Introduction: To Control RC Plane Through Mobile Phone's Accelerometer Using WiFi Module ESP8266 Explore
1.COMPARISON WITH RADIO CONTROLLED PLANE-
Cost Effectiveness
Decreases the efforts of the flyer
It works in 2.4-5 GHz Bandwidth where as RC works within 2.4GHz.
It has higher data transfer speed.
Step 1: INTRODUCTION
1.We(Chandan,Suraj,Chandan) have controlled RC plane by moving/tilting mobile phone and by moving a slider for motor using wifi module where there is wireless communication between plane and mobile phone.
2.It reduces the efforts of a flyer and also the cost of a plane.
Step 2: Ingredients
1.For plane construction:-
i.Styrofoam,
ii.Corrugated Sheets,
iii.3Servos,
iv.Brushless DC Motor(1100kV),
v.Battery(11.1V,2200mAh),
vi.Electronic Speed Controller(ESC)
vii.Plastic Electric Propeller
2. For Wireless Communication:-
i.Android mobile Phone,
ii.ESP8266 WiFi Module,
iii.FTDI Breakout Board,
iv.Jumper Wires,
v.3Resistors(1kΩ),
vi.Blank PCB(Printed Circuit Board)
3. Tools:-
i.Glue Gun,
ii.Soldering Iron Kit,
iii.Bread Board,
Step 3: A BASIC RC PLANE CIRCUIT DIAGRAM & DATA TRANSMISSION
DATA TRANSMISSION:-
1.There is
an inbuilt accelerometer sensor in mobile phone which provides data as a measure of rotation ( Pitch & Roll).
2.This data is measured through an app called "ROBOREMO APP".
3. Hence, data is transmitted from Roboremo app to WiFi module through WiFi connection between mobile phone and WiFi module.
4. This data is ,then used to control servos and motors through wired connection.
Step 4: DOWNLOADS
1.NODEMCU FLASHER MASTER:-
i.This is a software which has been downloaded for firmware update.
ii.Firmware is a type of software that provides control, monitoring and data manipulation of engineered products and systems.
2. INSTALLING ARDUINO IDE:-
i.Through this
IDE, Code is written on it, and is uploaded into ESP8266 WIFI Module.
3.Installing the ESP8266 Board via the Board Manager
Step 5: CONFIGURING ESP8266 WIFI MODULE USING ARDUINO IDE
1.Connections With FTDI BOARD For FIRMWARE UPDATE(UART Connections)
2.FIRMWARE UPDATE USING NODEMCU FLASHER MASTER
3.USING "AT" COMMANDS:-
At First, ‘Bare
Minimum Code’ is uploaded.
And then ‘AT’ commands are used for
changing mode ,Baud rate and TCP connection.
AT......OK
AT+CIOBAUD=115200(Setting Baud Rate)
AT+CWMODE=3(Both ‘Sta’ & ‘AP’ Mode)
AT+CIPMUX=0(For single connection)
AT+CIPSERVER=[,port](set as a server)
Step 6: FINAL CODE UPLOAD
#include <ESP8266WiFi.h>
// config: const char *ssid = "mywifi"; // You will connect your phone to this Access Point const char *pw = "qwerty123"; // and this is the password IPAddress ip(192, 168, 0, 1); // From RoboRemo app, connect to this IP IPAddress netmask(255, 255, 255, 0); const int port = 9876; // and this port const int chCount = 4; // 4 channels, you can add more if you have GPIOs :) Servo servoCh[chCount]; // will generate 4 servo PWM signals int chPin[] = {0, 2, 14, 12}; // ESP pins: GPIO 0, 2, 14, 12 int chVal[] = {1500, 1500, 1500, 1500}; // default value (middle) int x=1000; int motor=4; int usMin = 1000; // min pulse width int usMax = 2000; // max pulse width WiFiServer server(port); WiFiClient client; char cmd[100]; // stores the command chars received from RoboRemo int cmdIndex; unsigned long lastCmdTime = 60000; unsigned long aliveSentTime = 0; boolean cmdStartsWith(const char *st) { // checks if cmd starts with st for(int i=0; ; i++) { if(st[i]==0) return true; if(cmd[i]==0) return false; if(cmd[i]!=st[i]) return false;; } return false; } void exeCmd() { // executes the command from cmd lastCmdTime = millis(); // example: set RoboRemo slider id to "ch0", set min 1000 and set max 2000 if( cmdStartsWith("ch") ) { int ch = cmd[2] - '0'; if(ch>=0 && ch<=9 && cmd[3]==' ') { chVal[ch] = (int)atof(cmd+4); if(!servoCh[ch].attached()) { servoCh[ch].attach(chPin[ch], usMin, usMax); } servoCh[ch].writeMicroseconds(chVal[ch]); } } // invert channel: // example: set RoboRemo slider id to "ci0", set min 2000 and set max 1000 if( cmdStartsWith("ci") ) { int ch = cmd[2] - '0'; if(ch>=0 && ch<=9 && cmd[3]==' ') { x = (int)atof(cmd+4); if(!servoCh[ch].attached()) { servoCh[ch].attach(motor, usMin, usMax); } servoCh[ch].writeMicroseconds(x); } } // use accelerometer: // example: set RoboRemo acc y id to "ca1" if( cmdStartsWith("ca") ) { int ch = cmd[2] - '0'; if(ch>=0 && ch<=9 && cmd[3]==' ') { chVal[ch] = (usMax+usMin)/2 + (int)( atof(cmd+4)*51 ); // 9.8*51 = 500 => 1000 .. 2000 if(!servoCh[ch].attached()) { servoCh[ch].attach(chPin[ch], usMin, usMax); } servoCh[ch].writeMicroseconds(chVal[ch]); } } // invert accelerometer: // example: set RoboRemo acc y id to "cb1" if( cmdStartsWith("cb") ) { int ch = cmd[2] - '0'; if(ch>=0 && ch<=9 && cmd[3]==' ') { chVal[ch] = (usMax+usMin)/2 - (int)( atof(cmd+4)*51 ); // 9.8*51 = 500 => 1000 .. 2000 if(!servoCh[ch].attached()) { servoCh[ch].attach(chPin[ch], usMin, usMax); } servoCh[ch].writeMicroseconds(chVal[ch]); } } } void setup() { delay(1000); /*for(int i=0; i 500) { for(int i=0; i 500) { // every 500ms client.write("alive 1\n"); // send the alibe signal, so the "connected" LED in RoboRemo will stay ON // (the LED must have the id set to "alive") aliveSentTime = millis(); // if the connection is lost, the RoboRemo will not receive the alive signal anymore, // and the LED will turn off (because it has the "on timeout" set to 700 (ms) ) } }
#include <WiFiClient.h> #include <servo.h>
About the code:-1.Wifi Module is created as a server in AP mode , SSID and Password is set up and it is connected to the inbuilt WiFi Module of mobile phone.
2.Servos and Motor ID are checked and the data which is received as a string ,is converted into int, which is then fed to servo.Note:-Final code can also be downloaded from the given link named "ROBOREMO.ino".
Attachments
Step 7: CONNECTIONS OF ESP8266 WITH SERVOS AND FTDI BOARD AFTER FINAL CODE UPLOAD:-
1.Once the code is uploaded , FTDI Breakout Board is removed and Vcc & GND is connected to the ESC’s Vcc & GND.
2.Using coded SSID and password , mobile phone is connected to ESP8266.
Step 8: EDITING USER INTERFACE IN ROBOREMO APP:-
Step 9: About the Code
1.Wifi Module is created as a server in AP mode , SSID and Password is set up and it is connected to the inbuilt WiFi Module of mobile phone.
2.Servos and Motor ID are checked and the data which is received as a string ,is converted into int, which is then fed to servo.
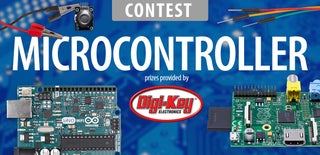
Participated in the
Microcontroller Contest 2017