Introduction: Math Calculations to Learn Arduino Programming Basics in Tinkercad
When I got started with Arduino there were too many books and online sketches to actually learn systomatically - classic example of information overload. I had no peers to learn from and with no prior programming experience I was in over my head. Probably like many teachers, I was only using Tinkercad for 3D designs and didn't realize I could put the circuits side to work. Across the country, Google Trends shows that Tinkercad querries lag behind Arduino, and probably even less together. In my state ( Maine), there is a gap between rural and urban schools in Tinkercad and Arduino interest. Even still, there is no avenue for Arduino in code.org for MS or HS teachers. So, how can science/math teachers, like you and me (Machias Memorial HS, Machias,ME) begin learning and teaching Arduino?
Fortunately for me (and you), Tinkercad added circuits and Arduino in 2017 and has continued to expand. This was a huge support to me for integrating hardware + code to test online before building. It has since become my go-to source for teaching students and other teachers how to "Arduino".
In hindsight, after acquiring several books and surveying many websites for help and ideas, it didn't take me long to realize that there was not a complete resource that walks new-to-Arduino teachers step by step through learning. With all science teachers having some level of comfort with mathematics, it occurred to me that this is a perfect place to enter Arduino sketch writing. There is no burden of learning electronics basics or Tinkercad:circuits, just sketch writing. Mathematics, by nature, is very step by step logical progression oriented and matches how sketches should be approached.
Begin your journey learning Arduino basics using some simple mathematics to learn a few key basics by following the sections. By the end of these lessons you should be comfortable enough to forge deeply into both teaching Arduino to your students and become comfortable with circuits. More math lessons, as well as space research projects, can be found in Tinkercad search > People > jlenke > circuits. Information on Arithmetic operations in Arduino can be found in reference. There are of course operations; search for these by function name.
Supplies
The list of supplies is short:
- Laptop, Chromebook, or tablet with internet connection. (Tinkercad is a cloud based design platform.)
- Tinkercad account
- a fiction book or Textbook
Step 1: Tinkercad Account
Grades: 4- 12; Time: 5 - 20 min
If you don't already have a Tinkercad account, you'll need to open one. I highly recommend signing in with email. My school uses Google for Education, which makes it pretty easy. However, better check with the IT admin, just make sure all pertinent FERPA files are kept safe.
Since you are going to be using Tinkercad a bunch, best to get a shortcut or bookmark set up. My students use Chromebooks, and I had the IT admin install Tinkercad as an app that allows rapid access to their accounts since they are already logged in to the Chromebook.
Establishing a Classroom to keep track of students work is the best tool available. Instead of running all around the room answering questions, the classroom feature allows me to peek into a students design and work with them across the room or across town. Learn about setting up Classroom.
.
Step 2: Arduino Organization
Grades: 4- 12; Time: 10 - 30 min
- Grab, drag and drop the Arduino (far right side bottom) onto the white workspace.
- Click the Code button at TOP RIGHT.
- Below LEFT of CODE is drop-down menu, change to TEXT ONLY. Click CONTINUE to accept.
- You will find a basic, functional code for BLINK pre-installed.
Almost every book and example begins with BLINK as it is the simplest electronic circuit to control. Well that's cool, but how does that teach Arduino and where should you go next?
If you have never seen an Arduino sketch before, this can appear daunting. But, like any complicated task, breaking it down into smaller sections is easier. Taking a look at the text lines in colors, they are actually divided into four sections even though it doesn't appear that way. Knowing what each section does is vital to know where to type commands, functions or actions.
Section 1: sketch notes
This will appear as
// C++ code
//
Notice that is in a funny color resembling orange. More importantly, ANYTHING between the // and the other // are completely ignored by the sketch. Imagine the controller thinking
Silly human words.
This is very much equivalent to those very first pages in a book that contain the Title page, publisher, dedication, ISBN and some other stuff. Very important information, but read only once and probably never again.
For a sketch we would put in equivalent items:
- Author, Date
- Description
- Important links to websites or electronic sensors
- Notes to the reader or reminders to the Author
Section 2: declarations
//
void setup()
Between the last comment line // and the purple void setup(), is section 2 that contains important information about the story. In a book, this might be a description of characters or their name pronunciations, while in a science book it might be a list of scientific terms and their values.
This sketch has none but later examples will have items like variables and circuits would have PIN identifications.
Section 3: setup
void setup()
{
pinMode(LED_BUILTIN, OUTPUT);
}
This section is the setup that sets the stage for any actions, functions, or commands in the last section. Just like in a book at the beginning, characters and scenes must be developed. In our case, we need to prepare any electronic equipment that is going to be used. The built in example is stating that the mode or direction of the PIN location LED_BUILTIN is going OUT. However for doing math, the most common equipment used will be the display or Serial Monitor.
It is important to NOTE that the curly braces {begin and end} whatever actions are being asked of the controller.
Section 4: loop
void loop()
{
digitalWrite(LED_BUILTIN, HIGH);
delay(1000); // Wait for 1000 millisecond(s)
digitalWrite(LED_BUILTIN, LOW);
delay(1000); // Wait for 1000 millisecond(s)
}
This section is where all the actual commands and functions are carried out in a LOOP, meaning forever until power is pulled or the command has reached the point to stop.
In a book these would be each of the chapters developing the story. In the builtin example the PIN location LED_BUILTIN is being sent instructions digitally (digitalWrite) to tell it to be HIGH ( or on). Then delay for some period (1000). NOTE that all time is kept in milliseconds.
Step 3: Count to Infinity.. by 1
Grades: 4 - 12; Time: 20- 40 min
The very first thing just about anyone does with a calculator is to add 1 + 1 + 1 + 1.... until our fingers get tired or we get bored. But what if a controller does it that never gets tired? There are no buttons to push, so how can this addition be carried out for a long time?
You have already thought of something and/or peeked at the answer. So now that you have come back to read the details, THE MOST IMPORTANT FIRST STEP IS TO SEND OUR WORK TO THE DISPLAY. Otherwise, there will be no way of knowing what is actually happening.
In void setup() communication between the controller (Arduino) and screen must have the command to begin
Serial.begin(9600);
- For this function, the first letter is capitalized
- There is a period between the device and action (begin)
- The speed of communication is set (9600). If you have ever used a dial-up modem you'll recognize this as 9600 baud.
- EVERY line must end in a semicolon;
Ok - Now what are we going to add 1 to? The controller (Arduino) must have something to perform an action on and this is called a variable or "x". In Section 2: declarations the variable is declared and given an initial value of 0. However, variable works in math but not for Arduino. The equivalent called integer (int), is used.
int x = 0;
With a calculator we would hit the [+] and [1] buttons followed by [=]. We have to accomplish the same thing, except there is no [=] button because the loop will automatically do this over and over and over again. All we have to do is ask to SEE the result. This is done using a print line (println) command of the new value of "x" sent to the Serial monitor or display (Serial).
Serial.println(x);
But how is 1 added to x? Fortunately Arduino comes with a built in "add 1" system. This is
++x;
Which is similar but not the same as x++; For more seeArduino reference. There is also another way to do this by making the new value of x be equal to the old value of x +1.
x = x+1;
Pause, or delay, for a small period of time for the humans to read the answer and we have completed all the steps.
Of course this action all began with a { begin and end }. Along the way there are comments to the reader that have been kept safe using a double slash //, which prevents the controller from having to read those notes.
int x=0; // declare x as variable
// declare starting value of x as 0
void setup()
{ // begin setup instructions
Serial.begin(9600); //initiate communication with serial monitor
} // end setup
void loop()
{ // begin loop commands
++x; // ++ means to add (1)to starting value of x
Serial.println(x); // send value of (x) to serial monitor
//println = give new line (ln)
delay(100); // pause of 1/4 second or 250 milliseconds
// to allow user to read values
} // end loop
The link at the start of this section will have all of this ready to go. Now let's see how it works:
- Open the code by clicking Code
- Open the Serial Monitor by clicking Serial Monitor on the bottom
- Open the graph by clicking the squiggly line representing a graph
- Click Start Simulation
Recap: What was learned?
- The serial monitor (display) must be turned on and communication at the right baud rate initiated
- Curly braces { } are important
- A variable titled int x was used and initially set to 0
- The result was sent to the display
- 1 was added to x, continuously ( two ways to do it: ++x and x = x+1 )
- There are four sections to an Arduino sketch
- delay is in milliseconds
Next step
With your class, have them open the link and get them to:
- count to infinity by adding 2 instead of 1 ?
- grow exponentially by multiplying the old x by 2 and making that be the new x ?
- set an initial value for x of 1,000 and count down ?
- change the delay rate to match actual time so that the display acts like a clock and reports the number of seconds in use ?
- Have them set the delay to 1 min or 1 hr or 1 day? ( Remember time is ONLY in milliseconds)
Step 4: Plot All Absolute Values Between Two Given Numbers
Grades: 6 - 12: Time: 20- 40 min CC: 6-NS; 7-NS; A-REI; F-IF
The absolute value of any number | x | is always positive. Is it possible then, to count from -100 to +100 by increasing x by 1 and graph the result? For starters we need to declare x as a variable again and of course turn the serial monitor. But how do we count between two numbers without going under or over those numbers?
Enter the for loop. The for loop will repeat a small loop inside of our bigger main loop until a counter has been reached. The smaller for loop is ended, the larger main loop is ended and the process starts all over again. Seems simple enough. But what is the lingo. Arduino Reference has all of the basics answered if ever needed and the nitty gritty details can be found as Arduino Reference>for . But, the gist of a for loop is
for (initial setup; condition until done; increment by) {
doSomething; // Do something until the for condition has been reached
}
**Errors often happen because there isn't a matching parentheses or curly brace.
In our case, we want to begin x at some value until it reaches some value by increasing by some amount. This looks like
for (int x = -100; x < 100; x = (x+1))
The mini loop is begun with x beginning at -100, then x will increase until it reaches +100 and increases by +1. If we were doing this on a calculator we would enter: [abs](-100) [=] followed by [ans] [+] [1] [=]...... But this would never end!
As we learned, the MOST important thing to do is display the result which becomes our "doSomething".
The beauty of this graph is that the result will essentially count down to 0 from 100, and then begin counting up to 100. The absolute value of |-100| = +100. Then add +1, |-100+1| = +99; |-99+1|=+98....
Next step
With your class, could you get them to:
- start at different beginning and ending values ?
- change the amount of increase ?
- change the shape of the graph by either changing the increase by amount or the end points ?
- explain why the graph is the shape that it is?
- explain why the graph immediately turns around at 0 and 100?
- graph the square root of the new x ?
Step 5: Calculate the Square and Cube of Entered Number
Calculate the square and cube of any number
Grades 6 - 12. Time: 20 -- 40 min 8.EE; N-RN;
What if you want the user to enter a value so the sketch can do some math operation on it? First, the controller has to be told to look at the input and wait until something appears (entered). Then the entered value has to be assigned to a variable ( "x"), and then operations can be carried out. Of course, new tools will be needed. Specifically Serial.parseFloat().
To get the controller to wait until a value has been entered, the tool is
while (Serial.available() == 0)
Open the link above and simulate, then open the serial monitor and following the instructions.
It will become obvious that the display will reveal more information with some formatting along the way. Using quotation marks (". x. ") with or without spaces will cause the serial monitor to print words. Remember that println is a new line while print is just information right after each other.
Next step
With your class, could you get them to:
- wonder why the maximum is 60,000? What is ovf?
- determine the action to perform a square? A cube?
- change the display by increasing spaces or adding other sentences?
- explain why ONLY the last line is println?
- explain the difference between float and int?
- research what parseFloat and parseInt are?
- research what while is?
Step 6: Put It All Together
Can you encourage your students to put it all together on their own and improve on it?
- Open a new Designs>Circuits
- Under Components>Basic pull down and go to Starters>Arduino
- Select FADE
- Switch code from Blocks to Text ONLY. Accept by clicking CONTINUE
- Inspect the sketch
It should look fairly familiar, except that instead "x" the variable "brightness" is used. Also, now electronics are incorporated.
Each numbered location (PIN) on the Arduino can be considered a light. Numbers 2 -13 can be ON or OFF, which is called HIGH and LOW respectively. This is digital equivalent of 1 and 0. But what if we want an in between value, like a dim light? For this we have to send message on a different scale, or analog. To send a message to the light, we need to WRITE a letter to it. Put these together and it is either analogWrite or digitalWrite.
All that's left is to tell Arduino which PIN(location) and what amount.
analogWrite(9, 200);
This example will send the value or 200 to PIN 9 and stay in that condition until power is lost or it is told to turn off. Which is
analogWrite(9,0);
This sketch will substitute the variable brightness instead of a single number. This is because it will be changing. Additionally, two for loops will be used. The first one counts UP and the second DOWN.
Next step
With your class, could you get them to:
- increase the step size from 5 to 50 or 100? What do they notice?
- what if the delay is increased as well? When can the eye no longer see the change?
- add lines to ask the user to select the step size? the minimum or maximum value ?
- add a second LED and have the second become dim while the first is becoming brighter and vice versa?
Step 7: Release the Curiousity
Tinkercad has a number of starter examples for Arduino. Now that your students have mastered some of the basics, it's time to encourage to explore other starters and see what they can create. The easiest way to do this is to merge two different starters. It is also good to have a general theme or goal to try to achieve. I do a lot of projects and research around space. Not ALL space projects have to leave the ground however. Being able to grow crops in an autonomous system will be needed for longer term space travel and lunar missions. How can LED's, relays, and fans be used to crow microgreens in two weeks unattended by a human?
Whatever your interests are push yourself to learn a new skill and see where you can go.
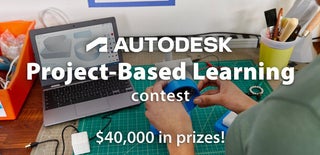
Runner Up in the
Project-Based Learning Contest