Introduction: ThermoClock: an OpenSource Arduino UNO OLED Clock That Also Measures Temperature
Hello, everyone !
Today I am going to show you how to make An OpenSource Arduino UNO OLED Clock That Also Measures Temperature. This project is an OpenSource and it's open to everyone. It doesn't require any special engineer skills, and will take you less than couple of hours once you have all the parts you need.
This clock will not only show you exact time and date but also it will show you the ambient temperature in both Fahrenheit and Celsius, with accuracy of 0.5°C (-25°C to +85°C) and with resolution of 0.0625°C. The pages are changing on every 10 seconds, but you can change that.
The OLED we will use in this project is 1.3″ inch in size,monochrome blue in color, features 132×64 pixels and uses the SPI Bus. .
The temperature sensor we will use is TMP102, it's very small on size, low on power and uses the I2C Bus to communicate with our Arduino.
And the DS1307 real time clock module uses the I2C bus so we will only need 2 pins to retrieve the date and time and display the results on the OLED display.
We will use the u8glib library to communicate with our display. This library has many available parameters with it you can display numbers, letters, bitmaps and other symbols on OLEDs and graphic LCDs also it works for E-ink displays as well as other libraries.
Let's get started ! :)
Step 1: Materials & Tools
Most of the stuff for this project came from the Adafruit store the rest from SparkFun. (I already had them :) )
To make it we will need:
- Arduino UNO board or similar;
- OLED display - (1.3inch; 128x64) or similar;
- DS1307 - RTC module;
- TMP102 - digital temp sensor;
- Prototyping PCB;
- Headers - female and male;
- Some wires - to connect everything;
- Soldering iron and wire - to solder everything on the PCB;
- Clips - to cut wire;
- Cutting tools (Scissors, cutter, etc.);
- Material for the enclosure (Plastic, cardboard, metal, etc.);
- Super glue.
Step 2: Building the ThermoClock
I give you 3 ways to build your clock.
First way, on Breadboard:
First step is to place the OLED on the breadboard. Then connect pin 13 to CLK, pin 11 to DIN, pin 9 to CS, pin 8 to D/C and pin 7 to RES. (Pins:13,12,11,10 are the SPI Bus of the Arduino UNO).
Second step is to place the TMP102 and the RTC modules on the breadboard and connect their SDA and CLK legs together then to the 'duino board (they are A4 and A5). Now connect their GND legs toghether then to ground on the UNO board, connect the +V leg of TMP102 to +3v and the +V leg of the RTC to +5v pins on the Arduino UNO.
Like on the Fritzing schematic above.
Second way, make your own PCB shield:
If chose this way you may wanna watch this episode of Ben Heck's Show: Let's Try PCB Etching! for info on different ways to make a PCB.
To get a ready done PCB download the fritzing file ThermoClock.fzz and open it with Fritzing. You can get Fritzing from here. You can also order the PCB trough Fritzing if you don't want to make it.
Third way, with Prototyping PCB:
This way is same as the first one but much stable. First place the headers for the OLED and the breakouts on the PCB and solder them. Then connect the GND/+V legs by soldering wires.Same for the SPI and I2C legs of the modules.
Like on the Fritzing schematic above.
Attachments
Step 3: Enclosure for the ThermoClock
I made the enclosure by cutting it out of transparent plastic film (Why transparent ? I want it to be visible cause its clock, not bomb :D), bending its sides and gluing them with super glue, so it can turn into a box big enough for the project. The size of the box is 75 x 55 x 32 mm X,Y and Z thick 1 mm. You can make you enclosure by 3D printing it, Laser cut it or craft it from cardboard, wood, metal or plastic.
Step 4: Code & Libraries
In this step I will show you the main code which you can copy or download it as full .ino file which you can open with Arduino IDE. The file is named ThermoClock_v2.6.16. and it's in BETA test so I want your suggestions on how and what should I change to make it better.
Now take a look at it.
<br><p>/*************LIBRARIES**********************/<br>#include <U8glib.h> // OLED
#include <Wire.h> // I2C #include <Time.h> // Time Manipulation #include <DS1307RTC.h> // DS1307 RTC /*************VARIABLES**********************/ char timebuf[10]; // Time char datebuf[10]; // Date int year2digit; // 2 digit year int year4digit; // 4 digit year int tmp102Address = 0x48;</p><p>const int SwitchTime = 10000; //switching time in millis /********************************************/ U8GLIB_SH1106_128X64 u8g(13, 11, 10, 9, 8); // CLK=13, DIN=11, CS=10, DC=9, Reset=8 // // // /****************DRAW FUNCTION***************/ void draw(void) {</p><p>u8g.setFont(u8g_font_fub17r); // select font u8g.setPrintPos(30, 28); // set position u8g.print(timebuf); // display time u8g.drawHLine(0,33,128); // draw line u8g.setPrintPos(12, 55); // set position u8g.print(datebuf); // display date }</p><p>void draw1(void) { float celsius = getTemperature(); float fahrenheit = (1.8 * celsius) + 32; u8g.setFont(u8g_font_fub17r); u8g.setPrintPos(15, 28); u8g.print(celsius); u8g.print(" C"); u8g.setPrintPos(12, 55); u8g.print(fahrenheit); u8g.println(" F"); }</p><p>float getTemperature(){ Wire.requestFrom(tmp102Address,2); </p><p> byte MSB = Wire.read(); byte LSB = Wire.read();</p><p> //it's a 12bit int, using two's compliment for negative int TemperatureSum = ((MSB << 8) | LSB) >> 4; </p><p> float celsius = TemperatureSum*0.0625; if (celsius > 180) { celsius = (celsius - 256); // For negative temperatures } else { celsius; } return celsius; }</p><p>void setup(void) { } void loop(void) {</p><p>tmElements_t tm; if (RTC.read(tm)) { year2digit = tm.Year - 30; // 2 digit year variable //year4digit = tm.Year + 1970; // 4 digit year variable</p><p>sprintf(timebuf, "%02d:%02d",tm.Hour, tm.Minute, tm.Second); // format time sprintf(datebuf, "%02d/%02d/%02d",tm.Day, tm.Month, year2digit); // format date /********************************************/</p><p>u8g.firstPage(); // Put information on OLED do { draw(); } while( u8g.nextPage() ); delay(SwitchTime);</p><p>u8g.firstPage(); // Put information on OLED do { draw1(); } while( u8g.nextPage() ); } delay(SwitchTime); // Delay }</p>
If you don't like the current font you can change it simply by editing the code. You can find all supported fonts here.
Libraries you will need:
DS1307RTC, u8glib and Time, download the libraries and then unzip them at /Program Files(x86)/Arduino/Libraries (default).
Attachments
Step 5: Almost Done !
As final step you have to upload the code to the 'duino and you are done !
Now you have your own ThermoClock and you will know the current time, date and temperature in Celsius and Fahrenheit.
You like it ? Please don't forget to vote for at least one of the contests I have joined with it ! :) (the top right corner !)
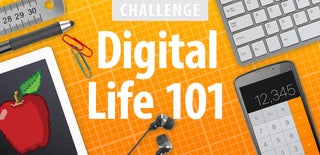
Participated in the
Digital Life 101 Challenge
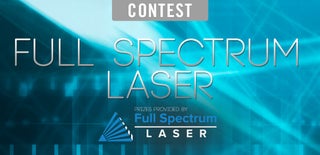
Participated in the
Full Spectrum Laser Contest 2016