Introduction: Programming Brushless Motor ESC Without RF Transmitter
This instructable is on programming ESC controllers without RF transmitters or ESC programmers. I know that there are already quite a few of them around (such as this), but for my particular ESC their method did not work out too well out of the box, although my solution is basically a small variation on those. I will also cover the basics of how to interface an Arduino and an ESC.
For this tutorial you are going to need:
- An Arduino or an Arduino clone. I only have a Uno so you may (but probably won't) have to adapt bits and pieces to your own version.
- Some software to program the Arduino with. Could be the Arduino IDE, could be CLION, could be any IDE or even command line Makefile sorcery, as long as it can push code to the Arduino.
- Serial communication to the Arduino. This could simply be taken care of by the default USB cable used for programming, or you could use any serial communication medium e.g. over Bluetooth.
- A serial monitor software. The most convenient one for most people is obviously the one that comes packaged with the Arduino IDE.
- A brushless motor. I have and EMAX 2215/25, or this.
- A brushless ESC with a 5V BEC that is suitable for your motor. I have a 20A Simon Series ESC, or this. You will also need its manual.
- An appropriate LiPo battery for the motor. Although the power Arduino provides is sufficient for the ESC, you also need to power up the motor to an extent. You will probably find that you can't hear the tones that guide you during programming very well at that power level. Plus, you will not be able to test the initial setup properly without one since obviously the tiny current from the Arduino cannot turn your propeller unless we are talking very small motors.
- A 120-ohm resistor, or a combination of resistors that add up to 120 ohms.
- 3 male to male jumper wires
Also you might be glad to learn there is very very little actual coding involved, and the whole thing is reasonably straightforward if you know a few tricks.
Step 1: Connecting the Components
A rule of thumb I have for any project, but especially for electronics projects which, unusually for a programmer like me, involve physical pieces, is build a little test a little. Otherwise you can easily end up building on stuff that doesn't work in the first place. So, let's start with how to hook things up and testing whether the basics work.
Before anything, make sure that the batteries are the last connections made here. LiPo batteries that people tend to use for such motors are high discharge beasts, and you don't want them connected to your ESC unless you actually need to move the motor.
Let's break down this schematic:
- Connect the signal pin of the ESC (this will probably be the yellow wire) to D9 pin of the Arduino. Note that D9 is chosen because it is a PWM capable pin and I have developed a habit of choosing it for my ESC for some reason, and you can change to another such pin if D9 isn't good for you.
- Connect the power (red wire) and ground (black wire) connections of the ESC to the VIN and GND pins, respectively. Note that VIN is a different port than 5V. You can choose any GND pin you wish, I chose GND1 because it is next to VIN. Do not mess up which cable goes where here. You might damage your Arduino.
- The most important piece that was missing from other instructables for me was the 120-Ohm resistor R1 connected to RESET and 5V pins of the Arduino. This prevents the Arduino from auto-resetting whenever a serial port connection is established. I will return to why this matters later, for now it is sufficient to note that you need to remove this resistor before uploading new sketches. This is because the auto-reset seems to be required for the upload procedure and running of the newly uploaded sketch without cutting the power supply. You can replace the resistor after the upload is complete and the new code is running.
- Connect the three cables from the brushless motor to the three motor pins on the ESC. The order is significant since it determines the default direction of the motor, but it is not a big deal to fix it. If during testing you notice the direction is not right you can simply swap any two of the three cables and that should fix things for you.
- Finally, and only when you are ready to run the thing, connect the batteries. WATCH THE POLARITY, DOUBLE CHECK EVERY TIME, RED TO RED, BLACK TO BLACK. Otherwise you will fry your controller (at least the part that controls the motor) almost instantly (as my previous two ESCs can testify).
- You should hear some beeping tunes from the motor as the ESC is initialised, and should check with your manual to confirm you get the "everything is a-okay" beeps. Go ahead and remove the battery connections for now. It is super important that you don't short the battery so watch those leads while they are not connected.
Step 2: Testing Basic Motor Functionality
Now in order to test this setup, we are going to hook the Arduino up with a computer using a USB cable in order to upload a test sketch. Remember to remove the resistor between RESET and 5V before uploading the sketch. Also make sure that the battery is not connected and everything seems off.
Once the resistor is removed, in order to upload a sketch, use the USB cable between the computer and and the Arduino. This should be sufficient to power up the Arduino and the ESC without dealing with big currents from the LiPo. Upload the following sketch (also available as a gist):
#include <Arduino.h> #include <Servo.h> static const int PROP_PIN = 9; Servo brushless; void setup() { Serial.begin(9600); brushless.attach(PROP_PIN); brushless.writeMicroseconds(0); //set initial value, check your manual Serial.println("Ready to receive commands"); } void loop() { if(Serial.available()) { long value = Serial.parseInt(); brushless.writeMicroseconds(value); Serial.print("Set PWM to "); Serial.print(value); Serial.println("ms"); } }
One important point is the value ESC expects upon startup. You should consult your manual for this. My manual states starting out with the throttle all the way
After the upload, open the serial monitor. You should see the ready message. Now you can submit values and hear your motor struggle to move and make clicking noises.
If you actually want it to move, e.g. to test the direction of the motor is correct, you have to connect the batterybefore connecting the USB cable. The ESC needs to detect the battery properties upon startup, so I am assuming you can't just plug the battery in while the ESC is powered by the Arduino but to be honest I haven't tried it. MAKE SURE THE PROPS ARE OFF WHILE TESTING.You wouldn't believe how deep they can cut once broken.
Step 3: Programming the ESC
Now that we know our connection works, we have to dive back into our manuals to find how our ESCs are programmed using a transmitter.
We are essentially going to use the serial connection as a replacement for the transmitter, with 2000 (the highest PWM width ESC can detect) representing "pushing the throttle stick all the way up", and with 0 representing "pulling the throttle stick all the way down". For my equipment, the ESC needed the throttle stick all the way up during initialisation to enter the programming mode.
You can take the following code (or the gist here) and modify it to suit your particular case. It reads either 0 or 1 from the serial console and respectively minimises or maximises the signal to the ESC. Remember to remove the resistor before the upload.
#include <Arduino.h> #include <Servo.h> static const int PROP_PIN = 9; Servo brushless; void setup() { Serial.begin(9600); brushless.attach(PROP_PIN); brushless.writeMicroseconds(2000); } void loop() { if(Serial.available()) { byte message[1]; Serial.readBytes(message, 1); char msg = (char) *message; if(msg == '0') { brushless.writeMicroseconds(0); Serial.println("MIN"); } else if (msg == '1'){ brushless.writeMicroseconds(2000); Serial.println("MAX"); } } }
The steps are simple.
- Connect the battery.
- Connect the USB cable to the Arduino and your computer.
- Open serial monitor.
- Follow the instructions on your manual to program whatever setting you wish, replacing pushing the stick up with typing 1, and pulling it down with typing 0 in the console.
This is the point where the resistor plays its role. Especially if your ESC starts off the programming with the maximum throttle, you will notice that unless you replace the resistor, the moment you establish the serial connection the ESC will react. This is because the initialisation of any serial connection resets the Arduino. Since this also changes the value of D9, it ruins our attempt at getting to the right menu, or can even exit the programming mode. This was the original reason why other instructables did not work for me, and also why I am writing one right now.
So, following the suggestion here, we introduce a 120-Ohm resistor between RESET and 5V in order to suppress the reset and voila. Now serial connections do not disturb the sequence of things. You can listen to the tones and react by typing 1 or 0 in the serial monitor.
Final Words
This setup is extra useful when you prefer establishing connections over Bluetooth, since it means newly connected devices do not cause the Arduino sketch to restart which might be undesirable in some cases. However, note that Bluetooth modules tend to be kind of slow to respond and are not too suitable for actually flying things in real time. My use case does not require that kind of temporal resolution so it works.
And that's about it. It is not very complex as I said in the introduction, but it does have a couple of finer points that might take more time than they deserve if you are unaware of them.
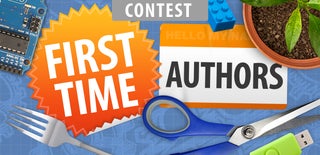
Participated in the
First Time Authors Contest 2016