Introduction: Possessed Halloween Book Stand!
Ever wanted to scare your friends and family with a truly spooky Halloween Decoration? Look no further! This little creation is sure to add a touch of magic to your Halloween festivities!
Using a small DC motor and a precise servo mechanism, this device turns the pages of a book with eerie precision. The result? An enchanting and mesmerizing book stand that's sure to confuse and surprise any audience!
Supplies
The Main Items that you need:
- 3D Printer + Filament
- Book
- DC Motor
- Arduino
- L298N Motor Driver Module
This project only uses a few components which means it's easy to create!
Full Parts List:
- 3D Printer
- Black PLA Filament
- Book (Hardcover books worked better in my experience)
- 2x M3*30 Screws (For mounting the DC motor, mine came with these screws)
- 9G Micro Servo
- Geared DC Motor
- Arduino Nano, Uno, etc.
- L298N Motor Driver Module (You can find these pretty much everywhere for around ~3$!)
- Large and Bright Yellow LED (Optional if you're going to print the tree.)
- Some tape
Step 1: Designing the Model
Designing the book flipper mechanism was quite easy once I got a few ideas from YouTube. However, the hardest part was designing the tree since this was my first time using Blender!
First, I watched a couple of videos on designing a stump and then made my own. Afterwards, I used shading in Blender to get some bark displacement and colour onto the model using this tutorial. However, while my renders looked great, the displacement was only on the render and not the mesh itself. So, after a few long hard days of learning, I managed to bake the map onto the actual mesh itself and export it as an STL!
Step 2: 3D Printing
First, head over to my Thingiverse page in order to download the required models!
The files named
- Base1
- Base2
- Base3
- Base4
- Lid1
- Lid2
- ServoARM
- Roller
Are all required for the book flipper itself.
However, the "Tree" file is optional, since it is merely the stand. If you do decide to print it, you will need to cut the model into smaller pieces to fit on most build plates. I cut mine into four equal pieces and printed them at 0.28 layer height and 4-5% infill in order to get a really nice diffused look!
After you've done that, simply slice and print the models!
I used eSUN PLA + Black for the entire decoration, but I would definitely recommend using a lighter colour such as white since the light from the tree is more diffused and much spookier!
Step 3: Assembling the Electronics
The electronics will be split into two different compartments. Base1 will hold both the Servo and the Motor. Base2 will store the Arduino and the Motor Driver.
Assembly with Base1
- First, slide the Servo into the allocated slot with the wire going in first.
- After that, mount your motor to the book stand by using the two screws that I mentioned in the Parts List!
Assembly with Base2
Simply store your Arduino and Motor Driver so there is enough space to connect the pins while also not going outside the box!
Step 4: Setting Up the Servo and Motor
Using some tape (I used black electrical tape) wrap it around the Roller with the sticky side facing outward!
This is so the motor will be able to get a grip on the page to curl it upwards
After that, attach the ServoARM to the Servo using a small screw that should come with your servo.
Step 5: Connecting the Electronics
The connections are very simple and only require a few wires. Check out the Schematic or the table below. Of course, you can change any of these by simply modifying the code which we will get to soon!
Pinout:
Arduino = L289N
D9 = ENA
D2 = IN1
D3 = IN2
Arduino = Servo
5V = +
GND = -
D10 = Signal
L289N
Out1 = Motor +
Out2 = Motor -
12V/VIN = 9V Battery +
GND = 9V Battery -
For the purposes of this tutorial, I used an Arduino Uno since it was easier to debug.
Step 6: Assembly of the Body
Assembling the body is simple. Just slide the two lids over Base1 and Base2 and then connect the remaining bases together! The pictures are self-explanatory.
If the connections are loose, you can use some glue to hold them together but make sure that the flipper mechanism itself works before you permanently stick them!
Step 7: The Code!
Now to begin coding!
The code is relatively simple since it only turns the motor on and off and moves the servo back and forth.
You can download the PossesedBookStand.ino file or copy and paste it from below.
#include <Servo.h>
Servo servoMotor;
int motorAPin1 = 2; // Connect L298N IN1 to Arduino pin 2
int motorAPin2 = 3; // Connect L298N IN2 to Arduino pin 3
int motorEnablePin = 9; // Connect L298N ENA to Arduino pin 9
int servoPin = 10; // Connect servo signal pin to Arduino pin 10
void setup() {
pinMode(motorAPin1, OUTPUT);
pinMode(motorAPin2, OUTPUT);
pinMode(motorEnablePin, OUTPUT);
servoMotor.attach(servoPin);
servoMotor.write(200);
}
void loop() {
// Turn motor A for 0.11 second
digitalWrite(motorAPin1, HIGH);
digitalWrite(motorAPin2, LOW);
analogWrite(motorEnablePin, 255);
delay(170);
// Stop motor A
analogWrite(motorEnablePin, 0);
delay(2000);
// Move servo to 180 degrees
servoMotor.write(0);
delay(2000);
// Move servo back to 0 degrees
servoMotor.write(200);
delay(3000);
}
Time for the code explanation!
#include <Servo.h>
This line includes the Servo library in the code, without it, we wouldn't be able to control the servo!
Servo servoMotor;
This line sets up a Servo named servoMotor in our code
int motorAPin1 = 2; // Connect L298N IN1 to Arduino pin 2
int motorAPin2 = 3; // Connect L298N IN2 to Arduino pin 3
int motorEnablePin = 9; // Connect L298N ENA to Arduino pin 9
int servoPin = 10; // Connect servo signal pin to Arduino pin 10
These lines specify what pins the Motor Driver Board and the Servo is connected to. You can change this part of the code to change what pins control what.
void setup() {
pinMode(motorAPin1, OUTPUT);
pinMode(motorAPin2, OUTPUT);
pinMode(motorEnablePin, OUTPUT);
servoMotor.attach(servoPin);
servoMotor.write(200);
}
This section tells the Arduino that the specified pins are outputs. It also sets the Servo pin and turns the Servo to 180 degrees which in our case is the default angle.
void loop() {
// Turn motor A for 0.11 second
digitalWrite(motorAPin1, HIGH);
digitalWrite(motorAPin2, LOW);
analogWrite(motorEnablePin, 255);
delay(170);
// Stop motor A
analogWrite(motorEnablePin, 0);
delay(2000);
This section turns the DC motor on and off quickly in order to get a small curve on the page.
If you want to learn more about the L298N motor driver, check out this website for a detailed guide!
// Move servo to 180 degrees
servoMotor.write(0);
delay(2000);
// Move servo back to 0 degrees
servoMotor.write(200);
delay(3000);
}
Finally, this part of the code turns the Servo motor to 180 degrees in order to flip the book and then turns it back!
Once you have uploaded your code, make sure to test it out with a book so it works!
When it does, you can use some glue like I did to permanently fix the Bases together.
Attachments
Step 8: Preparing the Tree
If you printed the Tree, there is one more step to follow!
Since the tree is so large, you will most likely have to print them in four pieces and stick them together using some glue.
In order to make the tree light up, you will need a light source that is preferably yellow and bright.
I used two large 10mm yellow LED powered by a coin cell battery for the light, however, I would recommend using something even brighter like an electric candle.
The pictures of the tree above were printed in white filament but having darker colours will make it harder to see the light
DO NOT use a real candle since it will melt and burn the plastic!
Step 9: Testing
Finally, you can test it out!
Grab a hardcover book and place it under the roller. You may need to tweak your code and book placement in order to find the sweet spot!
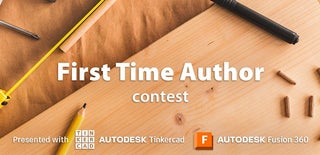
Runner Up in the
First Time Author