Introduction: Motion Detecting Night Light
Are you tired of bumping into stuff when you wake up for midnight snack or fumbling with a switch in absolute darkness or even stepping on someone's head when trying to use the washroom? (Trust me it happens....)
This simple project would take all that away in just about 15 minutes. So are you ready to take your Nights to the next step? The Night light uses an LDR (Light Detecting Resistor) or a Photoresistor to detect the absence of light. If such an absence is detected along with the detection of motion by the PIR or Passive Infrared sensor the light would switch on. In the presence of light, the lights would remain switched off regardless of the detection of motion.
Supplies
- An Arduino. You could use any Arduino for this project. However, I would be using the Arduino Uno for the sake of simplicity. Cost: $ 3 to $ 50 (USD) depending on which version you buy.
- An LDR (Light Detecting Resistor) or a Photoresistor. Cost: 15 to 20 Cents (USD).
- A PIR or Passive Infrared Sensor. Cost: $ 5 to $ 9 (USD)
- A 100k Ω Resistor.(Brown-Black-Yellow-Gold)
- A couple of Male-to-Male and Male-to-Female wires
- The sixth and most important requirement of all is a spirit of Curiosity. It is this spirit that inspires each and every one of us and brings light to the darkness. (Pun Intended)*
*Our project does just that......
Step 1: Connecting the LDR
An LDR is a component that has a (variable) resistance that changes with the light intensity that falls upon it. This allows them to be used in light sensing circuits and thus can be used to detect the presence or absence of light. Attach the LDR using the following steps
1) Connect the 5v pin of the Arduino to the positive rail of the breadboard.
2) Connect the GND pin of the Arduino to the negative pin of the breadboard.
3) Connect one pin of the LDR to the positive(+5v) rail of the breadboard.
4) Connect the other pin of the LDR to the Analog Input Pin 0 (A0) of the Arduino. Note: This can be any Analog In Pin provide that "#Define" value is changed in the final code
5) Connect the same pin to a 100kΩ resistor. (Brown-Black-Yellow-Gold)
6) Connect the other end of the resistor to the GND Rail.
7) The LDR is now connected. To test the LDR upload the following code in the Arduino. (You can skip this step if you can confirm that your LDR is Working).
/* === LDR Test Code with Serial Monitor===by Shriyans, https://www.instructables.com/id/Night-Light-Moti...>> */ #define LDR A0 int Brightness = 0;void setup() { Serial.begin(9600); pinMode(LDR,INPUT); } void loop() { Brightness = analogRead(0); Serial.print(Brightness); delay(100); }
8) Open the Serial Monitor with the Baud Rate of 9600. We will notice that the values between 0-1023 are printed. This is because the Arduino, with its built-in ADC (analog-to-digital converter), converts the analog voltage from the LDR (0-5V) into a digital value in the range of (0-1023).
9) In a bright room, the Arduino gives a value of about 700-1023 whereas, in a dark room, the Arduino gives values of 0-700.
Step 2: Connecting the PIR Sensor.
The PIR sensor itself has two slots in it, each slot is made of a special material that is sensitive to IR. When there is no motion, both slots detect the same amount of Infrared rays. When a warm body passes by the sensor, it first intercepts one half of the PIR sensor, which causes a differential change between the two halves of the PIR. These change pulses are what is detected. Thus, Motion can be detected through this module. To use this module do the following steps.
1) Connect the VCC(+) pin of the PIR sensor with the positive rail (+5v) of the Breadboard. 2) Connect the GND pin of the PIR sensor with the GND rail of the Breadboard 3) Connect the output pin of the PIR sensor with Pin 2 of the Arduino. Note: This can be any Digital I/O Pin provided that the "#Define" value is changed in the final code 4)To test the PIR upload the following code in the Arduino. (You can skip this step if you can confirm that your PIR is functioning properly.
/* === PIR Sensor Test Code with Serial Monitor=== by Shriyans , https://www.instructables.com/id/Night-Light-Moti... */ #define pirPin 2 //the digital pin connected to the PIR sensor's output //Note:This can be any Digital I/O Pin void setup() { Serial.begin(9600);//Starting Serial Monitor with a Baud Rate of 9600 pinMode(pirPin, INPUT);} void loop() { if (digitalRead(pirPin) == HIGH){ //If motion is detected... Serial.println("== Motion Detected ==");} else{Serial.println("== No Motion ==");} }
5) Move your hand in front of the sensor after about 15 seconds and check if Motion is detected, via the serial monitor in order to confirm the proper functioning of the PIR sensor. 6)The Distance Sensitivity and Time Delay can be set through the rotation of their respective potentimeters
Step 3: Connecting the LED
1) Connect the Positive end (Longer one)of the Led to pin 11 of the Arduino.
Note: This can be any PWM pin (3,5,6,9,10,11 on Arduino Uno and pins 2-13 on the Mega) provided that the "#Define" value is changed in the final code. 2) Connect the GND end of the Led (Shorter one) to the GND rail of the Breadboard. 3) Recheck all the connections and refer to the Schematic in order to proceed to the next step.
Step 4: Uploading the Code
Upload the following code into the Arduino.
If it is dark to a certain point only then the PIR would detect motion and the led would light up. Also, the degree of darkness of the room would increase the degree of brightness of the Led.
Arduino Create Link: Here
Fritzing Link: Here
<p>/* === Arduino Motion Detecting Night Light===<br> with Serial Monitor by Shriyans Gandhi , */ #define pirPin 2 //the digital pin connected to the PIR sensor's output //Note:This can be any Digital I/O Pin #define ledPin 11 //the PWM connected to the Led strip output //Note:This can be any PWM pin (3,5,6,9,10,11 on Arduino Uno and pins 2-13 on the Mega) #define LDR A0 //the Analog In Pin connected to the LDR's input //Note:This can be any Analog In Pin int Brightness = 0; //Declaring the variable "Brightness" int Darkness = 0; //Declaring the variable "Darkness" int calibrationTime = 30; //the time we give the sensor to calibrate void setup(){ Serial.begin(9600); //Starting Serial Monitor with a Baud Rate of 9600 pinMode(pirPin, INPUT); //Setting the pinmodes of the PIR sensor pinMode(ledPin, OUTPUT); //Setting the pinmodes of the Led pinMode(LDR, INPUT); //Setting the pinmodes of the LDR digitalWrite(ledPin, LOW);//Switching off the led strip by default //Give the sensor some time to calibrate (This piece of code prints dots on the Serial Monitor to show that conection with Arduino is stable.) Serial.print("Calibrating sensor "); for(int i = 0; i < calibrationTime; i++){ Serial.print("."); delay(1000); } Serial.println("PIR Activated"); delay(50); } void loop(){ delay(1000); Brightness = analogRead(LDR); Darkness = Brightness-700; Serial.println(Brightness); if (Brightness < 700 ){ //If it is dark to a certain point only then the PIR would detect motion and the Led would light up Serial.println("It is Dark."); if (digitalRead(pirPin) == HIGH){ //If motion is detected... Serial.println("Motion Detected...Light is on"); analogWrite(ledPin,Darkness/4);//the degree of darkness of the room would increase the degree of brightness of the Led //Darkness is divided by four as the LDR gives values of (0-1023),but Arduino can only Analog write the PWM values of (0-255) delay(100);} //A pause for 100 Milliseconds else{ Serial.println("No Motion Detected."); digitalWrite(ledPin, LOW);}//If Motion is not Detected the Led is not lit up } else{ Serial.println("It is not Dark."); digitalWrite(ledPin, LOW);}//If it is not dark enough the Led is not lit up }
Step 5: In the Future
Though this project is quite simple its possibilities are endless.This is just a basic glimpse of this project's potential. Some little tweaks and this same project can be used as an intrusion detection system, an energy-saving device or even an autonomous movement tracker. The Use-case scenarios are infinite. This can be used anywhere from 7000 ft down in mine shafts to all the way up in the International Space Station.
Just let your imaginations flow ...
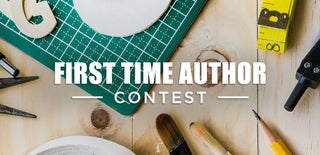
Participated in the
First Time Author Contest