Introduction: WiFi Enabled Arduino Over USB
This project uses Blynk, which is a service you can use to connect WiFi enabled things to a server. You can control devices like the Raspberry Pi or a specialized SparkFun Blynk Board from an app on your phone. The boards mentioned already have built in wireless capabilities, but the Arduino is not meant to do that - at least not without some help.
If you have never used Blynk before, this will be helpful: http://www.blynk.cc/getting-started/
Step 1: Install Necessary Software
- Download and install the Blynk library* for Arduino: https://github.com/blynkkk/blynk-library/releases...
- Download the Android or iOS app:
https://play.google.com/store/apps/details?id=cc.b...
https://itunes.apple.com/us/app/blynk-control-ardu...
* How to install a library: https://play.google.com/store/apps/details?id=cc.b...
Step 2: Set Up the App
- Open the Blynk app
- Create a new project
- Give it a cool name
- Select the board "Arduino UNO"
- Set the connection type to USB
- Tap "Create" - It should send you an email with your Auth token (you'll need this later)
- Click run
At the top it will probably say that your board is offline, we'll fix that in the next step.
Step 3: Upload and Run the Code
- Connect the Arduino board to your computer
- Navigate through the newly installed Blynk Library to find the Arduino code: Arduino > libraries > Blynk > examples > Boards_USB_Serial > Arduino_Serial_USB
- Change the "YourAuthToken" to the Auth token provided in the app:
char auth[] = "YourAuthToken";
- Once you change it, it might look like
char auth[] = "c3e7d746a05b4cah18723cbcb3631a97";
(I just made up some random stuff there - don't try using it for real)
Step 4: Run the Blynk Script
- With the Arduino code still going, run the .bat file located in: Arduino > libraries > Blynk > scripts. Type your serial port when it prompts you and press enter (Mine is COM3).
Open the app again and wait a little while. If it still doesn't come online, close the running .bat file and continue with the step below.
- Close the .bat file
- Edit blynk-ser.sh with wordpad (or something similar) located in: Arduino > libraries > Blynk > scripts - (you might need to change baud rate):
# === Edit default options to match your need === FROM_TYPE="SER" # SER, TCP TO_TYPE="SSL" # TCP, SSL COMM_BAUD=9600 SERV_ADDR=blynk-cloud.com ...
- Save the document and close it
- Run the .bat file again
If the app says everything is online, you can move on.
Step 5: Add a Button
We will use a virtual button on your phone to control what we just made:
- Press the (+) button at the top and select a button widget
- Set the output to V0
- Press Run
Now we have to tell the Arduino to do something when you press the button:
- Close the .bat file
- In the void setup() section, add:
pinMode(13, OUTPUT);
Now scroll down to the bottom of the code and add this below the void loop() section:
BLYNK_WRITE(V0) { int pinData = param.asInt(); digitalWrite(13,pinData); }
Upload the code again and run the .bat file. Now when you press the button it should turn on the onboard led.
Step 6: Going Further
If you want to do more than turn on an led, you can add these sections to your code to both send and receive data to your board:
How to send data (this is what you did before):
BLYNK_WRITE(V0) { int pinData = param.asInt(); }
How to recieve data:
- Make a value display in the app and set the input to V5.
- You can attach a button to the Arduino for example, and have the display tell you when the button is pressed by typing:
pinMode(2, INPUT);
in void setup(). Use the code below in void loop():
BLYNK_READ(V5) { int val = digitalRead(2); Blynk.virtualWrite(5, val); }
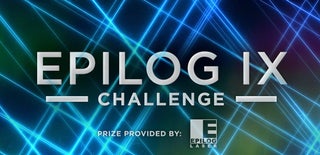
Participated in the
Epilog Challenge 9
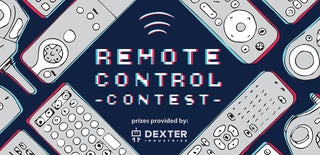
Participated in the
Remote Control Contest 2017
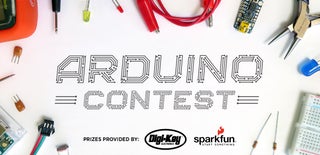
Participated in the
Arduino Contest 2017