Introduction: Twitter Sensing Thermoelectric Component
This Arduino project was originally intended to sense when someone tweeted with the word "warm" or the word "cold" and respond to this stimulus by lighting up a corresponding LED and setting a Peltier element (basically a solid state heat pump) to cool or warm the top. I underestimated the frequency of tweets with those words in them, so it was always going off. I decided to alter its purpose a little. Instead of detecting the tweets themselves, it will sample a few and determine which is currently more common. It then does the same action where it lights up an LED and turns on the Peltier element.
SAFETY CONSIDERATIONS:
- My design has an issue by which it can heat up the Peltier element to dangerous temperatures (I have taken readings of nearly 260 Fahrenheit, or 126 Celcius). If you follow my design and don't limit the current, do not touch the Peltier element without taking a temperature reading with a trustworthy instrument (I used an IR thermometer) and taking that in to consideration. Even when the element is not powered, it will be hot if it has been in use for a while.
- That said, by giving less power to the heat pump, we should be able to decrease the maximum temperature it reaches.
- This project uses a considerable amount of power. Use common sense, including the common sense to not attempt a project you don't understand fully.
Step 1: Preparing Your Supplies and Tools
You will need:
Tools:
Computer (which you clearly have)
Arduino Software (free and open source)
USB A to B programming cable (for Arduino)
Small Screwdrivers (potentially, if you use screw terminals on your motor controller or Arduino)
Soldering Iron (potentially, if you need to assemble a Wi-Fi or Ethernet adapter, or if you use a protoboard)
Multimeter (potentially, if things go wrong)
Thermometer (to see what's going on with the Peltier element)
Wire Strippers and Snippers
Materials:
Arduino microcontroller compatible board (I used an Arduino Uno)
Wi-Fi or Ethernet adapter for your board (I used a WiFly Shield)
Motor Controller which can handle at least 7 amps at 12 volts for however long you want this to run, I used the Dimension Engineering Sabertooth 5 amp model, which can handle more current than is advertised, though potentially with a detrimental effect on the unit (extra power output regulated to 5 volts is preferable, to do otherwise is out of the scope of this Instructable)
Peltier element (I used the one Sparkfun sells, but if you use another you will need to adjust things such voltage and current on the motor controller.)
CPU heatsink (just about any will do, but remember to get some thermal conductive gel)
Red and Blue LED (with matching resistors)
A protoboard or breadboard
Solder (If you plan to use a protoboard or need to assemble an ethernet or wifi adapter)
Wire of various gauges
A power supply (I used a computer PSU, but if you want, you could get something else which matches your power requirements)
A little piezo buzzer
Potentially an enclosure (but that's outside the scope of this Instructable)
Step 2: Assembly
Hook up the microcontroller of your choice to the Ethernet adapter of your choice. I did this by mounting the WiFly Shield to the Arduino. Then connect the microcontroller to the motor controller. I did this by wiring digital pin 3 on the microcontroller to the S1 PWM input on the motor controller, the motor controllers 5V out to the Arduino's vin pin, and the 0V pin on the motor controller to the ground pin on the Arduino.
Then attach the fan on the CPU heatsink and the leads of the Peltier element to the corresponding terminals on the motor controller. For me, this meant that the red leads for the Peltier and the fan went to terminal M1A, and the black to M1B.
Next, wire up the breadboard. Attach pin 8 from the Arduino (or whatever you want from your microcontroller) and run it to the breadboard, through a resistor, to the positive side of the blue LED and into the breadboard's ground which should be wired to the microcontroller's ground. Do the same for pin 9 and the red LED. Finally, wire pin 2 of the microcontroller through the piezoelectric buzzer to the microcontroller's ground.
Finally, set up power. This is really up to you. What I did using a computer PSU was bring the green PSU "Power On" wire low by connecting it to a black ground wire. Be certain you connect the right wires, or you could short something out, which would be dangerous. Leave it unplugged for the time being.
Next, connect a wire whose voltage is lower than the maximum voltage of your Peltier element and your motor controller (but not negative) to the positive input to your motor controller and a black ground wire to the negative input on your motor controller. I did a yellow 12V wire for my 15V max Peltier element. IMPORTANT NOTE: use the resistance of your Peltier element and Ohm's law to determine if the voltage you use will cause a current greater than the maximum current of your Peltier element. To do otherwise risks applying too much current, which can fry your component. Make sure you do this with the power turned off on your supply, or better yet, don't plug it in yet.
Step 3: Code
I have attached the Arduino code I used for my project. Note that my code is dependent on the WiFly library available here: http://forum.sparkfun.com/viewtopic.php?p=115626#p115626 .
<code>
#include <_Spi.h>
#include <Client.h>
#include <Configuration.h>
#include <Debug.h>
#include <ParsedStream.h>
#include <Server.h>
#include <SpiUart.h>
#include <WiFly.h>
#include <WiFlyDevice.h>
Client twitter = Client("199.59.148.201", 80); // the libraries included above have an object
// representing a connection to a remote server
String warm1 = "warm "; // the string to search for in the input data representing that the device will warm up
String in = ""; // a string which will remain empty for the time being
String cold = "cold "; // another string to search for
String results = "\"from_user\""; // another string to search for
int index; // a byproduct of messy code, this is part of a system to determine whether or not the post is new
// they rarely aren't
int buzz = 2;
int colds = 0;
int hots = 0;
int S1 = 3; // the output pin to the motor controller channel which controls the
int blue = 8; // the output pin connected to a blue LED
int red = 9; // the output pin connected to a red LED
int oldIndex[] = {0, 0, 0}; // a set of numbers for the index at which various substrings
// appear in the input, they are used to determine whether or not the post is new
void setup() // this function runs once at the beginning
{
WiFly.begin(); // setup the object connected to the Wi-Fi adapter
Serial.begin(9600); // setup the object connected to the serial connection of the microcontroller
// used for debugging the program
pinMode(S1, OUTPUT);
pinMode(buzz, OUTPUT);
pinMode(blue, OUTPUT);
pinMode(red, OUTPUT);
// these were used to set various pins to output
if(!WiFly.join("*******", "*********")) // the wifi object returns true on this function if
// it successfully connects to the network with the SSID and passworrd in the above parameters
{
tone(buzz, 100, 600);
Serial.println("Network not joined.");
while(1) // what's there to do if the connection fails?
{
}
}
tone(buzz, 3000, 1000);
}
void loop()
{
reconnect:
for(int i = 10; i > 0; i--) // get tweets over and over
{
if(!twitter.connected()) // if the server is not connected to, it runs through the connection process
{
Serial.println("connecting to server..."); // used for debugging
if(twitter.connect()) // runs the function, if it is successful returns true and provides debugging information
{
Serial.println("connected");
tone(buzz, 4000, 1000);
}
else
{
tone(buzz, 180, 600);
Serial.println("Connection Failed."); // or if it is false, it will provide debugging information
goto reconnect; // yeah, yeah, goto is frowned upon, I use it anyway
}
}
twitter.println("GET /search.json?q=\"warm\"+OR+\"cold\"&rpp=1 HTTP/1.0\r\n"); // this search string took
// a long time to work out and debug
// see dev.twitter.com if you want more info
twitter.println("Host:search.twitter.com\r\n");
delay(300); // I forget why I put this delay
if(!twitter.available()) // if there is nothing to read, go on ahead and print debugging info, otherwise:
{
Serial.println("Eh. I got nothing.");
tone(buzz, 180, 600);
goto wait_again;
}
while(twitter.available())
{
in+=char(twitter.read()); // repeatedly add the newest character to the input string
}
twitter.flush(); // clear the input from the server (just in case)
twitter.stop(); // close the server conncetion
Serial.println(in); // print this to a serial terminal as debugging information
tone(buzz, 6000, 1000);
Serial.println("New Post...");
if(in.indexOf(warm1) != -1 || in.indexOf(cold) != -1) // make sure that one of the search strings is present
{
if(in.indexOf(warm1) < in.indexOf(cold))
{
hots++; // add one to the hot score
}
else
{
colds++; // add one to the cold score
}
}
}
if(hots > colds)
{
warm(); // warm it up if hot won
}
else
{
cool(); // cool it down if cold won
}
wait_again:
oldIndex[1] = in.indexOf(cold);
oldIndex[2] = in.indexOf(warm1);
delay(10000); // delay so we aren't bombarding twitter with queries
in = ""; // clear the input string
colds = 0; // clear the hots and colds
hots = 0;
for(int i = 40; i > 0; i--) // this loop is just telling the motor controller to stop letting current through
{
digitalWrite(S1, HIGH);
delayMicroseconds(1500);
digitalWrite(S1, LOW);
delayMicroseconds(1500);
}
}
void warm()
{
for(int i = 40; i > 0; i--)
{
digitalWrite(S1, HIGH);
delayMicroseconds(1100);
digitalWrite(S1, LOW);
delayMicroseconds(1100); // start sending current in one direction (heating the top of the plate)
}
digitalWrite(red, HIGH); // turns on LED
delay(50000); // for 50 seconds (50000 milliseconds)
digitalWrite(red, LOW); // turns off LED
for(int i = 40; i > 0; i--)
{
digitalWrite(S1, HIGH);
delayMicroseconds(1500);
digitalWrite(S1, LOW); // stops the current
delayMicroseconds(1500);
}
}
void cool()
{
for(int i = 40; i > 0; i--)
{
digitalWrite(S1, HIGH);
delayMicroseconds(1900);
digitalWrite(S1, LOW);
delayMicroseconds(1900); //start sending current in the other direction
// (cooling the top and warming the bottom, which is dissipated by a heatsink)
}
digitalWrite(blue, HIGH); // turns on the LED
delay(50000); // for 50 seconds
digitalWrite(blue, LOW); // turns off LED
for(int i = 40; i > 0; i--)
{
digitalWrite(S1, HIGH);
delayMicroseconds(1500); // stops the current
digitalWrite(S1, LOW);
delayMicroseconds(1500);
}
}
</code>
Attachments
Step 4: Try It Out
Give it a go!
Make sure you set the code to your wi-fi network information.
Assuming nothing blows up, you should start hearing beeps and seeing flashing lights. If you don't think it's working, try using the Arduino serial monitor to check the debugging information from the Serial output of the code.
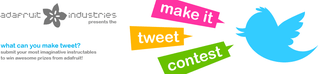
Participated in the
Adafruit Make It Tweet Challenge