Introduction: Thunderstorm Lightning Detector and Camera Trigger (or Droplet Detector)
Ever wanted to capture lightning on photo? Maybe this camera trigger will help you make incredible images. Sorry, it will not help you with the composition of your photo, the sensor sensitivity, shutter speed, aperture or whitebalance settings of your camera, but it will hit the shutterbutton as fast as possible when the lightning flash occurs.
This trigger is intended for cameras which can be controlled via a switch based remote control. I build it for my Canon EOS camera.
As a bonus it can be used as a droplet detector.
Step 1: Components
- 1x Arduino ProMicro (or any other Arduino)
- 2x optocoupler 4N25
- 2x diode 1N4148 (or any other diode)
- 2x resistor 470 ohm
- 3x Led
- 3x resistor 330 ohm
- 1x opamp LM358
- 4x photodiode (5mm IR Led Receiver 940 nm)
- 3x dipswitches
- 1x (trim)potmeter 10 kohm linear
- 1x resistor 10 kohm
- 2.5 mm jackplug (for connection to Canon EOS camera)
Step 2: Sensor Part
The sensor is build around an LM358 opamp and one or more reverse biased photodiodes in parallel. Adding photodiodes makes it more sensitive, especially during night conditions.
The output of the opamp is connected to a digital port of the Arduino. This MUST be an interrupt port in order to respond immediately to a lightning flash (interrupt the regular loop). The software must respond to a rising edge. Check the documentation of your Arduino for the correct port needed.
The opamp inputs are connected to analog inputs in order to read the threshold and the lightintensity.
Step 3: Camera Part
The camera is connected to the Arduino via optocouplers so it is electrically isolated. In my case the optocouplers are the “replacement” of the switches in my Canon RS60-E3 Remote Control and act as the shutter (-halfway) button.
Step 4: Arduino Software
Use the code shown below
/*
* Thunderstorm lightning detector and camera trigger.
*
* Pieter Horjus
* May 2020
*/
#include <Arduino.h>
int SHUTTERBUTTON_HALFWAY_PIN = 4;
int SHUTTERBUTTON_PIN = 5;
int PHOTOSEQUENCE_LED_PIN = 6;
int sensorPinThreshold = A8; // analog input pin for measuring the threshold
int sensorPinFlash = A9; // analog input pin for measuring the flashintensity
// Below specify the pin which is connected to the flashdetector.
// It MUST be a hardware-interrupt pin on your Arduino in order to respond immediately
// to the detected flash. Check the specification of the Arduino you are using.
int FLASH_DETECT_PIN = 7; // this pin is INT4 on an Arduino Leonardo
// (also Sparkfun Pro Micro)
volatile int maxValueMeasured = 0;
volatile long momentMaxValueIsMeasured = 0;
volatile boolean makingPhotoSequence = false;
volatile int flashcount = 0;
volatile long shutterbuttonPressedStartingmoment = 0;
volatile int sensorValueThreshold = 0;
volatile int sensorValueFlash = 0;
long shutterbuttonActiveTime = 300; // time (msec) the shutterbutton is pushed
// (increase in case you need continuous shooting)
long photosequencePeriod = 3000; // force an idle time (msec) between making two pictures
long currentTime;
long wakeupMoment = 0;
void setup(void)
{
Serial.begin(9600);
pinMode(SHUTTERBUTTON_HALFWAY_PIN, OUTPUT);
digitalWrite(SHUTTERBUTTON_HALFWAY_PIN, LOW);
pinMode(SHUTTERBUTTON_PIN, OUTPUT);
digitalWrite(SHUTTERBUTTON_PIN, LOW);
pinMode(PHOTOSEQUENCE_LED_PIN, OUTPUT);
digitalWrite(PHOTOSEQUENCE_LED_PIN, LOW);
pinMode(FLASH_DETECT_PIN, INPUT);
TXLED0; // deactivate TX led on Sparkfun
RXLED0; // deactivate RX led on Sparkfun
digitalWrite(SHUTTERBUTTON_HALFWAY_PIN, LOW);
attachInterrupt(digitalPinToInterrupt(FLASH_DETECT_PIN), detectedFlashInterruptRoutine, RISING);
}
void loop(void)
{
currentTime = millis();
checkPhotoSequence();
measureValuesLightsensors();
determineMaxValueMeasured();
checkWakeup();
showThreshold();
}
void detectedFlashInterruptRoutine() {
if (makingPhotoSequence == false) {
// immediately hit the shutterbutton so the camera can do its thing
digitalWrite(SHUTTERBUTTON_PIN, HIGH);
// from now on nothing is time-critical
digitalWrite(PHOTOSEQUENCE_LED_PIN, HIGH);
makingPhotoSequence = true;
flashcount++;
shutterbuttonPressedStartingmoment = millis();
Serial.print("amount flashes detected: ");
Serial.println(flashcount);
}
measureValuesLightsensors();
determineMaxValueMeasured();
}
void checkPhotoSequence() {
// The detectedFlashInterruptRoutine might have detected a flash.
// If so, it activated a photosequence.
if (makingPhotoSequence == true) {
// busy making a photo
if (currentTime > (shutterbuttonPressedStartingmoment + photosequencePeriod)) {
// Waited enough time after making a picture
// (including idle time after releasing the shutterbutton)
digitalWrite(PHOTOSEQUENCE_LED_PIN, LOW);
makingPhotoSequence = false;
} else if (currentTime > (shutterbuttonPressedStartingmoment + shutterbuttonActiveTime)) {
// end of pushing the shutterbutton
digitalWrite(SHUTTERBUTTON_PIN, LOW);
// end of pushing the shutterbutton halfway (it might be pushed during a wakeup)
digitalWrite(SHUTTERBUTTON_HALFWAY_PIN, LOW);
wakeupMoment = currentTime;
}
}
}
void measureValuesLightsensors() {
sensorValueThreshold = analogRead(sensorPinThreshold);
sensorValueFlash = analogRead(sensorPinFlash);
}
void determineMaxValueMeasured() {
if (sensorValueFlash > maxValueMeasured) {
maxValueMeasured = sensorValueFlash;
momentMaxValueIsMeasured = currentTime;
}
if (currentTime > (momentMaxValueIsMeasured + 10000)) {
// report 10 seconds after detection the max value measured
Serial.print("max: ");
Serial.println(maxValueMeasured);
maxValueMeasured = sensorValueFlash;
momentMaxValueIsMeasured = currentTime;
}
}
void checkWakeup() {
// if your camera has a sleeping mode, it might be wise to keep it awake
// so it reacts as fast as possible on shutterbutton pressed
long keepAwakeTime = 60000; // 60 seconds
if (currentTime > (wakeupMoment + keepAwakeTime)) {
// end of wakeup-sequence
// on systemstartup this is also hit (so test the end first)
digitalWrite(SHUTTERBUTTON_HALFWAY_PIN, LOW);
wakeupMoment = currentTime;
} else if (currentTime > (wakeupMoment + keepAwakeTime - 500)) {
// start of wakeup-sequence
digitalWrite(SHUTTERBUTTON_HALFWAY_PIN, HIGH);
}
}
void showThreshold() {
static long count = 0;
if ((count % 2500 == 0)) {
Serial.print("threshold: ");
Serial.println(sensorValueThreshold);
}
count++;
}
Step 5: Mounting Plate
Use thick drawing paper (used for watercolor drawing). Glue multiple layers on top of each other and sculpt a shape which fits the hot shoe of your camera. Use indelible wood glue. It makes the layers very stiff and sturdy. Secure the circuitboard on the mounting plate. Slide the plate on the hot shoe.
Step 6: The Testing Process (1)
I started construction during Christmas holiday 2019. I gave myself a deadline. I wanted to test the device by photographing the New Year fireworks in my neighborhood.
First tests were promising. The trigger responded to my tv remote control. At night it also detected a cameraflash at a distance of 30 feet. But was it enough?
Then the New Years fireworks came. It proved to be a success: 97 pictures shot in 30 minutes. I only needed to aim, the trigger operated the shutter.
Images: Canon EOS-300D, 18mm lens, manual focus, mode TV 2 sec, auto whitebalance.
Step 7: The Testing Process (2)
So I was ready to make pictures of the King's Day fireworks on April 27th and the 75-th End of WW-II Anniversary on May 5th here in the Netherlands.
Then came the coronavirus pandemic. No group gatherings allowed, no fireworks, no pictures.
Fortunately on April 30th, a small thunderstorm approached. First storm, four flashes, four detected, four pictures, two within view of the camera, two outside view. Not bad for a first attempt.
Images: Canon EOS-70D, 18 mm lens, manual focus, auto everything
Step 8: Ideas to Upgrade Your Triggerdevice
Add a display (OLED screen) which shows the threshold, measured intensity, flashcount, etc.
Step 9: Bonus 1: Droplet Detector
Look for the following line in the code
attachInterrupt(digitalPinToInterrupt(FLASH_DETECT_PIN), detectedFlashInterruptRoutine, RISING);
and change it to
attachInterrupt(digitalPinToInterrupt(FLASH_DETECT_PIN), detectedFlashInterruptRoutine, FALLING);
Power an infrared Led and position it opposite to your photodiode. This creates an infrared light barrier. Now you have a droplet detector. As soon as the droplet passes the barrier, the shutter is activated.
Start making amazing pictures.
Step 10: Droplet Detector, Capturing Technique
Capturing a repeating event (stream of droplets, left image) is pretty easy. You only need to manual focus the camera.
Capturing a single event (right image) is more challenging for your camera. I added a button and some extra software to the trigger device. When pushed the button activates the shutter-halfway circuit and keeps it activated. It is released when a droplet is detected. By manually activating it, the camera does the light measurement (aperture, sensor sensitivity, colorbalance, etc) in advance. So when the droplet is detected and the shutterbutton is activated, the camera immediately can open the shutter curtains.
Step 11: Bonus 2: Timelapse
Since all the electronics and drivers are present to activate/release the shutter button, it is very easy to build in timelapse functionality.
Create a loop:
- activate shutterbutton
- deactivate shutterbutton
- wait a while
Step 12: Points for Improvement
I used a small trimpotmeter for adjusting the threshold. This forces me to use a screwdriver for adjustment. Better use a potentiometer with a long shaft. This is particulary handy when lightconditions changes often.
Step 13: Conclusion
The lightning camera trigger works as expected. It even helps with making pictures of fireworks and droplets.
As you saw my pictures look very amateuristic, even bad. I have to work on my technical and artistic skills to improve this. But thats a whole different ballgame.
I hope you enjoyed reading this article.
Good luck and happy shooting.
Step 14: Links
Step 15: One Year Later ...
Here are two pictures i shot last year. It gives an impression what is possible.
Both pictures have an exposure time of 2 seconds. The left one is of a lightning strike at a distance of 15 km. The second one was of a storm front at a distance of 40 km. Watch the lightning strike behind the tree at the left lower counter.
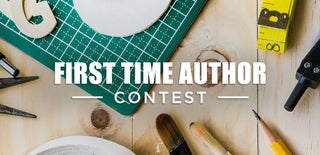
Participated in the
First Time Author Contest