self heating and a small time constant for a single chip sensor. The 24 bit ADC can provide enough resolution for any application(more than 0.0001°C), but some noise can be seen in the output if using more than 0.01°C of the resolution. The very low measurement noise of the sensor can for example enable you to use time constant compensation based on the current rate of temperature change without unbearable measurement noise. The sensor output and a value with software compensation of 4 s time constant during a transition from room temperature to finger temperature can be seen in the graph above. The full measurement range of the sensor is from -40°C to 125°C, but the accuracy of the sensor is only ±0.5°C outside the -5°C to +50°C temperature range.
The downside of the TSYS01 is that it is remarkably difficult to use without a nicely designed temperature sensor board. The 4mm by 4mm QFN16 package is a surface mount device, an therefore needs a soldering oven. Additionally the part needs a very stable 3.3V voltage source, a 100 nF capacitor very close to the chip and can only tolerate 3.3V logic level signals. Additionally programming your micro controller to interface with the device using SPI or I2C can be difficult, if you are not very familiar with neither of them(these are the interfaces supported by the sensor).
In the university project we had to design our own temperature sensor board, get the PCBs professionally manufactured, solder the components our selves and implement the SPI communication code, because no temperature sensor board for the sensor was available in the market at that time.
Now it is possible to buy a temperature sensor board that solves these difficulties for hobbyists and use it with an opensource Arduino driver! The board has a 3.3V linear regulator providing low noise power for the sensor, includes the required capacitor, is 2.54mm bread board compatible(!!) requiring no soldering oven, protects the sensor from over voltage, reverse voltage and electrostatic discharge. Therefore, it is now much easier to use for hobbyists, researchers and people doing prototype work for a company.
Step 2: Sourcing the Parts
To follow this instructable you need an Arduino compatible micro controller, the TSYS01 Temperature Sensor Board, a programming cable/device for your micro controller and the jumper wires to connect the temperature sensor board to the microcontroller.
Additionally, if your Arduino is a 5V logic level device, you should consider using a 5V to 3.3V logic level converter. This project will most likely also work without a logic level converter on a 5V Arduino, because the over voltage protection should protect the 3.3V temperature sensor, but using the over voltage protection for level shifting is a bad decision in terms of long term reliability. The safest option is to buy a 3.3V Arduino device. I am using the 3.3V 8MHz Pro Mini. That being said, I have tested the TSYS01 temperature sensor board with a 5V Arduino Nano without any issues. I have also accidentally connected 12V to the logic level pins of the device and it has been fine. My experience is that the over voltage protection relying on resistors and schottky diodes works fine.
I am suggesting to buy most of the stuff you need from the Elecrow webshop (http://www.elecrow.com/), because it is currently the only place to buy the TSYS01 Temperature Sensor Board and you can get most of the stuff you need with only one order and at the same time. If you are using the Arduino Pro Mini, you will also need some tin and access to a soldering iron to solder in the pins.
Bill of Materials for My Setup:
* TSYS01 Temperature Sensor Board, 25$ from http://www.elecrow.com/tsys01-temperature-sensor-b...
* 3.3V 8 MHz Arduino Pro Mini + USB Serial Converter, ~7$: (Search ebay for: Pro Mini atmega328 3.3V 8M Arduino Compatible+CP2102 USB 2.0 to UART TTL module). Make sure the description says that it is the 3.3V version.
* Bread Board, 4.50$: http://www.elecrow.com/16555cm-bread-board-with-sl...
* Male-Female Jumper Wires x 7, 2.80$: http://www.elecrow.com/254mm-femalemale-jumper-wi...
* Female-Female Jumper Wires x 5, 2.80$: http://www.elecrow.com/40-pin-dual-female-splittab...
For 5V Arduino owners:
* You should by logic level converter for high reliability and make your own setup regarding that.
* If you only want to test the TSYS01 Temperature Sensor Board without a logic level converter, it will most likely work, but I won't be responsible if it doesn't.
* You can buy a 3.3V Pro Mini clones really cheap from eBay, why not buy one and be safe? (Search ebay for: "Pro Mini atmega328 3.3V 8M Arduino Compatible+CP2102 USB 2.0 to UART TTL module") Make sure the description says that it is the 3.3V version.
Step 3: Choose Between I2C and SPI
The TSYS01 Temperature Sensor Board supports SPI and I2C communication. You will have to decide for one of them and connect the sensor board for one of them. The connection you are using should similarly reflect the settings you are using in your sketch.
I2C
The I2C connection requires only two data lines to be connected to the temperature sensor board. It also allows multiple devices to share the same two data lines: SDA(data signal goes here) and SCL(clock signal for timing). Selecting the preferred device to communicate with is done by sending a unique I2C address before sending the data packets. At the default Arduino transmission speeds, however I2C is much slower than SPI. Additionally I2C can only pull signal lines down to ground level. The signal level high is acchieved by using two resistors: One between 3.3V and SDA, and the other between 3.3V and SCL. This makes the signal levels to go to 3.3V if the I2C device is not actively pulling the lines down. Atleast most Arduinos should have internal pull-up resistors for the I2C data lines. However, in some cases these internal pull-up resistors may not be enough and external resistors with lower resistance values should be added.
SPI
The SPI connection requires more data lines than I2C, but is also faster, simpler and able to pull signal levels up without additional resistors. The signal lines required are: MOSI(data from master to slave), MISO
(data from slave to master), SCLK(clock signal) and CS(chip select). Because SPI has seperate data lines for different transmission directions, the data can simultaneously be transferred in both direction(not possible on I2C). The MOSI, MISO and SCLK data lines can be shared between multiple SPI devices, but the CS pin has to be used seperately for each device, because it is used to select the preferred device to communicate with. If you have enough available pins on your microcontroller, this is probably the better communication method to choose.
Step 4: Connecting the TSYS01 Temperature Sensor Board to Arduino
You should connect the TSYS01 for the communication method you chose in the previous step. The pin numbers for Arduino Pro mini are given in the brackets. If you are using another arduino, you should check the correct pin numbers yourself.
For I2C:
Board connection:
Arduino <-> TSYS01 Sensor Board
------------------------
3.3V - 12V (VCC) <-> Vin
GND <-> GND
SCL(A5) <-> SCLK/SCL
SDA(A4) <-> MOSI/SDA
Optionally you can also use:
A0 <-> Shutdown (If you want to use the shutdown feature of the board for reduced power consumption)
GND <-> CS/ADDR (If you want to use the alternative I2C address for the board. This is required to get two different sensor working on the same I2C bus.)
For SPI:
Board connection:
Arduino <-> TSYS01 Sensor Board
------------------------
3.3V - 12V(VCC) <-> Vin
GND <-> GND
SCLK(13) <-> SCLK/SCL
MOSI(11) <-> MOSI/SDA
MISO(12) <-> MISO
10 <-> CS/ADDR
GND <-> MODE (To select SPI mode from temperature sensor board)
Optionally you can also use:
A0 <-> Shutdown (If you want to use the shutdown feature of the board for reduced power consumption)
Step 5: Download the Arduino Driver and Create a Sketch
The Arduino driver and some design files for the sensor board can be found from a Github repository: https://github.com/Ell-i/ELL-i-KiCAD-Boards After creating a sketch for you project in the Arduino IDE the easiest way to get the code is to download a zip file from the repository, extract it, navigate to
ELL-i-KiCAD-Boards/TSYS01/Arduino/ and copy the Tsys01.h and Tsys01.cpp files to the directory of your sketch. Now the library should be ready to use.
Next you can test the sensor with the following simple sketch if you chose SPI. If you want to use I2C instead, you only need to comment the SPI sensor object creation line and uncomment the I2C replacement.
Example sketch:
#include "Tsys01.h"
#define slaveSelectPin 10
#define powerPin A0
Tsys01* sensor;
void setup() {
Serial.begin(9600);
//Create SPI sensor object
sensor = new Tsys01(TSYS01_SPI, powerPin,slaveSelectPin);
//Create I2C sensor object
//sensor = new Tsys01(TSYS01_I2C, powerPin);
}
void loop() {
sensor->startAdc();
//Delay to wait ADC conversion to finish.
//9ms should be enough but let's wait 10 ms to be safe.
delay(10);
float temperature = sensor->readTemperature();
Serial.print("The temperature is: ");
Serial.println(temperature,6);
//Delay to read less often
delay(500);
}
Step 6: Use TSYS01 in Your Own Project!
Now you should be ready to use the TSYS01 temperature in your own sensor board. It is also easy to generate high resolution temperature graphs using the sensor. For example the temperature of the exhaust air of my cpu cooler before, during and after a stress test is shown in the picture above. The horisontal axle is seconds and the vertical axle is exhaus air temperature in celsius. Having an arduino attached to the TSYS01 makes for a really accurate DIY temperature probe for use with a computer whenever you may need it! The graph in this instructable were made by printing CSV from the Arduino to the serial port and writing the serial data to file with a Python script. The code for doing this can be found from: https://github.com/Apocalyt/spiTSYS01DataToCSV
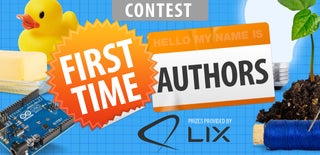
Participated in the
First Time Author Contest 2016
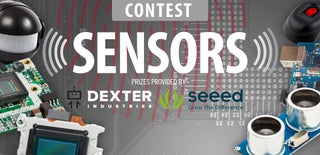
Participated in the
Sensors Contest 2016