Introduction: Old Doorbell + Pebble Smartwatch = Smart Old Doorbell
Christmas 2015. A time of receiving a significant number of deliveries at home. But also a time of missing lots of deliveries because being in the bathroom with your earphones on in the mornings is the perfect combination for not hearing the doorbell. The solution? Modify your doorbell from the 1980s to send a notification to your Pebble smartwatch when it is rung!
I never finished this project (got it to a state I was entirely happy with) but I wanted to share my journey and learning.
Step 1: System Operation Overview
The system operates as follows:
- The Arduino Uno is constantly generating a 200ms PWM which is transmitted as an RF signal. While a 200ms RF signal is being recieved by the Arduino Micro, no action is taken by the system.
- The Node-RED software is constantly running on the Raspberry Pi and polling the state of the pin connected to the Arduino Micro
- The doorbell is rung
- This activates the doorbell transformer which generates an AC current to actuate the doorbell chime
- The current sensor detects the AC current and outputs a voltage of approximately 2.5V (this was the average value detected over a number of tests)
- This output voltage is detected by the Arduino Uno
- The Arduino Uno generates a 100ms PWM (while the voltage is detected) which is transmitted as an RF signal
- The Arduino Micro detects the 100ms PWM signal
- The Arduino Micro output pin which is connected to the Raspberry Pi is changed from HIGH (5V) to LO (GND) state
- The Node-RED software detects this pin state change
- An email is sent with a predefined doorbell alert message to a designated email address
- This email is received by the smartphone
- This email notification is sent to the smartwatch which is paired to the smartphone
Step 2: Supplies
Step 3: Build the RF Transmitter
Build the Arduino Uno and RF transmitter circuit on a breadboard, according to the diagram (picture 3).
Cut into the output of the doorbell transformer and secure the inline current sensor onto this wire.
Attach current sensor to the breadboard, according to the diagram.
Flash the Arduino Uno with attached .ino file or equally, copy and paste the code below to flash.
---------------------------------------------------------------
Code
const int TransmitterDataPin = A5; //Connect A5 to data on RF transmitter module
const int DoorbellSensorPin = A4; //Connect A4 to the doorbell current sensor data pin
float DoorbellSensorValue = 0; //Raw value from doorbell current sensor
float DoorbellSensorVolt = 0; //Raw value from doorbell current sensor converted into a voltage
void setup()
{
Serial.begin(9600);
pinMode(DoorbellSensorPin, INPUT); //Reading value of voltage on doorbell wire
pinMode(TransmitterDataPin, OUTPUT); //Writing value going into Tx Data pin to be sent to Rx Data pin
}
void RfPwmTransmit(int p, int t) //Function to create a PWM with amplitude p and off time t for RF transmission
{
analogWrite(TransmitterDataPin, p);
delay(t);
}
void loop()
{
DoorbellSensorValue = analogRead(DoorbellSensorPin); //Read raw value of doorbell current sensor
DoorbellSensorVolt = DoorbellSensorValue * 5 / 1023; //Convert raw value of doorbell current sensor into a voltage
Serial.print(DoorbellSensorVolt);
Serial.print("\n");
if (DoorbellSensorVolt < 2.42 || DoorbellSensorVolt >= 2.55)
{
Serial.print("SHORT ");
RfPwmTransmit(255, 100);
RfPwmTransmit(0,100);
}
else //long burst
{
Serial.print("LONG ");
RfPwmTransmit(150, 200);
RfPwmTransmit(0,200);
}
}
Attachments
Step 4: Build the RF Reciever
Build the Arduino Micro and RF Reciever circuit on a breadboard, according to the diagram (picture 4) - FYI Raspberry Pi Pin 10 is the BCM18 Pin.
The resistors that are used are current limiting resistors, so their value was not hugely important during selection, I just selected resistors which would not hinder the circuit. I believe the Raspberry Pi to collector resistor is a 10k ohm resistor and the emitter to Raspberry Pi resistor is a 120 ohm resistor.
Flash the Arduino Micro with attached .ino file or equally, copy and paste the code below to flash.
---------------------------------------------------------------
Code
const int RecieverDataPin = A5; //Connect A5 to data on RF reciever module
const int RaspiOutputPin = 4; //Connect 4 to a Raspberry Pi GPIO pin
unsigned long PulseTime = 0;
void setup()
{
Serial.begin(9600);
pinMode(RecieverDataPin, INPUT); //Reading PWM on RF reciever Data pin coming from RF transmitter
pinMode(RaspiOutputPin, OUTPUT); //Writing high/low state to Raspberry Pi GPIO
}
void loop()
{
PulseTime = pulseIn(RecieverDataPin, HIGH);
Serial.print(PulseTime);
Serial.print("\n");
if(PulseTime <= 120000)
{
digitalWrite(RaspiOutputPin, HIGH);
}
else
{
digitalWrite(RaspiOutputPin, LOW);
}
}
Attachments
Step 5: Check the RF Communication
To ensure that the RF signals are operating correctly, I set up a dummy doorbell on the Arduino Uno as a simple push switch, and attached an LED across the Data Pin and GND on the RF Receiver module. Doing so shows that the LED flashes at differing speeds depending on if a 100ms or 200ms PWM is being received.
Step 6: Setup Node-RED
Set up a Raspberry Pi GPIO Input Node (Tutorial Link)
- Pin 10
- Digital Input
- Initialise Pin State = Low
Set up a message Node (paste the below in the payload text editor)
msg.payload = "Somebody is at the door";
msg.topic = "Doorbell Alert!";
return msg;
Set up an email Node with your email address (Tutorial Link)
Step 7: Closing Notes
Assumptions:
- You have a smartphone which receives email alerts
- You have a smartwatch which receives notifications from your smartphone
Unresolved Issues:
- False positives (doorbell alerts when the doorbell isn't rung)
- False negatives (doorbell alerts not received when the doorbell is rung)
- Replace the Arduino Uno with a bare bones arduino
- Use a lightweight power solution from the transmitter unit (not a USB powerbank)
- Reduce the time delay for the email notifications (maybe use a Arduino GSM Shield)
- Code in some form of accessible self diagnostics
- Use interrupts rather than polling for the receiver input
The system could have benefited from multiple improvements, but as my steam (and time) was limited I never got around to implementing this stuff. Overall a fun and educational project!
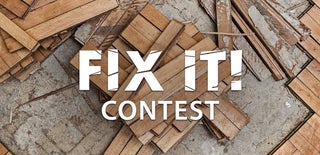
Participated in the
Fix It! Contest