Introduction: LED + Temperature Monitoring
This project comes with GUI is Microsoft C# and sketch code for Arduino.
Basically, it has a GUI to turn ON and OFF the LED and a temperature button to check the surrounding temperature.
Step 1: Parts
1 unit of Arduino UNO
1 unit of LED (Digital pin 13)
1 unit of LM35 (Analog A0 pin)
1 unit of resistor 47k Ohm
Jumpers
Step 2: How It Works: Arduino
Write the Arduino code based on Serial Communication.
Simple reading and select instruction.
Sample part of Arduino Code:
void loop(){
reading = analogRead(tempPin);
tempC = reading/9.31;
}
** This part simply means the temperature reading will keep on updating/ looping
void ParseCommand(String command){
if(command == commandToggle){
if(ToggleLED()){
Serial.println("Toggled LED");
}
else
{
Serial.println("Error Toggling LED");
}
}
else if (command == commandStatus)
{
GetLEDStatus();
Serial.println(LEDStatus);
}
else if
(command == commandTemp){
Serial.println(tempC);
}
else
{
Serial.println("Unrecognized Command");
}
}
**This part means that the command selected from the GUI if it matches the above, it will triggered.
Step 3: How It Works: Visual C#
Visual C# is used to produce User interface for this project.
public bool toggleLED()
{
try
{
serialPort1.Open();
serialPort1.Write("toggle" + "\n");
String status = serialPort1.ReadLine();
serialPort1.Close();
return true;
}
catch (System.Exception ex)
{
System.Console.WriteLine("Error toggling LED " + ex);
}
return false;
}
**This part of source code is used to read LED status (ON or OFF or Error)
public string getTemp()
{ // get the LED status from the arduino
try
{
serialPort1.Open();
serialPort1.Write("temp" + "\n");
String temp = serialPort1.ReadLine();
serialPort1.Close();
return temp;
}
catch (System.Exception ex)
{
System.Console.WriteLine("Error getting status " + ex);
}
return "ERROR";
}
**This part of source code is used to read temperature from LM35 (Readings in Celcius or Error)
Step 4: Final
This is simple project. Just a simple modification it can leads to bigger things or gadget.
Try and enjoy it, modify it to become better end product.
The Visual C# & Arduino source code is available at the attachment
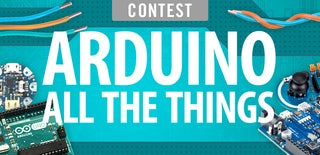
Participated in the
Arduino All The Things! Contest