Introduction: Make Alexa Skills With Cloud9 - No Credit Card or Hardware Required
[EDIT: Following the takeover of Cloud9 by Amazon, and its integration into AWS, this trick no longer works. Besides, Python 2 is now deprecated. Therefore, this tutorial is archived. Thanks to those of you who tried it!]
Welcome!
In this tutorial you will learn how to create your own Amazon Alexa skill using Cloud9. In case you didn't already know, Cloud9 is an online IDE supporting numerous programming languages. It's completely free, with no credit card required, at least for small projects like this one. An Alexa skill is an app for Alexa devices.
About Me
I have always loved programming and voice assistants but only recently took up programming the Amazon Echo. I faced two key problems with this:
- I don't know a lot of node.js. Therefore, I wanted to create Alexa Skills in Python
- As a Secondary School student I don't have a credit card, so I'm unable to use AWS lambda. The way I solved this problem was to use Cloud9.
I hope you enjoy this tutorial. If you get stuck anywhere check out the images as I have tried to add hints in the correct places. If you are still stuck please feel free to add a question or comment.
(Thanks to HeikoAL on Pixabay for the cover image)
Step 1: Sign Into Cloud9 and Amazon Developer Console.
To make our skill, we will need to create the main logic for the skill in Python on Cloud9. The integration with Alexa will take place on the Amazon Developer Console.
1. Cloud9
You will need a Cloud9 account already for this to work. Unfortunately, since Amazon recently took over Cloud9, and began the process of integrating it into AWS, creating new Cloud9 accounts on the legacy platform is no longer possible, but if you already have an account, you can still follow this tutorial.
Visit https://c9.io/login and login.
2. Amazon Developer Console
Now access https://developer.amazon.com and sign in at the top right corner. Click on 'Alexa', and then 'Your Alexa Dashboards' in the top right. You should see an older looking screen like the one above-right. Next, click on Get Started in the Alexa Skills Kit box.
Step 2: Set Up the Alexa Skill in the Amazon Dev Console
The next step will be to set up the skill on Amazon's side. If you already know how to do this, you can skip onto the following part.
- Click on Add a New Skill and you should be presented with a screen like the one above.
- In the radio buttons at the top, we should choose Custom Interaction Model
- Now choose the language of your skill.
- Important: You must choose English UK if your amazon account has a UK address, as testing will not work on a real life echo device if you choose US. It may still work on Echoism though.
- Now you should enter the name and invocation name of your skill. I would recommend making these the same for convenience. The name is what a user will see in the Alexa app, while the invocation name is what a user would say to trigger the skill.
To understand invocation name, consider this example phrase a user might say:
Alexa, ask Test about the weather.
This expression consists of two parts:
- Test - the invocation name of the skill
- about the weather - an utterance; we will learn about these in the next section.
Our skill will not be using the final three options in the console, so they can be left alone.
Now, click Save, and Next. The developer console may reformat slightly at this point, but it won't lose any data.
Step 3: Interaction Model
For the next step, you should now be on the interaction model tab of the console. This is where we tell Alexa how we would like it to interpret our commands. Choose the Launch Skill Builder Beta option.
We need to set up an intent for our skill. This is a function that it performs. Click the "Add an Intent" button and enter a suitable name. This can be anything, and does not need to be known by the user, however we will need it for the programming later on. I have named my intent `HelloWorld`.
In order to activate our intent, a user will say "Alexa, ask test <utterance>" where utterance is a phrase associated with this intent. To make this work, we should add some utterances. I have typed "for a greeting" and "for a hello" into this box, so that the following will trigger the intent:
- "Alexa, ask test for a greeting"
- "Alexa, ask test for a hello"
Once this has been completed, we need to press "save model" and "build model" at the top.
Important: Make sure to save first, and then build. The building may take a few minutes.
Finally, click the Configuration button at the top left.
Step 4: Over to Cloud9
Now that the skill has been registered with Amazon, we must return to Cloud9 and build the back end to our skill.
In your dashboard, create a new workspace and enter a memorable name. You can add a description if you wish but it isn't necessary. You should choose Python as the language. We will now press "create workspace". It will take some time to load but eventually you should be presented with a screen like the above. This is your workspace.
Cloud9 workspaces are Ubuntu Linux machines (similar to the Raspberry Pi, which is my own prior experience of Linux). They have a key advantage which is that they are "hosted", so we don't have to run the code on our local machine. Before we can write our code into the environment, we will prepare the environment by executing a few commands.
1. Upgrade pip
sudo -H pip2 install --upgrade pip
2. Install Flask:
sudo pip install flask
3. Install Flask-Ask, the library which interfaces with Alexa:
sudo pip install flask-ask
Now we can write our program. Click the green plus at the top and choose New File. Save the file as, e.g., HelloAlexa.py. In the next step we will enter the code to power the skill.
Step 5: The Code!
The following code will be used for the skill.
Note: instead of HelloWorld, use your intent name that you created earlier.
from flask import Flask, render_template
from flask_ask import Ask, statement
import os
app = Flask(__name__)
ask = Ask(app, '/')
@ask.intent('HelloWorld')
def hello():
return statement("Hello, world.")
if __name__ == "__main__":
host = os.getenv('IP', '0.0.0.0')
port = int(os.getenv('PORT', 8080))
app.debug = True
app.run(host=host, port=port)
Let's run through the code:
- The first three lines import our necessary modules: Flask-Ask, for Amazon Alexa, and Flask, a web library which Flask-Ask is based on.
- The next two lines create app and ask variables, which store the contextual data surrounding web requests made to the skill by Amazon's servers (app), and the data about our intents (ask).
- Line 6 is a decorator, which tells flask ask to trigger the function whenever a web request is received with a URL containing "HelloWorld".
- The function below the decorator doesn't run any code; it just returns the value "Hello, world". The statement() command is needed to convert the string into an Alexa statement (something Alexa will say). The flask-ask documentation gives some other options, such as question.
- The rest of the code starts the skill server when the program is executed on Cloud9. We can translate it as:
- if we run this code directly (as opposed to importing it)
- the code will listen on port 8080
- keep running the app and give debug output (useful for testing)
- run the skill according to the parameters we have specified.
Pitfall: If you forget the last 5 lines you will get a "No application seems to be running here" error.
The ports Cloud9 uses are 8080, 8081 and 8082 so any of these would have been fine in place of 8080.
Make sure to save your code before continuing to the next step.
Step 6: Link Cloud9 to Alexa
Now we need to link our Cloud9 code to our Alexa app in the developer portal.
Press the green run button in the corner of the C9 window, and copy and paste the link at the top of your terminal into your web browser (see the image for details). If you see an orange screen with an "Open The Application" button, you should click it. You should see a white screen which says Method Not Allowed on it. This may sound like you have made a mistake, however it simply means that Flask-Ask has been configured properly so that only the Alexa service may visit the page we tried to access.
Provided this has worked correctly, return to the Dev Console in Amazon and make sure you are in the "configuration" tab. Press HTTPS and paste the URL you just had into the text box that pops up. You can ignore the rest of the settings, just press Save and Next. You should now see a small box asking you about Certificates.
Choose the second option, namely "My development endpoint is a sub-domain of a domain that has a wildcard certificate from a certificate authority" and now press Save and then Next.
You should be onto the Test stage now. If you are, great! Move on to the next step. If not, check you have followed the instructions correctly!
Step 7: Testing!
This is the final step! Well done on getting this far. :)
You should see a page like the one above. If the (i) box where it says "Please Complete the Interaction Model" is set to disabled, enable it. We can start by testing the skill on here and then we can try with a real echo device.
Go down to the service simulator section and enter one of the utterances you created earlier. Now press Ask Test. You should see some blue text on both sides. The right hand side should contain the words "Hello, World" or some other text that you set your skill to respond with. If your right-hand text contains an error, check that your code is still running in C9 and that you typed the utterance in correctly.
Once your skill works in the Amazon Developer test section, you can move on to testing it with a real echo device. Make sure the echo device is signed in with the same account you used to make the skill.
Remember: To trigger the skill we need to start with "Alexa, ask Test" and then your utterance. This tells Alexa which app you want to talk to.
If you don't have a physical echo device then you can use the echo simulator https://echosim.io/welcome. You will need to sign in with your Amazon Developer account.
Thank you for reading my Instructable. I hope it worked for you. If you have any questions, make sure to post a comment, and if you liked it be sure to vote for it in the Voice Activated contest and the First Time Author contest.
Thanks again and good luck!
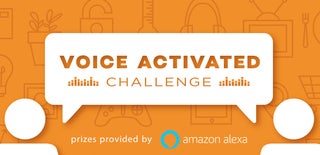
Participated in the
Voice Activated Challenge
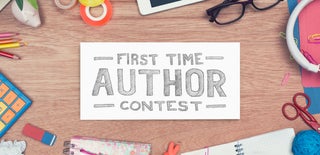
Participated in the
First Time Author Contest 2018