Introduction: Simple Arduino Photocell Circuit and Data Logging
For a while now I've been attempting to get data from my Arduino + Linksprite WiFi shield into my Adafruit IO dashboard. My first few attempts were admittedly way more advanced than what I should have started with (a weather station, I'm still working on it and will make an Instructable on it once I get it working).
I have since learned the error of my ways and decided to go back to basics. And what's more basic than data logging photocell values. It's a simple set up and coding it wasn't as complicated as I thought it would be. Also, my bread board was completely tied up with another project so I had to figure out a way to make this happen without dismantling the work I had already done for another project (not the weather station, a solar tracker. I may have too much on my plate and not enough resources...).
Therefore the idea for the SIMPLE PHOTOCELL ARDUINO CIRCUIT was born!
As you can see from the pictures above, I simply soldered a 10k resistor to one leg of a photocell and bent it in a way that allows it to be plugged correctly into the Arduino, bypassing the need for a bread board. I did notice that occasionally a contact would be loose and the reading in the serial monitor would stick at 1024 but if you just jiggle the contraption a round slightly to make sure that all the contact points are touching correctly would fix that issue.
Step 1: Parts List
- 10k resistor (brown, black, orange, gold) (I recommend buying in assorted bulk, because you never know, but the link is just to 10k's on Amazon)
- photocell - You'll only need one but if you plan on buying online expect 10-20. You may just want to run to Radio Shack or Fry's Electronics and pick one up.
- Arduino board - I have/used an Uno R3 but I'm sure you could use any Arduino compatible board.
- WiFi Shield - I have a Linksprite CC3000 that I bought from Amazon a while back. They don't appear to sell that particular one anymore but there are others, that are even less expensive than what I paid for mine, bummer. You may also want to look at the Adafruit HUZZAH ESP8266 Breakout. I'm pretty sure this is what I'm going to start buying from now on.
- USB cable - To connect the Arduino to your computer.
Step 2: Putting the Hardware Together
So, it's really simple. Solder one leg of the resistor to one leg of the photocell. Are you decent at soldering? Then you're almost done. After it cools bend the resistor so that the unattached end can reach the ground pin on the board. I had to bend mine back toward the other leg of the photocell since 5V and Ground are next to each other. Be careful that when you bend it you don't let it touch the other leg (A0) of the photocell and short it out (well, not short it out, but you won't get any readings and you'll be scratching your head wondering why).
To connect it to the board, the un-soldered leg of the photocell goes into the 5V pin, the un-soldered leg of the resistor goes into the Ground pin and the other leg of the photocell (the soldered one) goes into pin A0.
You're halfway there champ!
Step 3: Setting Up the Software - Adafruit IO
You will need an account on https://io.adafruit.com. Once you have it you will see a default dashboard with some dashboard blocks already set up for you. Click on the MY DASHBOARDS link on the left to get to the main screen. From here you'll want to click on MY FEEDS and create a new feed called photocell. Then click on the MY FEEDS link on the left to get back to the main page again. Then click on MY DASHBOARDS and click CREATE DASHBOARD, name it photocell (just keep it simple to start). Click on your photocell dashboard and then click on the blue square with the white +. You are going to create a gauge block. After you click Create on the gauge block click on Next Step to open up the feeds. Click CHOOSE on the photocell feed then click NEXT STEP. On the next section change the Gauge Max Value to 1024 and finish by clicking CREATE BLOCK. That will put the gauge block on your photocell dash board. Phew! Time to code the Arduino!
Step 4: Setting Up the Software - Arduino With WiFi Shield
This step may or may not apply to you, it really depends on what hardware you have. The Arduino board or clone should accept any Arduino code you program it with but the WiFi Shield you have may not work with the code I'm about to show you. I have a Linksprite CC3000. Most CC3000's are coded the same way, you just have to find the libraries that work with it and adjust things within the main code to make it work. With the Linksprite I found that the Adafruit CC3000 library works perfect with my shield and it just so happens that Adafruit IO is the same company.
TL;DR: Your WiFi shield may have to be coded differently and may require more effort on your part.
Here's the code I used:
#include "Adafruit_SleepyDog.h" #include "Adafruit_CC3000.h" #include "SPI.h" #include "utility/debug.h" #include "Adafruit_MQTT.h" #include "Adafruit_MQTT_CC3000.h"</p><p>int photocellPin = 0; // the cell and 10K pulldown are connected to a0</p><p>/*************************** CC3000 Pins ***********************************/</p><p>#define ADAFRUIT_CC3000_IRQ 3 // MUST be an interrupt pin! #define ADAFRUIT_CC3000_VBAT 5 // VBAT & CS can be any digital pins. #define ADAFRUIT_CC3000_CS 10 // Use hardware SPI for the remaining pins // On an UNO, SCK = 13, MISO = 12, and MOSI = 11</p><p>/************************* WiFi Access Point *********************************/</p><p>#define WLAN_SSID "---YOUR WIFI SSID (NAME)---" // can't be longer than 32 characters! #define WLAN_PASS "---YOUR WIFI PASSWORD---" #define WLAN_SECURITY WLAN_SEC_WPA2 // Can be: WLAN_SEC_UNSEC, WLAN_SEC_WEP, // WLAN_SEC_WPA or WLAN_SEC_WPA2</p><p>/************************* Adafruit.io Setup *********************************/</p><p>#define AIO_SERVER "io.adafruit.com" #define AIO_SERVERPORT 1883 #define AIO_USERNAME "---YOUR ADAFRUIT USERNAME---" #define AIO_KEY "YOUR DASHBOARDS ADAFRUIT KEY" // it's the yellow square with the key icon on your dashboard</p><p>/************ Global State (you don't need to change this!) ******************</p><p>/ Setup the main CC3000 class, just like a normal CC3000 sketch. Adafruit_CC3000 cc3000 = Adafruit_CC3000(ADAFRUIT_CC3000_CS, ADAFRUIT_CC3000_IRQ, ADAFRUIT_CC3000_VBAT);</p><p>// Store the MQTT server, username, and password in flash memory. // This is required for using the Adafruit MQTT library. const char MQTT_SERVER[] PROGMEM = AIO_SERVER; const char MQTT_USERNAME[] PROGMEM = AIO_USERNAME; const char MQTT_PASSWORD[] PROGMEM = AIO_KEY;</p><p>// Setup the CC3000 MQTT class by passing in the CC3000 class and MQTT server and login details. Adafruit_MQTT_CC3000 mqtt(&cc3000, MQTT_SERVER, AIO_SERVERPORT, MQTT_USERNAME, MQTT_PASSWORD);</p><p>// You don't need to change anything below this line! #define halt(s) { Serial.println(F( s )); while(1); }</p><p>// CC3000connect is a helper function that sets up the CC3000 and connects to // the WiFi network. See the cc3000helper.cpp tab above for the source! boolean CC3000connect(const char* wlan_ssid, const char* wlan_pass, uint8_t wlan_security);</p><p>/****************************** Feeds ***************************************</p><p>/ Setup a feed called 'photocell' for publishing. // Notice MQTT paths for AIO follow the form: <username>/feeds/<feedname> const char PHOTOCELL_FEED[] PROGMEM = AIO_USERNAME "/feeds/photocell"; Adafruit_MQTT_Publish photocell = Adafruit_MQTT_Publish(&mqtt, PHOTOCELL_FEED);</feedname></username></p><p>/*************************** Sketch Code ************************************/</p><p>void setup() { Serial.begin(115200);</p><p> Serial.println(F("Adafruit MQTT demo"));</p><p> Serial.print(F("Free RAM: ")); Serial.println(getFreeRam(), DEC);</p><p> // Initialise the CC3000 module Serial.print(F("\nInit the CC3000...")); if (!cc3000.begin()) halt("Failed");</p><p> mqtt.subscribe(&onoffbutton);</p><p> while (! CC3000connect(WLAN_SSID, WLAN_PASS, WLAN_SECURITY)) { Serial.println(F("Retrying WiFi")); delay(1000); } } uint32_t x = analogRead(photocellPin); // This is important! This part took me forever to figure out.</p><p>// You NEED the "uint32_t x =" part if you plan on trying to add a different sensor, then just follow "x" through </p><p>// the rest of the code</p><p>void loop() { x = analogRead(photocellPin); // Make sure to reset watchdog every loop iteration! Watchdog.reset();</p><p> // Ensure the connection to the MQTT server is alive (this will make the first // connection and automatically reconnect when disconnected). See the MQTT_connect // function definition further below. MQTT_connect();</p><p> // this is our 'wait for incoming subscription packets' busy subloop Adafruit_MQTT_Subscribe *subscription; while ((subscription = mqtt.readSubscription(1000))) { if (subscription == &onoffbutton) { Serial.print(F("Got: ")); Serial.println((char *)onoffbutton.lastread); } }</p><p> // Now we can publish stuff! Serial.print(F("\nSending photocell val ")); Serial.print(x); Serial.print("..."); if (! photocell.publish(x)) { Serial.println(F("Failed")); } else { Serial.println(F("OK!")); }</p><p> // ping the server to keep the mqtt connection alive if(! mqtt.ping()) { Serial.println(F("MQTT Ping failed.")); }</p><p>}</p><p>// Function to connect and reconnect as necessary to the MQTT server. // Should be called in the loop function and it will take care if connecting. void MQTT_connect() { int8_t ret;</p><p> // Stop if already connected. if (mqtt.connected()) { return; }</p><p> Serial.print("Connecting to MQTT... ");</p><p> while ((ret = mqtt.connect()) != 0) { // connect will return 0 for connected Serial.println(mqtt.connectErrorString(ret)); if (ret < 0) CC3000connect(WLAN_SSID, WLAN_PASS, WLAN_SECURITY); // y0w, lets connect to wifi again Serial.println("Retrying MQTT connection in 5 seconds..."); mqtt.disconnect(); delay(5000); // wait 5 seconds } Serial.println("MQTT Connected!"); }
Step 5: That's It
Like I said before, you may have to fiddle with the code to get it to work for you.
Now go forth and create!
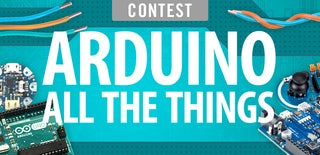
Participated in the
Arduino All The Things! Contest
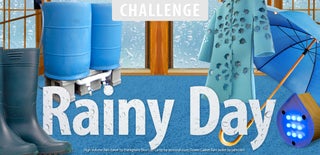
Participated in the
Rainy Day Challenge
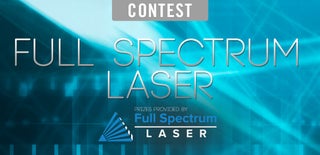
Participated in the
Full Spectrum Laser Contest 2016
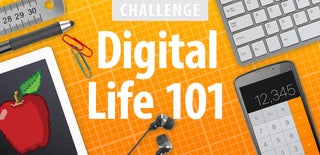
Participated in the
Digital Life 101 Challenge