Introduction: Roblox Lua Basics
This tutorial will go over the basics of Lua in Roblox studio and maybe a bit more to begin on your Roblox game dev experience.
Supplies
Roblox studio
Step 1: Variables
A variable is a named unit of data that is assigned a value. These are simple but essential for basically every script you make and you can use these to store values. For example you could have a variable called Ammo and make Ammo=(any number you need it to be). To make a variable you start with local then the name of the variable(it shouldn't have spaces or any symbols other than underscores)then to define it an = (this is optional if you don't want to define our variable right now this and the next step aren't useful to you) then type what should be stored in it(you can make this letters, numbers, symbols but no spaces). An example would be local ammo = 100 or local favoritefood = "pizza". Notice the ""? its just there when pizza is just a word and doesn't mean anything like I could write local copy = original and it would check for what original is in the script and if it doesn't find anything it returns a error because original hasn't been defined. That's why you need to put "" unless you are trying to find something you have previously defined. For example you may have made a variable like local mycar = script.parent where its contents don't have a "" but instead are real things. When you do something like putting a full stop after a part it will check for its children(the things under it) or if you write parent, the parent to the part.
Step 2: If Statements
If statements are just something that checks if a condition is met and if it isn't the code inside it wont run. It starts with if then the condition (in my example it is if ammo==5. Double equals signs are used to see if something is that and doesn't change the thing to be that if you get what I'm saying) then if you did it right and you press enter a then and an end will appear. Write your code in-between the then and end. You can have more if statements inside if statements as you can see in my example when I write elseif ammo>5 then and even more code but it is not necessary depending on what you are trying to achieve. You can also put just the else and no if but then you cant specify the condition and if the if statements condition is false it will just switch to the else part. When I run the code it outputs the amount of ammo in the log because the ammo is 10 and that doesn't == to five.
Step 3: Loops
loops are just made to repeat code until a specific condition is met. There are a bunch of loops so ill just talk about 2. While loops and repeat loops.
Step 4: While Loops
A while loop repeats a code until the condition it specifies is true. It checks the condition before repeating or carrying on with the program. To make one you need to write while then your condition (mine is to check if ammo is more than 0) then press enter and it should add a do and a end. Like a if statement, you write the code you want repeated in-between the do and end. And if you write code below the while loop it wont run until the while loop ends. If you write true for the condition then it will become an infinite loop.
Step 5: Repeat Loops
Just like while loops but the condition is only checked at the end so be wary of that. To write it just do repeat then press enter and an until should appear under it. write your condition after the until and your code in-between until and repeat.
Step 6: Break
When running an infinite loop or you just want to break the loop you can use break which stops the loop and carries on with the program. Put this in the loop with an if statement to carry on code if the condition is true or to keep looping until it is met.
Step 7: Functions
Functions are basically variables that store bunches of code. You can call this code any time and anywhere in the script as long as its after defining the function, not before. To make one do function putnamehere then press enter. A set of brackets and an end should appear. In those brackets you can add parameters, which are just variables that you define when your calling a function.
Calling a function is actually using the code you wrote basically. Do it by writing the name of the function and in the paranthesis put the values you want to go through the codes process.
Step 8: Pre Defined Functions
If you checked the pictures you must have seen print(). Its what's called a predefined function where you don't have to make the function and Roblox or Lua has offered it o use or if your using a plugin the creator. with print you can output a lot like words, names, variables- by just putting them in the brackets. Keep in mind that when you just want to put things that haven't been assigned to a value or sentences and words, you need to use "" and put your stuff inside of them. An example would be-
print(" Hello world is overrated and that's why I didn't begin with it") or
local words = "dogs like walking"
print(words)
Step 9: Thats It
Thats about most of the important lua code I remember and i hope this helps you get a basic understanding of lua.
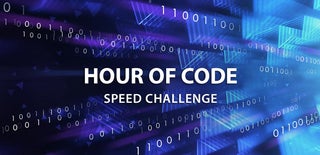
Participated in the
Hour of Code Speed Challenge