Introduction: IR Controlled RGB LED
This will allow full RGB control of an RGB LED (aprox. 1.6 million colours). This is merely a proof of concept; real applications might be to connect and RGB LED srtip for lighting your room or your PC.
Parts list:
- Arduino UNO Rev3
- RGB LED (or RGB LED strip / other RGB display)
- IR remote & receiver
- 3 220R resistors
- Prototyping board (I used a prototyping shield as well)
- 6 jumper cables
Here I use the bottom two rows of digits on my remote (4-9) to control the intensity of each colour.
Step 1: Wiring
You may need to play around with the pins used. I had to change mine until I found some that work. I think there is a bug with the IR library I used; I will need to experiment more with this.
Attachments
Step 2: Code
First, you will need to install the IR library: https://github.com/z3t0/Arduino-IRremote
Find the code here: https://gist.github.com/Marcel-Robitaille/bebb855812428b19bb2c
You may need to change the codes depending on your remote.
#include <./IRremote.h>
int pinR = 9; int pinG = 6; int pinB = 5; int pinSignal = 11;IRrecv irrecv(pinSignal); decode_results results;
long codes[6]= { 0xFD28D7, //4 0xFD18E7, //7 0xFDA857, //5 0xFD9867, //8 0xFD6897, //6 0xFD58A7 //9 }; int valR = 0; int valG = 0; int valB = 0;
void setup(){ Serial.begin(9600); irrecv.enableIRIn();
pinMode(pinR, OUTPUT); pinMode(pinG, OUTPUT); pinMode(pinB, OUTPUT);
}
void loop(){ if(irrecv.decode(&results)){ if(results.value == codes[0] && valR < 255){ valR++; }
else if(results.value == codes[1] && valR > 0){ valR--; }
else if(results.value == codes[2] && valG < 255){ valG++; }
else if(results.value == codes[3] && valG > 0){ valG--; }
else if(results.value == codes[4] && valB < 255){ valB++; }
else if(results.value == codes[5] && valB > 0){ valB--; } display(valR, valG, valB); Serial.print("Red: "); Serial.println(valR); Serial.print("Green: "); Serial.println(valG); Serial.print("Blue: "); Serial.println(valB); Serial.println(""); irrecv.resume(); } }
void display(int red, int green, int blue){ analogWrite(pinR, constrain(red, 0, 255)); analogWrite(pinG, constrain(green, 0, 255)); analogWrite(pinB, constrain(blue, 0, 255)); }
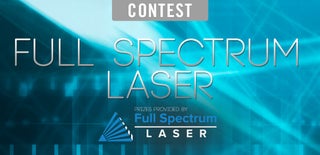
Participated in the
Full Spectrum Laser Contest 2016
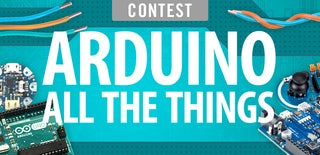
Participated in the
Arduino All The Things! Contest