Introduction: Playing Minecraft in the Terminal
Just to make it clear, you are not exactly playing Minecraft on the terminal. What you will be doing is that you will be playing the ASCII version of whatever window you happen to have opened on your monitor.
Supplies
Python (I am will be using Python 3.x)
Required Libraries
- Pillow (Python Imaging Library)
- os (Comes installed with python)
Second monitor (If you do not have this, please refer to the next step)
Step 1: Making a Second Monitor (Optional)
If you do not have a second monitor, you can use an app called Spacedesk. Install the server software on your laptop and the client software from the AppStore or Playstore on your Ipad or Tablet. Both your laptop and tablet must be connected through the same network so that they can be connected. Open the app on your tablet and click on your Laptop's IP and your tablet should be now working as a secondary monitor.
Their documentation can referred for a detailed setup. https://www.spacedesk.net/user-manual/
Step 2: How This Works
First let us understand how this works and what we are going to do. On one of our additional monitors, we can open up a window of Minecraft or any app for that matter. We will grab a picture of that display, and then convert it to ASCII characters. We can then print this on the terminal!
Step 3: Coding
Grabbing the Screen
To grab the portion of our screen we shall make use of the Python Image Library or Pillow. Lets import the library first.
import PIL.ImageGrab
Now grab the screen. Here 'bbox' is the portion of the screen we are referring too. This will return an image
image = PIL.ImageGrab.grab(bbox=(start_x, start_y, end_x, end_y), all_screens=True)
For me following are the coordinates
image = PIL.ImageGrab.grab(bbox=(-1024, 0,0, 768), all_screens=True)
ASCII Converter
Now we should convert the image to an ASCII string. To do this we will first resize the image to fit our terminal. The new height of the image must be the number of lines our terminal has. Next, we find the ratio between the height and the width. Lets put this in a function.
def ascii(image, new_height): width, height = image.size ratio = height/width/2 new_width = int(new_height/ratio) resized_image = image.resize((new_width, new_height))
Now you may be wondering, why we are dividing by 2 in the ratio declaration line. The answer is quite simple. Since characters on the screen have an unequal height and width and are not a square, the ratio between the height and width of the ASCII characters must divided, otherwise the image will be not in proportion. In our image, the pixels will be rectangular and not square.
Now lets convert this image to a gray-scaled one.
... gray_image = resized_image.convert("L")
To convert this into an ASCII image, we will analyze the image's pixels. Since the image is gray-scale, some pixels will be darker and some lighter. We can replicate this with ASCII characters, since some appear to occupy more space than the other and appear darker. We shall declare a list of such characters.
ascii_chars = ["@", "#", "S", "%","?", "*", "+", ";", ":", ",", "."]
Now we shall create a string that corresponds to the pixel darkness value of the image.
... pixel_data = gray_image.getdata() string = "".join([ascii_chars[pixel//25] for pixel in pixel_data])
All that is left, is to add new line after a certain number of characters that is, the new_width.
... length = len(string) ascii_string = "\n".join([string[i:(i+new_width)] for i in range(0, length, new_width)]) return ascii_string
Making it Run
Now we will simply put all this in a while loop, get the image, pass it through our ascii() function, print it and clear the screen.
import os while True: image = PIL.ImageGrab.grab(bbox=(start_x, start_y, end_x, end_y), all_screens=True) ascii = ascii(ascii, height) print(ascii) os.system('cls')# If you are on Mac or Linux write os.system('clear')
Step 4: Running the Code
The full code can be found on my GitHub .
On your additional monitor open up any app of your choice, which is Minecraft in our case.
Now go on your primary monitor and open up the terminal. Right click on the top bar of the window. Once a list is opened, click on 'Properties' and a window will open. Go on the 'Fonts' tab and reduce the font size to the smallest option available, to get a better resolution. Change your directory to the one where your python file is with this code. Type 'python' and your filename and click enter. Enjoy the experience!
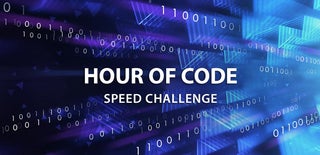
Participated in the
Hour of Code Speed Challenge