Introduction: My Old Phone + Arduino = Phonoduino
Hi, this is a project of my old Nokia 1100 phone and arduino mega. By using this many arduino and gsm based project is possible. The phone will work like a gsm module and you can sent message or call someone by using this phonoduino. You can also receive DTMF signal and control anything remotely. It can be used for home automation, home security and monitoring, bird feeder or anything you like. You can also type sms by computer keyboard and send instantly to someone or group by using arduino serial monitor. If you used multimedia phone instead of nokia 1100 you can sent image to another mobile or e-mail by this project. Following are the step by step guide for making the thing.
Phonoduino can be a best DIY for remote monitoring, security and control.
Step 1: Required Components
1. Old but working phone (I am using nokia 1100 but other phone will also work without touch phone)
2. Arduino Mega (UNO has less number of pin and can be used if DTMF is not required)
3. General purpose NPN transistor (2N2222 or BC547 x 16)
3. DTMF decoder IC (M-8870)
4. Resistors (5.6kΩ x 16, 100kΩ; 70kΩ; 390kΩ)
5. Capacitors (0.1µFx 2)
6. Crystal oscillator (3.579545MHz)
7. Vero-board and some wire
Required Tools
1. Screw Driver
2. Soldering Iron
3. Wire Cutter
4. Knife
5. Multimeter
Step 2: Preparing the Phone
Open the casing of the phone. Remove the screw using screw driver. Then remove the plastic insulation above the keypad of the phone. Now solder thin insulated wire (I used connecting wire from old Hard disk IDE cable) to the signal pad of every button. Inner dot pad is signal pad and outer circular pad is ground pad. When soldering be careful DON'T SHORT GROUND PAD AND SIGNAL PAD WITH SOLDER. Now solder one wire with ground pad.
After soldering all button add the display with frame. Out the wire from the hole of display frame and adjust it to the motherboard with screw. Top metal of the display frame is connected to ground. Now connect the wire of the power button to the ground wire or just connect to the metal frame and hold for some moment. If every thing is correct the phone will be ON. Successfully ON your phone? Congratulation! You have completed first step.
Step 3: Connecting With Arduino Mega
Above schematic shows how arduino digital pins (D3 to D18) are connected to phone keys. I used general purpose bipolar npn transistor (2N2222) for switching (on-off the phone key). Any other general purpose npn transistor such as BC547, 548 may be used. Arduino digital I/O pin is connected to the base of transistor through a current limiting resistor. A 12k resistor works fine. When a digital pin of arduino become high the BJT turn on and work as like a press on the key of mobile phone. If you are interested to know more about transistor follow the link: https://learn.sparkfun.com/tutorials/transistors. You can find the datasheet of 2N2222 here. Pin configuration of 2N2222 is given above.
When connecting different circuit together for successful operation one thing must be remember, there must be one common ground connection among them whatever the other connection is. I connect phone ground and arduino ground together with the emitter of transistors. Image of transistors board is uploaded herewith. For connecting arduino I/O port I added male pin header to the board. Pin headers are connected with base resistors through yellow jumper wire.
After making the board now carefully connect phone's keypad wire with the collectors of the transistors. I didn't add any resistor between transistor collector and keypad wire because according to phone schematic a resistor is internally connected to the signal pin of keypad.
Attachments
Step 4: DTMF Decoder for Remote Control and Home Automation
Required Component
1. MT8870D or CM8870 or HT9170 IC
2. Capacitor (0.1uF x 2, 15-20pF x 2)
3. Resistor (300K x 1, 100K x 2)
4. Crystal (3.579545MHz x 1)
5. Bread board
The circuit diagram of DTMF decoder/receiver is given above. Crystal is connected to pin 7 and 8. Two 20pf capacitor is connected between crystal and ground (it is not mandatory, you may not use pf capacitor or you may use any in range of 15pf - 30pf). For the resistor 100K you may use around 150K and for 300K around 400K may be used. Pin 5 & 6 should be connected to ground and pin 10 should be connected to vcc (this is not shown in schematic). For details see the datasheet of MT8870.
Image of my complete DTMF module is uploaded above. Pin configuration of Nokia headphone jack is given herewith. speaker +ive is connected to the pin 2 of the IC through 104pf (104pf = 0.1uf) capacitor and 100k series resistor. Speaker -ive is connected to ground. mic +ive and -ive will remain open. Head wires are insulated by different colored insulation. For soldering these wires to the pcb you have to remove the insulation. You can use sand paper or use match fire to burn the insulation. Put the terminal of the wire into fire for 2-3 seconds insulation will burn. Now you can solder the wire to pcb.
If you don't like to take burden of making this DTMF module you can use Seeedstudio DTMF shield, a very cool one.
Want to know more about DTMF? click here
Step 5: Programming Arduino
Image of my complete phonoduino (phone + arduino) setup is given above. DTMF input is not given to the arduino now. We will see this later of this project. Let us now write some code to give the live of the phonoduino. Code for automatic calling for any given number is attached.
int key0 = 16;
int key1 = 6;
int key2 = 12;
int key3 = 9;
int key4 = 5;
int kry5 = 15;
int key6 = 10;
int key7 = 14;
int key8 = 13;
int key9 = 18;
int keyStar = 17;
int keyOk = 11;
int keyClear = 3;
int keyDown = 4;
int keyUp = 8;
int keyOff = 2;
int keyHash = 19;
int pressTime = 100;
int pressDelay = 300;
int holdTime = 1000;
int value[16] = {16,6,12,9,5,15,10,14,13,18,17,11,3,4,8,2};
void setup() {
// initialize the digital pin as an output.
for(int i=2; i<20; i++)
pinMode(i, OUTPUT);
holdClear();
unlockPhone();
delay(2000);
holdClear();
delay(1000);
// call 0123456789
pressDigit(0);
pressDigit(1);
pressDigit(2);
pressDigit(3);
pressDigit(4);
pressDigit(5);
pressDigit(6);
pressDigit(7);
pressDigit(8);
pressDigit(9);
delay(100);
pressOk();
delay(5000);
}
// the loop routine runs over and over again forever:
void loop() {
}
void pressDigit(int digit){
digitalWrite(value[digit], HIGH); // press digit from 0 to 9
delay(pressTime);
digitalWrite(value[digit],LOW);
delay(pressDelay);
}
void pressOk(){
digitalWrite(keyOk, HIGH); // press ok
delay(pressTime);
digitalWrite(keyOk,LOW);
delay(pressDelay);
}
void pressClear(){
digitalWrite(keyClear, HIGH); // press clear
delay(pressTime);
digitalWrite(keyClear,LOW);
delay(pressDelay);
}
void pressStar(){
digitalWrite(keyStar, HIGH); // press star
delay(pressTime);
digitalWrite(keyStar,LOW);
delay(pressDelay);
}
void pressUp(){
digitalWrite(keyUp, HIGH); // press up
delay(pressTime);
digitalWrite(keyUp,LOW);
delay(pressDelay);
}
void pressDown(){
digitalWrite(keyDown, HIGH); // press down
delay(pressTime);
digitalWrite(keyDown,LOW);
delay(pressDelay);
}
void pressHash(){
digitalWrite(keyHash, HIGH); // press hash
delay(pressTime);
digitalWrite(keyHash,LOW);
delay(pressDelay);
}
void pressOff(){
digitalWrite(keyOff, HIGH); // press off
delay(pressTime);
digitalWrite(keyOff,LOW);
delay(pressDelay);
}
void holdOff(){
digitalWrite(keyOff, HIGH); // hold off
delay(holdTime);
digitalWrite(keyOff,LOW);
delay(pressDelay);
}
void holdClear(){
digitalWrite(keyClear, HIGH); // press clear
delay(holdTime);
digitalWrite(keyClear,LOW);
delay(pressDelay);
}
void unlockPhone(){
pressOk();
pressStar();
}
Want to call any number from serial monitor?
Code for calling from serial monitor is also attached. Just type phone number into terminal terminating with a coma (,) and press enter. Phonoduino will instantly dial the number. Coma indicates you just complete typing number and want to call. If you want to clear any number wrongly typed just type semicolon (;) and press enter. Then retype your number.
Want to send message from serial monitor?
Typing message from mobile phone is boring and time consuming. It will be easy to type sms from computer keyboard and will save lots of time. A sample arduino sketch is given to sent sms from serial monitor. Just upload the program to your arduino, open serial monitor, type your message, type colon(:), type number, type mobile number or any number you want to sent your sms and press enter key. Your phonoduino will sent the message to your desired number.
Example: Hello, I am from phonoduino.:123456789*
The above command will sent "Hello, i am from phonoduino." to the number "123456789"
<code> int pressTime = 100; int pressDelay = 300; int holdTime = 1000; int typeTime = 100; int typeDelay = 400; int value[16] = {16,6,12,9,5,15,10,14,13,18,17,11,3,4,8,2}; char number[12]; char massegeChar[160]; int count = 0; int numCount = 0; int charDelay = 300; int numberState = 0; void setup() { // initialize the digital pin as an output. for(int i=2; i<20; i++) pinMode(i, OUTPUT); Serial.begin(9600); holdClear(); } // the loop routine runs over and over again forever: void loop() { while (Serial.available()>0){ char character = Serial.read(); if(character == '*'){ sentSMS(); count = 0; numCount = 0; numberState = 0; } if(character == ':'){ numberState = 1; numCount = 0; //delay(10); } if(numberState == 1){ number[numCount] = character; numCount++; } if(numberState == 0){ massegeChar[count] = character; count++; } if(character == '#'){ count = 0; numberState = 0; } } } void pressDigit(int digit){ digitalWrite(value[digit], HIGH); // press digit from 0 to 9 delay(pressTime); digitalWrite(value[digit],LOW); delay(pressDelay); } void pressOk(){ digitalWrite(keyOk, HIGH); // press ok delay(pressTime); digitalWrite(keyOk,LOW); delay(pressDelay); } void pressClear(){ digitalWrite(keyClear, HIGH); // press clear delay(pressTime); digitalWrite(keyClear,LOW); delay(pressDelay); } void pressStar(){ digitalWrite(keyStar, HIGH); // press star delay(pressTime); digitalWrite(keyStar,LOW); delay(pressDelay); } void pressUp(){ digitalWrite(keyUp, HIGH); // press up delay(pressTime); digitalWrite(keyUp,LOW); delay(pressDelay); } void pressDown(){ digitalWrite(keyDown, HIGH); // press down delay(pressTime); digitalWrite(keyDown,LOW); delay(pressDelay); } void pressHash(){ digitalWrite(keyHash, HIGH); // press hash delay(pressTime); digitalWrite(keyHash,LOW); delay(pressDelay); } void pressOff(){ digitalWrite(keyOff, HIGH); // press off delay(pressTime); digitalWrite(keyOff,LOW); delay(pressDelay); } void holdOff(){ digitalWrite(keyOff, HIGH); // hold off delay(holdTime); digitalWrite(keyOff,LOW); delay(pressDelay); } void holdClear(){ digitalWrite(keyClear, HIGH); // press clear delay(holdTime); digitalWrite(keyClear,LOW); delay(pressDelay); } void typeA(){ digitalWrite(key2, HIGH); delay(typeTime); digitalWrite(key2, LOW); delay(typeDelay); } void typeB(){ typeA(); typeA(); } void typeC(){ typeA(); typeA(); typeA(); } void typeD(){ digitalWrite(key3, HIGH); delay(typeTime); digitalWrite(key3, LOW); delay(typeDelay); } void typeE(){ typeD(); typeD(); } void typeF(){ typeD(); typeD(); typeD(); } void typeG(){ digitalWrite(key4, HIGH); delay(typeTime); digitalWrite(key4, LOW); delay(typeDelay); } void typeH(){ typeG(); typeG(); } void typeI(){ typeG(); typeG(); typeG(); } void typeJ(){ digitalWrite(key5, HIGH); delay(typeTime); digitalWrite(key5, LOW); delay(typeDelay); } void typeK(){ typeJ(); typeJ(); } void typeL(){ typeJ(); typeJ(); typeJ(); } void typeM(){ digitalWrite(key6, HIGH); delay(typeTime); digitalWrite(key6, LOW); delay(typeDelay); } void typeN(){ typeM(); typeM(); } void typeO(){ typeM(); typeM(); typeM(); } void typeP(){ digitalWrite(key7, HIGH); delay(typeTime); digitalWrite(key7, LOW); delay(typeDelay); } void typeQ(){ typeP(); typeP(); } void typeR(){ typeP(); typeP(); typeP(); } void typeS(){ typeP(); typeP(); typeP(); typeP(); } void typeT(){ digitalWrite(key8, HIGH); delay(typeTime); digitalWrite(key8, LOW); delay(typeDelay); } void typeU(){ typeT(); typeT(); typeT(); } void typeV(){ typeT(); typeT(); typeT(); } void typeW(){ digitalWrite(key9, HIGH); delay(typeTime); digitalWrite(key9, LOW); delay(typeDelay); } void typeX(){ typeW(); typeW(); } void typeY(){ typeW(); typeW(); typeW(); } void typeZ(){ typeW(); typeW(); typeW(); typeW(); } void space(){ digitalWrite(key0, HIGH); delay(typeTime); digitalWrite(key0, LOW); delay(typeDelay); } void sentSMS(){ holdClear(); holdClear(); pressOk(); pressOk(); pressOk(); for(int i = 0; i< 160; i++){ switch(massegeChar[i]){ case 'A': case 'a': typeA(); delay(charDelay); break; case 'B': case 'b': typeB(); delay(charDelay); break; case 'C': case 'c': typeC(); delay(charDelay); break; case 'D': case 'd': typeD(); delay(charDelay); break; case 'E': case 'e': typeE(); delay(charDelay); break; case 'F': case 'f': typeF(); delay(charDelay); break; case 'G': case 'g': typeG(); delay(charDelay); break; case 'H': case 'h': typeH(); delay(charDelay); break; case 'I': case 'i': typeI(); delay(charDelay); break; case 'J': case 'j': typeJ(); delay(charDelay); break; case 'K': case 'k': typeK(); delay(charDelay); break; case 'L': case 'l': typeL(); delay(charDelay); break; case 'M': case 'm': typeM(); delay(charDelay); break; case 'N': case 'n': typeN(); delay(charDelay); break; case 'O': case 'o': typeO(); delay(charDelay); break; case 'P': case 'p': typeP(); delay(charDelay); break; case 'Q': case 'q': typeQ(); delay(charDelay); break; case 'R': case 'r': typeR(); delay(charDelay); break; case 'S': case 's': typeS(); delay(charDelay); break; case 'T': case 't': typeT(); delay(charDelay); break; case 'U': case 'u': typeU(); delay(charDelay); break; case 'V': case 'v': typeV(); delay(charDelay); break; case 'W': case 'w': typeW(); delay(charDelay); break; case 'X': case 'x': typeX(); delay(charDelay); break; case 'Y': case 'y': typeY(); delay(charDelay); break; case 'Z': case 'z': typeZ(); delay(charDelay); break; case ' ': space(); break; } delay(300); } pressOk(); pressOk(); callNumber(); } void callNumber(){ for(int i=1; i<12; i++){ pressDigit(number[i]-'0'); // number[i] - '0' is for converting character into intiger } pressOk(); holdClear(); holdClear(); }
I will published a more efficient arduino sketch later.
Step 6: Home Security and Automation Using Cell Phone
You can control your home appliances or another thing using this project or you can use it for security purpose. A simple demo will be given here. I have set a ultrasonic sensor to arduino. Our previously made DTMF module is also connected to the phone. When something come in front from ultrasound sensor phonoduino dial the number u previously set. After receiving the call I am controlling an LED (it can be anything you like). Let you set the ultrasound sensor in front of your door. When you are outside and then someone come in front of the door, phonoduino will call you and receiving the call you can open the door for your guest or ring an alarm for the thief.
The sample code is given an a video is uploaded.
Attachments
Step 7: Conclusion
This project is made for Seeed rephone contest. I will published more project using this phonoduino in future. Thank you, and I am apologized for my weak English.
If you have any query or need any help please comment and let me know your problem (or message me) and I'll try my best to help you.
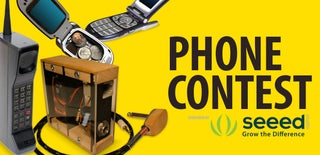
Participated in the
Phone Contest