Introduction: Pi++
Hello Instructables! This is my first 'ible, and centered on my favorite hobby. Computer programming! This is no ordinary programming introduction however, as the whole process is 100% compatible, and in fact dedicated to the Raspberry Pi! (I also created this instructable on my pi. It was a little painstaking because I had to use Chromium browser, and anyone who has a pi knows that it is not exactly snappy :) ) The language used in this 'ible will be C++ and the interface through the command line because it is MUCH faster on the pi than trying to use Code::Blocks or some other IDE. Enough blather let's get to it!
P.S. I am entering this in the coding contest, so if you like it I would LOVE your vote :D
Step 1:
Step 2: Prerequisites
I will assume no knowledge of the topics in this 'ible, so let us start with the prebasics shall we?
First of all, you will need a text editor. Preferably one that does syntax highlighting. Here are a few of my faves, and the commands to install them. (Note. I will be using Vim for the instructible for simplicity, so grab this one if you have no idea what to do.)
First off, open a terminal, or use the login shell on your pi (Or other Linux box)
Vim: Command-line text editor with lexer/syntax highlighting. Line number display is default in the lower right hand corner.
SciTe: GUI editor. also has lexer/syntax highlighting for MANY languages. Line numbering can be turned on in menu/view/line numbering
Nano: Command line text editor shipped default with Raspbian, no line numbering but a simple interface. Has syntax/lexer highlighting for at least shell scripting and C++
To install any of these, type into the shell "sudo apt-get install <Name of editor here minus <>>"
If you have not updated your package lists recently, type "sudo apt-get update" to refresh the lists. (If you have no idea what I am talking about when I say package lists, type it because yours are old. The package lists hold all the package names available to download.)
You will also need g++ compiler for c++. Type "sudo apt-get install g++" to get it.
Okay. Enough boring downloading of software! let's get to making our own already.
Step 3: Before the .exe Comes the Code...
Well, now that you have downloaded and installed a highlighting text editor, you can get to the tortuous heaven of creating your very own gadgets/schedulers/multiphysics simulators. WAIT I forgot. we need to go through writing C++ code first... Well, let's dive right in. Make your first project file in the terminal by doing something like this:
--Create a directory for all your projects like this:
<pi@raspberry ~>mkdir /home/pi/Documents/c++
--Navigate into your new directory:
<pi@raspberry ~>cd /home/pi/Documents/c++
--Create a directory for this specific project like this:
<pi@raspberry ~>mkdir ./helloworld
--Navigate into your project directory
<pi@raspberry ~>cd ./helloworld
Okey dokey. Now you have a whole directory dedicated to your project. Time to get coding.
You can create a new C++ file and open vim at the same time by entering the command:
vim main.cpp
This will create a C++ file called "Main.cpp" in your project folder and enter the Vim CUI. Begin by typing into Vim:
:syntax on
Including the colon. This is a Vim command-line instruction. It activates syntax highlighting. After this is done, press i on the keyboard to enter into editing mode. The complete code for the ubiquitous Hello World program is below:
#include <iostream>
int main()
{
std::cout << "Hello World!" << std::endl;
}
Now, let's talk a little bit about this.
Step 4: Teach a Man to Fish...
It is all well and good to splice code snippets together, but to make anything totally original, you need to know the SYNTAX and, (arguably) less important conventions for the language you are trying to use. I will also go over several common C++ types, loops etc.
In the example:
#include <iostream>
int main()
{
std::cout << "Hello World!" << std::endl;
return 0;
}
I want you to notice several things. Firstly, the "#include" statement in the top line. This is called a header file, and the net result of including it, causes the code within the header to be "pasted" on top of yours. This is useful if you need to use libraries or create custom classes, the latter out of he scope of this 'ible. I used the include statement to include the iostream header of the standard library into my code. This allows me to write to the standard output and read form the standard input. I used the std::cout object to print "Hello World!" to the shell. This is one of the several objects defined in iostream, which makes it the header that I use the most. Notice that the main part of my code is situated between a set of curly braces after a line that says simply "int main()". This is called a function. It is a block of C++ code that can be executed by calling it by name. Every C++ file meant for executable compilation needs a function called "main". This tells the computer where to start executing the code compiled by g++ to properly run the program. The parentheses after the function are a place to pass arguments to the function (an argument is a variable or constant that can be provided to the function to change its behavior). Beneath the std::cout, there is a "return" statement. This is for allowing the function to give back a value of its own type when called. for instance. in this function:
int foo(int bar)
{
int foobar = bar * 5;
return foobar;
}
Called like this:
int barfoo = foo(10);
would return 50 to be assigned to barfoo.
Here is another vital syntax note. Every line needs a semicolon at the end UNLESS IT IS A FUNCTION, LOOP OR CONDITIONAL. Misplaced or unplaced semicolons will cause your code to not compile or to behave erratically. Now that we have some code let's compile it!
Step 5: Soo... Why Did I Get G++ Again?
Un-compiled, your code does not do much. It just kinda sits there looking like a Christmas tree with all its highlighting and being.. text.. So the next and last step in your journey to an executable is compilation! First you will need to save your code and exit Vim. To do this, hit the escape key to enter command mode, and enter the command ":x" This will save the file and exit to the shell. From there simply do this:
<pi@raspberry ~>g++ ./main.cpp
g++ will compile your code, and unless there are build errors will spit out a file called "a.out". To confirm, enter the command "ls" (ell ess) into the shell, and check for a file named "a.out".
Execute your shiny new program like this:
<pi@raspberry ~>./a.out
You should see something like this:
pi@skynet ~ $ mkdir ./Documents/c++
pi@skynet ~ $ cd ./Documents/c++
pi@skynet ~/Documents/c++ $ mkdir ./helloworld
pi@skynet ~/Documents/c++ $ cd helloworld
pi@skynet ~/Documents/c++/helloworld $ vim main.cpp
pi@skynet ~/Documents/c++/helloworld $ g++ ./main.cpp
pi@skynet ~/Documents/c++/helloworld $ ls
a.out main.cpp
pi@skynet ~/Documents/c++/helloworld $ ./a.out
This is the line with your program output! \/\/\/
Hello World!
pi@skynet ~/Documents/c++/helloworld $
Compiled and run! Give yourself a hug nice job.
Step 6: Error Is the Spice of Life
Ah you are still here! g++ gave you errors you say? hmm. Let's take a look.
pi@skynet ~/Documents/c++/helloworld $ g++ ./main.cpp
./main.cpp: In function ‘int main()’:
./main.cpp:6:2: error: expected ‘;’ before ‘return’
pi@skynet ~/Documents/c++/helloworld $
Hmm. Looks like someone did not pay attention about the semicolons >.< First off, the compiler tells you that the error is in int main() in main.cpp. so let's look at our main file. Then it tells you that the error is on line 6! Yes, that line you so meticulously typed out. The compiler is the most thorough spellchecker known to man. Let's open Vim back up and take a look-see.
vim ./main.cpp
#include
int main()
{ Caret\/
std::cout << "Hello World!" << std::endl ||
return 0;
}
~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ Line counter\/ ~ "main.cpp" 7L, 89C 6,1 All
Okay, so we are on line six... AH! the compiler was right. let's fix that line and compile again after exiting Vim. It worked this time? Great! we can learn about a few other helpful things as long as you came back.
Step 7: It's Time to Operate Doctor!
Before you can progress to loops and conditionals, you will need to know the C++ comparison and mathematical operators. There are quite a few to remember, but proficiency will come with practice.
Here is the list:
== Comparison operator "is equal"
!= Comparison operator "is not equal"
<= Comparison operator "is less than or equal to"
>= Comparison operator "is greater than or equal to"
< Comparison operator "less than"
> Comparison operator "greater than"
|| conditional operator "or"
&& Conditional operator "and"
= Assignment operator "equals"
+ Math operator "add"
- Math operator "subtract"
/ Math operator "divide"
* Math operator "multiply"
-- Math operator "decrement"
++ Math operator "increment"
% Math operator "remainder of division"
Step 8: Exterme Conditioning
Well, we have looked at the VERY basics of C++ in our example program, but what if you want your code to have two possible outcomes? Statements called CONDITIONALS were born for the task.
There are three basic conditionals. Namely:
if
else
switch
if/else statements are the easiest, so let's start there. In this example code:
if (1 == 1)
{
std::cout << "The universe has survived!;
}else
{
std::cout << "This computer no longer exists...";
}
IF one is equal to one, print one thing, ELSE print something else. notice the "==" operator instead of "=" "==" is a comparison whereas "=" is an assignment. Here are some other operators:
!= Is not equal to
++ Increment by one
-- Decrement by one
+ Add
&& And
|| Or
>= Greater than / equal to
<= Less than / equal to
/ Divide
* Multiply
- Subtract
% Modulus find the remainder of a division
Those are all of the non-advanced C++ default operators, and all you should need for both conditional statements and loops. Let's get to the next statement, switch.
int foo = 3;
switch(foo)
{
case 1:
std::cout << "One";
break;
case 2:
std::cout << "Two";
break;
case 3:
std::cout << "Three";
break;
}
In a switch, the case whose value matches the value of the variable used (must be an int or enum) is selected and run. The break keeps the branch from looping infinitely. You may also add a default case to the end to handle an input outside what is expected.
Step 9: Getting Loopy
Another critical element of C++ is the loop. There are three kinds of loops, while, for and do.
Let us begin with the while loop.
int foo = 0;
while (foo < 100)
{
std::cout << foo;
foo++;
}
This code snippet will print every integral number from 0-100 to the console. The syntax of a while loop is: while(condition) {COMMANDS} the loop will run as long as condition is evaluated as true. You can make an infinite loop by putting '1' or "true" as the condition.
Here is a snippet of a for loop:
for (int i = 0; i < 100; i++)
{
std::cout << i;
}
This kind of loop is good for applications where you want to perform an incremental operation such as looking at each letter of a string or each element of an array. The syntax of a for loop is:
for (local variable;condition;callback){COMMANDS}
The code snippet from above initializes an integer variable 'i' and runs the loop as long as i's value is less than 100 incrementing it every loop cycle. The last loop to go over is a do. Here is another snippet:
do
{
std::cout << "Spam";
}while(true);
Syntax is: do {COMMANDS}while(condition); The do is very similar to the while, except it is guaranteed to run at least once. Also, notice the semicolon after the while portion. it is easy to forget.
This loop will run forever because the "true" inside the parentheses is equivalent to saying if 1 == 1.
Step 10: Do You Know How to Type?
Unlike in most scripting languages, in C++ you have to define the type of any variable you create. That is, what kind of value it will hold. I will go over the VERY basic types, because there are a tremendous number of types (several hundred) and it would take hours to type them all.
So here are the basic types:
int An integer number
float A floating point number holds decimals
double Similar to a float, but has greater precision
bool A Boolean value. Either true or false (0 or 1)
std::string A sequence of characters, requires <string> header
char A single character
To declare and initialize a variable, use this syntax:
typename name = value;
So you could initialize an integer variable with a value of sixteen like this:
int foo = 16
You can make an ARRAY of any type by declaring it like this:
type NAME [integer value] = {values};
This will create an array with a number of elements equal to the value between the brackets. you can index an array like this:
int foo[3] = {1,2,3};
std::cout << foo[0] << " " << foo[2];
This would output 1 3. Array indexing begins at zero.
Step 11: Whaddya Gonna Do Now Coder?
Well, your short time in my tutelage has come to an end (for now) For your further edification and education. check out these sites:
stackoverflow
cplusplus.com
stackexchange
Happy coding!
Dominic
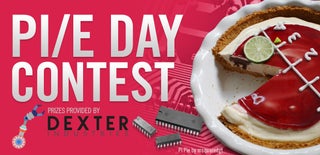
Participated in the
Pi/e Day Contest
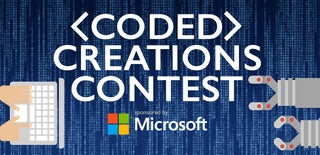
Participated in the
Coded Creations