Introduction: Use of ServoTimer2 and Servo Sweep.
I've been trying to make a humanoid robot recently- which means dealing with Servo motors. Everything worked just as fine just before i tried to make the robot TALK. When i needed to use the TMRpcm library. But there's some libraries like
#TMRpcm.h
#VirtualWire.h
etc libraries that uses the Timer1 of arduino, it appears that you cant use two devices simultaneously where both use the same timer.. So, if my robot talks- the servoes don't work.Because The Servo.h and the TMRpcm both works on arduino TImer1. Which is a mess.If you want to make both of them work you have to use another library for servoes,Which is ServoTimer2 library.This uses the Timer2 on arduino..Unfortunately on internet i haven't find any tutorials to understand how this ServoTimer2 library actually works and how to use it in code. So I've decided to make a tutorial so that people like me can understand better. We'll be using one servo motor with this library and make a simple Servo sweep code
You may watch the video to understand better.
Step 1: Parts & Assembly:
Main part is a Servo motor.
I'm using a micro servo sg90,any model is okay.
Then take a servo arm just like in pic2 and glue it on a hard cardboard (this is just to get a good visibility of degree) and then add the arm to the servo motor like in pic4.
Buy electric components on utsource.net
Step 2: Download and Add the ServoTimer2 Library to Arduino
First of all you'll have to download the library from here https://github.com/nabontra/ServoTimer2 and paste it to arduino library folder.
then goto sketch>include library>add zip.file from the library folder.
Now you're good to go.
Step 3: Build the Simple Circuit
This is the most easy part
Servo red wire to arduino vcc (5v)
grey - Gnd
Orange- digital 6
Step 4: The Code.
Before we get to know the ServoTimer2 code lets look back at Servo.h library sweep.
(the motor will rotate from 0 degree to 90 degree - wait for 1 sec- then to 180 degree-wait for 1 sec)
#include<Servo.h> Servo servo1; void setup() { servo1.attach(6); // put your setup code here, to run once } void loop() { // put your main code here, to run repeatedly: servo1.write(0); delay(1000); servo1.write(90); delay(1000); servo1.write(180); delay(1000); }
As you can see in this library if we wish to rotate a Servo to a certain position we had to just write the degree and the servo would do just fine. But in ServoTimer2 library we must write the Pulsewidth of the servo and the servo moves to that position using that. Most commonly 1500 means 90 degree. The maximum pulsewidth is 2250 and minimum is 750. Which would mean 750 is for 0 degree and 2250 is for 180 degree. But let me tell you, this varies from servo to servo. Just send in your values and see what happens. Don't worry this wont destroy or harm your servo motor a bit.
Now I'll make the same code for ServoTimer 2 and thus you'll get what actually the differences are.
code link https://github.com/ashraf-minhaj/Arduino-ServoTimer2-basic-sweep-/tree/code-sweep
#include"ServoTimer2.h" ServoTimer2 servo1; void setup() { servo1.attach(6); } void loop() { // put your main code here, to run repeatedly: servo1.write(750); //min pulse width for 0 degree delay(1000); servo1.write(1500); //pulse width for 90 degree delay(1000); servo1.write(2250); //max pulse width for around 180 degree delay(1000); }
This did work for me on arduino Uno and Arduino pro mini. just experiment with your values and see what happens. You may need to make the values like 500 or higher to 2700 to get it done.
Step 5: Last-Power Up the Arduino and Experiment
Now just power the arduino and see with a degree scale that for which value how much the servo rotate. And buy doing this you can make your project go. Happy Making.
If you like my work please Subscribe to my Youtube channel
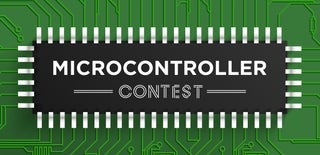
Participated in the
Microcontroller Contest