Introduction: Introduction to Neopixels
There are many fun applications of neopixels published on the internet with circuit diagrams and code examples. But there are not many basic coding tutorials.
The purpose of this instructable is to explain the basics of using neopixels for novices, including an introduction to how they are wired and how to program them using the neopixel library.
Step 1: What Is a Neopixel Anyway?
Neopixels are a specific brand of individually addressable RGB LED sold by Adafruit.
Individually addressable means you can program the fourth LED in the strip to do one thing, while the tenth one does something else, and all of the others do a third thing.
RGB means each light is made of a red LED, a green LED, and a blue LED. The color that the LED will appear depends on how brightly each of those three colors is powered. Neopixels can appear to be all the colors of the rainbow and also white.
The neopixels have three connections: a power, a ground, and a digital pin connection. They are based specifically on the WS2812, WS2811, and SK6812 LED drivers.
Step 2: How Do You Connect a Neopixel to Your Processor?
Each neopixel has six points of connection. On one end it has three pins that are for ground, digital pin in, and 5 volt power, on the other end it has three pins that are for ground, digital pin out, and 5 volt power. When you connect your circuit makes sure you connect the pin on your processor to the input pin. The output pin is connected to the next neopixel in the sequence. You cannot light a neopixel without a microprocessor.
Step 3: Which Processor Can I Use With My Neopixels?
In order to light a neopixel you need to connect it to a microprocessor and send instructions from that microprocessor to tell the neopixel when to turn on, and which colors to turn on. There are a huge number of microprocessor choices to operate neopixels, here are just a few choices that will work (and that I happen to have to photograph for this instructable):
- Adafruit trinket
- Adafruit protrinket
- arduino uno
- Raspberry Pi
- Adafruit Flora
- Adafruit Metro
- Circuit Playground (which has built in neopixels)
Step 4: Okay, Its All Connected. How Do I Program My Neopixels?
There are several example programs that come with the neopixel library. The first thing you should do is run one of these examples, like the strandtest example just to make sure that everything is connected correctly. But once you test your circuit, here is a break down of the commands you will need to operate a neopixel. Because the commands don't always agree with the formatting of the instructables editor I have included screen shots of the actual commands for some segments.
The first part of the program, which has to be in the first lines of your code, are the libraries that you will need to include. You need to include the Wire.h and Adafruit_Neopixel.h.
Right after the library statements, you need to add a statement to let the program know that you are using neopixels, how many you are using and what types of neopixels you are using.
Adafruit_NeoPixel strip9 = Adafruit_NeoPixel(1, 9, NEO_RGB + NEO_KHZ400);
In this statement, "strip9" is the name that will be used throughout the program to refer to this strip of neopixels. There are three parameters included in the parentheses.
- The first parameter indicates the number of neopixels included in the strip.
- The second parameter indicates the pin number to which the data pin of the neopixel is connected.
- The third parameter is the type of neopixel, you can add together more than one in this spot of the parentheses.
- NEO_RGB is used for pixels that are wired for red-green-blue.
- NEO_GRB is used for pixels that are wired for green-red-blue.
- NEO_KHZ400 is used for pixels with a 400 kiloHertz bitstream. The Flora pixels are this type.
- NEO_KHZ800 is used for pixels with an 800 kiloHertz bitstream.
In the setup() section of the code, you have to add a statement to start the neopixel strip, the first part of the command is the name you gave your neopixel strip earlier in the program.
strip9.begin();
Also in the setup section of the code, you can set the brightness of the neopixels using this statement.
strip9.setBrightness(255);
The number in the parentheses ranges from 0 - 255 with 0 being not lit and 255 being blindingly bright. Now the startup code is finished, the rest of these commands are for whenever you wish to turn on or change the appearance of your neopixels.
strip9.show(); This command tells the neopixel strip to begin showing the color and brightness you
requested.
strip9.setPixelColor (0, strip9.Color(255, 255, 255)); This command sets the color of one of the pixels.
The first parameter is the position of the neopixel, with 0 being the first one, the three
numbers represent the brightness of each of the three individual colors of light with
0 being not lit and 255 being blindingly bright. Setting all three colors to 255 will
produce blindingly bright white.
For more advanced programming, you can use the colorWipe() function to wipe a color across each of the neopixels in the object. With this function you can control the rate at which colors wipe across the neopixels, and the direction the colors wipe.
Attachments
Step 5: How Do I Power My Neopixels?
According to the Adafruit Learning Center Guide to Neopixels, "The approximate peak power use (all LEDs on at maximum brightness) per meter is:
- 30 LEDs: 9 Watts (about 1.8 Amps at 5 Volts).
- 60 LEDs: 18 Watts (about 3.6 Amps at 5 Volts).
- 144 LEDs : 43 watts (8.6 Amps at 5 Volts).
Mixed colors and lower brightness settings will use proportionally less power."
What does all that mean?
Watts is a unit of measure of electrical power. It means the rate at which energy is delivered to the circuit.
Amps is the unit of measure of electrical current. It means the volume flow rate of charges through the circuit.
Volts is the unit of measure of electric potential difference. It means the difference in electrical potential energy from before a device in a circuit to after a device in the circuit, or said another way, it is the potential for charge to flow through a circuit.
What do you use to deliver that power? You can use an alkaline battery pack, a rechargeable lithium battery, an AC to DC wall adapter, or just power it with a usb cord connected to a computer.
Based on these power requirements, you can decide how you want to power your project. This is the part of the project that can lead to injuries, fires, and other bad things so be cautious when deciding what to use to power your project and how you handle that power source. If your project is a wearable one, make sure that the battery is not touching your skin directly (where it can cause burns), and be very careful when powering projects that you plan to put on either a pet or a child. Too much current can cause the power source to overheat and burn or shock someone. Make sure your lithium polymer batteries are not somewhere where they can be damaged, stabbed, dropped, etc. as this may cause them to explode or overheat.
Step 6: What Projects Can I Make With My Neopixels?
There are limitless possibilities once you start playing with neopixels!
It is best to start with something simple like a small piece of jewelry, such as a lapel pin to practice the programming. After you master the basics, add a sensor and have the neopixels respond to that sensor value, blue when its colder fading to white then red as the area becomes warmer. Once you have an input and an output working together you can add wifi or bluetooth connectivity, or more sensors, or other outputs.
Here are some project ideas: (many of which have already been done by others)
- shoes that light up when you walk, and light up differently depending on how fast you walk
- a bracelet that lights up when your phone receives a text, maybe a different color pattern depending on the author of the text
- a volume of sound indicator for a classroom that goes from green when its quieter up to red when its too loud
- design a clock that uses neopixels to show the hour, minute, and second rather than using a moving hand or make a digital clock that writes the time using color contrast in a square made of many individual neopixels
These are just some thoughts about things you can make. If you visit the Adafruit learning center there are hundreds of projects explained in detaile for you to consider.
Step 7: Where Can I Find Out More Information About Neopixels?
I started my investigations in the Adafruit learning system with their guide on how to use neopixels:
https://learn.adafruit.com/adafruit-neopixel-uberg...
Here is a technical explanation about how to code neopixels without using the neopixel library:
https://wp.josh.com/2014/05/13/ws2812-neopixels-ar...
There are other projects using the neopixels there as well from sneakers to umbrellas to ties. I hope you make some cool and share your project on instructables!
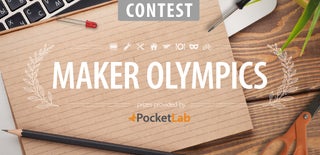
Participated in the
Maker Olympics Contest 2016
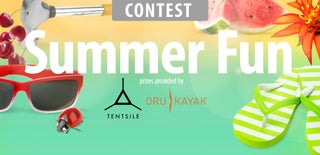
Participated in the
Summer Fun Contest 2016