Introduction: LEDura - Analog LED Clock
After a long time of just making various project I decided to make an instructable myself. For the first one, I will guide you through process of making your own analog clock made with awesome addressable LED ring. The inner ring shows the hours, outer ring shows minutes and seconds.
Beside showing the time, clock can also display the room temperature and it can be a very nice decoration in the room. Every 15 minutes, clock also makes some special effects – video shows them all, make sure to check it out. With the help of 2 buttons and potentiometer, user can chose between different mode and modifie colors by his own desire. I also upgraded it to automatically dim the LEDs if the room goes dark, so the user won’t be disturbed during night.
The clock can be placed on the desk, bed table or hanged from the wall.
Note: Pictures are not as good as the view in the reality because of high brightness.
Step 1: How to Read It?
Clock has 2 rings - smaller one for displaying hours and bigger one for displaying minutes and seconds. Some LEDs glow all the time - a so called compass that indicates main clock positions. On hour ring it represents 3, 6, 9 and 12'o clock, on minute ring it represents 15, 30, 45 and 0 minutes.
Step 2: What Will You Need
Materials:
- 1x Arduino Nano (you can use any other Arduino as well)
- 1x DS3231 RealTimeClock module
- 1x Addressable led ring - 60 LEDs
- 1x Addressable led ring - 24 LEDs
- 2x Buttons (NO - normaly open)
- 1x 100kOhm potentiometter
- 1x 5V power supply (capable of delivering 1 Amp)
- 1x Supply connector
- Some wires
- 1x 10kOhm resistor
1x Photoresistor
- Prefboard(optional)
- Terminal block wire connectors (optional)
- 25mm thick wood, size at least of 22cmx22cm
- 1mm thin mat PVC plastic size 20cmx20xm
Tools:
- Basic tools for building electronics (soldering iron, pliers, screwdriver, ...)
- Drill machine
- Hot glue gun
- Sand paper and some wood varnish
- CNC machine (maybe some friend has it)
Step 3: Electronics Components - Background
DS3231
We could determine the time using Arduinos build in oscillator and a timer, but I decided to use dedicated Real Time Clock (RTC) module, which can keep track of time even if we disconnect the clock from its power source. DS3231 board has a battery, which provides power when module is not connected to power supply. It is also more precise on longer periods of time than Arduinos clock source.
DS3231 RTC uses I2C interface to communicate with micro-controller – very simple to use and we need only 2 wires to communicate with it. Module also provides temperature sensor, which will be used in this project.
Important: If you are planing to use non-rechargeable battery for the RTC module, you should de-solder the 200 ohm resistor or 1N4148 diode. Otherwise your battery might blow up. More information can be found on this link.
WS2812 LED ring
I decided to use 60 LED ring to keep track of minutes and 24 LED ring for hours. You can find them on Adafruit (neoPixel ring) or some cheap versions on eBay, Aliexpress or other web shops. There is a big diversity among the addressable led strips and if it is your first time to play around with them, I recommend you to read through some usage descriptions – here are some useful links:
Addressable LED strip has 3 connectors: 5V, GND and DI/DO. First two are for powering the LEDs, last one is for the data. Be careful while connecting ring to Arduino – your data line must be connected to DI (data IN) pin.
Arduino
I am using Arduino Nano because it is small and sufficient enough for this project. You can use nearly any other Arduino, but then you must be careful while connecting everything to it. The buttons and LED rings can be on the same pins, but I2C connectors (for RTC module) may differ from platform to platform – check out their datasheet.
Step 4: Electronics - Power Supply
Arduino and LED strip must both be supplied with 5V power source so we know which voltage is needed. Since the LED rings it draws quite a lot of amps we can’t power it directly with Arduino, which can withstand max 20mA on its digital output. By my measurements, LED rings can together draw up to 500 mA. That’s why I bought an adapter which is capable of supplying up to 1A.
With the same power supply we want to power Arduino and LEDs - here you have to be careful.
Warning! Be extra careful when you are testing the LED strip – power adapter must NOT be connected to Arduino, when Arduino is also connected to PC with USB connector (you can damage your computer USB port).
Note: In the schematics below I used normal switch to select if Arduino is powered via power supply or via USB connector. But on the perfboard you can see that I added a pin header to select from which power source Arduino is powered.
Step 5: Electronics - Soldering
When you gather all the parts it is time to solder them together.
Because I wanted to make the wiring neat, I used perfboard and some terminal block connector for wires, so I can unplug them in case of modifications. This is optional - you can also solder the wires directly to the Arduino.
A tip: it is easier if you print the schematics so you have it in-front of you while soldering. And double check everything before connecting to power supply.
Step 6: Software - Background
Arduino IDE
We are going to program Arduino with its dedicated software: Arduino IDE. If you are playing with Arduino for the first time, I recommend you to check some instructables on how to do it. There is already plenty tutorials in the web, so I won’t go to details.
Library
I decided to use FastLED library instead of popular Adafruit. It has some neat math functions with which you can do great effects (Thumbs up to the developers!). You can find the library on their GitHub repository, but I added the .zip file of version that I am using in my code.
If you are wondering, how to add external library to Arduino IDE you can check some already made instructions
For the clock module I used Arduino library for the DS3231 real-time clock (RTC) (link), which you can easily install in Arduino IDE. When you are in IDE, click to Sketch → Include library → Manage libraries… and then filter your search with the name above.
Note: For some reason I currently can't add .zip files. You can find the library on my GitHub repository.
Step 7: Software - Code
Structure
The application is constructed with 4 files:
- LEDclokc.ino --> This is main Arduino application, where you can find functions for controlling the whole clock – they start with prefix CLOCK_.
- LEDclokc.h --> here are pin connection defines and some clock configurations.
- ring.cpp and ring.h --> here is my code for controlling the LED rings.
LEDclock.h
Here you will find all the clock definitions. In the beginning, there are definitions for wiring. Make sure they are the same as your connections. Then there are clock configurations – here you can find the macro for number of modes that the clock has.
LEDclock.ino
On the diagram, main loop is represented. Code first checks if any button is pressed. Because of the nature of switches, we must use debbouncing method to read their values (you can read more about this on the link).
When button 1 is pressed, variable mode is raised by 1, if button 2 is pressed, variable type is raised. We use these variables to determine, which clock mode we want to see. If both buttons are pressed at the same time, function CLOCK_setTime() is called so you can change the time of the clock.
Later code reads the value of potentiometer and stores it into variable – whit this variable user can change the colors of the clock, brightness etc.
Then there is a switch-case statement. Here we determine in which mode clock is currently in, and by that mode, corresponding function is called, which sets up the LEDs colors. You can add your own clock modes and re-write or modify the functions.
As described in FastLED library, you have to call function FastLED.show() on the end, which turns the LEDs to the color we previously set them up to.
You can find much more detailed descriptions between the code lines.
Whole code is attached below in the files below.
TIP: you can find whole project on my GitHub repository. Here the code will also be updated if I will add any changes to it.
Step 8: Make the Clock
Clock frame
I built the clock frame using CNC machine and 25mm thick wood. You can find the sketch drawn in ProgeCAD attached bellow. The slots for LED ring are a bit larger, because manufactures only provide the measurements of outer diameter – inner may vary quite a lot… In the back of the clock, there is a lot of space for electronics and wires.
PVC rings
Because LEDs are quite bright it is good to diffuse them somehow. First I tried with transparent silicone, which does the job of diffusing, but it is quite messy and it is hard to get it smooth on top. That is why I ordered a 20x20 cm piece of “milk” PVC plastics and cut two rings in it with CNC machine. You can use sandpaper to soften the edges so the rings slip in the slots.
Side holes
Then it is time to drill the holes for buttons, potentiometer and power supply connector. Firstly, draw every position with a pencil, then drill in the hole. Here it depends what type of buttons you have – I went with push buttons with slightly curved head. They have 16mm of diameter so I used wood drill of that size. Same goes for potentiometer and power connector. Be sure to erase all the pencil drawings afterwards.
Attachments
Step 9: Draw in the Wood
I decided to draw some clock indicators in the wood – here you can use your imagination and design your own. I burned the wood using soldering iron, heated to max temperature.
For the circles to be nicely round, I used a piece of aluminum, drilled a hole into it and followed the edges of the hole with soldering iron (look at the picture). Make sure that you are holding aluminum firmly, so it doesn’t slip while drawing. And be cautious while doing it to prevent injuries.
If you are making drawings and want them to be nicely aligned to the clock pixels, you can use “Maintenance mode” which will show you where the pixels are going to be located (go to chapter Assemble).
Protect the wood
When you are satisfied with the clock it is time to sand it and protect it with wood varnish. I used very soft sandpaper (value of 500) to soften the edges. I recommend you to use transparent wood varnish, so the color of the wood doesn’t change. Put a small amount of varnish on the brush and pull it in the direction of the annuals in the wood. Repeat it at least 2 times.
Step 10: Assamble
Firs put the buttons and potentiometer on their positions – if your holes are too big, you can use some hot glue to fix them in place. Then put the ring strip in its slots and connect its wires to the Arduino. Before you glue the LED ring into its place it is good to be sure, that LED pixels are in right place – centered and aligned with the drawing. For that purpose I added so called Maintenance mode which will display all the important pixels (0, 5, 10, 15, … on minute ring and 3, 6, 9 and 12 on hour ring). You can enter this mode by pressing and holding both buttons, before plugging the power supply to the connector. You can exit this mode by pressing any button.
When you have your LED rings aligned, apply some hot glue and hold them while glue gets firm. Then take your PVC rings and again: apply some hot glue to the LEDs, quickly position them and hold them for a couple of seconds. In the end, when you are sure that everything works you can hot glue the per board (or Arduino) to the wood. Tip: do not apply to much glue. Just a small amount so it holds in one place but you can easily remove it if you would like to change something later.
On the very end, insert coin cell battery to its holder.
Step 11: Upgrade - Photoresistor
The clock effects are especially nice in the dark. But this can disturb its user during the night, while he or she sleeps. That is why I decided to upgrade the clock with the feature of automatic brightness correction – when the room goes dark; the clock turns off its LEDs.
For that purpose, I used the light sensor - photo resistor. Its resistance will significantly rise; up to few mega ohms when it is dark and it will have only few hundred ohms when there is light shining on it. Together with a normal resistor they form the voltage divider. So when the resistance of the light sensor changes, so does the voltage on Arduino analogue pin (which we can measure).
Before soldering and assembling any circuit, it is wise to simulate it first, so you can see the behavior and make corrections. Whit the help of Autocad Tinkercad you can do exactly that! With just a few clicks I added the components, connected them and wrote the code. In the simulation you can see how the LEDs brightness is changed according to the value of photo resistor. It is very simple and straightforward - you are welcome to play with the circuit.
After simulation it was time to add the feature to the clock. I drilled a hole in the centre of the clock, glued the photo resistor, connected it like it can be seen on the circuit and added few lines of code. In the file LEDclock.h you have to enable this feature by declaring USE_PHOTO_RESISTOR as 1. You can also change at which room brightness the clock will dim the LEDs by changing the CLOCK_PHOTO_TRESHOLD value.
Step 12: Enjoy!
When you will power it for the first time, the clock will show some random time. You can set it up by pressing both buttons at the same time. Turn the knob to select the right time and confirm it with the press of any button.
I found inspiration in some very neat project on the internet. If you decide to build the clock on your own, check them out as well! (NeoClock, Wol Clock, Arduino Colorful Clock) If you ever decide to try to follow instructables, I hope you find making it as enjoyable as I did.
If you stumble across any trouble along the process of making it, feel free to ask me any question in the comments – I will gladly try to answer it!
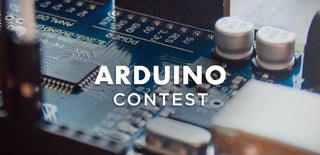
Participated in the
Arduino Contest 2020