Introduction: Sheepshead Card Game With Esp8266
Sheepshead is a card game my aunts and uncles would play during family get-togethers. It is a trick taking card game originated in Europe. There are several version so my version might be a bit different than what you play. In the version I implemented you can play with 3, 4 or 5 players, 5 being the ideal number of players. The game uses 32 card from a standard 52 card deck.
The basic rules can be found here: https://www.sheepshead.org/rules/sheepshead-basic...
A brief summary of the rules for a 5 player game; each player is dealt 6 cards and two card are dealt to the blind. The player to the dealers left is given the first chance to pick the blind, then the next player etc until someone picks the blind or until all players had a change to pick. If no one picks the game starts over and new cards are dealt. The person who picks the blind is called the picker. The picker chooses a partner by calling a suit, either Hearts, Clubs or Spades but must have a card of the same suit in his/her hand. The player who has the Ace of the called suit is the partner. Players must follow suit of the first card played, trump is a suit. The partner must play the Ace when the called suit is lead for the first time. The picker and partner must get 61 points to win the game. The players who are not the picker or the partner form a team and their points are joined together to beat them. See rules link above for scoring.
For 3 and 4 player game there is no partner and 10 or 8 cards are dealt out respectively.
I did not implement a game of leaster. When no player picks the game could continue by player leaster. The Leaster winner is the player who take at least one trick and scores the fewest number of points.
Game development:
My inspiration for this project came from wanting to create a Sheepshead application and also learn jquery. I also used dragula.js to help with the drag and drop of the cards. The esp8266 is put into AP mode. To connect, you need a WiFi device that can connect to the "Sheepshead" network and go to http://191.168.1.1 web site.
This is my first instructables so I would appreciate any feedback. I will try to answer your questions when I can.
Step 1: Building the Hardware
Hardware Requirements
This can be made with a Wemos D1 Mini or with a esp8266 - esp-07 or esp-12.
Esp8266 build:
- esp8266 (esp-07 or esp-12)
- white mounting board for esp8266 + connectors
- 6-pin female connector (optional)
- LM317 voltage regulator
- (2) 10K Ohm resistor
- 390 Ohm Resistor
- 220 Ohm Resistor
- 20 Ohm Resistor
- (2) momentary push buttons
- 100n ceramic capacitor
- 10uF electrolytic capacitor
- 220uF electrolytic capacitor
- 1N4002 diode (optional)
- barrel connector (I used a side mount and soldered to a 3 pin male connector)
- Project box
- Power supply
I included the ExpressSCH and ExpressPCB files I used to build the circuit. As you can see from the picture I did not add the buttons or use the J2 connector(which can be used with FTDI232RL module for programing the esp8266). If you want to use this as an esp8266 programmer you will need the buttons and the J2 connector. To flash software or data you will need to reset the device (SW1) keep it press while pressing the (SW2) button, then releasing the (SW1) button then upload the code.
Before placing any components verify the circuit by testing each connection. Verify the voltage regulator is outputting 3.3 volts and double check all connections. If you are not sure then I recommend using a Wemos D1 mini as no soldering is required.
Step 2: Loading the Software
Software
I included the software for this project. I will give a brief explanation of each class and what it is used for but I'm not going to go into details. This game is a proof of concept that a card game can be made on an esp8266. The game has a few issues that I did not work out yet. For example when a user logins in and losses connection they are not released from the game and cannot reconnect to the game. The only way around this is to reset the game and start over. The game is a bit slow and sometimes does not refresh the cards. The user can refresh the browser which should display the cards correctly. If the user saves the link on their home screen (for iPhone's) then the refresh button is not available making it impossible to refresh. Sometimes it is hard to drag and drop cards to/from the blind on small devices.
Card.h and Card.ino Card class
This class holds the card suit, rank, point and which cards are trump.
Deck.h and Deck.ino Deck class
This class holdd the card deck information. It has method to shuffle the deck and to get cards from the deck. It uses a random seed to shuffle the deck
Hand.h and Hand.ino
This class implements the IGame interface and holds information on each players Hand. Who the Picker, Partners and Dealer are. It hold who's turn it is, what is the called suit, what is th e lead suit, what hand number, the number of players, who the winner is, etc.
Player.h and Player.ino Player class
This class holds Player information, like player name, team points, if the player picked or passed and displays message to the player.
IGame.h and IGame.ino IGame interface class.
This class holds the Deck, Card, and Player classes to control the game flow.
Sheepshead.ino
Holds the setup and loop function to serve up the web site.
login.html and login.js files
These files control the flow for user login web site page
sheepshead.html and sheepshead.js files
These files control the flow of the web site sheepshead game.
Card images
holds the image of each card including the back of the card.
Here is a brief explanation loading software onto the esp8266. There are several examples on the web that can explain it in more detail. This is how I loaded the software and data using the Arduino IDE software.
- Install the Arduino software and the esp8266 boards files, see https://github.com/esp8266/Arduino for more information.
- If using an Wemos D1 mini, set the Flash size to 4M (1M SPIFFS). If use a generic esp8266 esp-07 or esp-12, set the Flash size to 1M (512 SPIFFS).
- You may need to install some additional libraries into the Arduino software.
- To install the SPIFF data follow this link https://github.com/esp8266/arduino-esp8266fs-plug...
- unzip the Sheepshead software and place onto your computer.
- Start the Arduino IDE software and open the Sheepshead project
- Install the Sheepshead code on to the esp8266 device by clicking the upload button. If you are not using the Wemos D1 Mini you may need to put the device in flash mode by clicking the reset button (SW1) keep it pressed while pressing the (SW2) button, then releasing the (SW1) button then upload the code.
- Install the data on to the esp8266 device by going to tools on the menu and clicking "ESP8266 Sketch Data Upload". You will need to follow the same steps as above to put the device in flash mode.
- Once the software and data are loaded you are ready to play the game.
Attachments
Step 3: Playing the Game
To connect you need a WiFi device that can connect to the "Sheepshead" network then go to http://191.168.1.1 web site.
- Players join by entering their name and clicking Join. The game assumes that you will have 5 players, if not a player must select the number of players before the last player joins the game.
- Once the last player joins the cards are dealt out and game starts when the player clicks the Start button.
- The game will not let a player play an invalid card or play out of turn.
- When a card is clicked it will increase in size to be more visible. If clicked a second time the card will be played.
- The players name is highlighted with white letters.
- The player who is to play next will have a red box surrounding their name and card.
- The game starts by allowing a player to pick the blind or pass. When a player picks the blind they can drag and drop the cards from the blind to/from there hand. Once the picker has the cards they want they must pick a called suit from the drop down.
- The game will validate that they have an appropriate card for the called suit.
- Play starts when the first player plays the first card, each player plays one card and the winner for the hand is displayed. The points are calculated and displayed for each player/team.
- The winner of the hand plays the first card and each player plays one card.
- Play continues until all cards are played
- A winner is determined.
- The next person becomes the dealer and a new game begins.
Step 4: Conclusion
This is my first Instructable and I welcome your feedback. As I said this is a proof on concept that a card game can be made on an esp8266 device. It has a few issues but is playable. I look forward to your comments and suggestions.
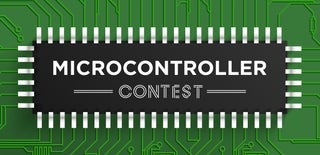
Participated in the
Microcontroller Contest