Introduction: Movable LED Disc Light Using Arduino
For a school project I was tasked to use an Arduino to make something interactive. I really wanted to do something with light. The result ended up becoming a disc-shaped light that you can move around on a flat surface to move it over to a different color.
The colors and their position in the virtual plane are relatively easily programmable. you could even create little games to play by moving the disc around.
This might sound very complicated, but the only things you need to recreate the same principle are:
- an Arduino
- an LED-strip
- a USB optical mouse
In this instructable I will show you how I made this disc and show you how you could make one too, if you so desire..
Step 1: Preparing Your Mouse
To start off, We'll have to disassemble the USB mouse.
Before doing anything to the mouse, I would suggest plugging it in a PC or laptop to check if it still works. The mousebuttons and scrollwheel don't have to work, as long as you can move the cursor on your screen, we can use that mouse for this project.
We don't actually need all of the parts of the mouse. We are looking for the optical sensor and its lens.
The optical sensor is the part of the mouse with the light that shines through the bottom. The lens is the part made of transparent plastic right under the optical sensor.
When ready, Disassemble your mouse and cut off the connector part of the USB cable connected to the mouse. You should find at least 4 smaller cables inside with different colors. Strip the outer part of the USB cable so you can separate the wires inside and plug them into your arduino. The wires should be connected as folows:
- A red or orange wire should be plugged in the 5v pin of the Arduino
- A White wire, which is the data wire, plug it into pin 5*
- A green wire, which is the clock wire, plug it into pin 6*
- A black or blue wire, which is the ground wire, put it in one of the GND pins
Once you've done this, you willl need some software to fetch the sensor's data. For this I am going to redirect you to another instructable which I used to figure all of this out. In step 3 he shows how to get the data of the change in X and Y directions. He also goes in further detail about the use of the sensor of a mouse, so if you're still confused, please read through it here.
*If you want to, you can change these pins later in the code, but these pins are the standard.
Step 2: Tools and Materials
To make this disc, you will at least need:
Technology-wise:
- a USB optical mouse
- a programmable RGB LED strip
- an Arduino
- some wires
- A power source
For the design/outer shell:
- Two sturdy plastic plates
- A sheet of frosted see-through plastic
- roughly 5 mm thick wood (for laser cutter)
- Glue and/or screws for construction
If you want to make the disc exactly like I did you will also need access to a laser cutting machine.
Step 3: Getting the Sensors X and Y Position
Once you've downloaded the PS2Mouse library, you can check if your sensor is transferring the data by loading the example sketch PS2Mouse.ino onto your Arduino. If everything went right, the sensors light will turn on, and when checking the Arduino IDE's Serial monitor you should get a continuous stream of X and Y differences.
If everything works, we can continue to change this example code so that it doesn't just give a difference, but a position. You can do this by changing this piece of code:
Serial.print(stat, BIN);
Serial.print("\tx=");
Serial.print(x, DEC);
Serial.print("\ty=");
Serial.println(y, DEC);
And replacing it with:
if (x != 0 and y != 0)
{
xpos += x;
ypos += y;
} Serial.print("x=")
Serial.print(xpos);
Serial.print(" y=");
Serial.println(ypos);
Also, add this just before the "void setup(){" line
int xpos = 0;
int ypos = 0;
If you did all that and check the Serial monitor now, you should see that it gives back a stream of x and y positions instead of differences. We have done this by simply adding the difference of both x and y positions on separate integers, xpos and ypos. If you start or reset the Arduino, it will start back on 0,0.
Once this works, we are done with the mouse! When we add the LED-strip, we can let the lights communicate with the x and y position of our sensor to navigate through the colors we tell it to display.
Step 4: Adding the Lights
Now we will need to connect the LED-strip to our Arduino. I cut my strip to a length of 28 LEDs, because I thought it would make for a nice sized disc and this amount is easier to make the code with later than say, 29 or 30 LEDs.
Now you should be able to make this disc work with any programmable RGB LED strip, but depending on the type of LED-strip you have, you will need different code and/or a different library. The type of strip I used is a Dotstar.
Some LED-strips already come with pre-soldered cables. If yours does, you can use these to connect to your Arduino, otherwise you will have to add the wires yourself. Lucky for us, the way to connect these wires is almost identical to those of the USB mouse. The 5v needs to connect to the Arduino's 5v, the GND to the Arduino's GND, and the other two wires have to go into pins. In my case I put the Clock wire in pin 13 and the Data wire in pin 11.
I would suggest finding the example/test code (usually called "strandtest") online, taking its setup and adding it to our already existing code. Also, it is obviously not a bad idea to test if your LEDs all work like intended.
Step 5: Coding
To make the LEDs react correctly to the position of our soon-to-be disc, we need to give every LED a position around the center of it (which will be the optical sensor). I Chose to make two big lists in my code, one with all the 28 LEDs x positions, and one with all the y positions, both in the right order:
int ledxlist[] = {-295,-285,-262,-229,-182,-128,-62,0,62,128,182,229,262,285,295,285,262,229,182,128,62,0,-62,-128,-182,-229,-262,-285};<br> int ledylist[] = {0,-62,-128,-182,-229,-262,-285,-295,-285,-262,-229,-182,-128,-62,0,62,128,182,229,262,285,295,285,262,229,182,128,62};
We can use these lists to cycle through every LEDs x and y position from the middle of our disc, and adding the difference every loop so it follows every movement of the disc.
This code will do this for you, put it in the void loop above our previously added code:
int i,x,y;<br>for (i = 0; i < 28; i = i + 1){ if (x != 0 and y != 0){ ledxlist[i] = x + ledxlist[i]; ledylist[i] = y + ledylist[i]; } //PUT YOUR COLOR BLOCKS HERE }
If you did this right, the values in both your lists should change exactly the same amount as the corresponding xpos and ypos values we get from the mouse sensor. At this point we just have to assign some blocks of color.
(ledylist[i] > (LOW_Y_EDGE))and (ledylist[i] < (HIGH_Y_EDGE)) and (ledxlist[i] > (LOW_X_EDGE))and (ledxlist[i] < (HIGH_X_EDGE)){<br>strip.setPixelColor(i,(COLOR));<br>strip.show();<br>}
This is the basic setup I used to assign a block of color. If you replace all the (FULL CAPS) parts with values you can assign a rectangle of color.
For example: if you want a square of size 400 around 0,0 you put in -200 for LOW_Y_EDGE and LOW_X_EDGE and 200 for HIGH_Y_EDGE and HIGH_X_EDGE.
The color has to be a color code. You can use an online color picker to find the codes of specific colors.
You can add more than one of these blocks of color to your virtual color plane, so you can move around to and from the different colors you assigned to different positions.
If all of this works, you should be able to move your mouse sensor over a flat surface to move through your color plane. If it all works, we can finish it by making a nice casing for it all!
Step 6: Making the Outer Shell
Now that we have all the electronics sorted, we can start finishing off our disc by making the disc itself.
To start off I drew out a layered skeleton in Illustrator, which I lasercutted out of some 5 mm thick wood.
I glued the skeleton together, placed the mouse sensor at the bottom, the Arduino on the middle circle. The LED-strip I I glued around the middle circle, with the LEDs facing outward.
I personally wanted to still be able to remove the Arduino easily if necessary, so I secured it with some small wooden slats instead of gluing it onto the skeleton.
The mouse sensor is something that might need some attention. The lens has to be above the underlying surface at a specific height to work correctly. It is very likely that if you make the lens level with the botton of your disc, it will not work when you set it down, so be careful about that.
Once everything is secured and sturdy, we can finish off the design. I took two sturdy plastic plates to cover the top and bottom. This turned out to work very nicely, as the LEDs slightly shine through the plastic, giving the plates a nice soft glow when the disc is turned on. Keep in mind that the bottom plate needs to have a hole in it for the lens of the sensor to read the surface.
I glued the bottom plate to my skeleton, but decided to use screws for the top plate. This way the electronics were still gonna be accessible when I needed to change or fix anything later.
We are almost done. To finish of the design I used a see-through frosted plastic sheet between the gap left by the two plates. I cut out a long rectangle and wrapped it around like a cilinder, so it would cover up the bare LEDs and the electronics inside. The frosted plastic should diffuse the light of the LEDs, turning it into a nice, smooth light source.
And that's it! With some more complicated coding it would be possible to even create moving blocks of color and even little games.
Thanks so much for reading! This is my first instructable and I am not the best at explaining things, so if you still have questions feel free to ask me. Goodbye! :D
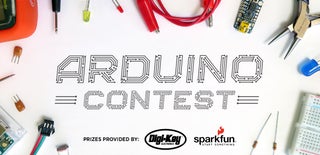
Participated in the
Arduino Contest 2017