Introduction: Talking, Motion Activated Skull
This is a fun but fairly detailed mechanical build due to the jaw mechanics. Part one deals with the software and coding and is detailed in this document:
The project is built around a plain old arduino, a servo to animate the skull jaw to the sound, a low cost $2 mp3 player module (with build in speaker amplifier), and an ultrasonic HC-SR04 distance sensor to detect your porch victims.
Here's a video of the completed, distance triggered animated laughing skull, perfect for the front porch or mounted to watch over a candy bowl!
Supplies
Tools:
- Hot glue gun
- X-acto knife
- Diagonal cutters
- Needle nose pliers / foreceps
- Dremel rotary tool
- Cutting wheels
- High speed cutting bit
- 1/16", 3/64th" and/or 1/32" drill bits
Parts:
- 1 - USB cable (for arduino dev work)
- 1 - Arduino board (recommend "Funduino" red, with extra pin headers)
- 1 - 9v Alkaline Battery w/attached clip
- 1 - DFPlayer mini, serial controllable MP3 player
- 1 - 4GiB µSD card (w/ten scary skull sounds preloaded)
- 1 - Speaker (8ohm, 3W)
- 1 - Ultrasonic PING distance sensor (HC-SR04, 4pins)
- 2 - Red LEDs 2 - 100Ω resistors (for LEDs)
- 2 - 1kΩ resistors (for MP3 serial lines)
- 4 - Female / Female connecting wires
- 12 - Male / Female connector wires
- 1 - SG90 micro 9g servo (for moving the mouth)
- 1 - popsicle stick (for reinforcing the servo mount)
- (opt) - breadboard (if building other things vs making skull electronics permanent)
- (opt) - foam board and velcro (unmounting & use arduino/mp3 in other projects)
- Skill with a a dremel cutter and hot glue (adult supervision)
Step 1: The Software Build - Blink
This build guide is intended to be done in conjunction with the Let's Code Blacksburg "Arduino Cookbook". You hook up a component like a servo, and you use the "Servo Recipe" from the Arduino cookbook to get it working, then move on to the next step.
1) Use First Cookbook Recipe - Blink
Before you build anything or do any real programming, you need to first open your the Files / Examples / 01. Basics / Blink, click the compile/upload icon, and verify that blink is actually working (blinking your pin 13 LED indicator). If that works, then try changing the blink speed of the LED. This will verify that your computer and the installed arduino IDE software (from www.arduino.cc) is properly configured to talk to your arduino.
This is not the same eye blinking code we will be using later, so once working, you can discard this program, but be aware, each time you get a new piece of code working, you should save it, and give it a meaningful name. All the steps of code are all pre-done and working in the Lets Code Blacksburg! github repo for this project here.
Step 2: Step 2) Mouth Servo & Rough Calibration
This next step is purely to check to make sure your servo is working, and that you get the approximate values you think you will use to open and close your skull's mouth. Before we actually attach the servo to the skull, we need to just hook the servo up to pin 6 (see right/below), load the sample LCBB_TalkingSkull_2_servotest.ino code from the github repository, and test the servo movement starting with open/closed values of ~90 and ~110.
NOTE: We are not hooking the servo to the skull at this point.
This step is merely for testing your servo and verifying it's working well.
Once working, save your code and call it TalkingSkull_servotest. We will integrate this and your other code recipes in a complete "dish" at the end of the coding section.
Stretch Goal:
Write a function called laughCount(n) that will take in integer “n” and laugh (move the servo) that many times! (if you can't do that, we'll give you the code later).
Step 3: Step 3: Ultrasonic “ping” Distance Sensor (the Eyes)
Start a new program, and save it to the file named Talking-Skull_3_ping-test before getting started.
To read up on the theory of the Ultrasonic distance sensor, look up the INPUT: Ultrasonic “Eyes” Range Sensor section of the “LCBB Arduino Cookbook” here. We're going to hook up our US-100 or HC-SR04 distance sensor to Ground and VCC (outer two pins) and pins 4 & 5 for Trigger and Echo on the sensor respectively.
The code for this is located here if you want to just copy/paste it. Get this code to compile and upload , and turn click your arduino serial monitor icon to see if you're recording the approximate distance to a large flat object like a book or jacket. If you get an error when clicking on the serial monitor, check your arduino software's tools / port setting and make sure the correct serial device is selected.
You should be getting back data like this as you move objects away from and closer to your distance sensor:
Distance = 17 Distance = 17 Distance = 18 Distance = 19 Distance = 21 Distance = 20 Distance = 16 Distance = 12 Distance = 8 Distance = 5 ************** Someone is close! ************** Distance = 5 move mouth, play sound
If your code is working.. SAVE IT with the meaningful name TalkingSkull_3_pingtest .
Stretch Goal:
Now when your distance sensor is triggered, instead of just printing “move mouth, play sound”, instead, pull the needed variables, setup and loop code to actually move the skull's mouth servo opened and closed.
Step 4: Step 4: Implement the DFPlayer Mini, Serial Controlled MP3 Player
The DFPlayer is a 3.3v input/output device and it is very sensitive and will die if it is not hooked up exactly right. When hooking up this circuit, leave it unplugged from USB and the battery and double check everything before you plug in USB or power it up. Hooking this MP3 player wrong can destroy the serial control lines, making it useless for this project.
WARNING: Do not hook up USB cable or 9V battery before examining it closely. Not being extra careful at this stage can easily blow the DFPlayer's serial I/O control lines. Ask me how I know… I inadvertently blew two DFPlayers while writing this workshop. Learn from my mistakes.
Notice that the DFPlayer is connected to the 5volts pin for power, but that its serial TX (transmit) and
RXC (receive) lines are buffered (made safe) by going through two 1k ohm resistors. These resistors just drop a couple of volts off the 5v on the arduino I/O pins so they're safe to use with the DFPlayer's I/O pins. Not doing this, will blow the serial control lines of the DFPlayer. Since we are not using a breadboard for this workshop, we're going to be hooking these resistors directly in to two M/F connection wires, and pushing them directly into pins 10 & 11 of the arduino, as seen in the photos.
Just like we're hooking the serial lines, here's what the whole DFPlayer looks like all using the direct connect method. Pin 1 (5v) is the upper left pin. Upper right is the unit's pin 16 (_BUSY). This is how we are going to tell when the DFPlayer is busy playing a sound (which is when we want to be moving the mouth). This code requires that you install the library. Do this from the arduino menu Sketch / Include Library / Manage Libraries and search for the DFPlayer_Mini_MP3 library. Install it if not already installed.
Here's the code for implementing a basic, sequential sound file MP3 player:
// Hardware wiring of DFPlay // // DFPlay mini Vcc(1)RX(2)TX(3)DACR(4)GND(7) +(6) (8) ... _BUSY // | | | | | | | | // Arduino 5v 11* 10* A0 GND | | 12* // \Spkr/ // * requires 1k resisitor
Here is the recipe code for getting the DFPLayer MP3 working. Once you get it working, save this code to a filename like Talking-Skull_4_dfplayer-test.
Step 5: Step 5: Bringing All the Code All Together With the Skull Hardware!
Now, if you previously disconnected the servo or ultrasonic range finder, now go ahead and connect in each of the components as shown (making sure the servo has all three pins correctly connected).
If you got your DFPlayer working in step 4, then you should not need any “new code” except the code from steps 2, 3 and 4, combined with the laughCount(n) challenge in step 3, and carefully bring it all together.
If you need to see the form of what it all looks like together, take a peek out at LCBB_TalkingSkull_5_ping-servo-dfplayer_complete and bring in whatever code you need. After you have your code working, BE SURE TO SAVE IT with a meaningful name such as TalkingSkull_ping-servo-dfplayer. Once you have this all working, you're ready to load it all into the skull, hot glue your speaker into place and do your final testing
Follow the “Part-2” LCBB_Talking-Skull_Pt-2_Hware-Hacking-Guide.pdf out on our github site!
Here are some photos to visually step you through the mechanical steps of the skull build (using any standard 6" plastic skull with an articulating jaw).
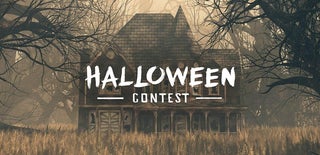
Participated in the
Halloween Contest