Introduction: Play Ode to Joy and Other Classical Music With Arduino
This is a basic tutorial (Great for beginners!) using the Arduino Uno or similar version to play Ode to Joy by Beethoven. This was one of my first projects with Arduino.
What You Will Need:
-Arduino Uno or similar version, which is Arduino compatible. (I used the Uno R3 Plus)
-Jumper Cables X7
-Transistor
-Buzzer
-1kOhm Resistor X2
-Small Breadboard
-USB Cable
NOTE: These can all be purchased at most electronics or hobby stores, such as:
http://www.element14.com/community/welcome
You will also need to download the Arduino IDE software to write your program. This can be found at
Step 1: Examine the Circuit
The above picture is the circuit diagram for this project, it is a good idea to look at it and refer to it later to visualize the layout. As you can see the circuit is quite simple, but make note of which wires go where, otherwise it will not function properly.
Step 2: Wire the Breadboard
The pictures show most of the layout for the project, however I will give directions so mistakes are less likely.
-Connect one end of a jumper cable to A,25, leave the other end, I will refer to it later as cable 1.
-Add a 1KOhm resistor with its terminals in D,25 and D,21.
-Add a new jumper cable with its terminals in E,25 and F,25.
-Add the buzzer with positive terminal in J,25 (depending on the size of the buzzer, where the negative terminal attaches may very, however it will be in line J. Mine ended up in J,22. Keep note of where this terminal enters as it will affect the next two steps).
-Add a new jumper cable with its terminals in E,21 and F, location of negative terminal of buzzer.
-Add a new jumper cable with its terminals in G, location of negative terminal of buzzer and G,14
-Add a 1kOhm resistor with its terminals in D,13 and D,9.
-Add a new jumper cable with its terminals in E,13 and F,13.
-Connect one end of a new jumper cable to E,9, leave the other end, I will refer to it later as cable 2.
-Connect one end of a new jumper cable to G,12, leave the other end, I will refer to it later as cable 3.
-Add the transistor with flat side facing the resistors and terminals in I,14 and I,13 and I,12.
Congratulations you have now wired the breadboard!
Step 3: Connect Breadboard to Arduino
Attach the wires as follows to the Arduino board.
-Wire 1 attaches to 5V Pin
-Wire 2 attaches to I/O 8
-Wire 3 attaches to GND (Ground) Pin
Congratulations, all the wiring is now set up... Wasn't that easy?
Step 4: Program Your Project
Open the Arduino IDE sketch software and plug your Arduino board into your computer via USB.
Copy and paste the following code into the sketch. Now go up to tools and ensure that the board is selected as Arduino Uno and the serial port is correct (this can be checked by going into device manager in the control panel and see which port disappears when the Arduino is unplugged). Now upload the sketch by clicking on the arrow pointing right in the top left corner of the screen.
If all goes well you should hear the buzzer playing a serenading melody.
Here is The Code:
/*
Buzzer tutorial
*/
int buzzerPin = 8; //using digital pin 8
#define NOTE_C6 1047
#define NOTE_D6 1157
#define NOTE_E6 1319
#define NOTE_F6 1397
#define NOTE_G6 1568
#define NOTE_A6 1760
#define NOTE_B6 1976
#define NOTE_C7 2093
void setup()
{
//nothing to set up
}
void loop(){
//tone(pin,frequency,duration)
tone(buzzerPin, NOTE_A6, 500);
delay(500);
tone(buzzerPin, NOTE_A6, 500);
delay(500);
tone(buzzerPin, NOTE_B6, 500);
delay(500);
tone(buzzerPin, NOTE_G6, 500);
delay(500);
tone(buzzerPin, NOTE_A6, 500);
delay(500);
tone(buzzerPin, NOTE_B6, 500);
delay(200);
tone(buzzerPin, NOTE_C7, 500);
delay(300);
tone(buzzerPin, NOTE_B6, 500);
delay(500);
tone(buzzerPin, NOTE_G6, 500);
delay(500);
tone(buzzerPin, NOTE_A6, 500);
delay(500);
tone(buzzerPin, NOTE_B6, 500);
delay(200);
tone(buzzerPin, NOTE_C7, 500);
delay(300);
tone(buzzerPin, NOTE_B6, 500);
delay(500);
tone(buzzerPin, NOTE_A6, 500);
delay(500);
tone(buzzerPin, NOTE_G6, 500);
delay(500);
tone(buzzerPin, NOTE_A6, 500);
delay(500);
}
Now that you have programed your Arduino try playing with the sketch. The values next to the notes at the top change the frequency of the buzzer note, the 500, 300 and 200 values indicate the delay in milliseconds. Go and make your own melody for your Arduino to play.
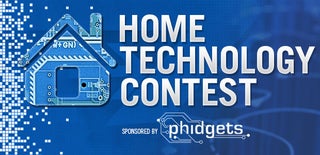
Participated in the
Home Technology Contest