Introduction: Wake Me Up - a Smart Alarm Clock
Wake me up is a smart alarm clock that can also be used as a smart light.
The built-in ledstrip simulates natural light coming into your room. This enables a calm, natural way to get your day started.
The alarm clock is also equipped with a 4*7 segment display for reading the time, speakers for waking up with your favourite music, a touchbutton, a ldr for adapting the brightness of the ledstrip and a temperature sensor which you can use to view the temperature of your room.
Attachments
Supplies
A list of the exact prices I paid can be found here:
Microcontrollers and computers:
- Raspberry Pi 4I used the raspberry pi 4 4GB however, any raspberry pi model 3+ should be fine.
- Arduino Uno
The arduino is used to control the 4*7 segment clock display.
Sensors:
- TMP36: temperature sensor
- LDR: Light dependant resistor
Actuators:
- WS2801: Individually adressable ledstrip
- LCD display: A 16*2 LCD display.
- 4*7 segment clock display
IC's:
- 74HC595: Shiftregister for the lcd display
- MCP3008: 8-bit Analog to digital converter
- Logic level converter: Used to communicate between raspi and arduino
Other stuff:
- An adafruit MAX9744 amplifier to power the speakers
- Any speaker, I used a Visaton 4Ohm 8Watt full range speaker (Art. No. 2240)
- A 9volt power supply to power the amplifier
- A 5volt power supply to supply the ledstrip and other components.
Keep in mind that each led in the ledstrip can drow 60mA so make sure your power supply is sufficient. - A few 220Ohm resistors
- A small breadboard to put in your case.
Tools:
- A soldering iron.
- Something to make the case (I used a 3d printer with PLA and PETG and some vinyl stickers to get the wooden effect.)
Step 1: Setting Up the Raspberry Pi
The raspberry pi is our main micro controller.
The raspberry pi runs our local webserver, database, controls the ledstrip, speakers,...
Part 1: Installing Raspbian
Use this tutorial to install raspbian: https://www.raspberrypi.org/documentation/installa...
Make sure SSH is enabled
Part 2: Getting connected
In order to get connected to the internet, you need to get acces to your raspberry pi's terminal. I recommend using putty. In the terminal type:
wpa_passphrase "YourNetwork" "YourSSID" >> /etc/wpa_supplicant/wpa_supplicant.conf
"YourNetwork" is the name of your wifi network and "YourSSID" is the password of the network.
Once you've added the network try to reboot the Raspberry Pi.
Type in the command 'ping 8.8.8.8' this wil send a packet to the google servers if you get response your network is set-up and working!
Part 3: Install the needed programs
We're gonna need some install some extra programs to get this project up and running.
Before we get started run these 2 commands to make sure that everything is upgraded.
sudo apt update
sudo apt upgrade
This might take a while.
Apache
sudo apt install apache2 -y
sudo apt install php libapache2-mod-php -y
MariaDB
sudo apt install mariadb-server mariadb-client -y
sudo apt install php-mysql -y
PHPMyAdmin
sudo apt install phpmyadmin -y
Python pip
We need to install pip to enable some python libraries
pip3 install mysql-connector-python
pip3 install flask-socketio
pip3 install flask-cors
pip3 install gevent
pip3 install gevent-websocket
Step 2: Connecting the Electronics
I've added 2 schemes, 1 is a breadboard scheme for testing purposes. I recommend building the first scheme and trying to get the code to work.
I've added the fritzing files below.
Attachments
Step 3: Database Design
This is the database scheme I made. The words are in dutch but I will explain each table in detail.
Table 1: tblMuziek
This a pretty basic table. It saves the artist name, song name and the filename of a song.
Table 2: tblLedstrip
This table keeps track of the current state of the ledstrip it saves the state of the ledstrip. We need this for the smartlight function.
Table 3: tblSensoren
This table keeps track of the sensors in our alarm. We store the name of the sensor and the MCP3008's channel
Table 4: tblMeting
This table stores the values of our sensors together with their time.
Table 5: tblWekker
This table stores the password and name of your alarm clock (e.g. Bedroom) This table is not mandatory but I added it because I assume that you'll have more than 1 alarm clock in your house.
Table 6: tblAlarm
This is probably the most important table. It keeps track of the alarm's you've set and what the clock has to do (which song to play, which led sequence, On what days should it go off,...). It is very import to keep track of 2 dates. 1 date is used to store at what hour the alarm should go off. The other one keeps track of the last time the alarm went off. To know what weekday it should go off I used a varchar that contains a number of 7 digits. The first digit is Monday, the second Tuesday,... If it's a 1 then it should go off, if it's a 0 it shouldn't. Example: 1111100 this means that this alarm should go off on Monday, Tuesday, Wednesday, Thursday and Friday.
Step 4: Arduino Setup
This step is very easy. There will be a link to my github at the next step where you can download the arduino file.
What does the program do?
The program waits for serial data to come in from the pi.
As soon as the data is recieved it parses throught the string and sends back a confirmation to the pi.
The string will be something like this: '1201' this means that it's 12:01. I used a common anode 7segment display this means that the Digits should be high and the A,B,C,D,E,F,G and DP should be low to turn them on. If you use a common cathode you should just change HIGH to LOW & LOW to HIGH.
Here's a link with more info about how 7 segment displays work. (with the use of library):
https://www.instructables.com/id/Using-a-4-digit-7...
Here's a link about 7 segment displays without the use of a library:
https://create.arduino.cc/projecthub/SAnwandter1/p...
.
Step 5: Raspberry Pi Backend
You can download my code using Github. (https://github.com/VanHevelNico/WakeMeUp)
How to install the program:
The backend is written in python using flask. You can make a service that starts this program (app.py) automatically.
You should put the frontend code in the html file of the apache server we downloaded earlier. (/var/html)
How does the program work?
When the alarm clock is turned on go to your clock's ip adress (it will be displayed on the lcd)
As soon as you go to that ip adress in your browser your computer wil send a socket.io request to the backend saying that a client has connected. When the backend recieves this a few threads will start which I will explain below.
Setup
This intializes all the objects needed.
GetTemp
This reads the mcp3008 channel 0 and converts the binary data to the actual temperature and puts it in the database with the current date and hour.
GetTempGrafiek
This gets the past 20 values of the temperature sensor and emits it to the frontend.
tijd_sturen
This method gets the current time and checks if the minute has changed. If it has changed the program sends the new time to the arduino using serial communication
checkAlarmen
This is the most important method. It gets all the alarms that are turned on and checks if any of these alarms has to go of between now and 5 minutes ago (this is a buffer to make sure each alarm goes of when it needs to). If the alarm has to go off we'll start the music, the ledstrip,... We read the force sensitive resistor continuously and when the value drops below 1000 (read the fsr is pressed) We turn of the alarm and update the alarm in the database. We set the date that the alarm went off for the last time to the current date.
statusLight
This method emits the value of the ledstrip and turns the ledstrip on if needed.
lichtAanpassen
This an extra method to make sure the ledstrip and alarm light don't conflict.
Step 6: Case
The link to my files can be found here: https://www.thingiverse.com/thing:4461071
I used a 3d printer to print the casing. It's printed in 4 different parts:
- The front plate with holes for the speaker and some walls for the 7 segment display
- The outer ring for the ledstrip in transparant PETG.
- The middle part
- The backplate with a hole for the lcd and a hole for the cables.
In the original model there was no hole for the 7 segment display however this is needed because otherwise the light of the 7 segment display won't shine through.
As you can see after putting all the components in I used vinyl stickers with a wooden look to make the end result look better. The clock display shines throught the sticker which creates a very nice looking effect.
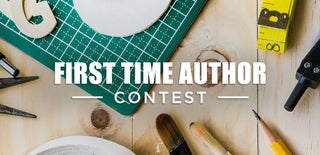
Participated in the
First Time Author Contest