Introduction: WiFi Enabled Temperature Controlled Smart Plug
In this instruction set we will be looking at how to build a WiFi enabled temperature probe with using a simple ESP8266 for the heavy lifting and a DHT11 temperature/humidity sensor. We will also be using the circuit board that I have created and is also on sale now in the channel’s tindie store if you would like to purchase.
Let’s get started with the bill of materials you will be needin:
ESP8266 WiFi Module
DHT11 Sensor
TP-Link Smart Plug
You can also pickup the entire module at the tindie store for the channel:
https://www.tindie.com/products/misperry/wifi-enab...
Also check out JLCPCB for PCB Fabriction. They are who I used to make the PCB:
Free Shipping on First Order & $2 PCB Prototyping on https://jlcpcb.com
Step 1: Adding the Code to ESP8266
We will now need to flash the following code onto the ESP8266. This code can be found at the following github repository: https://github.com/misperry/SpaceHeater_Thermostat
In the code below you will only have to set up the top few definition sections:
MQTT Server: <ip address of your mqtt server>
MQTT User: <user name of the mqtt server login account>
MQTT Password: <password for your mqtt server login>
MQTT_sensor_topic: <this has to match the topic defined in the Home assistant .yaml file>
—WiFi
Said: the said of the wifi network you are connecting to
Password: the WiFi password.
Once you have this filled out in the below code you can then compile and check for errors and if 0 errors you may flash it onto the ESP8266.
/*
* File Name: TempHumSensor.ino * * Application: HomeAssistant Space Heater Thermostat * * Description: This code is for the ESP8266 WiFi enabled arduino * compatible device. This will relay the temperature information * of the DHT11 device to the HASS frontend for processing. * * Author: M. Sperry - http://www.youtube.com/misperry * Date: 03/09/2018 * Revision: 1.0 * * */#include #include #include #include #include #include
#define CON_TIME_OUT 20 //Timeout of no connection to wifi #define MQTT_TIME_OUT 10 //Timeout of no connection to MQTT server
#define DHTPIN 0 //Pin which is connected to the DHT sensor #define DHTTYPE DHT11 //Type of sensor is the DHT11, you can change it to DHT22 (AM2302), DHT21 (AM2301)
#define mqtt_server "" // Enter your MQTT server adderss or IP. I use my DuckDNS adddress (yourname.duckdns.org) in this field #define mqtt_user "" //enter your MQTT username #define mqtt_password "" //enter your password #define MQTT_SENSOR_TOPIC "ha/bedroom_temp" //Enter topic for your MQTT
// Wifi: SSID and password const char* ssid = ""; const char* password = "";
//DHT SEtup DHT_Unified dht(DHTPIN, DHTTYPE); uint32_t delayMS;
WiFiClient wifiClient; PubSubClient client(wifiClient);
// function called to publish the temperature and the humidity void publishData(float p_temperature) { // create a JSON object // doc : https://github.com/bblanchon/ArduinoJson/wiki/API%20Reference StaticJsonBuffer<200> jsonBuffer; JsonObject& root = jsonBuffer.createObject(); // INFO: the data must be converted into a string; a problem occurs when using floats... //convert to fahrenheit p_temperature = (p_temperature * 1.8) + 32; // convert to fahrenheit root["temperature"] = (String)p_temperature; root.prettyPrintTo(Serial); Serial.println("");
char data[200]; root.printTo(data, root.measureLength() + 1); client.publish(MQTT_SENSOR_TOPIC, data, true); }
// function called when a MQTT message arrived void callback(char* p_topic, byte* p_payload, unsigned int p_length) { }
void reconnect() { // Loop until we're reconnected while (!client.connected()) { Serial.print("INFO: Attempting MQTT connection..."); // Attempt to connect if (client.connect("ESPBlindstl", mqtt_user, mqtt_password)) { Serial.println("INFO: connected"); } else { Serial.print("ERROR: failed, rc="); Serial.print(client.state()); Serial.println("DEBUG: try again in 5 seconds"); // Wait 5 seconds before retrying delay(5000); } } }
void setup(void) {
Serial.begin(9600);
// We start by connecting to a WiFi network Serial.println(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) { delay(800); Serial.print("."); }
Serial.println(""); Serial.println("WiFi connected"); Serial.println("IP address: "); Serial.println(WiFi.localIP()); // init the MQTT connection client.setServer(mqtt_server, 1883); client.setCallback(callback);
// Initialize DHT sensor dht.begin(); Serial.println("DHT11 Unified Sensor Data");
//Print temp sensor details sensor_t sensor; dht.temperature().getSensor(&sensor); Serial.println("------------------------------------"); Serial.println("Temperature"); Serial.print ("Sensor: "); Serial.println(sensor.name); Serial.print ("Driver Ver: "); Serial.println(sensor.version); Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id); Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" *C"); Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" *C"); Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" *C"); Serial.println("------------------------------------"); // Print humidity sensor details. dht.humidity().getSensor(&sensor); Serial.println("------------------------------------"); Serial.println("Humidity"); Serial.print ("Sensor: "); Serial.println(sensor.name); Serial.print ("Driver Ver: "); Serial.println(sensor.version); Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id); Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println("%"); Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println("%"); Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println("%"); Serial.println("------------------------------------");
//Set delay between sensor readings based on sensor details delayMS = sensor.min_delay / 1000; }
void loop(void) {
float temperature;
if (!client.connected()) { reconnect(); }
delay(delayMS);
// Get temperature event and print its value. sensors_event_t event; dht.temperature().getEvent(&event); if (isnan(event.temperature)) { Serial.println("Error reading temperature!"); temperature = 0.00; } else { temperature = event.temperature; Serial.print("Temperature: "); Serial.print(temperature); Serial.println(" *C"); } // publish to MQTT publishData(temperature); }
Step 2: Setting Up Your TP-LINK Smart Plug
You will need to set u your TP-LINK smart plug, or any smart plug for that mater, the way that the manufacturer recommends.
Make sure to take note of the MAC address on the device. If your device is like my TP-LINK device you cannot st a static IP address. Thus, you will need to configure your router for DHCP Reservation. This will take the MAC address of your device and when that device requests for an address the router will give it the same address every time.
Here is a link to how to set this up with Linksys
Step 3: Setting Up Home Assistant
Now to set up Home Assistant. For this you will need to add the following configurations to the configuration.yaml file that is located in the /home/homeassistant/.homeassistant folder structure on the device you installed it to.
Once finished adding this to your home assistant configuration you will need to restart your home assistant software for the changes to take affect.
Also I will be using the TP-LINK smart plug fort the switching device and the definition is below in the configuration file. The IP address that is used for the device is the one that you set up for the DHCP reservation in the previous step.
This configuration can also be found at the following github repo: https://github.com/misperry/SpaceHeater_Thermosta...
<br><p>mqtt:<br>switch: - platform: tplink name: Bedroom Heater host: 192.168.2.11</p><p>sensor 1: platform: mqtt state_topic: 'ha/bedroom_temp' name: Bedroom Temp unit_of_measurement: '°F' value_template: '{{ value_json.temperature }}'</p><p>automation: - alias: _Temp Bedroom Temp High trigger: - platform: numeric_state entity_id: sensor.Bedroom_Temp above: 73 </p><p> action: service: homeassistant.turn_off entity_id: switch.Bedroom_Heater</p><p> - alias: _Temp Bedroom Temp Low trigger: - platform: numeric_state entity_id: sensor.Bedroom_Temp below: 73 action: service: homeassistant.turn_on entity_id: switch.Bedroom_Heater</p>
Step 4: Final Steps
Now with your home assistant configuration and your Arduino code set up you will be ready to activate the whole system. Thus, place your heater/fan/cooler plug into the smart plug and plug In the smart plug. Once it is ready you will need to plug in a small USB charger and then the WiFi enabled Temperature probe. Once all is online you should be able to look in your home assistant dashboard and see the new temperature being reported.
Thank you very much for all your help and support. make sure to leave a like and come visit the channel at http://www.youbue.com/misperry and see what all we have there for you. Make sure you subscribe and share with your friends to help out the channel.
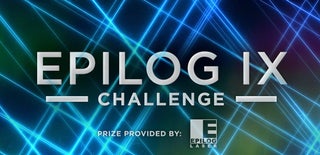
Participated in the
Epilog Challenge 9