Introduction: JR6001 MP3 Module Controlled by Arduino
While the JR6001 module was cheap and easy to find, there was very little information on how to actually use this module (often described as a voice playback module). There is a PDF (see attached), but the information is somewhat cryptic. I wanted to use the JR6001 for a project I was commissioned to do, and once I got it working, I thought others might be interested as well.
Attachments
Supplies
Arduino-based microprocessor (I used a MEGA clone)
some buttons or switches (I used a rotary switch)
speaker (I used an 8 Ohm, 3 Watt speaker)
breadboard
jumpers
1K resistor
power supply (I used a power module)
Step 1: Connections
The JR6001 uses 3.6 to 5.2 volts. My MEGA was running at 5V, so I ran both at that voltage. Make sure to have a common GND.
To control the MP3 player, there must be a "Serial" connection. "Serial" is used for programming and serial monitor, so I used "Serial1" instead. The TX1 on the MEGA is pin 18. It connects to the RX of the JR6001. The RX1 on the MEGA is pin 19, and it connects to the TX of the JR6001. While the supply voltage for the JR6001 has a wide range, the logic voltage needs to be 3.3V. The data sheet recommends putting a 1K resistor from the MEGA TX1 to the JR6001 RX to accommodate this difference. If your micro-controller was powered using 3.3V, you could skip the resistor. (While I did not try this, it seems likely that a logic shifter could be used instead of the resistor as well.)
Connect SPK+ and SPK- on the JR6001 to the positive and negative terminals of a speaker.
Note: the JR6001 has a built-in amplifier circuit, but it is not very loud. I may add a second amplifier board to give it a little more volume.
Step 2: Add the MP3s
The PDF recommends renaming your MP3 files 00001.mp3 and 00002.mp3 and 00003.mp3 and so on. I did this, and the A7 command (see below) worked fine.
If you plug in the JR6001 to a computer, it should pop up as a flash drive. Delete the files that came with the module and drag-and-drop copy the files you want to the JR6001 to play.
I kept everything in the main USB flash drive area and did not create any sub-directories. According to the PDF, there can be sub-directories, and there are ways to select them. I did not experiment with any of that.
Step 3: Programming
Here is the set-up code:
// Communication for MP3 Player
Serial1.begin(9600);
// Sets the MP3 player to loop tracks
Serial1.println("B4:01");
// Sets volume to 30
Serial1.println("AF:30");
// Play the default MP3 file
Serial1.println("A7:00001");
The BAUD rate I used was 9600. It worked fine, so I did not experiment with other BAUD rates.
The B4 command sets the playback mode:
- B4:00 - Whole-Circle mode (I have not tried)
- B4:01 - Mono-Loop mode - plays the same track over and over
- B4:02 - Single-Stop mode - plays the track and then stops
- B4:03 - Total-Random mode (I have not tried)
- B4:04 - Catalog-Loop mode (I have not tried)
- B4:05 - Catalog-sequential-playback mode (I have not tried)
- B4:06 - Sequential-Play mode (I have not tried)
Once the B4 command is set, it stays in that mode until a new command is issued. I think the default is 01. See the PDF for more (as I said, somewhat cryptic) information on the play modes.
The AF command changes the volume. There seem to be 31 settings (0 to 30):
- AF:00 - no volume
- AF:10 - volume set to 10
- AF:15 - half volume
- AF:20 - the default volume at start up
- AF:30 - full volume
The A7 command plays a given track:
- A7:00001 - plays the track labelled "00001.mp3" on the JR6001 module
- A7:00005 - plays the track labelled "00005.mp3"
- etc
Other commands I did not try include:
- A2 - replays from the start of the track
- A3 - pauses track
- A4 - stops track
- A5 - plays next track
- A6 - plays previous track
- A8 - sets the directory (but I could not understand the instructions)
- AE - is called "End Play" but the instructions make it sound more like a reset command
- B0 - decreases the volume (I assume B-zero, not B-oh)
- B1 - increases the volume
- B7 - combination play (but the instructions seem to contradict the A7 command above)
- B8 - ends combination play
I was able to get the JR6001 to cycle through my five tracks using the pictured rotary switch and the following code:
void loop() {
// Check switch two
if (!digitalRead(BUTTON_0_PIN)) {
FastLED.delay(DEBOUNCE_TIME);
if (!digitalRead(BUTTON_0_PIN)) switchTwo = 1;
}
if(!digitalRead(BUTTON_1_PIN)) {
FastLED.delay(DEBOUNCE_TIME);
if (!digitalRead(BUTTON_1_PIN)) switchTwo = 2;
}
if(!digitalRead(BUTTON_2_PIN)) {
FastLED.delay(DEBOUNCE_TIME);
if (!digitalRead(BUTTON_2_PIN)) switchTwo = 3;
}
if(!digitalRead(BUTTON_3_PIN)) {
FastLED.delay(DEBOUNCE_TIME);
if (!digitalRead(BUTTON_3_PIN)) switchTwo = 4;
}
if(!digitalRead(BUTTON_4_PIN)) {
FastLED.delay(DEBOUNCE_TIME);
if (!digitalRead(BUTTON_4_PIN)) switchTwo = 5;
}
} // End of loop()
void changeTrackTo() { // Tell MP3 player to change tracks
oldSwitchTwo = switchTwo;
if (switchTwo == 1) Serial1.println("A7:00001");
else if (switchTwo == 2) Serial1.println("A7:00002");
else if (switchTwo == 3) Serial1.println("A7:00003");
else if (switchTwo == 4) Serial1.println("A7:00004");
else if (switchTwo == 5) Serial1.println("A7:00005");
else Serial1.println("A7:00001"); // Default for odd input
Serial.println(); Serial.print("Switch Two Setting = "); Serial.println(switchTwo);
} // End of changeTrackTo()
Here is the code I used to set up the digital input pins:
// Switch 2 is for FX MP3 selection
#define BUTTON_0_PIN 9
#define BUTTON_1_PIN 11
#define BUTTON_2_PIN 13
#define BUTTON_3_PIN 15
#define BUTTON_4_PIN 17
void setup() {
// Set up switch two
pinMode(BUTTON_0_PIN, INPUT_PULLUP);
pinMode(BUTTON_1_PIN, INPUT_PULLUP);
pinMode(BUTTON_2_PIN, INPUT_PULLUP);
pinMode(BUTTON_3_PIN, INPUT_PULLUP);
pinMode(BUTTON_4_PIN, INPUT_PULLUP);
}
Step 4: Conclusion
This is not a complete tutorial on how to code for the JR6001, but this code worked for me, and this is more information than I was able to find anywhere else. And I looked.
If you try out some of the things I did not try (or understand), please post below.
P.S. I had a buddy translate the AD_KEY functions and I included them in the photo. I have used only the 15K PLAY/PAUSE feature, but I have no reason to think the others do not work as well.
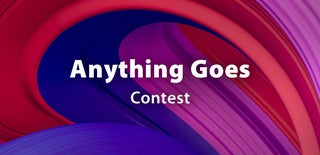
Participated in the
Anything Goes Contest