Introduction: Original Light Sensitive Piano
This Arduino project is a new method to create sound. You can use this Instructable to make any instrument you would like to play. Light sensors replace the keys and when a finger approaches the light sensor, the corresponding note is played. The code can be used either to listen to music or for playing music.
Step 1: Parts List
We need these following parts and tools to make this Arduino based instrument:
1. 2 Arduino Uno's
2. Light sensors (photoresistors; as many required for your instrument)
3. 10k ohm resistors
4. Speakers
5. Jumper cables
6. Arduino code
7. Breadboard
Step 2: Schematic
1. One end of the photoresistors goes to ground.
2. The other end is connected to an analog pin number and a resistor connects it to five volts.
3. The speaker is connected to ground and a digital pin.
Step 3: Definitions
First you will want to define all of the notes so that they can then be applied to the song or instrument you are creating. These definitions/variables will assign a note to a frequency. For example NOTE_C4 instead of the number 262. The numbers represent the frequencies and when you code for one of the notes a different frequency will play.
Step 4: Void Setup
The setup is where you will make your songs:
1. Fist you establish an integer called melody and then you assign the notes in the song to the integer.
2. Then you establish an integer called noteDurations which will determine how long each of the notes is played for.
3. pinMode tells the arduino which pins you will be using and if they are recording information or sending out information. The speaker will be an output and then the photoresists will be inputs.
4. tone(8, melody2[thisNote], noteDuration2); -Tells the arduino which pin to use(in this case eight) and then which melody and noteDurations to play.
Code:
int melody[] = {
NOTE_C4, NOTE_G3, NOTE_G3, NOTE_A3, NOTE_G3, 0, NOTE_B3, NOTE_C4 };
int melody2[] = { NOTE_A3, NOTE_C4, NOTE_D4, NOTE_D4, NOTE_D4,NOTE_E4, NOTE_F4, NOTE_F4, NOTE_F4, NOTE_G4,NOTE_E4,NOTE_E4, NOTE_D4, NOTE_C4, NOTE_C4, NOTE_D4, NOTE_A3, NOTE_C4, NOTE_D4, NOTE_D4, NOTE_D4,NOTE_E4, NOTE_F4, NOTE_F4, NOTE_F4, NOTE_G4,NOTE_E4,NOTE_E4, NOTE_D4, NOTE_C4, NOTE_D4, NOTE_A3, NOTE_C4, NOTE_D4, NOTE_D4,NOTE_D4, NOTE_F4, NOTE_G4, NOTE_G4, NOTE_G4, NOTE_A4, NOTE_AS4, NOTE_AS4, NOTE_A4, NOTE_G4, NOTE_A4, NOTE_D4, NOTE_D4, NOTE_E4, NOTE_F4, NOTE_F4, NOTE_G4, NOTE_A4, NOTE_D4, NOTE_D4, NOTE_F4, NOTE_E4, NOTE_E4, NOTE_F4, NOTE_E4, NOTE_D4 };
// note durations: 4 = quarter note, 8 = eighth note, etc.: int noteDurations[] = { 4, 8, 8, 4, 4, 4, 4, 4 }; int noteDurations2[] = { 8,8,4,4,8,8,4,4,8,8,4,4,8,8,8,3,8,8,4,4,8,8,4,4,8,8,4,4,8,8,2,8,8,4,4,8,8,4,4,8,8,4,4,8,8,8,3,8,8,4,4,4,8,3,8,8,4,4,8,8,2 };
void setup() { // put your setup code here, to run once: pinMode(A1, INPUT);
pinMode(A2, INPUT);
pinMode(A3, INPUT);
pinMode(A4, INPUT);
pinMode(A5, INPUT);
pinMode(A0, INPUT);
pinMode(A6, INPUT);
pinMode(8, OUTPUT);
Serial.begin(9600);
// to calculate the note duration, take one second divided by the note type.
//e.g. quarter note = 1000 / 4, eighth note = 1000/8, etc. int noteDuration2 = 1000 / noteDurations2[thisNote]; tone(8, melody2[thisNote], noteDuration2);
// to distinguish the notes, set a minimum time between them. // the note's duration + 30% seems to work well: int pauseBetweenNotes2 = noteDuration2 * 1.30; delay(pauseBetweenNotes2);
// stop the tone playing: noTone(8); }
}
Step 5: Void Loop
Void loop is where you will be playing your music:
1. First you assign an ldr number to each photoresistor.
2. Then you create your if statements. If a photoresistor reads that the numbers pass a certain point then that means a finger approached the photoresistor and then you write the function, "tone," for whichever note you want that specific photoresistor to play.
Ex: if (ldr1 > 650) {tone(8, NOTE_A3); }
void loop() {
// put your main code here, to run repeatedly:
int ldr1 = analogRead(A1);
int ldr2 = analogRead(A2);
int ldr3 = analogRead(A3);
int ldr4 = analogRead(A4);
int ldr5 = analogRead(A5);
int ldr0 = analogRead(A0);
int ldr6 = analogRead(A6);
Serial.println(ldr4);
if (ldr1 > 650) {tone(8, NOTE_A3); delay(500);} else noTone(8);
if (ldr2 > 450) {tone(8, NOTE_C4); delay(500);} else noTone(8);
if (ldr3 > 350) {tone(8, NOTE_D4); delay(500);} else noTone(8);
if (ldr4 > 570) {tone(8, NOTE_E4); delay(500);} else noTone(8);
if (ldr5 > 450) {tone(8, NOTE_F4); delay(500);} else noTone(8);
if (ldr0 > 900) {tone(8, NOTE_G4); delay(500);} else noTone(8);
if (ldr6 > 1500) {tone(8, NOTE_A4); delay(500);} else noTone(8); }
Step 6: Make a Playlist
If you would like to create an arduino iPod then this step is for you:
My songs are- Marry Had a Little Lamb, Star Wars, Fur Elíse C major, Hallelujah, Pirates of the Caribbean, Beethoven Ode to Joy
1. First insert the notes for your song:
int melody7[] = {
NOTE_E4,NOTE_E4,NOTE_F4,NOTE_G4,NOTE_G4,NOTE_F4,NOTE_E4,NOTE_D4, NOTE_C4,NOTE_C4,NOTE_D4,NOTE_E4,NOTE_E4,NOTE_D4,NOTE_D4, NOTE_E4,NOTE_E4,NOTE_F4,NOTE_G4,NOTE_G4,NOTE_F4,NOTE_E4,NOTE_D4, NOTE_C4,NOTE_C4,NOTE_D4,NOTE_E4,NOTE_D4,NOTE_C4,NOTE_C4, NOTE_D4,NOTE_E4,NOTE_C4,NOTE_D4,NOTE_E4,NOTE_D4,NOTE_E4,NOTE_C4, NOTE_D4,NOTE_E4,NOTE_F4,NOTE_E4,NOTE_D4,NOTE_C4,NOTE_D4,NOTE_G4, NOTE_E4,NOTE_E4,NOTE_F4,NOTE_G4,NOTE_G4,NOTE_F4,NOTE_E4,NOTE_D4, NOTE_C4,NOTE_C4,NOTE_D4,NOTE_E4,NOTE_D4,NOTE_C4,NOTE_C4, };
2. Then insert the durations for each note (4 represents a quarter note, 8 represents an eighth note, etc.):
int noteDurations7[] = {
4,4,4,4,4,4,4,4,4,4,4,4,3.75,8,2,
4,4,4,4,4,4,4,4,4,4,4,4,3.75,8,2,
2,4,4,4,8,8,4,4,4,8,8,4,4,4,4,2,
4,4,4,4,4,4,4,4,4,4,4,4,3.75,8,2 };
3. In Void Loop write your if statement:
if (ldr0 > 900)
{for (int thisNote = 0; thisNote < 61; thisNote++) { int noteDuration7 = 1000 / noteDurations7[thisNote];
tone(8, melody7[thisNote], noteDuration7);
int pauseBetweenNotes2 = noteDuration7 * 1.30;
delay(pauseBetweenNotes2);
// stop the tone playing: noTone(8);}}
Attachments
Step 7: Create the Instrument
Now all you have to do is make an instrument and place the photoresistors where you will move your fingers to in order to play the notes.
Step 8: Code
The second code is just in case you run out of pins on your first Arduino then you can implement a second Arduino into the schematic.
Step 9:
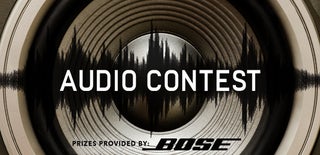
Participated in the
Audio Contest 2017