Introduction: RFIDuino
This is my first Instructable, I may have missed some things in this. I will edit if I find something missing.
If the embedded videos don't work, links:
Uses RFID reader, Arduino Uno, PHP/MySQL/Apache.
1. Reader sends data to Arduino
2. Arduino sends data to web server
3. PHP
A. Decodes Card Data
B. Checks date
C. Checks database for card #, if user is active, if they are allowed in today@current time
D. If access granted
i. Log user info to database
ii. Send "Open Password" Over UDP to the sending Arduino
iii. Arduino decodes UDP Packet
a. If "Open Password" is correct, send signal to transistor which will close the strike's ground circuit and open and change led.
b. If "Open Password" is incorrect, do nothing.
iv. Send "Flush" packet over UDP to the sending Ardiuno
E. If Access Denied
i. Log user info to database
ii. Send "Flush" packet over UDP to the sending Ardiuno
Step 1: Assemble RFIDuino
UDPSendReceive.pde:
This sketch receives UDP message strings, prints them to the serial port
and sends an "acknowledge" string back to the sender
A Processing sketch is included at the end of file that can be used to send
and received messages for testing with a computer.
created 21 Aug 2010
by Michael Margolis
This code is in the public domain.
*/
#include <SPI.h> // needed for Arduino versions later than 0018
#include <Ethernet.h>
#include <EthernetUdp.h> // UDP library from: bjoern@cs.stanford.edu 12/30/2008
// Enter a MAC address and IP address for your controller below.
// The IP address will be dependent on your local network:
byte mac[] = { 0xDF, 0xAD, 0xCE, 0xEF, 0xFE, 0xWE };
byte ip[] = { 0, 0, 0, };
IPAddress server(0,0,0,0);
char serverName[] = "http://whatever.com";
int keepAlive = 0;
volatile long reader1 = 0;
volatile int reader1Count = 0;
unsigned int localPort = 70056; // local port to listen on
char packetBuffer[UDP_TX_PACKET_MAX_SIZE]; //buffer to hold incoming packet,
EthernetClient client;
EthernetUDP Udp;
void reader1One(void) {
reader1Count++;
reader1 = reader1 << 1;
reader1 |= 1;
}
void reader1Zero(void) {
reader1Count++;
reader1 = reader1 << 1;
}
void setup() {
Ethernet.begin(mac,ip,dns);// start the Ethernet
Udp.begin(localPort);// and UDP
Serial.begin(9600);
pinMode(5, OUTPUT);
// Attach pin change interrupt service routines from the Wiegand RFID readers
attachInterrupt(0, reader1Zero, RISING);//DATA0 to pin 2
attachInterrupt(1, reader1One, RISING); //DATA1 to pin 3
delay(10);
// the interrupt in the Atmel processor mises out the first negitave pulse as the inputs are already high,
// so this gives a pulse to each reader input line to get the interrupts working properly.
// Then clear out the reader variables.
// The readers are open collector sitting normally at a one so this is OK
for(int i = 2; i<4; i++){
pinMode(i, OUTPUT);
digitalWrite(i, HIGH); // enable internal pull up causing a one
digitalWrite(i, LOW); // disable internal pull up causing zero and thus an interrupt
pinMode(i, INPUT);
digitalWrite(i, HIGH); // enable internal pull up
}
delay(10);
reader1 = 0;
reader1Count = 0;
}
void loop() {
if(reader1Count >=26){ //If card is read.
Serial.print(reader1);
client.connect(server, 80); //Connect to Server.
client.print("GET rfiddoor/door.php?data="); //Send card data.
client.print(reader1);
client.println("&door=12door");//Send door name.
if(client.connected()){
Serial.print("Sending Card Data (");
Serial.print(reader1); //print card data to serial output.
Serial.println(") to Server....");
}else{
Serial.println("Cant connect to Server, Retrying...");
}
client.stop();
}
reader1 = 0;
reader1Count = 0;
delay(100); //Give arduino time to receive the UDP packet.
int packetSize = Udp.parsePacket(); //Process UDP packet.
if(packetSize){ //if UDP packet is received act, else keep card data and resend data(start loop over while retaining card data).
Serial.print("Packet Contents:");
IPAddress remote = Udp.remoteIP();
Udp.read(packetBuffer,UDP_TX_PACKET_MAX_SIZE);//Read packet into buffer.
Serial.print(packetBuffer);//Print UDP packet.
Serial.print("Card is ");
if(packetBuffer[0] == 'p' && packetBuffer[1] == 'a' && packetBuffer[2] == 's' && packetBuffer[3] == 's' && packetBuffer[4] == 'w' && packetBuffer[5] == 'o' && packetBuffer[6] == 'r' && packetBuffer[7] == 'd') { //If UDP packet contains password open, else stay closed
digitalWrite(5, HIGH);// ### This will read the packet buffer. If it contains the "open command" it will keep the strike ###
Serial.println("Approved");// ### open for 5 seconds and print out that the card was approved. The webclient will be stopped ###
//client.stop();// ### the reader will be reset. ###
//reader1 = 0;// ### If the "denied command" is received it will stay locked and it will print out that the card ###
//reader1Count = 0;// ### was denied. The webclient will be stopped and the reader will be reset. ###
delay(5000);// ### ###
digitalWrite(5, LOW);// ### If a packet isn't recieved it will keep the reader data and resend. ###
}else{// ###################################################################################################
Serial.println("Denied");
//client.stop();
//reader1 = 0;
//reader1Count = 0;
digitalWrite(5, LOW);
}
Serial.println("____________________________________________");
}
}
Step 2: Prepare MySQL Database
Step 3: Make Access PHP Script
Step 4: Create a Web Gui to Show/add Users
The second is the actual list of users
Step 5: GUI to Edit User Info
Step 6: Add Doors
It will also remove white space and the word "door".
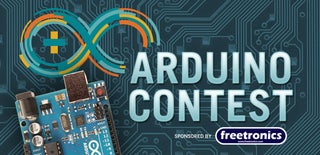
Participated in the
Arduino Contest