Introduction: LED-Matrix-Uhr
I've already published a new version.
Ich hsbe bereits eine neue Version hochgeladen.
Die Idee kam mir, als meine alte Uhr, die ich auf mein Schreibtisch deponiert hatte, um immer die Zeit beim Spielen im Blick zu halten, kaputt ging. Da meine Programmierkenntnisse sich in der Zeit um ein Vielfaches gebessert haben und ich mir eine Herausforderung geben wollte, habe ich ein wenig recherchiert, wie viel eine Zahlenanzeige kostet. Zur gleichen Zeit fand ich meine NDS Spiele und wollte doch liebe eine Uhr in einem Retrolook, und meiner Ansicht nach gehören Pixel zur Rertoszene, sodass ich mich dazu entschied, eine LED Matrix dafür zu verwenden.
Ich hsbe bereits eine neue Version hochgeladen.
Die Idee kam mir, als meine alte Uhr, die ich auf mein Schreibtisch deponiert hatte, um immer die Zeit beim Spielen im Blick zu halten, kaputt ging. Da meine Programmierkenntnisse sich in der Zeit um ein Vielfaches gebessert haben und ich mir eine Herausforderung geben wollte, habe ich ein wenig recherchiert, wie viel eine Zahlenanzeige kostet. Zur gleichen Zeit fand ich meine NDS Spiele und wollte doch liebe eine Uhr in einem Retrolook, und meiner Ansicht nach gehören Pixel zur Rertoszene, sodass ich mich dazu entschied, eine LED Matrix dafür zu verwenden.
Step 1: Montage
Ich verwende bei dieser Uhr 140 NeoPixel, einen Arduino Leonardo und eine RealTimeClock. Die Leds habe ich in einer 5x28 Matrix angeordnet und Strom mit 5V und Gnd des Arduinos verbunden und den Datenpin an Pin 6 angeschlossen. Das RTCModul habe ich auch an 5V angeschlossen und mit SCK und SDA des Arduinos verbunden.
Step 2: Programm
Anbei ist der Code für den Arduino, den man eventuell noch modifizieren muss, wenn man die Matrix anders angeschlossen hat:
//Einstellungen
//Aktiviert die Librarys. Nict aendern!
#include
#include
#include
#include
#include
#include
//definiert den Pin, an dem die Matrix angeschlossen ist.
#define PIN 6
//definiert den Anschluss des Minute+ Knopfes
#define button1 2
//definiert den Anschluss des Stunde+ Knopfes
#define button2 4
//definiert die Zeit zwischen Umsprung der Sekundenzahl in Millisekunden dar.
int interval = 1000;
//definiert die Helligkeit. (bei Akku/USB max 70-100)
int brightness = 25;
//stellt die Matrix ein
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(28, 5, PIN,
NEO_MATRIX_TOP + NEO_MATRIX_LEFT +
NEO_MATRIX_COLUMNS + NEO_MATRIX_ZIGZAG,
NEO_GRB + NEO_KHZ800);
//definiert die verschiedenen Farben
uint32_t white = matrix.Color(255, 255, 255);
uint32_t black = matrix.Color(0, 0, 0);
uint32_t blue = matrix.Color(0, 0, 255);
uint32_t brown = matrix.Color(139, 69, 19);
uint32_t grey = matrix.Color(100, 100, 100);
uint32_t green = matrix.Color(0, 255, 0);
uint32_t orange = matrix.Color(255, 135, 0);
uint32_t red = matrix.Color(255, 0, 0);
uint32_t violett = matrix.Color(255, 0, 255);
uint32_t yellow = matrix.Color(255, 255, 0);
//definiert die Hintergrundfarbe und Farbwechsel. (black, white, blue, red, green, yellow, brown, grey, orange, violett)
uint32_t background = black;
//definiert die Farbe der Ziffern und DFarbwechsel. (black, white, blue, red, green, yellow, brown, grey, orange, violett)
uint32_t color = white;
int doublepoint_flash = 1;
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
int hours_e2 = 0;
int hours_z2 = 0;
int minutes_e2 = 0;
int minutes_z2 = 0;
int secs_e2 = 0;
int secs_z2 = 0;
int hours_e = 0;
int hours_z = 0;
int minutes_e = 0;
int minutes_z = 0;
int secs_e = 0;
int secs_z = 0;
unsigned long previoussecond = 0;
int point = 0;
void setup() {
matrix.begin();
matrix.setBrightness(brightness);
matrix.fillScreen(background);
matrix.show();
pinMode(button1, INPUT_PULLUP);
pinMode(button2, INPUT_PULLUP);
pinMode(10, OUTPUT);
digitalWrite(10, HIGH);
}
void hand(uint8_t i, uint8_t x, uint32_t color) {
if(i==0) {
zero(x, color);
}
else if(i==1) {
one(x, color);
}
else if(i==2) {
two(x, color);
}
else if(i==3) {
three(x, color);
}
else if(i==4) {
four(x, color);
}
else if(i==5) {
five(x, color);
}
else if(i==6) {
six(x, color);
}
else if(i==7) {
seven(x, color);
}
else if(i==8) {
eight(x, color);
}
else if(i==9) {
nine(x, color);
}
}
void zero(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 5, color);
}
void one(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+1, 0, 4, color);
matrix.drawFastHLine(x, 4, 3, color);
matrix.drawPixel(x, 1, color);
}
void two(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x, 1, background);
matrix.drawPixel(x+2, 3, background);
}
void three(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 5, color);
matrix.drawFastVLine(x, 1, 3, background);
matrix.drawPixel(x+1, 2, color);
}
void four(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+2, 0, 5, color);
matrix.drawFastVLine(x, 0, 3, color);
matrix.drawPixel(x+1, 2, color);
}
void five(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x+2, 1, background);
matrix.drawPixel(x, 3, background);
}
void six(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x+2, 1, background);
}
void seven(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+2, 0, 5, color);
matrix.drawFastHLine(x, 0, 2, color);
}
void eight(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
}
void nine(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x, 3, background);
}
void loop() {
tmElements_t tm;
if(RTC.read(tm)) {
secs_e2 = tm.Second;
secs_z2 = tm.Second;
minutes_e2 = tm.Minute;
minutes_z2 = tm.Minute;
hours_e2 = tm.Hour;
hours_z2 = tm.Hour;
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
secs_z2 = secs_z2 - secs_e2;
secs_z2 = secs_z2 / 10;
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
minutes_z2 = minutes_z2 - minutes_e2;
minutes_z2 = minutes_z2 / 10;
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
hours_z2 = hours_z2 - hours_e2;
hours_z2 = hours_z2 / 10;
}
hours_e = hours_e2;
hours_z = hours_z2;
minutes_e = minutes_e2;
minutes_z = minutes_z2;
secs_e = secs_e2;
secs_z = secs_z2;
if(secs_e == 0) {
doublepoint_flash = 1;
}
if(secs_e == 2) {
doublepoint_flash = 1;
}
if(secs_e == 4) {
doublepoint_flash = 1;
}
if(secs_e == 6) {
doublepoint_flash = 1;
}
if(secs_e == 8) {
doublepoint_flash = 1;
}
if(secs_e == 1) {
doublepoint_flash = 0;
}
if(secs_e == 3) {
doublepoint_flash = 0;
}
if(secs_e == 5) {
doublepoint_flash = 0;
}
if(secs_e == 7) {
doublepoint_flash = 0;
}
if(secs_e == 9) {
doublepoint_flash = 0;
}
hand(hours_z, 1, color);
hand(hours_e, 5, color);
hand(minutes_z, 11, color);
hand(minutes_e, 15, color);
hand(secs_z, 21, color);
hand(secs_e, 25, color);
if(doublepoint_flash == 1) {
matrix.drawPixel(19, 1, color);
matrix.drawPixel(19, 3, color);
matrix.drawPixel(9, 1, background);
matrix.drawPixel(9, 3, background);
}
else if (doublepoint_flash == 0) {
matrix.drawPixel(19, 1, background);
matrix.drawPixel(19, 3, background);
matrix.drawPixel(9, 1, color);
matrix.drawPixel(9, 3, color);
}
matrix.show();
}
//Einstellungen
//Aktiviert die Librarys. Nict aendern!
#include
#include
#include
#include
#include
#include
//definiert den Pin, an dem die Matrix angeschlossen ist.
#define PIN 6
//definiert den Anschluss des Minute+ Knopfes
#define button1 2
//definiert den Anschluss des Stunde+ Knopfes
#define button2 4
//definiert die Zeit zwischen Umsprung der Sekundenzahl in Millisekunden dar.
int interval = 1000;
//definiert die Helligkeit. (bei Akku/USB max 70-100)
int brightness = 25;
//stellt die Matrix ein
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(28, 5, PIN,
NEO_MATRIX_TOP + NEO_MATRIX_LEFT +
NEO_MATRIX_COLUMNS + NEO_MATRIX_ZIGZAG,
NEO_GRB + NEO_KHZ800);
//definiert die verschiedenen Farben
uint32_t white = matrix.Color(255, 255, 255);
uint32_t black = matrix.Color(0, 0, 0);
uint32_t blue = matrix.Color(0, 0, 255);
uint32_t brown = matrix.Color(139, 69, 19);
uint32_t grey = matrix.Color(100, 100, 100);
uint32_t green = matrix.Color(0, 255, 0);
uint32_t orange = matrix.Color(255, 135, 0);
uint32_t red = matrix.Color(255, 0, 0);
uint32_t violett = matrix.Color(255, 0, 255);
uint32_t yellow = matrix.Color(255, 255, 0);
//definiert die Hintergrundfarbe und Farbwechsel. (black, white, blue, red, green, yellow, brown, grey, orange, violett)
uint32_t background = black;
//definiert die Farbe der Ziffern und DFarbwechsel. (black, white, blue, red, green, yellow, brown, grey, orange, violett)
uint32_t color = white;
int doublepoint_flash = 1;
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
int hours_e2 = 0;
int hours_z2 = 0;
int minutes_e2 = 0;
int minutes_z2 = 0;
int secs_e2 = 0;
int secs_z2 = 0;
int hours_e = 0;
int hours_z = 0;
int minutes_e = 0;
int minutes_z = 0;
int secs_e = 0;
int secs_z = 0;
unsigned long previoussecond = 0;
int point = 0;
void setup() {
matrix.begin();
matrix.setBrightness(brightness);
matrix.fillScreen(background);
matrix.show();
pinMode(button1, INPUT_PULLUP);
pinMode(button2, INPUT_PULLUP);
pinMode(10, OUTPUT);
digitalWrite(10, HIGH);
}
void hand(uint8_t i, uint8_t x, uint32_t color) {
if(i==0) {
zero(x, color);
}
else if(i==1) {
one(x, color);
}
else if(i==2) {
two(x, color);
}
else if(i==3) {
three(x, color);
}
else if(i==4) {
four(x, color);
}
else if(i==5) {
five(x, color);
}
else if(i==6) {
six(x, color);
}
else if(i==7) {
seven(x, color);
}
else if(i==8) {
eight(x, color);
}
else if(i==9) {
nine(x, color);
}
}
void zero(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 5, color);
}
void one(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+1, 0, 4, color);
matrix.drawFastHLine(x, 4, 3, color);
matrix.drawPixel(x, 1, color);
}
void two(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x, 1, background);
matrix.drawPixel(x+2, 3, background);
}
void three(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 5, color);
matrix.drawFastVLine(x, 1, 3, background);
matrix.drawPixel(x+1, 2, color);
}
void four(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+2, 0, 5, color);
matrix.drawFastVLine(x, 0, 3, color);
matrix.drawPixel(x+1, 2, color);
}
void five(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x+2, 1, background);
matrix.drawPixel(x, 3, background);
}
void six(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x+2, 1, background);
}
void seven(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawFastVLine(x+2, 0, 5, color);
matrix.drawFastHLine(x, 0, 2, color);
}
void eight(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
}
void nine(uint8_t x, uint32_t color) {
matrix.fillRect(x, 0, 3, 5, background);
matrix.drawRect(x, 0, 3, 3, color);
matrix.drawRect(x, 2, 3, 3, color);
matrix.drawPixel(x, 3, background);
}
void loop() {
tmElements_t tm;
if(RTC.read(tm)) {
secs_e2 = tm.Second;
secs_z2 = tm.Second;
minutes_e2 = tm.Minute;
minutes_z2 = tm.Minute;
hours_e2 = tm.Hour;
hours_z2 = tm.Hour;
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
if (secs_e2 > 9) {
secs_e2 = secs_e2 - 10;
}
secs_z2 = secs_z2 - secs_e2;
secs_z2 = secs_z2 / 10;
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
if (minutes_e2 > 9) {
minutes_e2 = minutes_e2 - 10;
}
minutes_z2 = minutes_z2 - minutes_e2;
minutes_z2 = minutes_z2 / 10;
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
if (hours_e2 > 9) {
hours_e2 = hours_e2 - 10;
}
hours_z2 = hours_z2 - hours_e2;
hours_z2 = hours_z2 / 10;
}
hours_e = hours_e2;
hours_z = hours_z2;
minutes_e = minutes_e2;
minutes_z = minutes_z2;
secs_e = secs_e2;
secs_z = secs_z2;
if(secs_e == 0) {
doublepoint_flash = 1;
}
if(secs_e == 2) {
doublepoint_flash = 1;
}
if(secs_e == 4) {
doublepoint_flash = 1;
}
if(secs_e == 6) {
doublepoint_flash = 1;
}
if(secs_e == 8) {
doublepoint_flash = 1;
}
if(secs_e == 1) {
doublepoint_flash = 0;
}
if(secs_e == 3) {
doublepoint_flash = 0;
}
if(secs_e == 5) {
doublepoint_flash = 0;
}
if(secs_e == 7) {
doublepoint_flash = 0;
}
if(secs_e == 9) {
doublepoint_flash = 0;
}
hand(hours_z, 1, color);
hand(hours_e, 5, color);
hand(minutes_z, 11, color);
hand(minutes_e, 15, color);
hand(secs_z, 21, color);
hand(secs_e, 25, color);
if(doublepoint_flash == 1) {
matrix.drawPixel(19, 1, color);
matrix.drawPixel(19, 3, color);
matrix.drawPixel(9, 1, background);
matrix.drawPixel(9, 3, background);
}
else if (doublepoint_flash == 0) {
matrix.drawPixel(19, 1, background);
matrix.drawPixel(19, 3, background);
matrix.drawPixel(9, 1, color);
matrix.drawPixel(9, 3, color);
}
matrix.show();
}
Step 3: Fertig
Ich habe bereits an einer weiteren besseren Version der Uhr gearbeitet, die auch wie die LaMetric Internet hatte, allerdings habe ich die Matrix zerstört, ohne Bilder zu haben. Ich werde versuchen, die Matrix zu reparieren und dann dieses Instructables zu ergänzen, was allerdings noch ein paar Wochen dauern könnte. Falls ihr mich unterstützen wollt, würde ich mich über ein Vote in der Kategorie Microcontroller freuen.
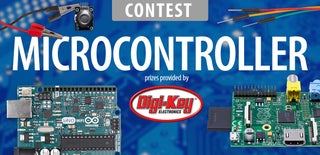
Participated in the
Microcontroller Contest 2017