Introduction: WIFI Display for Production Management
I am little bit Series about IOT and Single board computers.
I am always wish to use it beyond Hobby & Fun Projects (real Production and Manufacturing).
This Instructable is about to Creating 4 digit 7-segment WIFI display with ESP nodemcu to Show hourly Production Input. I’m Working in Electronics manufacturing industry, where we use Manufacturing Execution System (MES) to Monitor & Control Production Floor Input, Output & Process. In this project I’m Creating Small display unit which will show the Production input Quantity as per line, shift and hour.
In technical this Project is similar to a Youtube Subscriber count display, where we use API/HTTP response from online.But here we are going to create our own api to interact with our local MES system to Get Input quantity.
Step 1: Parts & Tools Used:
Hardware Parts Used:
- ESP nodemcu
- TM1637 4 digit clock Display
- Push switch
- 10k resistor
- few jumper wires
Software Tools Used:
- Arduino IDE
- Xampp for PHP/Apache web server
Arduino Library's Used:
1. Wifi manager by tzapu & i Customized for my custom fileds (wifimanager)
2. ESP_EEPROM for storing my custom values in Flash memory
3. SevenSegmentTM1637 For Display
Step 2: Customizing Wifi Manager
In this First i installed wifi manager first and then i Copied the Wifi manager Folder and passed again in the Same in the Arduino library folder, then renamed as WiFiManager_custom.
Folder Root directory Mostly like
C:\Users\your computer name\Documents\Arduino\libraries
Then i opened wifimanager_custom folder and renamed header .cpp file as same as wifimanager_custom,added same in the header & .cpp files also.
And Added my Custom Form & Button in header.
in HTTP_PORTAL_OPTIONS[] PROGMEM i added my button form for Menu.
and added new Form for entering line & shift. i created this form as simple text form.
After this we are going to create action functions for this forms in .cpp file, for that we have to do function declaration in header file.
/* my custom functions */
void handleCustomForm(); void handleCustomSave();
i declared my custom functions in header file. that it , our work in header is finished we have to go with .cpp file to create our function & actions.
Step 3: Custom Functions for Form Action
Now we open our wifimanager_custom.cpp file.
and we have to add our http response handler to call our functions when our form is post.
server->on(String(F("/custom_config")), std::bind(&WiFiManager::handleCustomForm, this)); // MY custom handle
server->on(String(F("/custom_save")), std::bind(&WiFiManager::handleCustomSave, this)); // MY custom handle
these will call our custom functions when the form is posted.
1.handleCustomForm()-> will create a page with our custom form for line & shift input & save button.
2.handleCustomSave()-> this function will get the form values & store in the Flash memory locations 0 (line)& 50(shift).
Step 4: Connections & Main Program
Connections are very simple..
Connections and wiring:
nodemcu TM1637 Display
3.3v ------>Vcc
G ------>Gnd
D2 ------> CLK
D3-------> DIO
nodemcu---> push switch
- pushbutton attached to pin D8 from +5V
- 10K resistor attached to pin D8 from ground
we finished customizing our wifimanager. now we have to create our main program.
1. our wifi manager will Connect with the wifi network with last used credentials to connect , if it fails it opens a AutoConnectAP wifi server.we can configure new wifi cridentials, line& shift by connecting to this wifi server.
2. then it will enter in to main loop.
Our main loop will contain two part. one is confi subroutine when we need to change line,shift or add any wifi credential to call demand mode AP to configure. this will be called when a push button connected to the D8 pin is Pressed.
void loop()
{
config_loop();
----------------------
-----------------------
}
void config_loop()
{ Serial.println("");
Serial.println("Waiting For Config button Status...");
//display.print("Wait");
if ( digitalRead(TRIGGER_PIN) == HIGH )
{
display.print("Conf"); //WiFiManager
//Local intialization. Once its business is done, there is no need to keep it around WiFiManager wifiManager;
//reset settings - for testing
//wifiManager.resetSettings();
//sets timeout until configuration portal gets turned off //useful to make it all retry or go to sleep //in seconds
//wifiManager.setTimeout(120);
//it starts an access point with the specified name
//here "AutoConnectAP" //and goes into a blocking loop awaiting configuration
//WITHOUT THIS THE AP DOES NOT SEEM TO WORK PROPERLY WITH SDK 1.5 , update to at least 1.5.1 //WiFi.mode(WIFI_STA);
if (!wifiManager.startConfigPortal("OnDemandAP")) { Serial.println("failed to connect and hit timeout"); delay(3000); //reset and try again, or maybe put it to deep sleep ESP.reset(); delay(5000); } }
//Serial.println("Button status False.Back to Main loop"); //display.print("Main loop"); //display.clear();
}
Second one will be our main program to get HTTP response from particular server & Display the input quantity in the Display.
For this First we have to get our line & shift detail from the Flash storage of ESP (address 0-> line ,50-> shift)
EEPROM.begin(100); // eeprom storage
EEPROM.get(0, line); // get Value from address 0
EEPROM.get(50, shift); // Get Value From address 50
then we have to pass this line & shift details to our http server by get method to get the value of input & output.
String Base_url="removed"; // my base url
HTTPClient http; //Object of class HTTPClient
String URL=Base_url+"?"+"line="+line+"&shift="+shift;
Serial.println(URL);
http.begin(URL);
int httpCode = http.GET();
Serial.println(http.getString()); // this will print all http response string ;
if you want to how all the text then your work is finished here it self we can directly display it in tm1637 display.
display.print(http.getString());
But i dont want to show all the text, because it contain input,output in json form & some other general text about its database & etcs.
so first i removed that general text from the response string by using Substring() function.
i counted length of general text and trimed it.
if (httpCode > 0)
{ const size_t bufferSize = 100; //DynamicJsonDocument jsonBuffer(bufferSize); DynamicJsonDocument root(bufferSize);
//JsonObject& root = doc.parseObject(http.getString());
String json_string=http.getString().substring(121); /* this is my offset of general text if your responsee dont have any thing like that you can remove this code; */
//Serial.println(json_string);
DeserializationError error = deserializeJson(root, json_string);
//JsonObject& root = jsonBuffer.parseObject(http.getString());
if (error)
{ Serial.print(F("deserializeJson() failed: "));
Serial.println(error.c_str());
return;
}
else{
const char* input = root["input"];
const char* output = root["output"];
Serial.print("Input:");
Serial.println(input);
Serial.print("Output:");
Serial.println(output);
display.print("..in..");
display.clear(); // clear the display
display.print(input);// print COUNTING SOME DIGITS
}
thats it our main program is finished.
Step 5: Creating Web Server
i m Using xampp as my web serve & PHP code to get data from my SQL database to get exact quantity.
But i cant share all original codes of it. because its confidentiality of my company. but i will show the how to create one web server , show dummy static input & output quantity.
For this you should need any web host, i m using here xampp as my host.
you can download xampp here.
install xampp...if you need clear instruction you can use this link.
After installing xampp you have to go to your root directory.
C:\xampp\htdocs\
all your php programs should be inside of this root.
i created my page in the name callled esp_api.php
this is my php code. here i m just displaying static values of input & output;
$line=$_GET['line'];
$shift=$_GET['shift'];
echo ("myString"); //general Text
if($line=='a0401' and $shift='dd') { $result['input']=100; $result['output']=99; }
else { $result['input']=200; $result['output']=199; }
$myObj->input =''.$result['input'].'';
$myObj->output =''.$result['output'].'';
$myJSON = json_encode($myObj);
echo $myJSON;
Now our HTTP response API is finished.
Our http base url will be like
you_ip_address/esp_api.php
you can check your API response text by
http://localhost/esp_api.php?line=a0401&shift=dd
here i mentioned line as a0401 and shift as dd.
Step 6: Final Step!!!
Enter your computer ip address in Base URL
String Base_url="removed"; // your base url
and Upload to your ESP nodemcu. Once you done just turn on your wifi from your mobile or laptop, you will get network called AutoConnectAP. connect with it and enter your credential & line configs.
Then reset your device & check in your network is connected once it connected then every thing is done.
You can see the input is displayed in the display.
if you want to change any line or wifi credential you can press push switch for few seconds, display shows confi.
you entered in to demandAP mode. your can change & reset device.
The main moto of his instructable to show you how we can use our hobby & fun projects in the real production & manufacturing area and show
IOTs & single board computers are Cheap in Price Not in Performance.
Not Limit to IOT's.
Please share your commands & Feedbacks...
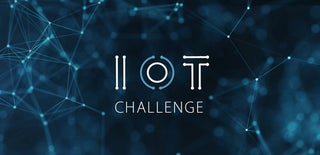
Participated in the
IoT Challenge