Introduction: Arduino | Maze Solving Robot (MicroMouse) \ Wall Following Robot
Welcome, I'm Isaac and this is my first robot "Striker v1.0".
This Robot was designed to solve a simple Maze.
In the competition we had two mazes and the robot was designed to able to identify them and solve both.
Any changes in the maze may need changes in the code or the design but it's easy to do.
Step 1: Parts
First, You need to know what are you dealing with.
Robots = Electricity + Hardware + Software
1- Electricity: batteries have many specs you should only know how much Current and Voltage you need.
2- Hardware: " Body, Motor, Motor Driver, Sensors, Wires and The Controller " you should only get the important parts that do the task, no need to get a fancy expensive controller for a simple task.
3- Software: The code is all about logic. Once you understand how the controller works it will become easy to build the functions.
Parts List:
- Arduino UNO
- 12v DC motors (x2)
- Wheels (x2)
- Motor Driver (L298N)
- Distance Sensor (Ultra Sonic)
- Wires (Jumper wires 1-pin male-male)
- 12v Battery (1000 mAh)
Tools List:
- Battery Charger
- Acrylic sheet
- Soldering Iron
- Wire cutter (optional)
- Nylon Zip Wrap
For Extra fun you can use LEDs to light it up but it's optional.
Step 2: Body Design
The main Idea was to stack the parts above the body and use the Nylon Zip Wraps to wrap them all together thanks to their lightweight.
I used CorelDRAW to design the body, check the designs above and I made extra holes in case of any future changes.
I went to a local workshop to use their laser cutter to cut the acrylic sheet, then I stacked the parts on it.
Later, I made some changes cuz the Motors were longer than I expected.
your robot doesn't have to be built the same way as mine. also the design is very flexible you can get creative.
Design PDF file and the CorelDRAW File are attached.
If you weren't able to laser cut the design, just buy the body from any store if its available.
Step 3: Implementation (building)
The design was made to fix the sensors on the body.
Step 4: Wiring
Here is a schematic diagram of the connections. connections are tied to the code and you can change the connections in the code if you want to use other pins.
Example: if you're connecting the motor driver to pin 2 but want to change it to pin 3, change the wire pin and change the code to pin 3 as well.
Sensors
Lets talk about "The Ultrasonic sensor"
An Ultrasonic sensor is simple radar that measures the distance of an object by using sound waves. It does that by sending out a sound wave and wait for it to bounce back, it records the time it took for the round trip and divides it by 2 to get the distance.
Ultrasonic Sensor connections:
- GND: connect this to the Ground.
- VCC: connect to the power source 5 voltages.(Alert! if you connect it to more than 5v it will be damaged)
- Echo: connect this to any pin on the Arduino. (match it to the code)
- TRIG: connect this to any pin on the Arduino. (match it to the code)
Remember: All grounds (sensors, Arduino, Driver) should be connected together to the battery, so make a common Ground that leads to the battery and connect all GND pins to it.
For sensors power source (Vcc), also group the 3 Sensors to a one 5v Pin, you can use the Arduino 5v pin or the Driver 5v pin, I recommend the Driver.
--------------------------------------------------------------------------------------------------
Motor Driver
The L298N H-bridge: it controls the speed and direction of two DC motors.
it can be used with motors that have a voltage of between 5 and 35V DC.
It also has an on-board 5v regulator (5v Supply), so if your battery voltage is 12v you can use the on-board 5v to supply the sensors or any other parts.
Pins map (Check the drivers picture):
- DC motor 1 “+” > connect this the motor #1
- DC motor 1 “-” > connect this the motor #1
- 12v jumper > keep this connected to enable the 5v regulator.
- Power Source > Connect your battery positive here
- GND > connect this the battery negative
- 5v output (if 12v jumper in place) > connect the sensors here
- DC motor 1 enable jumper > Remove the jumper and connect it to the Arduino this is used to control the speed of motor 1 (match it to the code).
- IN1 Direction Control > connect it to the Arduino this is used to control the direction of motor 1 (match it to the code).
- IN2 Direction Control > connect it to the Arduino this is used to control the direction of motor 1 (match it to the code).
- IN3 Direction Control > connect it to the Arduino this is used to control the direction of motor 2 (match it to the code).
- IN4 Direction Control > connect it to the Arduino this is used to control the direction of motor 2 (match it to the code).
- DC motor 2 enable jumper > Remove the jumper and connect it to the Arduino this is used to control the speed of motor 2 (match it to the code).
- DC motor 2 “+” > connect this the motor #2
- DC motor 2 “-” > connect this the motor #2
Note: This Driver allows only 1A per channel, draining more current will damage the IC. this simply means that each motor should only drain 1A Max.
------------------------------------------------------------------------------------------------------
Battery
I used 12v Battery with 1000 mAh.
The table Above shows the voltage drop when the battery discharges. just keep it in mind and recharge the battery when it drops.
To calculate how much time you have:
Discharge time is the battery capacity (Ah or mAh) divided by the current drained.
Example: for a 1000mAh battery with a load that draws 300mA you have:
1000/300 = 3.3 hours
logically if you drain more current you get less time. just make sure that you don't exceed the Battery Discharge Current Limit or it will be damaged.
Again remember to connect all grounds to a common Ground to the battery negative.
Step 5: Coding
I had fun coding this robot. so make sure to enjoy that as well.
The main goal is to get out the maze without hitting the walls.
I had 2 simple mazes so I build it to use 2 algorithms:
- The blue maze uses right wall following algorithm.
- The red maze uses left wall following algorithm.
The photo above shows the way out in both mazes.
if you want to force only one algorithm please check the following
I made a code that can solve both mazes, so to be able to know which wall to follow I made those 3 variables:
1- first_turn = false ;
2- rightWallFollow = false ;
3- leftWallFollow = false ;
so at first they are all set to false and the robot will only go forward until it finds the first turn, if the turn is right, then the robot will follow the the right wall, and it will set first_turn and rightWallFollow to true and when it can't detect any walls it will stop (assuming that it is out of the maze). If the first turn was left then it will set first_turn and leftWallFollow to true and follow the left wall.
if you want to overwrite that you can always set them true as you want, for example:
to make the robot follow the right wall:
first_turn = true ;
rightWallFollow = true ;
leftWallFollow = false ;
if you want it to follow the left wall:
first_turn = true ;
rightWallFollow = false ;
leftWallFollow = true ;
- for readsensors I found 2 methods to get sensor readings, one kinda slow but accurate and the other is fast with less accuracy, So I put both in that section but I commented out the slow ones.
That means it's there but it's not used. to use it just uncomment it and comment the other one. If you want to use it just remove the "//" and comment out the other one as shown in the photo.
I separated each function to make the code more simple (also you can find some lines that were commented out that were used for experiments). so the main flow is the code runs the main function and calls out the other functions as needed.
Code flow:
- defining the pins
- defining output and input pins
- check sensors' readings
- use sensors' reading to define walls
- check first route (if it was left then follow the left wall, if it's right follow the right wall)
- Use PID to avoid hitting the walls and to control motors' speed
You can use this code but change the pins and the constant numbers to get the best results.
Follow This Link for the code.
https://create.arduino.cc/editor/is7aq_shs/391be92...
Follow This Link for the library and the Arduino Code File.
Step 6: Have Fun
Make sure to have fun :D
This is all for fun don't panic if it's not working or if there is any thing wrong. track the error and don't give up.
Thanks for reading and I hope it helped.
E-mail:
Is7aq.s7s@gmail.com
Follow me on LinkedIn:
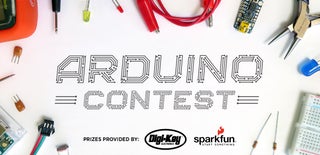
Participated in the
Arduino Contest 2017