Introduction: Musical DoodleBug on Circuit Playground
This is a variation of the classic “tone command” tutorial – with a few major twists – and a cute face.
Using one Arduino board – no wires, no soldering or extra components – the tutorial shows how to:
- · Create a touch-sensitive 12-note musical keyboard,
- · Change octaves (60+ notes) just by tilting the board.
- · Change the tempo by tilting the board
- · Change from “single-note” to automatically generated arpeggios.
- · Use buttons to switch between major, minor and blues chords.
- · Colorful RGB LEDs light up while you play.
- · Use simple math to generate chords instead of hardcoded note-names
Successes and Failures
I made this as a teaching tool for use with my local Maker Group. I tried to provide some fun activities for every age and skill level, kids through adults.
The code works fine, but it is more of a framework. It is a next step after playing with the basic “pitch library” and named notes, a way to explore the math behind musical intervals and chording. The illustrations are also an attempt to finally create some reusable graphics for use with my local Maker Group, to find the right mix of engaging vs informative, and to create old-fashioned printable hand-outs that we can reference as a group.
The project is meant to be the first of a multi-part series. This first project uses the basic "tone" command, but it introduces basic techniques that will be useful if you are planning on working with MIDI on Arduinos. Most MIDI Arduino implementations controls notes using numbers, not note names. So being able to navigate musical structures via mathematical relationships is very useful.
Or if you want to re-configure this project to act more like a guitar or bass, then the mathematical technique of specifying relationships will also make chords and neck positions easier to accomplish in any tuning.
And as a teaching tool --- there should be something to annoy beginners and experts alike. (You're welcome)
Beginners will need to:
- · understand how to work with an array
- · coordinate multiple inputs to determine which array element to use
- · set and release status-flags
- · pass data to a function outside the main loop
- · use the result from the function while back inside the main loop
- · understand how to test if two Booleans are simultaneously true
- · understand nested tests for combinations of buttons and switches
- · use all of these to work with musical chord intervals using math
Experts will be annoyed because:
- · Not all repetitive actions have been turned into functions
- · After using math, we regress to text based musical terms
- · There is no error checking or trace outputs
- · Sensor data is not properly “conditioned” or restrained
- · Buttons and pads are not de-bounced
- · Variable names are annoyingly long
When a user reaches the limits of this project they might be inspired enough to learn more about timers and interrupts, MIDI and polyphony etc. (like this MIDI Arduino guitar).
Step 1: Tools and Materials
Okay, there isn't much to talk about here. Unlike previous "Tone" tutorials (or Accelerometer, or Capacitive or Neo Pixel) there is nothing to do besides plugging in a board and starting.
Hardware
Circuit Playground board from Adafruit – that’s it, that’s all the hardware you need.
This board is a fantastic all-in-one Arduino compatible board that is loaded with sensors and outputs. The board features:
- · ATmega32u4 Processor, running at 3.3V and 8MHz
- · MicroUSB port for programming and debugging with Arduino IDE
- · USB port can act like serial port, keyboard, mouse, joystick or MIDI
- · 2-pin JST power connector
- · 10 x mini NeoPixels, each addressable RGB LED one can display any color
- · 1 x Motion sensor (LIS3DH triple-axis accelerometer with tap detection, free-fall detection)
- · 1 x Temperature sensor (thermistor)
- · 1 x Light sensor (phototransistor)
- · 1 x Sound sensor (MEMS microphone)
- · 1 x Mini speaker (magnetic buzzer)
- · 2 x Push buttons, left and right
- · 1 x Slide switch
- · 8 x alligator-clip friendly input/output
- · Includes I2C, UART, and 4 pins that can do analog inputs/PWM output
- · All 8 pads can act as capacitive touch inputs
- · Green "ON" LED so you know its powered
- · Red "#13" LED for basic blinking
- · Reset button
- · Power and ground pads for adding extra components
- · Size = 2” diameter (~51mm)
That’s a lot of power for about $20 US.
If that’s not enough, the board is extensible. The I2C bus is available so you can add a chain of additional sensors, displays and outputs. The serial RX/TX line is also available so you can talk directly to other boards bit by bit.
(I’m using an early release “Developer Edition” of the board, so be sure to check for the latest production version. Adafruit is already publishing Circuit Playground tutorials, so be sure to check there for the latest info.)
Did You Notice?
There are no schematics here, no wiring and hook-up diagrams, no extra parts or instructions for soldering. Why is that? Because all those things have already been taken care of for you; just plug in the Circuit Playground and start coding.
Libraries and Supporting Code
Adafruit seems to be positioning the Circuit Playground board for the education and new-maker markets. So they have gone to great lengths to make it easy to program right out of the box. If you are familiar with any Arduino, then writing code for the Circuit Playground board will be easy.
But there are some differences:
- There is no set-up code required for the built-in sensors and outputs. That’s because the folks at Adafruit have installed, named and pre-configured almost everything on the board and hidden all those annoying pin declarations, etc. It is ready to go straight out of the box
- .
- You can still attach additional components to the board (potentiometer, motor, LCD screen etc.), but you will have to set up the external hardware the old fashioned way (define the pin, set the mode etc.) and load any needed libraries.
- All the functions for the built-in sensors and outputs have been wrapped into a single library with a consistent format. No need to load multiple libraries, create names for all your sensors etc. Just ask for something like “CircuitPlayground.temperature()” to get the thermistor’s value, or “CircuitPlayground.motionZ()” to get a reading from the accelerometer.
Visit Adafruit's learning guide and Circuit Playground tutorials for more, in-depth info about using the Circuit Playground and to download the latest library and drivers. I am using the early-release development board, so be sure to get the latest files.
The Project Sketch
Download the attached .INO file and load it into your board. I tried to provide helpful comments inside the sketch. The project might seem simple, but it does include some techniques that can be difficult for new programmers to grasp.
Attachments
Step 2: Concept and Basic Program Flow
Because the Circuit Playground board has all the required components built in and pre-configured, we can concentrate on learning more sophisticated programming techniques. These techniques are a good introduction to working with musical “intervals” and chord structure.
Finding the Right Tone (aka Pitch or Frequency)
This project is based around the Arduino “Tone” command. It uses the Tone command to create musical notes on the Circuit Playground’s built-in speaker.
The Arduino IDE’s built-in example for using the Tone command (File/Example/02.Digital/toneKeyboard) uses the popular “Pitches” library to create accurate musical notes. The Pitches library is a collection of named frequencies. You can play a certain frequency (a musical note) by telling the Tone function to (for example) play NOTE_C4. This is really easy and fun, at least for a short time. But hand typing every single note and duration becomes tedious quickly.
So to make it easier to work with, this project modifies the Pitches library and turns the collection of frequencies into an “array” called “notePitch[]” Since an array acts like a numbered list, it becomes easy to ask for the frequency stored in a specific location in the array – “Give me the frequency contained in the 48th slot in the array,” and, “Now give me the frequency that is four slots away.” This gives us a lot of power and allows us to quickly find any combination of notes we want.
NOTE: The notePitch[] array is actually just one long line of text. But this is awkward to view on the screen. To make it easier to read, that long line of text has been formatted into a series of shorter lines that contain the frequencies of the 12 notes in an octave. The first line goes from note “0” to note “11,” the second line from 12 to 23, third is 24 to 35 etc. The first note of every line is the C note, and that slot number is divisible 12 with no remainder.
Because musical octaves have twelve notes, we can now change octaves just by moving twelve slots within the array. Because chords have a specific mathematical relationship to the root note, we can now construct a “major” chord by also asking for the notes that are four and seven spots away from the root note.
The rest of the project is simply collecting information about which note(s) we want to play, how long the notes should last, and which order the notes should be played in. The project uses some of the Circuit Playground’s many sensors and inputs to tell the software how to do this.
Requesting a Specific Note
We use the Circuit Playground’s built-in capacitive touch-pads to create the 12-note keyboard. Simply touch one or more of the pads to play any of the 12 notes in a single octave. Seven of the pads are used to represent the seven notes C, D, E, F, G, A and B. The sharp/flat notes are represented by simultaneously touching the surrounding notes – so you get C# by touching C and D at the same time.
When we touch a pad the code sends a numeric value to a variable named “noteCurrent” and calls a function named “makeChord.” The number sent to the noteCurrent variable represents the note’s distance away from the C note of the current octave. The makeChord function coordinates the note and octave values to determine which array item to use. The function uses that numeric value and other data to find the correct item in the notePitch[] array. That array item (slot) contains the correct frequency for the requested note, which is really what we're after.
Changing Octave and Tempo
The built-in 3-axis accelerometer is used to control both the octave and tempo.
You can cause the board to play in different octaves by physically tilting the board from right to left. The values read from the sensor are translated (mapped) into numbers that correspond to the available octaves. Although the sensor can return values that range from -10 to +10, we map that range to five (5) possible choices to match the five (5) available octaves.
The makeChord function uses this number to move up or down the notePitch array in groups of 12 items at a time. So if the sensor reading gets mapped to a value of 3, the function multiplies 3 by 12 to get 36. The 36th item in the notePitch array is the C note of the third available octave.
NOTE: We only use some of the available octaves contained in the notePitch[] array. The really low and really high octaves are not accessed in this project because “they don’t sound good.” This project only uses the octaves that sound musically pleasing with the tiny speaker on this board.
You can also change how long the note plays by physically tilting the board forwards and backwards. The process is similar to that used for changing octaves, but the sensor reading is mapped to a range of time and stored in a variable called “noteDuration.”
Notes, Chords and Arpeggios
We now know which note to play, which octave it’s in and how long the note should play. With this information we can request that Tone play that single note - or we can build a chord around that note and create an arpeggio from that chord – all by counting up a few slots in the array of frequencies.
Normally, only a single note plays. But by using different combinations of the built-in buttons and slide switch, you can make the board play arpeggiated triads of major, minor or blues chords, change from ascending to descending arpeggios, or create a musical “riff.”
Finally, We Actually Play the Notes
At last, we have everything we need to begin playing the notes. Control now goes back into the main loop and the code actually executes the Tone command to play the notes.
What About the LEDs
The 10 LEDs are used to indicate which octave is in use and the duration of the notes. Since we use two tilting-axes to control these, we mimic the axes with the LEDs. The LEDs are arranged in two rows of five, one row on each side of the board. We start by lighting one LED in each row.
We take the accelerometer data, the same info used for octave and tempo selection, and use it to configure the LED display.
If the board if perfectly horizontal on both the X and Y axes then the middle LED in both rows is set to the color blue. We change the LEDs' colors to show octaves and we change the location (which pair of LEDs is lit) to show the tempo.
The octave changes when the board is tilted left or right. When the board is tilted we change the color of the LED that is physically higher. When the octave moves one position the higher LED turns green, when the octave moves two positions the LED turns red. The opposite, lower LED remains blue.
When the tempo (noteDuration) changes we "move" the pair of lit LEDs up or down the row. The colors act the same, but we light the LEDs that are closer to the physically lower end of the row.
Expansion and Going Farther
You still have a light sensor, a temperature sensor and an unused axis on the accelerometer that are not being used to gather input. How could you use these inputs to control the notes that get played?
You could change the code to make the accelerometer select the notes and use the touch-pads to select different chords – diminished, augmented, inverted and extended with VIIs or doubled.
Or instead of tempo, use the other axis to “slide” or “bend” the note.
Or maybe blink the LEDs to match the tempo.
Just have fun and explore.
Step 3: How to Get the Basic Notes
The Tone Command
Like most basic Arduino boards the Circuit Playground does not have “analog out” capability. So you cannot output super high-quality music with the tone command. But you can approximate basic audio frequencies with digital pulses controlled with the tone function. The Arduino.cc site says that tone, “Generates a square wave of the specified frequency (and 50% duty cycle) on a pin.”
NOTE: Although the simple chips on basic Arduinos cannot output true audiophile quality sounds there are other, more advanced, libraries that can create stunning sounds given the limited computing power of the chips. A current favorite of mine is MOZZI, a library capable of turning simple Arduinos into a capable DSP unit. And there are many others, plus lots of Instructables to show you how.
To play a note we use a command like, “CircuitPlayground.playTone(frequency, duration).” Notice that the form is slightly different than the raw Arduino command and you do not need to specify a pin number. Like the basic tone command, the “duration” parameter is optional.
In this project we pass the “frequency” and “duration” to the tone command in the form of variables. The compiler knows to use the contents of the variables to fill the parameter. For example, we pass the variable “chord_III” for the frequency and the variable “noteDuration” to the duration parameter in a command like:.
CircuitPlayground.playTone(chord_III, noteDuration);
Other sections of the tutorial explain how "chord_III" and "noteDuration" get the correct data. Originally, I was going to keep all the functionally as pure, clean mathematical relationships. But I noticed that beginning coders could find their way around the code easier when there were familiar musical terms ("breadcrumbs" if you will) left in the code. So the code is slightly less efficient, but there are enough landmarks for newbies to find their way around the program.
The Touch Pads
All eight of the Circuit Playground’s GPIO pins are preconfigured to act as “capacitive touch-pads.” This means that each pad can sense the electricity from a human touch. When nothing is touching the pad, the reading is very low. When a pad is touched the reading becomes much higher.
The code tests to see if the pads are reading higher than a specified minimum – if not the result is false (off), if it is high enough then the result is true (on).
We use this configuration like a piano’s keyboard. Each pad corresponds to a musical note, and the sharp/flat notes are triggered by touching two pads at the same time.
You can touch the pads themselves, or you can attach alligator clips and wires, conductive paint, conductive thread, or anything else that conducts electricity back to the pads. You’ve probably seen the “banana piano” project. You can do the same thing with this board.
Other Ways to Use the Pads
This project uses the touch-pads as buttons, or more accurately, as digital on/off inputs. But these pads can be used in a number of interesting ways. Capacitive pads can also be used more like analog inputs that can sense the location of the human body from several inches away (see various Theremin projects and the CapSense library). Also see various “capacitive touch” Instructables to learn more about the concepts and electronics behind capacitive sensing.
These same GPIO pins can also be reconfigured variously as digital input or output pins, true analog input pins, serial communication lines and an I2C port. You can hook up buttons, potentiometers, sensors, motors and displays just like any other Arduino type board.
Basic Commands
Using the Circuit Playground’s touch pads is easy. Adafruit has pre-installed the required hardware and provided an easy command to test the state of each pad. Just use “CircuitPlayground.readCap(X)” where “X” is the pin number of that pad.
In this project we test to see if the pad’s reading is greater than a specified threshold. We set that minimum threshold by specifying a value in the variable “touchThreshold.” So our test becomes something like:
“If the value returned from CircuitPlayground.readCap(1) is greater than the value contained in touchThreshold then execute the specified action.”
Implementation
We use the touch-pads as a musical keyboard where each pad corresponds to one of the 12 notes in an octave. When a pad is touched, the code sends a value to the “noteCurrent” variable. But instead of sending the name of the note (like NOTE_C4), we send a numeric value that represents the note’s distance from the C note of the octave. So if the pad for the D note is touched we send the value 2 because D is two tones away from the beginning C note. Other parts of the code then use “noteCurrent” to look up the correct frequency in the notePitch[] array.
Two Pads at Once
The only tricky part of this code is testing to see whether two notes are touched simultaneously. Remember, the sharp/flat notes are triggered by pressing two pads simultaneously to mimic the black keys on a piano keyboard.
We must test for these combination-touches at the beginning of the code, before we test the individual pads. If we don’t test for the combos at the beginning, the code will take the first “true” condition it finds, execute a command and then leave the test procedure without finding that second touch.
If we are touching pads 1 and 2, and we test for isolated touches first like:
- If 1 do X
- Else if 2 do Y
- Else if 3 do Z
- If 1 and 2 do A
- Else if 2 and 3 do B
Then the test for pad 1 will always be true before we can ever test for the combination of 1 and 2 – we will never detect the combination touches.
So we have to make our test do something like:
- If 1 and 2 do A
- Else if 2 and 3 do B
- Else if 1 do X
- Else if 2 do Y
- Else if 3 do Z
Now we catch the 1 and 2 combination. If there was no combination touch, we continue and test for individual touches – all conditions get tested.
The Extra Pad
Note that one capacitive pad is not used in this project. You could use this pad as an extra button, or attach a potentiometer or other device. In another project I used the pin to send serial data to a MIDI sound board. The MIDI sounds are much nicer and you can get multi-voice polyphony – all with nearly the same basic code techniques used for this project
Step 4: Creating Chords and Arpeggios
Buttons and Switches
Select Chord Type
The function "makeChord" creates a collection of variables for "chord_I, chord_III, chord_IV" etc. These variables contain the frequencies for the notes in a specified chord. Since the Arduino tone function cannot play these notes all at once (as a "real" polyphonic chord) we play the notes one at a time in an arpeggio. We can create an arpeggio that uses any chord structure. This project uses major, minor and blues triads. We can also play the arpeggios as a series of rising or falling notes.
With all these options we need a way to select which one we want to execute. We use the Circuit Playground's built-in slide-switch and pair of buttons. These three inputs can be used individually or in combinations. We could use the buttons to step forwards and backwards in a list of actions, but I decided to use them in a nested structure with the slide switch as the first fork of the decision tree.
If the slide-switch is ON we execute one set of actions for each combination of button presses, but if the switch is OFF we execute a different set of actions for button presses.
This gives us eight options to choose from. That's a reasonable number of options, and the code allows us to explore some basic nesting structures.
Other Combinations
This following may seem obvious to some of you, but if you have never created nested or sequential menus then a short primer might explain the possibilities.
Each button and the slide-switch have two possible states: ON or OFF. So used individually we have three pairs of possible on/off states or 2+2+2=6. So we can easily do three separate true/false tests.
Or you can create a single test with more available options. Using just the two buttons we can have four possible combinations for a single test:
- None
- Left
- Right
- Both
You could also use the four-state-button-test and slide-switch separately. That give you two sets of tests - a four-state and a two-state test.
If we also use the slide switch and its two possible states, we can double the possibilities to eight usable states. This is done by nesting the button tests inside a test of the slide switch. If the slide switch is set to ON the four possible button states control four actions. However, if the slide switch is set to OFF those same buttons can now trigger four different actions for a total of eight conditions.
Switch is ON
- 1. None
- 2. Left
- 3. Right
- 4. Both
Switch is OFF
- 5. None
- 6. Left
- 7. Right
- 8. Both
These are the basic combinations for tasks that require "instant feedback." However, if you can wait for a moment between testing and execution, you can even create complex menu systems. If you test for the sequence, click-and-hold or double-clicks you can set the modality of all the buttons.
As an example:
- If the left button is not held for more than three seconds and not double-clicked, execute the basic set of actions for all buttons
- else if the left button is held for more than three seconds, execute a second set of actions for all buttons
- else if the left button is double-clicked, execute a third set of actions for all buttons
- (repeat for the right button for action-sets four and five
Sloppiness and Debounce
This project does not need super accurate timing for the button presses, so we can get away with the simplest tests. But eventually you will need to learn about "debouncing" your button presses. Debouncing is required when a project can execute several commands during the time a button is pressed, or the "noise" (quick and random on/offs as the button begins to make contact) causes erratic behaviors.
The Accelerometer
The triple-axis accelerometer is the only “sensor” we use for this project. We use it to accomplish the same task as a pair of potentiometers - only way more fun. Instead of twiddling knobs we change behaviours by tilting, twisting and turning the entire Circuit Playground board in 3D space.
The accelerometer sensor is very easy to use, but difficult to explain. The easiest way to quickly understand how it works is to load the “demo.ino” example sketch from the Circuit Playground’s library. Open the serial monitor window in the Arduino IDE. Gently tilt the board and watch the numbers change. Now shake the board and watch the numbers spike even higher.
In this project we use the accelerometer much like a pair of potentiometers. But instead of twisting two knobs to set values, we tilt and rotate the board in three-dimensional space to control values.
The sensor returns a range of values between -10 and +10. We don't need 20 possible values or negative numbers, so we translate the value into a useful range with the "map" command.
We send one of the mapped values (from the Y axis) to a variable called "currentOctave" and the other (from the X axis) to variable called "noteDuration." These values are used elsewhere to set the audio frequency of a musical note and to specify how long the note lasts.
How the Sensor Works
This type of sensor is essentially a weight attached to the end of a spring. The other end of the spring is attached to the board. When the board is moved the weight tends to remain stationary for a moment due to inertia. If the board moves towards the weight the spring compresses. When the board moves away from the weight the spring stretches. The spring eventually returns to its "neutral" length once the board stops changing velocity.
A constant electric current is always being sent through the spring. The amount of the current that flows through the spring and returns to the sensor is measured. The spring's resistance changes as it stretches or compresses. For example, the resistance may decrease in compression and increase during stretching. This change in resistance changes the amount of electricity returning to the sensor.
With some math we can tell whether the spring is being compressed or stretched and by how much, then translate that into a G-force reading. Luckily, all the complex math is handled by the Circuit Playground's supporting library. All we have to do is ask for a reading of an axes and we automagically get a nicely formatted reading for the G-force acting along that axis.
For more info on how accelerometers work read Sparkfun's introduction to Accelerometers.
For details on using and configuring all the capabilities of this specific sensor visit Adafruit's introductory tutorial for the Circuit Playground and the in depth tutorial for the LIS3DH. (Remember, make sure your version of the Circuit Playground board is still using this sensor).
Axis and Orientation
Although we use it as more of a tilt-sensor in this project, it’s called a triple-axis (or 3-axis) accelerometer because it senses acceleration when moved (1) forwards and backwards, (2) from left to right and (3) up or down. It can sense movements on all three axes simultaneously. The accelerometer actually has three such sensors in a single package, one sensor for each of the X, Y and Z axes.
The axes are labeled X, Y and Z on the Circuit Playground board and the software library uses these same names when you ask for a sensor reading with a request like, “CircuitPlayground.motionZ().” Notice that the accelerometer measures forces ALONG an axis, not around an axis like a gyroscope.
Gravity also exerts a constant acceleration downwards and the accelerometer reports this force. (Think of the origin of the term "G-force") We can tell when the board is tilted towards the ground, and we can tell which direction and how much the board is tilted. We make use of this to create a three-dimensional control knob.
While very useful, an accelerometer provides only a fraction of the data we can get about an object's orientation and movement through space. For more details we need a gyroscope, or a magnetometer (or both) and maybe even an altimeter. For more on how these sensors contribute and interact read Sparkfun's excellent introduction to IMUs and Adafruit's fun example on how to use the data.
You may notice that you cannot get the maximum values from both axes at the same time with this code. It is possible to constrain and condition the sensor readings and the translation so that both the X and Y axis can yield their maximums simultaneously. But this makes the code a little more confusing and was not part of the emphasis for this project. If you don't know how, or don't even know what this means, watch Adafruit's Tony D's tutorial on using the accelerometer as a mouse for some ideas and techniques.
Step 5: Using the RGB LEDs
NeoPixel Basics
“NeoPixel” is the brand-name Adafruit uses for some of their addressable RGB and RGBW LEDs. There is a wide range of NeoPixel products that include flexible strips, rings, panels and individual LEDs. The Circuit Playground has ten NeoPixels arranged in two arcs of five.
The term RGB means that you can control the amounts of (R) red, (G) green and (B) blue light emitted from the LED. Some also have a (W) white LED added. They are called "addressable" because each of the LEDs has a unique address. You can send commands to any address and have that command only affect that address.
So it's easy to write commands to:
- set pixel #24 to red,
- set pixel # 37 to green
- and #49 to blue - (or purple, or orange or pink.)
NeoPixels have always been easy to use thanks to the excellent library provided by Adafruit. But the Circuit Playground makes it even simpler. Adafruit has pre-configured the LEDs, hardware and software. The type, pin number and quantity have all been preset. Now, all you have to do is make a simple call to set your colors on specific LEDs. To set pixel #3 to value 170 (blue) use the following code:
- CircuitPlayground.setPixelColor(3, CircuitPlayground.colorWheel(170));
This project uses the "colorWheel" command to set the colors, but you can also use the traditional NeoPixel methods to specify individual red, green and blue values. A quick primer is available at Adafruit.
If you are familiar with NeoPixels you will notice other differences with this board. The LEDs are running on 3.3v instead of 5v, so they will never be as bright as they are on a full-power 5v board. The global brightness was pre-set to about 30 percent. (You can set them back to 100 percent brightness in the code.)
Visit THE NEOPIXEL UBER GUIDE to find out everything yo need to know about using NeoPixels.
Or browse Adafruit's huge collection of other projects that use NeoPixels.
Implementation
We use readings from two accelerometer axes to control two variables - octave and tempo. So we need to simultaneously display two separate values with the LEDs. There are several possible ways to accomplish this, but this project uses the LEDs' colors to indicate the octave and the location of the lighted LEDs to indicate tempo.
First, we need to create pairs of matching LEDs - one on each side of the board. Since we only have ten LEDs I hardcoded the pairs using their "pixel address" to create five pairs.
L--R
0---9
1---8
2---7
3---6
4---5
When the board is flat we light up the 2--7 pair, but when the board is tilted we light up the next pair (1--8 or 3--6). If the board is tilted even more, we light up the next pair.
Next, we have to decide what color to make the LEDs. We use another axis of the accelerator to determine the colors. If the board is flat, in the active pair are set to a blue color. If the board is tilted so the left side is slightly lower, we turn the right active LED to green. If the board is tilted more the LED is set to a red color.
If the board is tilted on both axes simultaneously we move the "active" pair of LEDs but keep using the same colors. So we can now display two types of data simultaneously.
NOTE: I thought this was a very clever solution, but I now thinks that kids really like to se the LEDs flash in time to the music and change color based on the note. And so do adults. Plus no one ever looks at the display for feedback because you can also hear the affects. It's still a fun coding exercise, but changing the LEDs to make your own custom light show could be an even better one.
Step 6: The Case and Keyboard
You do NOT need a case or auxiliary keyboard for this project to work -- but it sure can be fun to experiment. All you need for this project is the Circuit Playground board. Just hold the board in your hand and touch the pads to create music.
Those big, gold touch-pads make the Circuit Playground easy to use. And the pads are conductive on both sides of the board. Unfortunately, that makes it easy to accidentally play a note we don't really want to play. Luckily, there is a simple fix for that.
Simple Touch Guard
Just adhere the Circuit Playground to a non-conductive disk - whatever you have laying around. Cut a circle out of paper, cardboard, wood etc. Make the disk slightly bigger that the Circuit Playground board. Hot-glue or tape the Circuit Playground onto the disk making sure only the tops of the touch-pads are easy to reach.
Or just use an appropriately sized plastic lid, candy container or wooden spool - almost anything that helps keep unwanted fingers away from the touch-pads.
I find it easier to hold and play when it's taped to the top a 3xAAA battery box w/switch. It even makes it portable.
Alligator-Clip Keyboard and More
A lot of the classic tone tutorials use alligator clips to expand the size and reach of the keyboard. They often attach the other end of the alligator clip to some conductive surface. Remember, anything that conducts electricity will trigger the pads just like a direct touch does. Conductive paint works, so does aluminum foil or even fruits and vegetables. You can even sew the project into your shirt.
Get Creative - Add Some Googly Eyes
If you enjoy this type of activity you can get very creative with the cases and keyboards. Since kids love to make noise and funny faces, I decided to try to create an entertaining crafts project. Hopefully, the goofy face plus the technology will get the whole maker-family together on one project.
Make yours look however you want, but I used:
- a plastic lid from a jar of peanut butter
- conductive copper tape
- wire
- some paper
- paint or markers optional
- a pair of googly eyes
- a tiny puffball pompom
- hot glue
Making the face is easy. Cut a disk of paper to the same size as the plastic lid. Place seven pieces of the conductive copper-tape where the "mouth" will be. Trace around the circuit playground board and the touch-pads used to trigger notes. Place seven more pieces of tape where the touch pads will be.
Cut and strip the ends of seven pieces of wire. Now use a second layer of copper-tape and tape one end of the wire to the "mouth" keys and the other end of the wire to the tape for the corresponding capacitive touch pads.
Next, place the Circuit Playground board on the lid and add a final layer of copper tape to connect the capacitive pads with the copper tape. A dab of hot glue will help keep the connections strong and the board in place. Test to make sure the connections work and that you can plug in the USB cable. Adjust as needed.
Color the paper shapes and cut them out. Glue the "mouth" shape to the lid, then glue the "face" shape to the lid. The face-shape should cover the capacitive touch pads, but keep the LEDs exposed.
Glue on the googly eyes and fuzzy nose.
Plug it in and play the toothy piano.
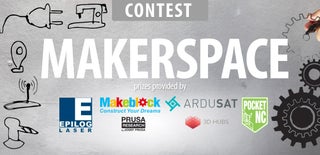
Participated in the
Makerspace Contest