Introduction: Understanding the Laser Shooting Game
This simple but somewhat complex game was designed to be our coursework, and our enjoyment aswell! It was based on microchip microcontrollers 16F628A, as our teachers forbade the Arduino, in order to avoid community-made projects. However, this project is quite easy to reproduce using Arduino, but a lot of adaptations might be necessary, demanding some intermediate programming and electronics knowledge.
This game is made with 4 targets, which contains each one a indicator LED and a small LDR. The objective is to hit the randomly selected target with a laser gun, then try to hit as many points as possible.
We made this instructables to serve as a inspiration or a guide about understanding how this kind of project can be done, so we might not post the exact layout or scripts. But leave us a comment if you want some help!
Some useful questions about this guide:
So why didn't you guys use the Arduino???
As mentioned, the Arduino is known for its quite big community behind it, and a coursework should be done by the students themselves. Using it could jeopardize the authenticity of a project, thus turning obsolete all the reason behind the project, so this marvel platform could not be used.
Also, the PIC itself is so much cheaper than using a full Arduino Board or the ATMEL chip itself, though it has much less potential.
So why are you guys sharing it on Instructables anyway?
Because we love electronics as much as you guys love, so to make it community friendly, we're adding a lot of tips to the guide, so you guys can reproduce it if you want (and hopefully share with us!). ALSO, to make it as a general guide to any electronics project, we will post lots of logical thinking behind our hardware and software choosings. And also add any tips you guys find helpful, this is a beatiful community after all!
Still, this is not meant to be a step by step guide to reproduce the project, because it would need some heavy adaptations to make it Arduino friendly.
If there's any doubts, questions, critics, confessions, love messages, just post them below! :D
Step 1: Dealing With the Display
Problem: We needed to add a 7-segment display in our project, and control 4 of them using 2 different microcontrollers, this meant that each one should deal with 14 LEDs to control, but they have only 14, leaving them without any left pins for the peripherals, how can we use them?
Solution: There are some well known solutions for this problem, we used a shift-register and some BCD to 7-segment display converter to deal with the display, using only 3 pins!
Why?: The other well known solution is to use some multiplexation, the explanation of both goes as follow:
How can you deal with 14 bits using 3 pins???
It is quite simple actually, think it as this way: controlling 14 LEDs mean it will have to use 14 pins, BUT it could not care about all of them at the same time. -Say what?
You can send them as a serial data, which works much like a teacher reading a text out loud, while the students write then down with a pencil. The controller works as the teacher, it will tell how the LEDs should be each one at a time, like its human counterpart, it will tell what word should you write at each time. But as a disadvantage, you will have to work in a circuit, which will work as the student, who listens and writes. Without the circuit, you should not expect to the LEDs to work by themselves as much the pencils writing likewise.
The communication works with 3 pins, one is obviously the one which tells how the LEDs will be, AKA DATA, but another two are very important as well. The another one is the Shift Clock, the one which synchronizes the circuits, telling how fast the data is being sent. Last and maybe the least, the Latch Clock, that might also not be present, is the one used to tell "-Hey! You can show how the display looks, just like I told you, OK?" And it is needed because specially the IC we're using (and most of the people), does receive the data immediately, but does not show then just yet, it NEEDS to be told to do so, that's what the third one does.
The advantage of using the circuit with the third pin is that it holds for you the number you just sent, thus, you can just print the number when you what, see?
The multiplex solution is also used, but in our case seemed like a risky and not interesting path. It does need the "student" circuit, but it does not hold it for you, which means you'll have to keep sending him what it looks like all the time, which might lead to use of timer interruptions (techy-techy stuff), which is not quite necessary. There are some other reasons but moving on...
They send to each display 4 bits, which is logically enough to lit 4 LEDs, but we will use some decoders, the one that actually reads them as a Binarry Code Decimal (BCD), reading 0010 as 2, and 1001 as 9. It will decode them to an actual 7-segment display, which is finally our goal. *whew*
Step 2: Building the Display
We could not (or maybe did not even try), to find a already-made library to drive our displays to victory, so we read the datasheet for 74595 (popular model of shift-register), so we made it ourselves (kind of). It's not veeery complicated as its behavior is rather simple. So what as myself did on this step is to tell how should the "teacher" tell its "student" the numbers. It works like this:
I tell: Number is 42!
The program thinks:
-It is made of digits 4 and 2. And in BCD they are 0100 and 0010.
-I should print this sequence: 01000010.
-The data pin should be 0, sets it and pulse the shift clock to send it.
-The data pin should be 1, sets it and pulse the shift clock to send it.
-The data pin should be 0, sets it and pulse the shift clock to send it...
-(After sending the last data), pulses the latch clock to confirm it.
Done.
As a challenge, you can write the script equivalent in Arduino IDE. I had some fun myself with it. But with you want to skip the jibber-jabber, you can use the function shiftOut() from Arduino IDE. It will send everything alright, but it will not do the first two steps, which can be done with some loops and ifs.
Also, we've etched a dual layer PCB to hold the displays and the ICs. We tried to avoid a dual layer, but it seemed like more elegant solution than adding 10 jumpers to the board. After some trial and error, we got a functional board.
Step 3: Dealing With the Lasers
Problem: Making a laser shooting game with just a plain laser pointer is not as exciting. Mostly because the pointer itself does not feel like a gun, and does not even behave like one. Building the game should take another step then just using a plain laser pointer from a souvenir shop.
Solution: Making a gun with the laser is just a matter of crafting skills, there are lots of room for creativity. We actually disassembled a old Playstation 2 gun and glued the laser inside. Also, the gun would actually shoot the laser in pulses like a good sci-fi blaster laser with a simple electrical circuit, the second circuit board of our project.
Why?: Because we suck at crafting stuff like 3D models, if you are good enough to make a gun model with a 3D printer (lucky you!), go for it! Also, the laser circuit is easy peasy, and a very good project for a electronics newbie.
How can you make a laser behave like an actual blaster???
Think it simple: how does a laser blaster work? You press is once, it just pulses a *pew*, and you press it again to *pew* again. As you can see, it should time the laser, so it can just pulse and rest afterwards, even if you hold the trigger.
Did I say time? Sure I did! The solution for most timing issues is the heavenly 555 timer. So we should look for a schematic for a 555 circuit which would work as follows:
- When triggered, it will pulse the output;
- Have a little cooldown time, so it cannot be spammed;
Why it should have a cooldown time? Because we wanted to make the game a precision task. If the cooldown time were absent, the player could just spam the trigger effortlessly and just have a all-time on laser gun to just point and shoot. Sure it wouldn't have to be like that, but that's how we planned it.
The game is lots of fun with a laser pointer actually, I lied shamefully just so you would read everything. But you know, it's all about crafting cool stuff, and I want everyone to make cool stuff, yip.
Step 4: Building the Lasers
So the projects should work like this: the board is actually outside the weapon, as it will probably not fit inside, and the gun itself will contain the laser and a button activated by the trigger finger.
So the board, how it should be done?
->If it needs to be triggered by a button and just pulse once, it will probably be on monostable mode. And it is called monostable because it has only one (thus mono) stable state, the other one is unstable, as it will commute soon. -Say what?
By stable state, we mean a state in which it just stays put, it does not change without a interaction, therefore, a unstable state must be the one that changes by itself sooner or later. It should work with a monostable because the low state (no *pew*) is very stable, it will not change until I press the button. And the high state (many *pew*), should be unstable, as it will soon change to low state to make it just a pulse. Got it?
By extension, a bistable mode would be unsuitable because it would have to be pressed both to turn on and off. And this would make us just go back to a regular laser pointer with a toggle switch. Likewise for astable, as it will just swap constantly, pulsing without your control, like a laser SMG with a broken trigger, which might sound great but this is a precision game, it should be like a sniper gun.
->Also, adding a small capacitor on the input will make the board susceptible only by the pulse, making the act of holding the trigger to get lasers all the time for no avail. It works because magic, and electronics, let's just say that the capacitor will gladly pass the current when the voltage is changing, but if the voltage just stops changing, it will not let any current pass. It's a AC coupling. If this capacitor were missing, you could just keep the trigger pressed, and it would just keep shooting at the maximum rate, no fun no fun.
->And the last part, cooldown. It's the easiest to think but the one which actually makes the board rather complicated. How to make the gun not responsible by a short time after the *pew*? If I just said time, I will gladly just say that you should add another 555 for another timing. And how will it disable the first 555? All 555 circuits have a kill-switch called reset, as long it is grounded, the timer will also be grounded. So we just add a transistor to ground the first timer's reset button by the second timer. Done!
Sidenote: Some of you guys know that using two 555 might lead to the 556 IC, which is just the same with double in the same package, like two bananas in the same peel. It would probably be the best to use it, but I was afraid that we could not find it, and everyone sure have plenty of 555 timers, so we used two of them anyway.
Step 5: Dealing With the Mainboard
Problem: We need to figure out the components the microprocessor (or Arduino) in order to make this project work, what do we still need?
Solution: This is the part we started to cry. Beacuse it would be so much easier with Arduino, but we could not manage to make our microcontroller read the voltage as a analog data. This is simple with Arduino, which you use the analogRead() command, but this wasn't as accessible for us. So we made our own circuit that can read analog data. How?
With comparators, not unlike the ones from Minecraft, they can compare voltages, in our case, if the LDR is lit enough, the comparator will switch off. This can be programmed just like a analogRead if we control the voltage level sent to the threshold, which we done by adding a voltage divider and a trimpot.
So 4 comparators we added in a board with 2 controllers, one dealing with timing and the other for scores. Maybe just one could do the trick, but we would have to use interruptions (we-just-hate-this-stuff-at-that-time), and also, making it modular could mean a easier repair [citation needed].
Adding connectors is also a good habit, as it lets you screw or snap out the connections without desoldering.
Why?: Well, connectors are pretty cheap, and makes the project look much more professional with very little effort. But it's not all about looks, the connectors let you snap out the wires with a screwdriver, otherwise you would have to desolder them, that's just horrible. There's nothing more heartbreaking than a copper node that got loose after some intense desoldering.
Also, you could join the last laser board in a bigger board, but you know, sometimes being modular is good.
Step 6: Building the Mainboard
So here's the deal: If you were to do this project, you could just skip all this stuff about comparators, because Arduino already has them inside, the well known analogpins. But you can still see how beautiful it turned out to be. A friend of ours has made this PCB to using a CNC. As you might already know, it's basically a drill that will remove the copper mechanically (not chemically like corrosion), it also makes the holes to fit the components and the silk screen at the top, indicating the component placing, gotta love technology!
There's not much to say about it anymore, just a bunch of comparators, the brains, and the connectors.
Step 7: Results
At last, the project presentation and results went prettty well!
Sorry if the video does not focus on the game itself, its much more oriented to the presentation itself (or maybe it has no focus whatsoever).
I hope you liked the long read!
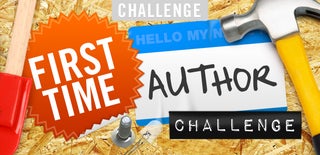
Participated in the
First Time Author Challenge