Introduction: Micro:bit Messenger
I created this Instructuble to enter it into the Micro:bit Contest. It is a simple circuit to make it easy for classrooms to recreate. It uses pins 0,1 and 2 for selecting a letter, A is a space, B is delete and AB is to send the message. This will broadcast to all microbits in the same radio group of 99.
Supplies
For each messenger you will need:
- Micro:bit
- 3 tactile buttons (should be able to use capacitve touch on the pads)
Step 1: Connect It All Up
NOTE: You should be able to do this using the the pads as capacitve touch sensors and not use tactile buttons.
These directions will need to be repeated for each messenger.
- Connect the first tactile switch to the micro:bit on Pin 0 and the other side of the tactile switch to ground
- Connect the second tactile switch to the micro:bit on Pin 1 and the other side of the tactile switch to ground
- Connect the third tactile switch to the micro:bit on Pin 2 and the other side of the tactile switch to ground
You can look at the image for reference for how the connection should be setup.
Step 2: Code It Up
We are using micro:bit python for scripting this up.
1. We setup the radio to communicate on channel 99. You can change this value to something else but it will have to match on all devices that need to communicate with each other.
radio.set_group(99)
2. Setup global variables.
letters = "0123456789ABCDEDFGHIJKLMNOPQRSTUVWXYZ!@#$%^&*()_-=+" # All posible letters message = "" # Place to store are message index = 0 # Store index of last selected letter
3. Define function for showing the full message
def show_message(): basic.clear_screen() basic.show_string("{" + message + "}")
4. We have pin 0 is wired to the first button and will go to GND(LOW) if pressed. Our method we define a method that subtracks 1 everytime that button is pressed. Then we convert that index to to a letter and display it.
def on_pulsed_p0_low(): global index global letters index = (index - 1) if index < 0: index = 0 basic.show_string(letters[index]) pins.on_pulsed(DigitalPin.P0, PulseValue.LOW, on_pulsed_p0_low)
5. Now we handle pin 1 which increments the index up and displays the new letter
def on_pulsed_p1_low(): global index global letters index = (index + 1) if index > len(letters)-1: index = len(letters)-1 basic.show_string(letters[index]) pins.on_pulsed(DigitalPin.P1, PulseValue.LOW, on_pulsed_p1_low)
6. Now pin 2, here we assign the current letter to message.
def on_pulsed_p2_low():<br> global message global letters global index message += letters[index] show_message() pins.on_pulsed(DigitalPin.P2, PulseValue.LOW, on_pulsed_p2_low)
7. I could have added space into the letters but thought it would be more helpful to have it assigned to the A button.
def on_button_pressed_a(): global message message += " " show_message() input.on_button_pressed(Button.A, on_button_pressed_a)
8. Then we need a way to remove characters from the message, to do that we use button B.
def on_button_pressed_b(): global message global letters global index message = message[0:-1] show_message() basic.show_string(letters[index]) input.on_button_pressed(Button.B, on_button_pressed_b)
9. We need to send the message to other devices. We assign this by pressing both button A and B at the same time
def on_button_pressed_ab(): radio.send_string(message) input.on_button_pressed(Button.AB, on_button_pressed_ab)
10. Lastly, we need to handle receiving the message
def on_radio_received_string(arg0): basic.show_string("{" + arg0 + "}") radio.on_received_string(on_radio_received_string)
Here is the complete code:
radio.set_group(99) letters = "0123456789ABCDEDFGHIJKLMNOPQRSTUVWXYZ!@#$%^&*()_-=+" # All posible letters message = "" # Place to store are message index = 0 # Store index of last selected letter basic.show_icon(IconNames.Happy) def show_message(): basic.clear_screen() basic.show_string("{" + message + "}") def on_pulsed_p0_low(): global index global letters index = (index - 1) if index < 0: index = 0 basic.show_string(letters[index]) pins.on_pulsed(DigitalPin.P0, PulseValue.LOW, on_pulsed_p0_low) def on_pulsed_p1_low(): global index global letters index = (index + 1) if index > len(letters)-1: index = len(letters)-1 basic.show_string(letters[index]) pins.on_pulsed(DigitalPin.P1, PulseValue.LOW, on_pulsed_p1_low) def on_pulsed_p2_low(): global message global letters global index message += letters[index] show_message() pins.on_pulsed(DigitalPin.P2, PulseValue.LOW, on_pulsed_p2_low) def on_button_pressed_a(): global message message += " " show_message() input.on_button_pressed(Button.A, on_button_pressed_a) def on_button_pressed_b(): global message global letters global index message = message[0:-1] show_message() basic.show_string(letters[index]) input.on_button_pressed(Button.B, on_button_pressed_b) def on_button_pressed_ab(): radio.send_string(message) input.on_button_pressed(Button.AB, on_button_pressed_ab) def on_radio_received_string(arg0): basic.show_string("{" + arg0 + "}") radio.on_received_string(on_radio_received_string)
Step 3: Done
All you have to do is repeat this for multiple micro:bit controllers and you will be up and running to send messages back and forth.
See it in action:
Play with it yourself:
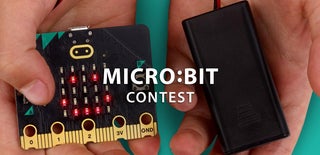
Participated in the
Micro:bit Contest