Introduction: Intro to Neopixels
Hello! Today, I'll show you how to get you neopixels up an running quickly and easily.
Step 1: Parts
For the project, you'll need:
- Neopixels (preferably a ring of them)
- A 1000uf capacitor
- 300 ohm resistor
- 470 ohm resistor
- Jumpers
- Male header pins (4)
- Pushbutton
- Arduino Uno
- USB B cable
- Arduino IDE
- Neopixel Library
- Breadboard
Here are the links to download the software:
Neopixel Library: https://github.com/adafruit/Adafruit_NeoPixel
Arduino IDE: https: www.arduino.cc/en/Main/Software
Step 2: Assemble the Circuit
This circuit is easy to assemble and should take 10 minutes max. Your circuit can look different in the end, so I'm only going to give general guideline to building the circuit.
- Break up the male pin headers in to 4 individual pins. Place them accordingly so that the Neopixel ring will be able to be connected to the breadboard.
- Once you have put in the Neopixel ring, place the 1000f capacitor across its power leads.
- Place your 300 ohm resistor on the breadboard. Connect it to the "input" port of the Neopixel ring through a jumper.
- Place the 470 ohm resistor on your breadboard. Place the push button on one of its leads and connect the other lead of the resistor to the Output pin of the Neopixel Ring.
That's the basic premise of the circuit. It ought to come out looking somewhat similar to the circuit in the above image. Now you need to hook up the Arduino. This part is very straightforward.
- Hook up a jumper to the 5v pin and one to the ground, and hook up the other ends of said jumpers to the power bus of the breadboard.
- Connect a jumper to the non-occupied lead of the push button. Insert the other end of that jumper into digital pin 2.
- Now, put a jumper to digital pin 6 of your Arduino. Connect the other end of that jumper to the open end of the 300 ohm resistor.
Alright! If you had any trouble following those direction, just check out the picture s and you should be able to piece together the circuit.
Step 3: Code
// This is a demonstration on how to use an input device to trigger changes on your neo pixels.
// You should wire a momentary push button to connect from ground to a digital IO pin. When you // press the button it will change to a new pixel animation. Note that you need to press the // button once to start the first animation!
#include
#define BUTTON_PIN 2 // Digital IO pin connected to the button. This will be // driven with a pull-up resistor so the switch should // pull the pin to ground momentarily. On a high -> low // transition the button press logic will execute.
#define PIXEL_PIN 6 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 12
// Parameter 1 = number of pixels in strip, neopixel stick has 8 // Parameter 2 = pin number (most are valid) // Parameter 3 = pixel type flags, add together as needed: // NEO_RGB Pixels are wired for RGB bitstream // NEO_GRB Pixels are wired for GRB bitstream, correct for neopixel stick // NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels) // NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip), correct for neopixel stick Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH; int showType = 0;
void setup() { pinMode(BUTTON_PIN, INPUT_PULLUP); strip.begin(); strip.show(); // Initialize all pixels to 'off' }
void loop() { // Get current button state. bool newState = digitalRead(BUTTON_PIN);
// Check if state changed from high to low (button press). if (newState == LOW && oldState == HIGH) { // Short delay to debounce button. delay(20); // Check if button is still low after debounce. newState = digitalRead(BUTTON_PIN); if (newState == LOW) { showType++; if (showType > 9) showType=0; startShow(showType); } }
// Set the last button state to the old state. oldState = newState; }
void startShow(int i) { switch(i){ case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/off break; case 1: colorWipe(strip.Color(255, 0, 0), 50); // Red break; case 2: colorWipe(strip.Color(0, 255, 0), 50); // Green break; case 3: colorWipe(strip.Color(0, 0, 255), 50); // Blue break; case 4: theaterChase(strip.Color(127, 127, 127), 50); // White break; case 5: theaterChase(strip.Color(127, 0, 0), 50); // Red break; case 6: theaterChase(strip.Color( 0, 0, 127), 50); // Blue break; case 7: rainbow(20); break; case 8: rainbowCycle(20); break; case 9: theaterChaseRainbow(50); break; } }
// Fill the dots one after the other with a color void colorWipe(uint32_t c, uint8_t wait) { for(uint16_t i=0; i
void rainbow(uint8_t wait) { uint16_t i, j;
for(j=0; j<256; j++) { for(i=0; i
// Slightly different, this makes the rainbow equally distributed throughout void rainbowCycle(uint8_t wait) { uint16_t i, j;
for(j=0; j<256*5; j++) { // 5 cycles of all colors on wheel for(i=0; i< strip.numPixels(); i++) { strip.setPixelColor(i, Wheel(((i * 256 / strip.numPixels()) + j) & 255)); } strip.show(); delay(wait); } }
//Theatre-style crawling lights. void theaterChase(uint32_t c, uint8_t wait) { for (int j=0; j<10; j++) { //do 10 cycles of chasing for (int q=0; q < 3; q++) { for (int i=0; i < strip.numPixels(); i=i+3) { strip.setPixelColor(i+q, c); //turn every third pixel on } strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) { strip.setPixelColor(i+q, 0); //turn every third pixel off } } } }
//Theatre-style crawling lights with rainbow effect void theaterChaseRainbow(uint8_t wait) { for (int j=0; j < 256; j++) { // cycle all 256 colors in the wheel for (int q=0; q < 3; q++) { for (int i=0; i < strip.numPixels(); i=i+3) { strip.setPixelColor(i+q, Wheel( (i+j) % 255)); //turn every third pixel on } strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) { strip.setPixelColor(i+q, 0); //turn every third pixel off } } } }
// Input a value 0 to 255 to get a color value. // The colours are a transition r - g - b - back to r. uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if(WheelPos < 85) { return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } if(WheelPos < 170) { WheelPos -= 85; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } WheelPos -= 170; return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); }
Attachments
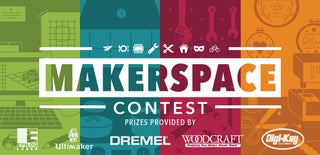
Participated in the
Makerspace Contest 2017