Introduction: Senior Radio - Raspberry Pi
The Senior Radio project is an open-source accessible designed internet radio device with both hardware and software components. It is intended to be used for users familiar with controlling a pocket radio where dials controls volume and the selected radio station. The project is made with a user-centered design, focused on a user who want a similar size and controls as a standard handheld radio.
It was built to satisfy the needs of allowing impaired individuals be able to listen to the thousands of different internet streaming content that is usually only normally available through an internet browser. Many people do not have the ability to use such a device easily, so this design aims to make a familiar interface for a modern technology.
The core functionality is on Github, stored in a python 3.7.3 program called seniorRadio.py. It reads and writes a json file to store the state of the radio, so when you turn it off it will remember the current audio level and station selected. In addition, it reads an online hosted json file that you can change! This allows you to update the radio stations remotely if you are giving this to someone else.
It is currently created to connect to a bluetooth speaker, but could easily be made to play off an onboard speaker as well.
The creator of this device is likely not the user, and needs at least some experience with electronics and basic programming knowledge. Linux experience is also very useful.
To the user, the functionality is simple:
On power up it will play the last station selected over the default audio device. The left dial controls the station, where a few clicks of the dial changes to a new URL. It will roll over after the last or first entry. The right dial controls the audio level. The LED indicates the program is on. And the pushbutton pauses or "turns off" the radio. So once powered on, a user can just hit the button to pause/play, or "turn off/on", the radio and the two dials to control it.
Supplies
Linked on each item is the specific one I used. Cost me about $60 total with plenty of left over supplies!
Basic electronic and linux knowledge - for assembly and debugging
Raspberry Pi Zero W (Zero WH has headers pre-soldered and likely useful)
MicroSD card-8 GB+ and ability o
MicroUSB 5V power adapter - Link also includes microHDMI adapter and microUSB OTG to USB A adapter for setup if you need them
Bluetooth speaker
2 Rotary encoder - same link as above
LED (optional) - bought LED + push button above
Step 1: Setup (headless) Raspberry Pi W
We need to setup the operating system on the raspberry pi that will run the software. None of this is unique to this project. For debugging, I'd recommend setting it up so you can also access it in headless mode. This means you access the pi over your WiFi network through GUI or a SSH terminal and don't need a keyboard, mouse, and monitor to use it every-time. You could do this without needing to connect to a monitor/mouse/keyboard ever if you're more tech-savvy. Some example instructions here. However it is less straight forward, and below is another way to set it up.
This first time you will likely need:
- Raspberry Pi W
- MicroSD to SD card adapter [to write OS onto SD card]
- MicroSD card (~ 8 GB+) [to store OS and our program]
- MicroUSB power (5V)
- MicroUSB to USB A [for mouse/keyboard]
- Mini HDMI to HDMI adapter [for display output]
- Keyboard
- Mouse
- Monitor
First, you'll need to flash the operating system onto the rPi micro SD card.
I used raspian buster, you download this onto a computer with a SD card read/writer and then flash the raspian OS onto the SD card. BalenaEtcher is an easy software to do this
Next put the SD card into the pi slot, connect the monitor, keyboard and power. Proceed through the setup steps that raspian prompts you to, including connecting it to your WiFi network and pairing your bluetooth speaker through the GUI. Good practice would be to update it using the following commands
sudo apt-get update
sudo apt-get upgrade
If you want to include marking your pi headless, we're going to need to grab the pi's ip address first. Through a terminal you can find it through the
ifconfig
command. You then need to enable SSH and VNC over the interfacing options in
sudo raspi-config
After a restart, you can now test accessing the pi over SSH or VNC. SSH is just a terminal interface, and you may find it easier to use the GUI to pair a bluetooth speaker. You can use a program like VNC connect to easily access your pi on a separate computer on your network. You can use a program like putty to SSH into it.
Furthermore, you can setup a VNC cloud account so you can access the device off network if needed
Step 2: Install Senior Radio Software
This can be done from a terminal window of the raspberry pi. First make sure you have git installed
sudo apt-get install git
Depending on which operating system you installed, these steps may be different as you may need to install additional packages, the system will tell you if so. First we need download the senior radio code from github. https://github.com/Bunborn/seniorRadio Ideally, you are making our own repo so you can make changes and easily change the radio stations in the same way as I do. Fork the repo if so
However, you can use the same as mine no problem if you want to host your radio json file in another spot
Then clone down the repo in whatever location you want it to be. The default directory is fine. Get the URL for which github repo you want by hitting the green clone or download button.
Back in the terminal window,
git clone HTTPS_URL_HERE
You should now see an extra directory named seniorRadio when looking using ls. Fantastic!
The code is meant to be self-documenting, taking a look through it would be useful. Especially for debugging purposes and if you are changing the system.
Step 3: Install Additional Software
Before we move on to the circuit and testing it out, we also need to download VLC and the python api for it. You may already have VLC installed. The first two commands will update the pi, and the last two commands below will install VLC and then the python api
sudo apt-get update sudo apt-get upgrade
sudo snap install VLC sudo apt-get install vlc-python
In addition, also install gpiozero, which we will use to interface with the pi's GPIO pins. You may already have it installed depending on your operating system.
sudo apt-get install gpiozero
Step 4: Find Radio Stations Streams
Next, one of the most fun parts! Time to select whatever streams you want.
In my internetStations.json file you can see my stream links, mainly for news and music. But there is a ton of hosted content out there. The key here is to find the direct stream URL address. For example, the BBC news and music stations can be found here on this blog. These direct streams won't have any graphical interface and will automatically play the stream through your browser media player. These are the URLs we need so VLC can read them! You can test any link quickly in your web broswer, or through VLC on your normal computer to assure it will work on the pi.
To find these stations, you can look for the direct stream online if the service hosts it. A quick way could also be to play the stream, right click on the browser and hit "inspect element". Navigate to the network tab and it should indicate the stream 'direct' URL. This youtube video demonstrates this. Refreshing the page with the tab open should help.
Once you have your stations, you want to put them online so your seniorRadio python program can grab them. There are many options to do this, you could use sites like Github, bitbucket, or even sourceforge. The main purpose of hosting this list online is so the user setting it up can change the stream list online remotely.
At the start of the python program there is a variable called url, simply change this to be the URL of your json file with the station URL links.
You can follow the same format as my internetStation.json file. If you wanted to also use github, you could simply fork my seniorRadio repository, and edit your own internetStation.json file and point it to that "raw" URL. You can find that below in the Github GUI once you clicked on the file.
Step 5: Create Circuits
Now we need to setup the circuit. This will change based on your equipment, but below is how I set mine up.
https://pinout.xyz/ for reference on which pins are which. Since we are using the gpiozero library, we are concerned with the BCM pin numbering only. Note that I bought a few extra KY040 rotary encoders - the dials - to test them out as they all don't all have the same accuracy. Each one would have some "bouncing", or simply incorrect output, so I chose the best of the two I had. In the code you will note there are some steps to limit this bouncing as well.
I connected my pushbutton to BCM pin 17 and ground
My LED to a 1k ohm resistor then BCM pin 27 and ground
My KY040 rotary encoders had the + lead to 5V, the GND to ground and SW disconnected. These were connected over a mini breadboard
Station rotary encoder dt pin connected to BCM pin 21
Station rotary encoder clk pin connected to BCM pin 20
Audio level rotary encoder dt pin connected to BCM pin 19
Audio level rotary encoder clk pin connected to BCM pin 16
If you change these pins, just update the number in the python program as well where it sets up the handlers
I used a mini breadboard to make this easier. I used M/M and M/F jumper wires as well to facilitate the interface. I soldered some jumper wire leads onto the pins of the pushbutton/LED I purchased earlier. To not solder, you could get push buttons and LEDs that already have jumper wire leads or would easily connect to one.
Step 6: Test It!
Now, you should have a functioning system! Let's test it out. Navigate to the folder with the python program, likely:
cd seniorRadio python3 seniorRadio.py
Currently, there is a 45 second delay at the start of the program to give it time to find the bluetooth speaker on power up. You can comment out this line if you don't need it during testing. The program may tell you that you need to install additional packages, go ahead if so. May also need to install a newer version of Python.
<p>sudo apt-get install python3.7</p>
Some possible issues even if the program runs:
I hear no sound
Make sure the speaker is on and selected in your raspberry pi. Through the GUI you should be able to pair it and then select it as your audio output device. Furthermore, check your audio drivers. A common issue is you may need to reinstall the pulseaudio package
Audio choppy or cutting in and out
Try moving the device closer to your WiFi router. In addition, make sure the bluetooth speaker is close by. Could also be an audio driver issue
Step 7: Put Into a Permanent Enclosure
Next, putting it in a box or similar device for it to live in permanently. I recommend having one that you can access later still for debugging. I got this small wooden box at a craft store for $2, and linked it on the supplies page. One of my friends recommended a cigar box.
I then cut out holes in the front for the push button and rotary switches, and drilled a hole in the back of the box for the microUSB power cord.
I tested the mount and made sure it worked, and voilà!
Finally, I used super glue to hold the push button. My breadboard had an adhesive on the bottom that I connected to part of the box. I used a small strip of duct tape to secure the back of the pi to the box, so it could be moved later if needed.
Step 8: Setup Program to Run on Startup
Finally, you likely will want this python program to run on startup of the raspberry pi so you don't need to do it manually. There are many ways to do this that you can find online.
Personally, I set it up using the second way on this SparkFun tutorial : https://learn.sparkfun.com/tutorials/how-to-run-a-...
Here, it waits for the system to enter the graphical desktop before running the program. It then is delayed by the python program for 45 seconds to give the system time to reconnect to the bluetooth speaker. If you are using an integrated speaker, you can remove this delay. Note that the bluetooth speaker should be on first! (for the bluetooth solution)
Now you can try powering off and re-powering on your pi! After about 90 seconds your pi should begin playing the radio streams once again. On hitting the pushbutton it will pause/play. Note on these reboots it re-reads that internetStations json file so if you change your stations you need to restart the device for them to work. Congratulations!
If you made this, or made modifications to the project, please let me know!
Github page : https://github.com/Bunborn/seniorRadio
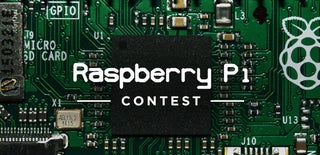
Participated in the
Raspberry Pi Contest 2020