Introduction: Upcycle Brushless DC Motors From Printers
If you are at all interested in robotics and electronics you will probably have disassembled an old printer or two (if you haven't, I highly recommend it, there are always interesting parts, and you can learn a lot about how the experts put electro-mechanical machines together). If you have taken a laser printer apart, you will likely have come across brushless DC motors, which range in size.
These motors have some pros and cons, obviously it varies between printers, and the functions of the motors within the printers, but I have found the following to be true most of the time.
Pros
- They normally have an integrated ESC (electronic speed control) circuit, which means that you can control them with logic, rather than needing an external ESC or H-Bridge
- Some have a collection of gears that can be re-used fairly easily
- Some have an RPM output
Cons
- Usually designed around a 24V supply
- Relatively low power/torque
The motors normally have about 5 or 6 input pins, I will go through the process that I use to determine which pin is which, and demonstrate some code to control the motors.
Step 1: Salvage Some Motors
The first step of course is to tear down a printer and see what kind of motors you can find.
There are a few ways to tell that the motor you have is a BLDC, as opposed to a stepper or brushed-DC motor.
- The motor windings have three connections (a brushed motor will have only two)
- When you turn the motor it turns smoothly, there isn't significant "cogging" as there is with steppers (this is not universal, since powerful brushless motors for RC toys tend to have strong magnets and exhibit a strong cogging effect, but it does hold true so far for all the printer motors I have played with)
Coincidentally all of the motors I have recovered so far have been from HP printers, but I expect they would be very similar from other manufacturers. The motors in this particular Instructable are from an HP Color LaserJet 3000 series.
Step 2: Determining Pinout and Protocol
Finding Documentation
After some digging I discovered that googling "HP Printer service manual" invariably brings up some pretty decent documentation.
The easiest way to find pertinent information in the hundreds of pages is to search for references to "motor" and then go from there.
In the images I have attached some diagrams that I pulled from the service manuals.
Deciphering the Documentation
The control signals are pretty simple (I am using 5V logic, which it seems happy with), this is what I was able to find by reading the documentation and scouring the internet.
- /ACC requires a PWM for speed control
- /DEC is a "brake" (I think of it as an enable pin)
- REV controls direction (high is one way, low is the other)
- FG is an output, it appears to output one (or two) pulses per revolution
Remember that there are all kinds of motors, some have no speed control or ability to reverse, depending on their function.
Determine Which Pin is Which
Obviously the first step is to metabolise any documentation that you can find. I find it helpful to print the diagrams out so that I can scribble notes on them.
The next thing is to find the +24V and GND pins. These should be pretty easy, there is normally an electrolytic capacitor across the power lines, you can look for the side with the stripe to determine which is is negative.
Now that you know which these pins are, you can use the diagram to determine the rest of them. If you didn't have a diagram, then I would try to apply power on +24/GND pins and pull all the others low via 10kOhm resistors. Then go through each one in turn, pulling it high (+5V) via a 10kOhm resistor. Once you find the pin that makes the motor turn, you will know that you have found /DEC. The next step would be to remove the grounded pins one at a time until you found the one that made the motor stop, that would more than likely be /ACC.
At this point we need to involve a microcontroller (unless you have a signal generator) to put a PWM onto /ACC and REV to start controlling the speed and direction.
Step 3: Speed Control Code
In the previous step we determined which were the following input pins on the motor
- /DEC (NOT decelerate, so pulling it high causes the motor to run)
- /ACC (NOT accelerate, so 100% duty cycle is 0 speed)
- REV controls direction (high is one way, low is the other)
- +24VDC
- GND
I have used the MediaTek LinkIt One or Arduino boards for this step, since all of the available libraries make it quick and easy. Since this is not exactly a beginner's project I am going to assume that you know how to upload code to them (if not, check out the getting started guide).
This code uses the analogWriteAdvanced function in order to work with high frequency PWM, which my motor wanted.
On Arduino boards in the past I have used the TimerOne library to make the PWM setup easy.
This code simple provides an interface for testing, connect to the board via a serial connection and you will be able to control the motor by sending the following characters
- 'w' to increase speed
- 's' to decrease speed
- 'd' to toggle the brake on or off
- 'r' to toggle direction
//define the pin numbers
int accPin = 9;
int decPin = 13;
int revPin = 10;
int fgPin = 12;
//here we store the current pin states
int accLevel = 0;
boolean decState = LOW;
boolean revState = LOW;
//some PWM variables to increase the frequency
//You may have to mess around to find a freq that your motor likes
int cycle = 1600; // Divide output into 9+1 = 10 portions
int sourceClock = PWM_SOURCE_CLOCK_13MHZ;
int divider = PWM_CLOCK_DIV8; // The PWM frequency will be 13MHz / 8 / 10 = 162.5KHz
void setup() {
//start a serial port
Serial.begin(9600);
//setup the pins
pinMode(accPin, OUTPUT);
pinMode(decPin, OUTPUT);
pinMode(revPin, OUTPUT);
pinMode(fgPin, INPUT);
//make sure motor doesnt start up right away
digitalWrite(decPin, LOW);
}
// duty: 0-100
void setPwm(unsigned char duty)
{
int __duty = map(duty, 0, 100, 0, 1600);
analogWriteAdvance(accPin, sourceClock, divider, cycle, __duty);
}
void loop()
{
if (Serial.available() > 0) {
char inByte = Serial.read();; //incoming serial byte
if (inByte == 'w') {
//accLevel = LOW;
accLevel = min(accLevel + 10, 100); //100% PWM is zero speed
}
else if (inByte == 's') {
//accLevel = HIGH;
accLevel = max(accLevel - 10, 0); //0% PWM is full speed
}
else if (inByte == 'r') {
//reverse direction
revState = !revState;
}
else if (inByte == 'd') {
//brake
decState = !decState;
}
Serial.print("revState: ");
Serial.println(revState);
digitalWrite(revPin,revState);
Serial.print("decState: ");
Serial.println(decState);
digitalWrite(decPin,decState);
Serial.print("accLevel: ");
Serial.println(accLevel);
setPwm(accLevel);
//digitalWrite(accPin,accLevel);
}
}
Step 4: Where to From Here
Now you know how to control the BLDC motors from printers you can start building things. The motor's don't tend to have a lot of torque, but if you make use of the copious gears that are available in a printer then they will definitely be usable in small robots etc.
A colour laser printer tends to have four identical motors with four identical sets of gears, which are just crying out to be turned into an all-wheel-drive robot.
If I build one you can rest assured you will see it here!
If you have any questions, please comment and I will try to clarify, I expect there is a lot more for us to learn about using these salvaged motors to their full potential.
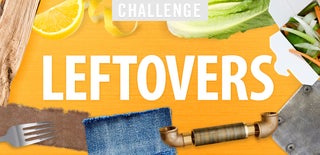
Participated in the
Leftovers Challenge