Introduction: Smart Switch Having 6 Outputs & 5 Inputs
Hello Makers,
I have made this smart switch using an ESP8266 and Raspberry Pi, which has the capacity of controlling 6 electrical devices.
Introduction video :-
Hardware: ESP8266, Raspberry PI3B, SSR relay board, optocoupler, MINI SMPS
Software: Mosquitto MQTT broker, Node-RED, Arduino IDE
Library: PubSub client (Arduino), node-red-contrib-alexa-home-skil
All devices/relays can operate using conventional switch gives me freedom to install it in my existing switch board without changing wiring.
All devices/relays can controlled via web page.
All devices/relays can controlled via Android application (MQTT-Dash).
All devices/relays can controlled via voice command.
Raspberry Pi used as MQTT broker and Node-RED used for to make dashboard & provide Alexa connectivity.
All devices/relays can also control using "OK Google" using tasker but currently some part of node red is not ready so I didn't mentioned here.
I have made this smart switch using an ESP8266 and Raspberry Pi, which has the capacity of controlling 6 electrical devices.
Introduction video :-
Hardware: ESP8266, Raspberry PI3B, SSR relay board, optocoupler, MINI SMPS
Software: Mosquitto MQTT broker, Node-RED, Arduino IDE
Library: PubSub client (Arduino), node-red-contrib-alexa-home-skil
All devices/relays can operate using conventional switch gives me freedom to install it in my existing switch board without changing wiring.
All devices/relays can controlled via web page.
All devices/relays can controlled via Android application (MQTT-Dash).
All devices/relays can controlled via voice command.
Raspberry Pi used as MQTT broker and Node-RED used for to make dashboard & provide Alexa connectivity.
All devices/relays can also control using "OK Google" using tasker but currently some part of node red is not ready so I didn't mentioned here.
Step 1: Making of Custom Made PCB Using Esp8266
I have used Esp8266 as MCU. It has 11 usable io pins. From which I used 6 pins as output & 5 pins as inputs.
Remark fo D8 pin:-
Pin D8 required to be pulled low during esp bootup. So i have used it as output. But if I directly connect it with relay board then it creates problem, because connecting relay board to Gpio pin pulls that pin high & in case of Gpio D8 esp will not boot.
So I used pc817 optocoupler to resolve this problem.
Remark for Rx & TX pin :- until all testing not competed I had commented both this pin in code as this pins are used for fleshing & debugging of esp.
After final resting I removed commenting "//" so Rx & TX pin started working as output.(as per code )
For powering up esp I used Easy power make minismps. Having 230vac to 5 V dc 3 Watt. (600 ma).
For relay board connection I provided 8 pin female header. One VCC one pin for ground & remaining 6 for relay switching.
For all 5 input I provided pulldown resistor & provide 6 pin female header.
One Vcc pin & 5 for inputs.
Remark fo D8 pin:-
Pin D8 required to be pulled low during esp bootup. So i have used it as output. But if I directly connect it with relay board then it creates problem, because connecting relay board to Gpio pin pulls that pin high & in case of Gpio D8 esp will not boot.
So I used pc817 optocoupler to resolve this problem.
Remark for Rx & TX pin :- until all testing not competed I had commented both this pin in code as this pins are used for fleshing & debugging of esp.
After final resting I removed commenting "//" so Rx & TX pin started working as output.(as per code )
For powering up esp I used Easy power make minismps. Having 230vac to 5 V dc 3 Watt. (600 ma).
For relay board connection I provided 8 pin female header. One VCC one pin for ground & remaining 6 for relay switching.
For all 5 input I provided pulldown resistor & provide 6 pin female header.
One Vcc pin & 5 for inputs.
Step 2: Connecting Relay Board
I have used 8 channel SSR relay board for switching my applieances. As shown in image I made belt using male female header & connect esp board with relay board.
As I have used only 6 Outputs 2 relay are spare.
As I have used only 6 Outputs 2 relay are spare.
Step 3: Connecting Esp Board With Relay Board.
After making esp board I had commectd both board using custom made male female header cable. & Attached both boards using hot glue.
As shown in attached image.
As shown in attached image.
Step 4: Raspberry Pi Setup. Mosquito & Node Red
I flesh 32 GB SD card with raspbian is & indertedi Raspberry Pi 3B.
As node Red & mosquito is inbuilt in raspbian I just boot RPI & update & Upgrade it for to get all updates.
I had prepared nodered sketch which I attached.
Main hart of this project is mosquito Mqtt broker.
As it is inbuilt on raspbian I didn't have to do anything else.
I have used mqtt sub/pub tool to read & write messages of esp8266.
I used nodered Alexa contrib library written by Ben Hardill. Using which I integrated Alexa for voice command.
For making Dash board I used nodered dashboard tool.
As node Red & mosquito is inbuilt in raspbian I just boot RPI & update & Upgrade it for to get all updates.
I had prepared nodered sketch which I attached.
Main hart of this project is mosquito Mqtt broker.
As it is inbuilt on raspbian I didn't have to do anything else.
I have used mqtt sub/pub tool to read & write messages of esp8266.
I used nodered Alexa contrib library written by Ben Hardill. Using which I integrated Alexa for voice command.
For making Dash board I used nodered dashboard tool.
Step 5: Arduino Code for Esp8266
In Arduino ide I wrote sketch using mosquito pubsub library written by "Nick O'Leary" guy who also wrote nodered.
Esp will connect local network on which my raspberry Pi & Alexa connected.
Esp subscribe topics & published on topics as mentioned in Arduino code.
If any input pin state change fore more than 20 Milli second then corresponding output toggles & esp published new state of output.
+++++ Arduino code++++++
#include
#include
// Update these with values suitable for your network.
const char* ssid = "Your network id";
const char* password = "your password";
const char* mqtt_server = "192.168.1.165"; //IP of your mqtt server(Raspberry PI)
//Output defination
byte night_lamp = 16 ; // D0
byte front_light = 0 ; // D3
byte rear_light = 2 ; // D4
byte fan = 15 ; // D8
byte socket1 = 3 ; // RX
byte socket2 = 1 ; // TX
//Input defination
byte night_lamp_in = 5 ; // D1
byte front_light_in = 4 ; // D2
byte rear_light_in = 14 ; // D5
byte socket_in = 12 ; // D6
byte fan_in = 13 ; // D7
//Input Bit status by reading input pin status
byte night_lamp_sts;
byte front_light_sts;
byte rear_light_sts;
byte fan_sts;
byte socket_sts;
//Input debounse delay to avoide unwanted switching
unsigned long night_lamp_sts_dly;
unsigned long front_light_sts_dly;
unsigned long rear_light_sts_dly;
unsigned long fan_sts_dly;
unsigned long socket_sts_dly;
//Output Setpoint
int night_lamp_stpt;
int front_light_stpt;
int rear_light_stpt;
int fan_stpt;
int socket1_stpt;
int socket2_stpt;
WiFiClient espClient;
PubSubClient client(espClient);
char msg[50]; // to storing MQTT message
void setup() {
//Output Setup
pinMode ( night_lamp , OUTPUT );
pinMode ( front_light , OUTPUT );
pinMode ( rear_light , OUTPUT );
pinMode ( fan , OUTPUT );
pinMode ( socket1 , OUTPUT );
pinMode ( socket2 , OUTPUT );
//Output initialised
digitalWrite ( night_lamp , HIGH );
digitalWrite ( front_light , HIGH );
digitalWrite ( rear_light , HIGH );
digitalWrite ( fan , HIGH );
digitalWrite ( socket1 ,HIGH );
digitalWrite ( socket2 ,HIGH );
//Input Setup
pinMode ( night_lamp_in , INPUT );
pinMode ( front_light_in , INPUT );
pinMode ( rear_light_in , INPUT );
pinMode ( socket_in , INPUT );
pinMode ( fan_in , INPUT );
//Printing initial input status **** do not connect rx & tx pin to relay during debugging ***
/*
Serial.begin(115200);
Serial.println();
Serial.print ("night_lamp_sts "); Serial.println ( night_lamp_sts );
Serial.print ("front_light_sts "); Serial.println ( front_light_sts );
Serial.print ("rear light_sts "); Serial.println ( rear_light_sts );
Serial.print ("fan_sts "); Serial.println ( fan_sts );
Serial.print ("socket_sts "); Serial.println ( socket_sts );
*/
//Setup WiFI
setup_wifi();
client.setServer(mqtt_server, 1883);
client.setCallback(callback);
night_lamp_sts_dly = millis();
front_light_sts_dly = millis();
rear_light_sts_dly = millis();
fan_sts_dly = millis();
socket_sts_dly = millis();
//Initialised input status bit
night_lamp_sts = digitalRead(night_lamp_in);
front_light_sts = digitalRead(front_light_in);
rear_light_sts = digitalRead(rear_light_in);
fan_sts = digitalRead(fan_in);
socket_sts = !digitalRead(socket_sts);
}
// XXXXXXXXXX uncomment Serial.print statement for debugging purpose.
void setup_wifi() {
delay(10);
// We start by connecting to a WiFi network
//Serial.println();
//Serial.print("Connecting to ");
//Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
//Serial.print(".");
}
//Serial.println("");
//Serial.println("WiFi connected");
//Serial.println("IP address: ");
//Serial.println(WiFi.localIP());
}
void callback(char* topic, byte* payload, unsigned int length) {
String payloadval_str;
int payloadval_int, payload_pwm;
for (int i = 0; i < length; i++) payloadval_str += (char)payload[i];
payloadval_int = payloadval_str.toInt();
payload_pwm = map(payloadval_int, 100, 0, 0, 1024);
recieved_cmd(topic, payloadval_int, payload_pwm);
//Serial.println("Message Recieved");Serial.print(topic);Serial.print(" ");Serial.println(payloadval_int);
}
void reconnect() {
// Loop until we're reconnected
if (!client.connected()) {
//Serial.print("Attempting MQTT connection...");
// Attempt to connect
if (client.connect("ESP8266Client")) {
//Serial.println("connected");
// Once connected, publish an announcement...
client.publish("ashish/bed1/sw1/", "SB1 is Connected");
// ... and resubscribe
client.subscribe("ashish/bed1/sw1/cmd/#");
} else {
//Serial.print("failed, rc=");
//Serial.print(client.state());
//Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
}
}
//Serial.println("Pub start");
snprintf (msg, 1, "0");
client.publish("ashish/bed1/sw1/initialised", msg);
//Serial.print(msg);
}
void loop() {
if (!client.connected()) {
reconnect();
}
else client.loop();
if (digitalRead(front_light_in) != front_light_sts) front_light_call();
else front_light_sts_dly = millis();
if (digitalRead(rear_light_in) != rear_light_sts) rear_light_call();
else rear_light_sts_dly = millis();
if (digitalRead(fan_in) != fan_sts) fan_call();
else fan_sts_dly = millis();
if (digitalRead(night_lamp_in) != night_lamp_sts) night_lamp_call();
else night_lamp_sts_dly = millis();
if (digitalRead(socket_in) != socket_sts) socket_call();
else socket_sts_dly = millis();
}
void recieved_cmd(String in_str, int in_int1, int in_int2){ // this function handle all MQTT request
if (in_str == "ashish/bed1/sw1/cmd/front_light") {
//Serial.print("MQTT >> Front Light "); Serial.println(in_int2);
front_light_stpt = in_int1;
analogWrite(front_light, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/rear_light") {
//Serial.print("MQTT >> Rear Light "); Serial.println(in_int2);
rear_light_stpt = in_int1;
analogWrite(rear_light, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/fan") {
//Serial.print("MQTT >> Fan Light "); Serial.println(in_int2);
fan_stpt = in_int1;
analogWrite(fan, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/socket1") {
//Serial.print("MQTT >> Socket1 "); Serial.println(in_int2);
socket1_stpt = in_int1;
analogWrite(socket1, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/socket2") {
//Serial.print("MQTT >> Socket2 "); Serial.println(in_int2);
socket2_stpt = in_int1;
analogWrite(socket2, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/night_lamp") {
//Serial.print("Night Lamp "); Serial.println(in_int2);
night_lamp_stpt = in_int1;
analogWrite(night_lamp, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/all_device") {
//Serial.print("MQTT >>All Devices "); Serial.println(in_int2);
digitalWrite ( night_lamp , HIGH );
digitalWrite ( front_light , HIGH );
digitalWrite ( rear_light , HIGH );
digitalWrite ( fan , LOW );
digitalWrite ( socket1 ,HIGH );
digitalWrite ( socket2 ,HIGH );
}
}
void front_light_call(){ //This function handle hardwired switching request
if(millis() - front_light_sts_dly > 500) {
if (front_light_stpt > 0) {
front_light_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/front_light", "0");
analogWrite(front_light, 0);
}
else {
front_light_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/front_light", "100");
analogWrite(front_light, 1024);
}
front_light_sts = ! front_light_sts;
}
}
void rear_light_call(){ //This function handle hardwired switching request
if (millis() - rear_light_sts_dly > 500) {
if (rear_light_stpt > 0) {
rear_light_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/rear_light", "0");
analogWrite(rear_light, 0);
}
else {
rear_light_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/rear_light", "100");
analogWrite(rear_light, 1024);
}
rear_light_sts = ! rear_light_sts;
}
}
void night_lamp_call(){ //This function handle hardwired switching request
if (millis() - night_lamp_sts_dly > 500) {
if (night_lamp_stpt > 0) {
night_lamp_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/night_lamp", "0");
analogWrite(night_lamp, 0);
}
else {
night_lamp_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/night_lamp", "100");
analogWrite(night_lamp, 1024);
}
night_lamp_sts = ! night_lamp_sts;
}
}
void fan_call(){ //This function handle hardwired switching request
if (millis() - fan_sts_dly > 500) {
if (fan_stpt > 0) {
fan_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/fan", "0");
analogWrite(fan, fan_stpt);
}
else {
fan_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/fan", "100");
analogWrite(fan, fan_stpt);
}
fan_sts = ! fan_sts;
}
}
void socket_call(){ //This function handle hardwired switching request
if (millis() - socket_sts_dly > 500) {
if (socket1_stpt > 0 and socket2_stpt == 0 ) {//10
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt == 0 and socket2_stpt > 0 ) {//01
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt == 0 and socket2_stpt == 0 ) {//00
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt > 0 and socket2_stpt > 0 ){//11
socket1_stpt = 0;
socket2_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/socket1", "0");
client.publish("ashish/bed1/sw1/cmd/socket2", "0");
}
socket_sts = !socket_sts;
analogWrite(socket1, socket1_stpt);
analogWrite(socket2, socket2_stpt);
}
}
Esp will connect local network on which my raspberry Pi & Alexa connected.
Esp subscribe topics & published on topics as mentioned in Arduino code.
If any input pin state change fore more than 20 Milli second then corresponding output toggles & esp published new state of output.
+++++ Arduino code++++++
#include
#include
// Update these with values suitable for your network.
const char* ssid = "Your network id";
const char* password = "your password";
const char* mqtt_server = "192.168.1.165"; //IP of your mqtt server(Raspberry PI)
//Output defination
byte night_lamp = 16 ; // D0
byte front_light = 0 ; // D3
byte rear_light = 2 ; // D4
byte fan = 15 ; // D8
byte socket1 = 3 ; // RX
byte socket2 = 1 ; // TX
//Input defination
byte night_lamp_in = 5 ; // D1
byte front_light_in = 4 ; // D2
byte rear_light_in = 14 ; // D5
byte socket_in = 12 ; // D6
byte fan_in = 13 ; // D7
//Input Bit status by reading input pin status
byte night_lamp_sts;
byte front_light_sts;
byte rear_light_sts;
byte fan_sts;
byte socket_sts;
//Input debounse delay to avoide unwanted switching
unsigned long night_lamp_sts_dly;
unsigned long front_light_sts_dly;
unsigned long rear_light_sts_dly;
unsigned long fan_sts_dly;
unsigned long socket_sts_dly;
//Output Setpoint
int night_lamp_stpt;
int front_light_stpt;
int rear_light_stpt;
int fan_stpt;
int socket1_stpt;
int socket2_stpt;
WiFiClient espClient;
PubSubClient client(espClient);
char msg[50]; // to storing MQTT message
void setup() {
//Output Setup
pinMode ( night_lamp , OUTPUT );
pinMode ( front_light , OUTPUT );
pinMode ( rear_light , OUTPUT );
pinMode ( fan , OUTPUT );
pinMode ( socket1 , OUTPUT );
pinMode ( socket2 , OUTPUT );
//Output initialised
digitalWrite ( night_lamp , HIGH );
digitalWrite ( front_light , HIGH );
digitalWrite ( rear_light , HIGH );
digitalWrite ( fan , HIGH );
digitalWrite ( socket1 ,HIGH );
digitalWrite ( socket2 ,HIGH );
//Input Setup
pinMode ( night_lamp_in , INPUT );
pinMode ( front_light_in , INPUT );
pinMode ( rear_light_in , INPUT );
pinMode ( socket_in , INPUT );
pinMode ( fan_in , INPUT );
//Printing initial input status **** do not connect rx & tx pin to relay during debugging ***
/*
Serial.begin(115200);
Serial.println();
Serial.print ("night_lamp_sts "); Serial.println ( night_lamp_sts );
Serial.print ("front_light_sts "); Serial.println ( front_light_sts );
Serial.print ("rear light_sts "); Serial.println ( rear_light_sts );
Serial.print ("fan_sts "); Serial.println ( fan_sts );
Serial.print ("socket_sts "); Serial.println ( socket_sts );
*/
//Setup WiFI
setup_wifi();
client.setServer(mqtt_server, 1883);
client.setCallback(callback);
night_lamp_sts_dly = millis();
front_light_sts_dly = millis();
rear_light_sts_dly = millis();
fan_sts_dly = millis();
socket_sts_dly = millis();
//Initialised input status bit
night_lamp_sts = digitalRead(night_lamp_in);
front_light_sts = digitalRead(front_light_in);
rear_light_sts = digitalRead(rear_light_in);
fan_sts = digitalRead(fan_in);
socket_sts = !digitalRead(socket_sts);
}
// XXXXXXXXXX uncomment Serial.print statement for debugging purpose.
void setup_wifi() {
delay(10);
// We start by connecting to a WiFi network
//Serial.println();
//Serial.print("Connecting to ");
//Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
//Serial.print(".");
}
//Serial.println("");
//Serial.println("WiFi connected");
//Serial.println("IP address: ");
//Serial.println(WiFi.localIP());
}
void callback(char* topic, byte* payload, unsigned int length) {
String payloadval_str;
int payloadval_int, payload_pwm;
for (int i = 0; i < length; i++) payloadval_str += (char)payload[i];
payloadval_int = payloadval_str.toInt();
payload_pwm = map(payloadval_int, 100, 0, 0, 1024);
recieved_cmd(topic, payloadval_int, payload_pwm);
//Serial.println("Message Recieved");Serial.print(topic);Serial.print(" ");Serial.println(payloadval_int);
}
void reconnect() {
// Loop until we're reconnected
if (!client.connected()) {
//Serial.print("Attempting MQTT connection...");
// Attempt to connect
if (client.connect("ESP8266Client")) {
//Serial.println("connected");
// Once connected, publish an announcement...
client.publish("ashish/bed1/sw1/", "SB1 is Connected");
// ... and resubscribe
client.subscribe("ashish/bed1/sw1/cmd/#");
} else {
//Serial.print("failed, rc=");
//Serial.print(client.state());
//Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
}
}
//Serial.println("Pub start");
snprintf (msg, 1, "0");
client.publish("ashish/bed1/sw1/initialised", msg);
//Serial.print(msg);
}
void loop() {
if (!client.connected()) {
reconnect();
}
else client.loop();
if (digitalRead(front_light_in) != front_light_sts) front_light_call();
else front_light_sts_dly = millis();
if (digitalRead(rear_light_in) != rear_light_sts) rear_light_call();
else rear_light_sts_dly = millis();
if (digitalRead(fan_in) != fan_sts) fan_call();
else fan_sts_dly = millis();
if (digitalRead(night_lamp_in) != night_lamp_sts) night_lamp_call();
else night_lamp_sts_dly = millis();
if (digitalRead(socket_in) != socket_sts) socket_call();
else socket_sts_dly = millis();
}
void recieved_cmd(String in_str, int in_int1, int in_int2){ // this function handle all MQTT request
if (in_str == "ashish/bed1/sw1/cmd/front_light") {
//Serial.print("MQTT >> Front Light "); Serial.println(in_int2);
front_light_stpt = in_int1;
analogWrite(front_light, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/rear_light") {
//Serial.print("MQTT >> Rear Light "); Serial.println(in_int2);
rear_light_stpt = in_int1;
analogWrite(rear_light, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/fan") {
//Serial.print("MQTT >> Fan Light "); Serial.println(in_int2);
fan_stpt = in_int1;
analogWrite(fan, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/socket1") {
//Serial.print("MQTT >> Socket1 "); Serial.println(in_int2);
socket1_stpt = in_int1;
analogWrite(socket1, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/socket2") {
//Serial.print("MQTT >> Socket2 "); Serial.println(in_int2);
socket2_stpt = in_int1;
analogWrite(socket2, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/night_lamp") {
//Serial.print("Night Lamp "); Serial.println(in_int2);
night_lamp_stpt = in_int1;
analogWrite(night_lamp, in_int2);
}
if (in_str == "ashish/bed1/sw1/cmd/all_device") {
//Serial.print("MQTT >>All Devices "); Serial.println(in_int2);
digitalWrite ( night_lamp , HIGH );
digitalWrite ( front_light , HIGH );
digitalWrite ( rear_light , HIGH );
digitalWrite ( fan , LOW );
digitalWrite ( socket1 ,HIGH );
digitalWrite ( socket2 ,HIGH );
}
}
void front_light_call(){ //This function handle hardwired switching request
if(millis() - front_light_sts_dly > 500) {
if (front_light_stpt > 0) {
front_light_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/front_light", "0");
analogWrite(front_light, 0);
}
else {
front_light_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/front_light", "100");
analogWrite(front_light, 1024);
}
front_light_sts = ! front_light_sts;
}
}
void rear_light_call(){ //This function handle hardwired switching request
if (millis() - rear_light_sts_dly > 500) {
if (rear_light_stpt > 0) {
rear_light_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/rear_light", "0");
analogWrite(rear_light, 0);
}
else {
rear_light_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/rear_light", "100");
analogWrite(rear_light, 1024);
}
rear_light_sts = ! rear_light_sts;
}
}
void night_lamp_call(){ //This function handle hardwired switching request
if (millis() - night_lamp_sts_dly > 500) {
if (night_lamp_stpt > 0) {
night_lamp_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/night_lamp", "0");
analogWrite(night_lamp, 0);
}
else {
night_lamp_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/night_lamp", "100");
analogWrite(night_lamp, 1024);
}
night_lamp_sts = ! night_lamp_sts;
}
}
void fan_call(){ //This function handle hardwired switching request
if (millis() - fan_sts_dly > 500) {
if (fan_stpt > 0) {
fan_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/fan", "0");
analogWrite(fan, fan_stpt);
}
else {
fan_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/fan", "100");
analogWrite(fan, fan_stpt);
}
fan_sts = ! fan_sts;
}
}
void socket_call(){ //This function handle hardwired switching request
if (millis() - socket_sts_dly > 500) {
if (socket1_stpt > 0 and socket2_stpt == 0 ) {//10
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt == 0 and socket2_stpt > 0 ) {//01
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt == 0 and socket2_stpt == 0 ) {//00
socket1_stpt = 1024;
socket2_stpt = 1024;
client.publish("ashish/bed1/sw1/cmd/socket1", "100");
client.publish("ashish/bed1/sw1/cmd/socket2", "100");
}
else if (socket1_stpt > 0 and socket2_stpt > 0 ){//11
socket1_stpt = 0;
socket2_stpt = 0;
client.publish("ashish/bed1/sw1/cmd/socket1", "0");
client.publish("ashish/bed1/sw1/cmd/socket2", "0");
}
socket_sts = !socket_sts;
analogWrite(socket1, socket1_stpt);
analogWrite(socket2, socket2_stpt);
}
}
Step 6: Nodered Sketch
[{"id":"4ee3d2a.c06182c","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Rear_Light","topic":"ashish/bed1/sw1/rear_light","qos":"0","broker":"7980bb5f.184444","x":180,"y":120,"wires":[["5c1c613d.3c34"]]},{"id":"50b7c57a.5e05ac","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Night_Lamp","topic":"ashish/bed1/sw1/night_lamp","qos":"0","broker":"7980bb5f.184444","x":190,"y":534,"wires":[["9d429700.4bb298"]]},{"id":"297e2f3b.b4a7f","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Fan","topic":"ashish/bed1/sw1/fan","qos":"0","broker":"7980bb5f.184444","x":170,"y":220,"wires":[["a3e96244.855ce"]]},{"id":"49a96ae6.7437d4","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Front_Light","topic":"ashish/bed1/sw1/front_light","qos":"0","broker":"7980bb5f.184444","x":190,"y":20,"wires":[["4b2695c3.37e1dc"]]},{"id":"a6405d1f.4e8b9","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Socket1","topic":"ashish/bed1/sw1/socket1","qos":"0","broker":"7980bb5f.184444","x":180,"y":320,"wires":[["c4fd9ef9.a3063"]]},{"id":"4b2695c3.37e1dc","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"front light","inputtrigger":true,"x":380,"y":20,"wires":[["db0ab654.3f8a28"]]},{"id":"5c1c613d.3c34","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"rear light","inputtrigger":true,"x":380,"y":120,"wires":[["d5813ca6.5f331"]]},{"id":"a3e96244.855ce","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"fan","inputtrigger":true,"x":370,"y":220,"wires":[["79580806.6f9e28"]]},{"id":"c4fd9ef9.a3063","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"socket1","inputtrigger":true,"x":380,"y":320,"wires":[["f65df10b.678a4"]]},{"id":"9d429700.4bb298","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"night lamp","inputtrigger":true,"x":390,"y":520,"wires":[["3eee0d3c.2ec242"]]},{"id":"d1374527.23a918","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"All devices","topic":"ashish/bed1/sw1/all_devices","qos":"0","broker":"7980bb5f.184444","x":180,"y":640,"wires":[["d3b0ee4a.050b"]]},{"id":"db0ab654.3f8a28","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":550,"y":20,"wires":[["1a63d9f9.bb5966"],["f40c9e41.311f3"]]},{"id":"f40c9e41.311f3","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":20,"wires":[["ed52b8ef.322d08"]]},{"id":"1a63d9f9.bb5966","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":690,"y":20,"wires":[["ed52b8ef.322d08"]]},{"id":"d5813ca6.5f331","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":120,"wires":[["b7757bef.5a2da8"],["1297239c.f484cc"]]},{"id":"b7757bef.5a2da8","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":690,"y":120,"wires":[["721732ab.b1f8ec"]]},{"id":"1297239c.f484cc","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":120,"wires":[["721732ab.b1f8ec"]]},{"id":"79580806.6f9e28","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":220,"wires":[["daf71170.2b6f"],["491d6c14.74b384"]]},{"id":"daf71170.2b6f","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":710,"y":220,"wires":[["1d3dd882.b0b8f7"]]},{"id":"491d6c14.74b384","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":220,"wires":[["1d3dd882.b0b8f7"]]},{"id":"f65df10b.678a4","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":320,"wires":[["e4962564.4f1e38"],["c9ea4caf.0a1c3"]]},{"id":"e4962564.4f1e38","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":710,"y":320,"wires":[["59d6709d.0e5a5"]]},{"id":"c9ea4caf.0a1c3","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":320,"wires":[["59d6709d.0e5a5"]]},{"id":"3eee0d3c.2ec242","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":534,"wires":[["aa79b47d.ed17b8"],["46816734.4eeac8"]]},{"id":"aa79b47d.ed17b8","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":710,"y":534,"wires":[["fef43b79.e54488"]]},{"id":"46816734.4eeac8","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":534,"wires":[["fef43b79.e54488"]]},{"id":"b60b9c31.639fc","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":654,"wires":[["cdd5a640.b81d78"],["3009c3d4.7ae1fc"]]},{"id":"cdd5a640.b81d78","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":710,"y":634,"wires":[["fef43b79.e54488","59d6709d.0e5a5","1d3dd882.b0b8f7","721732ab.b1f8ec","ed52b8ef.322d08","6fc46ae1.7dc424","d4740469.2bd6d8","5ba4d0a8.bca1f","8cc3adc7.3a78f","ea68c68a.3ed318","ab1da280.8fbd7","49b23beb.2165a4","9c2b5673.a26538"]]},{"id":"3009c3d4.7ae1fc","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":720,"y":674,"wires":[["721732ab.b1f8ec","1d3dd882.b0b8f7","59d6709d.0e5a5","fef43b79.e54488","ed52b8ef.322d08","6fc46ae1.7dc424","d4740469.2bd6d8","5ba4d0a8.bca1f","8cc3adc7.3a78f","ea68c68a.3ed318","ab1da280.8fbd7","49b23beb.2165a4","9c2b5673.a26538"]]},{"id":"ed52b8ef.322d08","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Front Light","topic":"ashish/bed1/sw1/cmd/front_light","qos":"","retain":"","broker":"7980bb5f.184444","x":990,"y":20,"wires":[]},{"id":"721732ab.b1f8ec","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Rear Light","topic":"ashish/bed1/sw1/cmd/rear_light","qos":"","retain":"","broker":"7980bb5f.184444","x":990,"y":120,"wires":[]},{"id":"1d3dd882.b0b8f7","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Fan","topic":"ashish/bed1/sw1/cmd/fan","qos":"","retain":"","broker":"7980bb5f.184444","x":970,"y":220,"wires":[]},{"id":"59d6709d.0e5a5","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Socket1","topic":"ashish/bed1/sw1/cmd/socket1","qos":"","retain":"","broker":"7980bb5f.184444","x":980,"y":320,"wires":[]},{"id":"fef43b79.e54488","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Night Lamp","topic":"ashish/bed1/sw1/cmd/night_lamp","qos":"","retain":"","broker":"7980bb5f.184444","x":990,"y":534,"wires":[]},{"id":"1e24a543.40864b","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Socket2","topic":"ashish/bed1/sw1/socket2","qos":"0","broker":"7980bb5f.184444","x":180,"y":420,"wires":[["96598cdd.0409b"]]},{"id":"96598cdd.0409b","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"socket2","inputtrigger":true,"x":380,"y":420,"wires":[["3909c390.30a30c"]]},{"id":"3909c390.30a30c","type":"switch","z":"8ea73ec5.2e8d5","name":"switch","property":"on_off_command","propertyType":"msg","rules":[{"t":"true"},{"t":"false"}],"checkall":"false","repair":false,"outputs":2,"x":570,"y":420,"wires":[["b9aac2d4.29c38"],["99bfa0c1.58364"]]},{"id":"b9aac2d4.29c38","type":"function","z":"8ea73ec5.2e8d5","name":"on_off","func":"if (msg.payload == \"on\")\n{\n msg.payload = 100\n}\nif (msg.payload == \"off\")\n{\n msg.payload = 0\n\n}\nreturn msg;\n","outputs":1,"noerr":0,"x":710,"y":420,"wires":[["6fc46ae1.7dc424"]]},{"id":"99bfa0c1.58364","type":"function","z":"8ea73ec5.2e8d5","name":"Setpoint","func":"msg.payload = msg.bri\nreturn msg;","outputs":1,"noerr":0,"x":840,"y":420,"wires":[["6fc46ae1.7dc424"]]},{"id":"6fc46ae1.7dc424","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Socket2","topic":"ashish/bed1/sw1/cmd/socket2","qos":"","retain":"","broker":"7980bb5f.184444","x":980,"y":420,"wires":[]},{"id":"62feabc6.1cd3e4","type":"mqtt in","z":"8ea73ec5.2e8d5","name":"Boot Up initialised","topic":"ashish/bed1/sw1/initialised","qos":"2","broker":"7980bb5f.184444","x":210,"y":740,"wires":[["d3b0ee4a.050b"]]},{"id":"d3b0ee4a.050b","type":"alexa-local","z":"8ea73ec5.2e8d5","devicename":"all devices","inputtrigger":true,"x":390,"y":640,"wires":[["b60b9c31.639fc"]]},{"id":"d4740469.2bd6d8","type":"mqtt out","z":"8ea73ec5.2e8d5","name":"Night Lamp","topic":"ashish/bed1/sw1/cmd/all_devices","qos":"","retain":"","broker":"7980bb5f.184444","x":1010,"y":640,"wires":[]},{"id":"49b23beb.2165a4","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Rear Light","label":"Rear Light","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":190,"y":180,"wires":[["5c1c613d.3c34"]]},{"id":"ab1da280.8fbd7","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Fan","label":"Fan","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":170,"y":280,"wires":[["a3e96244.855ce"]]},{"id":"ea68c68a.3ed318","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Socket1","label":"Socket1","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":180,"y":380,"wires":[["c4fd9ef9.a3063"]]},{"id":"8cc3adc7.3a78f","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Socket2","label":"Socket2","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":180,"y":480,"wires":[["96598cdd.0409b"]]},{"id":"5ba4d0a8.bca1f","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Night Lamp","label":"Night Lamp","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":190,"y":580,"wires":[["9d429700.4bb298"]]},{"id":"1a7c28cf.0b06d7","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"All Devices","label":"All Devices","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":190,"y":700,"wires":[["d3b0ee4a.050b"]]},{"id":"9c2b5673.a26538","type":"ui_switch","z":"8ea73ec5.2e8d5","name":"Front Light","label":"Front Light","group":"f309372f.eb1298","order":0,"width":"3","height":"1","passthru":true,"decouple":"false","topic":"","style":"","onvalue":"100","onvalueType":"num","onicon":"","oncolor":"","offvalue":"0","offvalueType":"num","officon":"","offcolor":"","x":190,"y":80,"wires":[["4b2695c3.37e1dc"]],"inputLabels":["ashish/bed1/sw1/cmd/front_light"]},{"id":"7980bb5f.184444","type":"mqtt-broker","z":"","name":"ashish pi vf","broker":"192.168.1.165","port":"1883","clientid":"","usetls":false,"compatmode":true,"keepalive":"60","cleansession":true,"birthTopic":"","birthQos":"0","birthPayload":"","closeTopic":"","closeQos":"0","closePayload":"","willTopic":"","willQos":"0","willPayload":""},{"id":"f309372f.eb1298","type":"ui_group","z":"","name":"SWITCH BORD01","tab":"f5e4e4e1.267548","order":1,"disp":true,"width":"7","collapse":true},{"id":"f5e4e4e1.267548","type":"ui_tab","z":"","name":"Ashish Bed Room","icon":"home","order":1}]
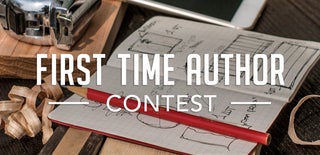
Participated in the
First Time Author