Introduction: Simple Arduino Robot Arm
With Robot arms you can gather a lot of experience with DC motors, stepper and controlling robots with Arduino or Raspberry PI controller.
The main aim of this Instructables is to show how to control motors with the potentiometer, and the joystick and how to apply this knowledge to the robotic arm. At the end gained experience can be transferred to other robo - projects.
For examples I will use OWI robotic arm, because it’s not too expensive (around 40 Euro) and easy to handle. For beginners, it’s a good start. For learning purpose - I will try to keep the code as simple as possible.
All I can say is, let's rock.
Step 1: Basics - L298N
Driving two DC motors with L298N
In many robotic projects you will use DC motors and stepper. In this step we will connect two DC motors to the L298N H-Bridge motor driver and we will use a small program to make them turn.
We’ll learn how to control the speed and direction of the motors.
We will use a brushed DC motors which is cheap and widely used in the maker projects.
NOTE: Nice future of the brushed DC motors is that they can be used as generators. When you will rotate rotor electricity will be induced in coils, and current will flow in the electrical circuit. We are using this future to power LED lights from a small wind turbine. It is a cheaper way to create your own generator.
ATTANCION: Same effect can cause some problems in your electrical circuit; when a DC motor spins, energy is built up and stored in the inductance of the motor coils and when DC motor stops or manually turned on - it will create current in the opposite direction. For this reason you mast use a diode in your circuit to ensure that the motor cannot send current back when it is forcibly turned or brakes.
Using DC motors
Small motors are used for many applications, from small fans to robot cars.
Consider following parameters during motor selection:
- The operating voltage - this can vary, from 3 V to more than 12 V.
- The current without a load - the amount of current the motor uses at its operating voltage while spinning freely, without anything connected to the motor’s shaft.
- The stall current - the amount of current used by the motor when it is trying to turn, but cannot because of the load on the motor.
- The speed at the operating voltage - the motor’s speed in revolutions per minute (RPM).
WARNING We will use a 9V battery to power motors. If battery is improperly connected it can damage your electronics. To avoid some mistakes follow the schematics carefully. Avoid short circuits (connecting power directly to ground), and while you’ll be sharing the ground line between power supplies, don’t try to connect two separate voltage sources to each other - do not connect the 9V supply and the Arduino’s 5V supply into the same supply row on the breadboard.
The L298N can handle up to 3 amperes at 35 Volts DC,which is suitable for most hobby motors.
Required Components:
- Arduino UNO
- L298N Driver
- 2 DC Motors
- Jumper Wires
- 6-12V Supply
The L298N controller board, shown in the picture (Variant 1), has +12V and +5V terminals. The +12V pin is where the motor power is attached. This pin can accept voltages from +7VDC to +35VDC.
12V DC Motor Supply (recommended):
- Connect the 12V DC motor power supply to the H-Bridge module.
- Enable the onboard 5V DC regulator by shorting the jumper pin.
- Connect 12V DC motors to the H-Bridge module.
- You can power your Arduino from the onboard 5V DC regulator.
NOTE: remove the +12V jumper shown if you are using powers higher or lower than +12V.
If you are using DC motors with 3-6 Voltage rating then you should use connection shown in the picture (Variant 2).
6 V DC Motor Supply:
- Connect 6V DC motor power supply to the H-Bridge module.
- Disable the onboard 5V DC regulator by opening the jumper pin.
- Connect 3V-6V DC motors to the H-Bridge module.
- Use a separate power supply for your Arduino, and common the power ground rails.
- Connect a 6V DC power source to the H-Bridge driver module
1
Attachments
Step 2: L298N With 2 DC Motors - Sketch
First we will use 'define to create fixed variables for our connected pins.
# define - before void setup(), we use #define statements to create fixed variables. #define is a useful C++ component that allows the programmer to give a name to a constant value before the program is compiled. Defined constants in Arduino don’t take up any program memory space on the chip. The compiler will replace references to these constants with the defined value at compile time.
https://www.arduino.cc/reference/en/language/struc...
Or you can assign pins using const var.
const int EnA=10; //Half Bridge 1 Enable
const int In1=3; //Motor Control 1
const int In2=2; //Motor Control 2
pinMode(pin, mode) - Configures the specified pin to behave either as an input or an output. We will first define a I/O pins as an output in void setup() using the following function:
pinMode(In1,OUTPUT); // set control pin 1 as OUTPUT
To eliminate some confusion - all pins have the same names as in L298N Motor Controller Board.
But its a not a dogma you can assign your own variable names which is most suit you.
Next, to perform some motor action, we use digitalWrite(pin, value) to write a HIGH or a LOW value to a digital pin. To move Motor 1 forward we use following command:
digitalWrite(In1, HIGH);
digitalWrite(In2, LOW);
And to reverse Motor 1 we just change pins values:
digitalWrite(In1, LOW);
digitalWrite(In2, HIGH);
To reverse the direction in which the DC motor rotates you simply reverse the polarity of the DC current that you apply to it. Changing the speed however is a different story. It can be done with help of Pulse Width Modulation (PWM). With PWM the motor is sent a series of pulses. The width of the pulses are varied to control the motor speed, pulses with a narrow width will cause the motor to spin quite slowly. Increasing the pulse width will increase the speed of the motor.
Arduino analogWrite() function writes an analog value (PWM wave from 0 to 255) to a pin:
analogWrite(EnA, 180); // speed up Motor 1 up to 70% of nominal speed.
To stop motors we just assign LOW values for all In-Pins.
We can use this Sketch as a template for our next experiments with Potentiometer and Joystick.
Attachments
Step 3: Control Motors With Potentiometer
In this Step we will add two potentiometers to our arrangement, and add two sketches:
- First one will show us how to to control motor speed with potentiometers;
- Second, the more advance sketch will be used to change motors speed and direction.
Required Components:
- Arduino UNO
- L298N Driver
- 2 DC Motors
- 2 10 KOhnm Potentiometers
- Jumper Wires
- 6-12V Power Supply
Connect components according to the wiring diagram.
In the first sketch we have defined two potentiometers and add two MotorSpeed variable to store analog values.
Then in new motorSpeed() function we have added:
MotorSpeed = analogRead (Pot); // read the analog value from the potentiometer
After that we had to convert our Analog input values which are in a range between 0-1024 to the PWM values in a range 0-255. For this step we are using map () function - which converts a value from one range to another. The map () function takes no less than five parameters:
map (value, fromLow, fromHigh, toLow, toHigh)
and returns us another value. The map() function parameters' meanings are as follows:
- value: This is the value we already have and want to map into a new range
- fromLow: This is the lower limit of the possible input range
- fromHigh: This is the upper limit of the possible input range
- toLow: This is the lower limit of the possible output range
- toHigh: This is the upper limit of the possible output range
MotorSpeed1 = map(MotorSpeed1, 0, 1023, 0, 255); // Convert to range of 0-255 with help of map() function
At last we are setting motors, speed with analogWrite () functional.
analogWrite(EnA, MotorSpeed1);
analogWrite(EnB, MotorSpeed2);
In the second sketch we have made some small adjustments:
- We have created separate brake() function - in order to save extra typing, because when potentiometer shaft in the middle then motor will stops.
- Then in the if () - else if () loop, we check potentiometer values and accordingly change motors directions and speed.
When the potentiometer value lay between 450-500 units (the midpoint), the brake() function will be called. As the potentiometer value increases from 500 to 1023, the speed forward increases. Similarly, the speed increases in the reverse direction when potentiometer values go from 462 to 0.
Now you have create project which can be applied to drive robot car, and in the next step we will manipulate OWI robot arm with for DC motors.
Step 4: Control OWI Robotic Arm
You can expand the previous project by adding additional L298N motor control board and two potentiometers.
Sketch will not change drastically - we must add additional two motors in our if() - if else() loop. At the end you should be able to control your robotic arm with four potentiometers.
NOTE: OWI robotic arm is not a good solution for your experiments because it has simple 3V DC motors, and when you switch on they will move very fast. You should be very careful by controlling it with poty, and usually it requires some additional potentiometers mounted on the robot arm joints - in order to make close loop.
Additionally, you can use the Arduino motor shield to control four DC motors.
But our main aim in this Instructables is to learn how to control different types of robots (robot car, arm etc.) with the help of the potentiometers and joysticks, and with a code which uses basic Arduino IDE functions(without extra libraries).
Step 5: Control Two Motors With With Joistick
Short introduction to the Joysticks
Joystick is a simple device which communicates motion in 2D (X and Y-axes) to the controller. It consists two independent 10K potentiometers (one per axis); it is two boxes on the sides of the joystick. It has also a small push button which is identified as Switch - the switch sends a low (or ground) when the joystick knob is pressed. At the end you can compare Joystick with two potentiometers, which will significantly simplify our programming task - we can use the same methods as in previous examples.
Reading analog values from Joystick
In order to read the joystick’s physical position, we need to measure the change in resistance of a potentiometer. It can be done with Arduino analog pins using Analog to Digital Converter (ADC). As the Arduino board has an ADC resolution of 10 bits, the values on each analog channel (axis) can vary from 0 to 1023. So, if the stick is moved on X axis from one end to the other, the X values will change from 0 to 1023 and a similar thing happens when moved along the Y axis. When the joystick stays in its center position the value is around 512. In the picture you can see how the analog values from potentiometers are distributed between X and Y axes. In this step we will change speed and direction of the 2 DC motors with the help of a joystick.
Required Components:
- Arduino UNO
- L298N Driver
- 2 DC Motors
- Joystick
- Jumper Wires
- 6-12V Power Supply
Connect components according to the wiring diagram.
The joystick board has 5 terminals. GND, +5V, VRX, VRY and SW where:
- GND and +5V are power supply pins - 5 V and GND on the Arduino board
- VRX is the X-axis potentiometer output - Analog input A0
- VRY is the Y-axis potentiometer output - Analog input A1
- SW is the push button terminal - Digital input pin 4
Motors connection and L298N is the same as in the previous step.
Code explained
The sketch starts by initializing connections of Joystick module to the Arduino.
#define VRX A0 // Joystick X Position a Pin A0
#define VRY A1 // Joystick X Position a Pin A1
In setup() function: We initialize the VRX and VRY pins (X and Y axes potentiometer of the joystick) as an INPUT.
pinMode(VRX, INPUT);
pinMode(VRY, INPUT);
And same as in the previous example in a loop () function, we we read analog values of the VRX and VRY using analoglRead() function.
xPosition = analogRead(VRX); //Reading the horizontal movement value (X-direction)
yPosition = analogRead(VRY); //Reading the vertical movement value (Y-direction)
Same as in previous example we are using if() - else if() loop to make comparison between measured analog values from joystick, and accordingly perform motor actions.
That's it - now we can control motors with joystick.
Step 6: Control a Robot Arm With Joysticks
As shown in the circuit diagram, we need only four Arduino terminal pins: A0 -A4 in order to send analog values from joysticks, and at least four PWM pins PWM signal outputs which allow us to control the direction of rotation as well as the speed by varying the duty cycle of the active PWM signal. The active PWM pin decides the motor direction of rotation (one at a time, the other output is logic 0). Other Pins you can connect to any available digital pin.
In Sketch - we can use the advantage of the #define - we just need to change pin connection number, and it will be automatically changed in the program.
In general sketch is doesn't change too much we just added two additional motors and used if() - if else() loop to control them.
In next instructables we will learn how to assemble robot arm with servo motors and control it with joysticks
Step 7: Conclusion
Robots can be used in many ways - as manipulators for some routine tasks, self-driving platforms, and most importantly, you can transfer your robotic experience to some other projects like house automation, greenhouse or garden automation, etc. The hardware and software used in your automation projects are almost the same (sensors, motors and servos, etc.) as in your robot.
It depends on your imagination and creativity how you will use this knowledge - to build big robots or precise manipulators which will improve your workflow, or create some IoT projects.
Doesn’t matter what type of the projects you will start just having fun and let your imagination run wild!
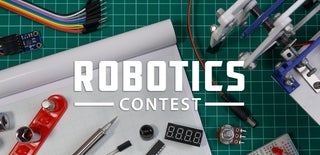
Participated in the
Robotics Contest